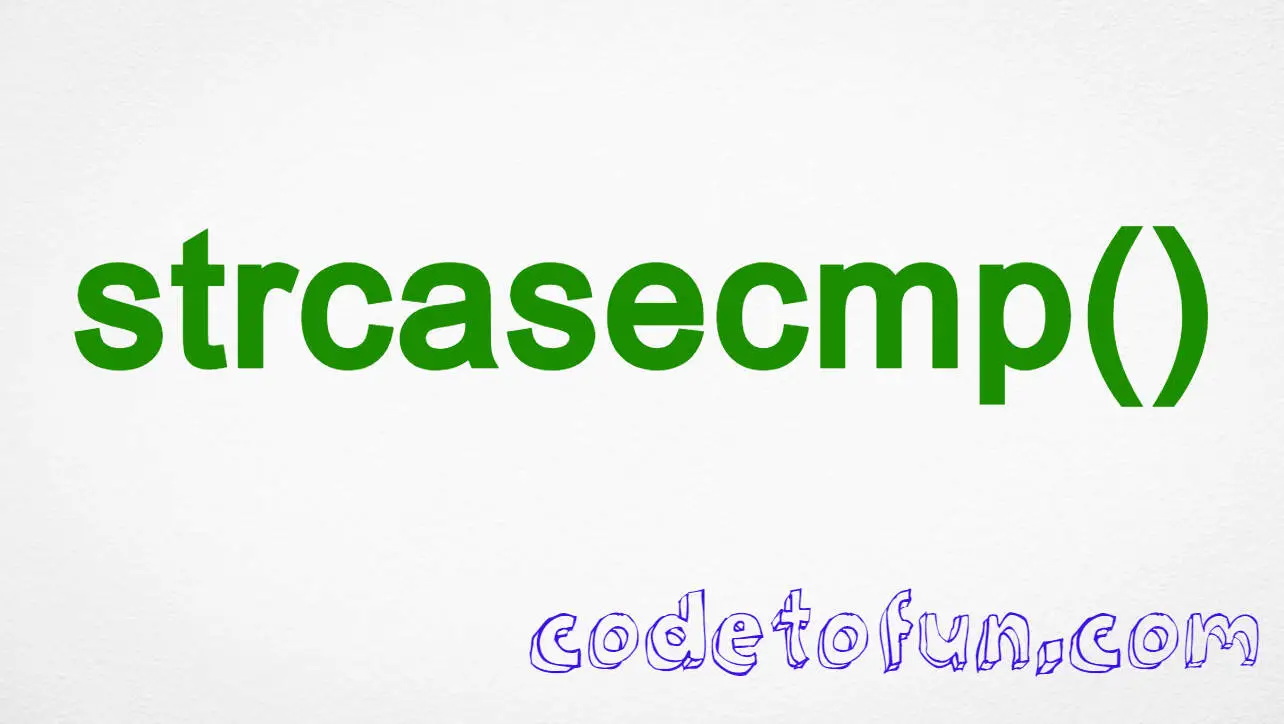
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Check Strong Number
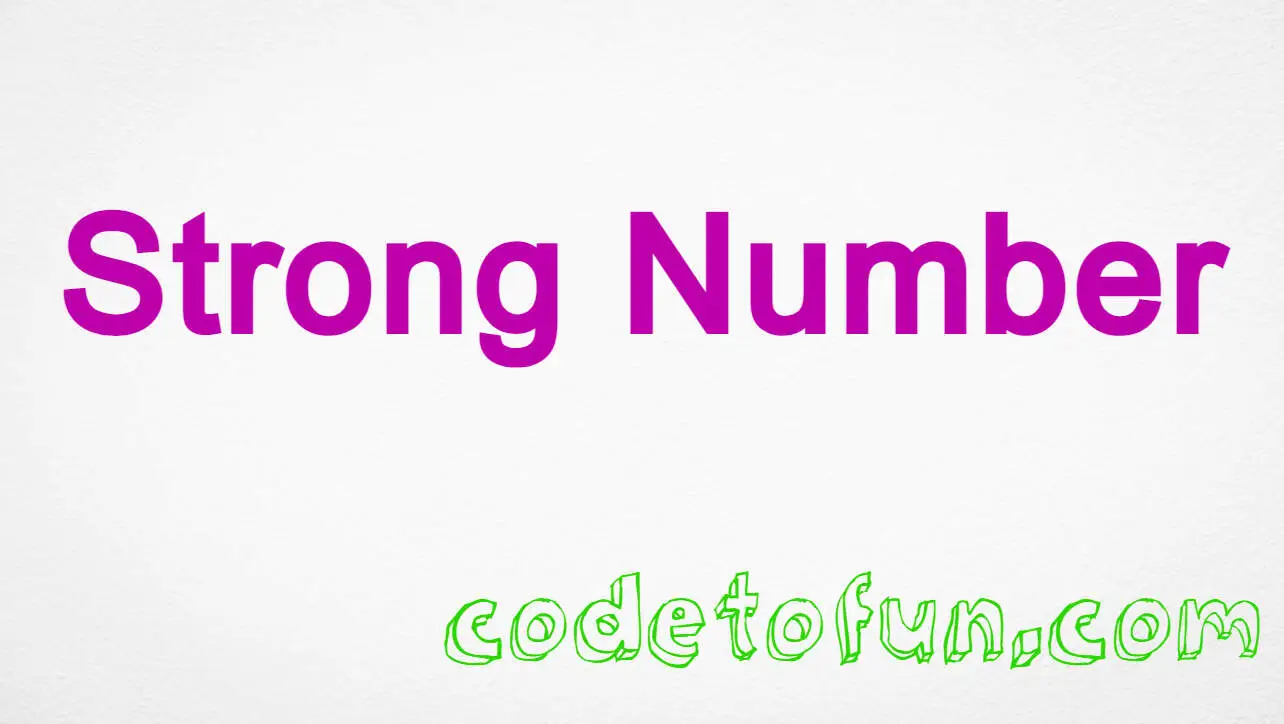
Photo Credit to CodeToFun
π Introduction
In the world of programming, exploring number properties is a fascinating journey. One such property is related to Strong Numbers.
A Strong Number (also known as a Digital Factorial) is a number in which the sum of the factorials of its digits is equal to the number itself.
In this tutorial, we'll delve into a C program that checks whether a given number is a Strong Number or not.
π Example
Let's take a look at the C code that checks whether a given number is a Strong Number.
#include <stdio.h>
// Function to calculate the factorial of a number
int factorial(int num) {
if (num == 0 || num == 1) {
return 1;
} else {
return num * factorial(num - 1);
}
}
// Function to check if a number is a Strong Number
int isStrongNumber(int num) {
int originalNum = num;
int sum = 0;
while (num > 0) {
int digit = num % 10;
sum += factorial(digit);
num /= 10;
}
return (sum == originalNum);
}
// Driver program
int main() {
// Replace this value with your desired number
int number = 145;
// Call the function to check if the number is a Strong Number
if (isStrongNumber(number)) {
printf("%d is a Strong Number.\n", number);
} else {
printf("%d is not a Strong Number.\n", number);
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the main function.
145 is a Strong Number.
Compile and run the program to check if the number is a Strong Number.
π§ How the Program Works
- The program defines a function factorial to calculate the factorial of a number.
- Another function isStrongNumber is defined to check if a given number is a Strong Number.
- Inside the isStrongNumber function, it iterates through each digit of the number, calculates the factorial, and adds it to the sum.
- The result is compared with the original number to determine if it is a Strong Number.
π Between the Given Range
Let's take a look at the C code that checks for strong numbers in the range of 1 to 200.
#include <stdio.h>
// Function to calculate the factorial of a number
int factorial(int num) {
if (num == 0 || num == 1) {
return 1;
} else {
return num * factorial(num - 1);
}
}
// Function to check if a number is strong
int isStrongNumber(int num) {
int originalNum = num;
int sum = 0;
while (num != 0) {
int digit = num % 10;
sum += factorial(digit);
num /= 10;
}
return (sum == originalNum);
}
// Driver program
int main() {
printf("Strong Numbers in the Range 1 to 200:\n");
for (int i = 1; i <= 200; ++i) {
if (isStrongNumber(i)) {
printf("%d ", i);
}
}
printf("\n");
return 0;
}
π» Testing the Program
Strong Numbers in the Range 1 to 200: 1 2 145
Run the program to see the strong numbers in the range of 1 to 200.
π§ How the Program Works
- The program defines a function factorial to calculate the factorial of a given number.
- It also defines a function isStrongNumber to check if a number is a strong number.
- Inside the main function, it iterates through numbers from 1 to 200 and checks if each number is a strong number using the isStrongNumber function.
- The strong numbers found in the range are printed.
π§ Understanding the Concept of Strong Number
To determine if a number is a Strong Number, we need to calculate the factorial of each digit of the number and then sum these factorials. If the sum is equal to the original number, then it is a Strong Number.
For example, let's consider the number 145:
- The factorial of 1 is 1.
- The factorial of 4 is 24.
- The factorial of 5 is 120.
The sum of these factorials is 1 + 24 + 120 = 145, which is the original number. Hence, 145 is a Strong Number.
π Conclusion
Understanding and implementing programs that check for mathematical properties, such as Strong Numbers, enhances problem-solving skills in programming. Feel free to modify and expand upon this code for your specific needs. Happy coding!
π¨βπ» Join our Community:
Author
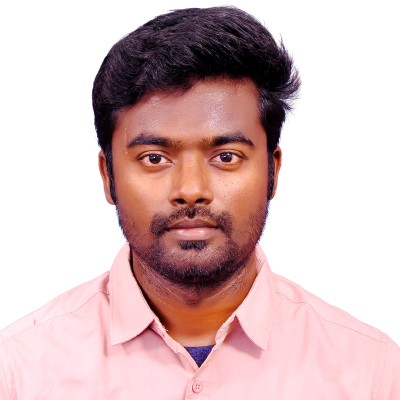
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
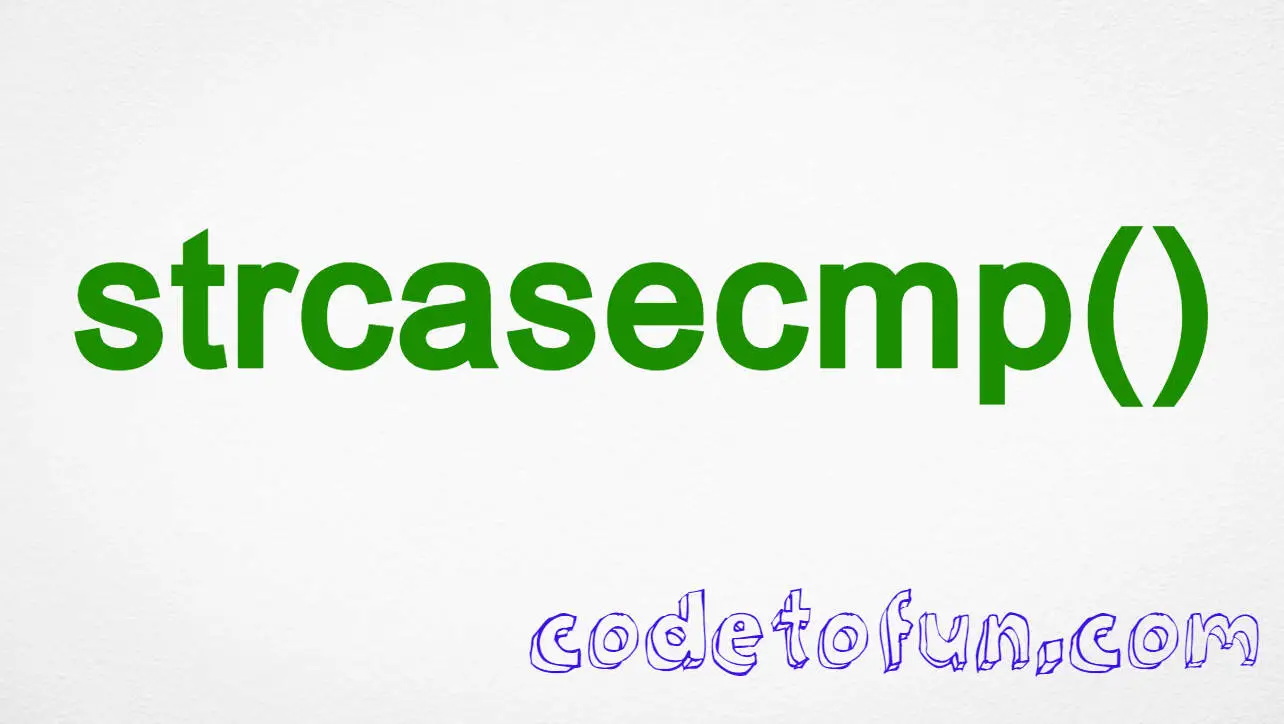
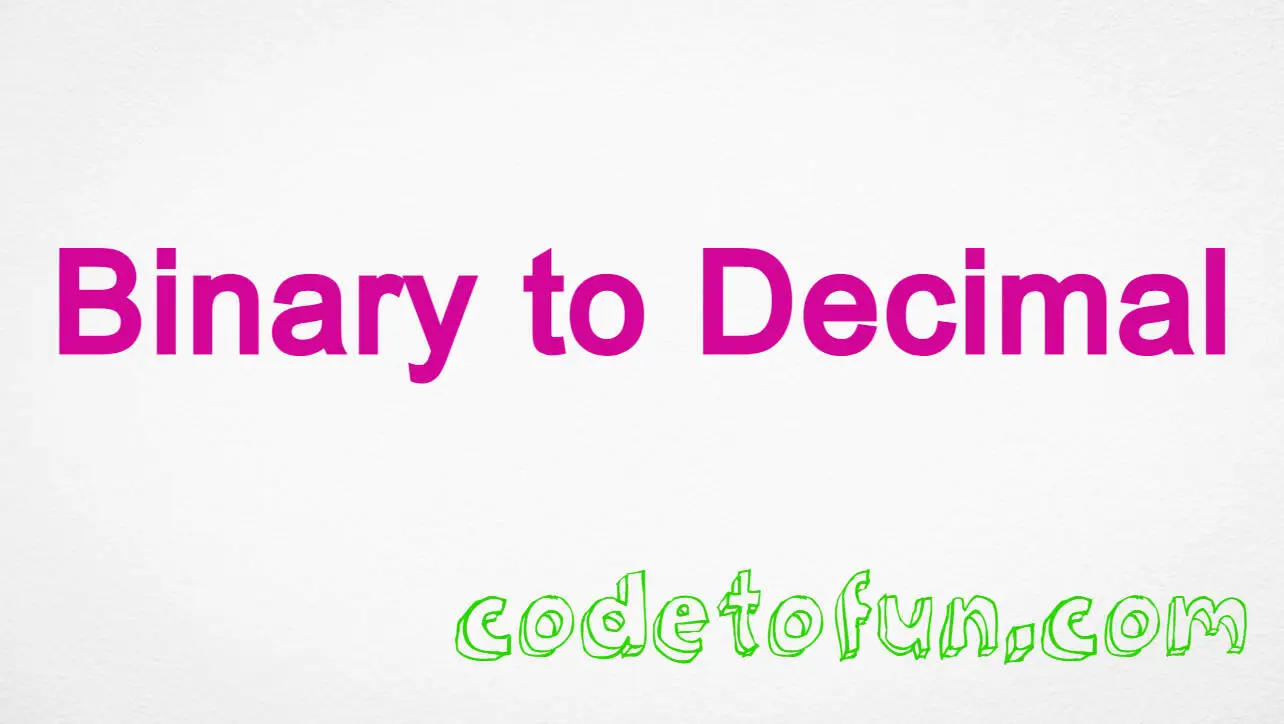
C Program to Converter a Binary to Decimal
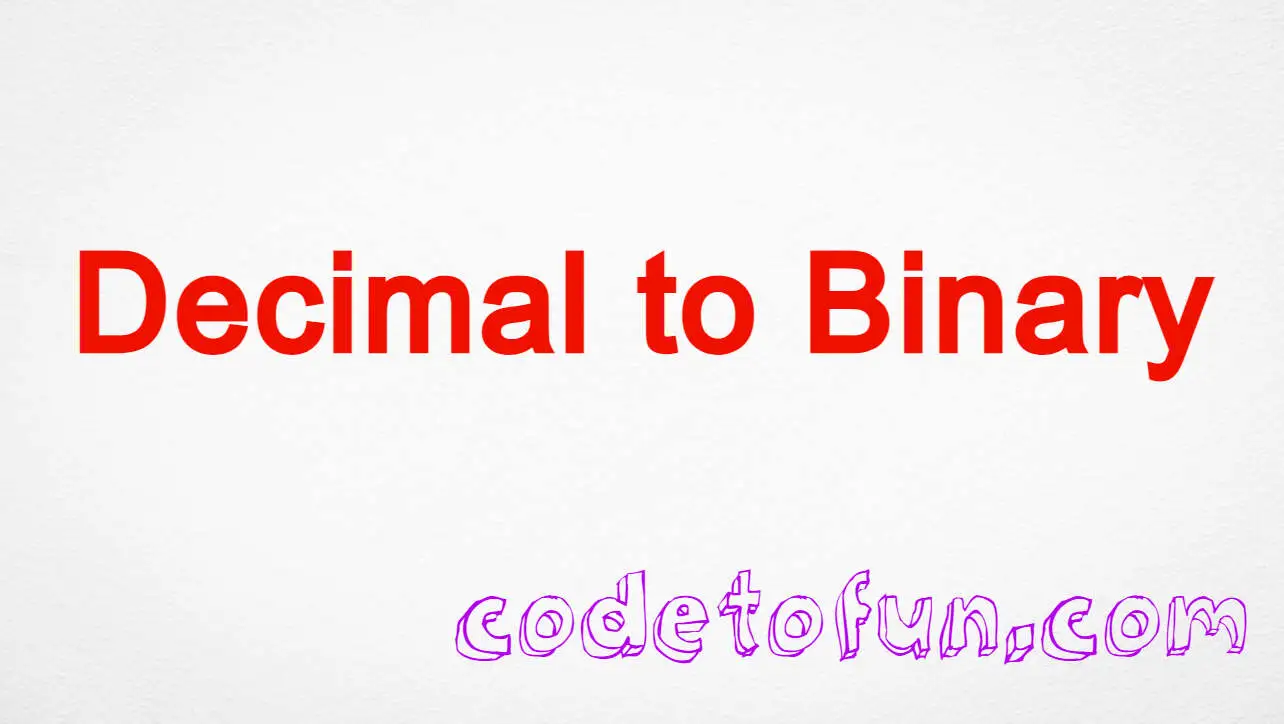
C Program to Converter a Decimal to Binary
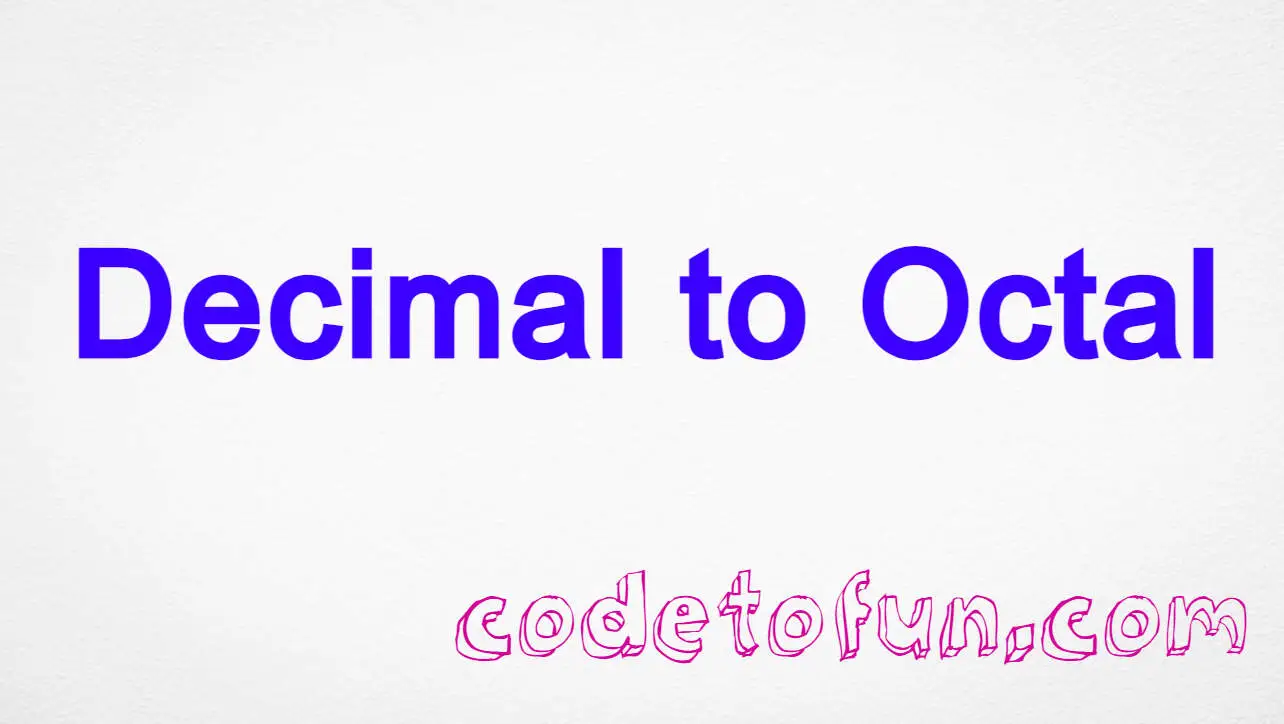
C Program to Converter a Decimal to Octal
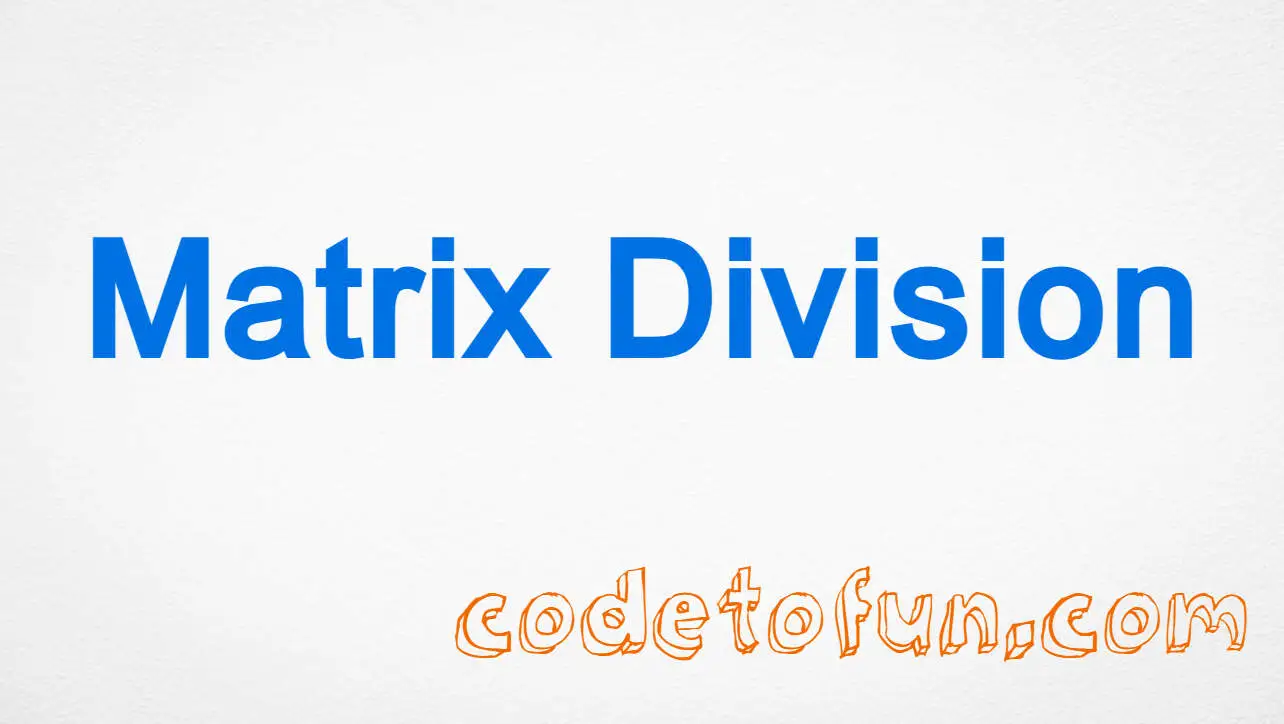
C Program to Perform Matrix Division
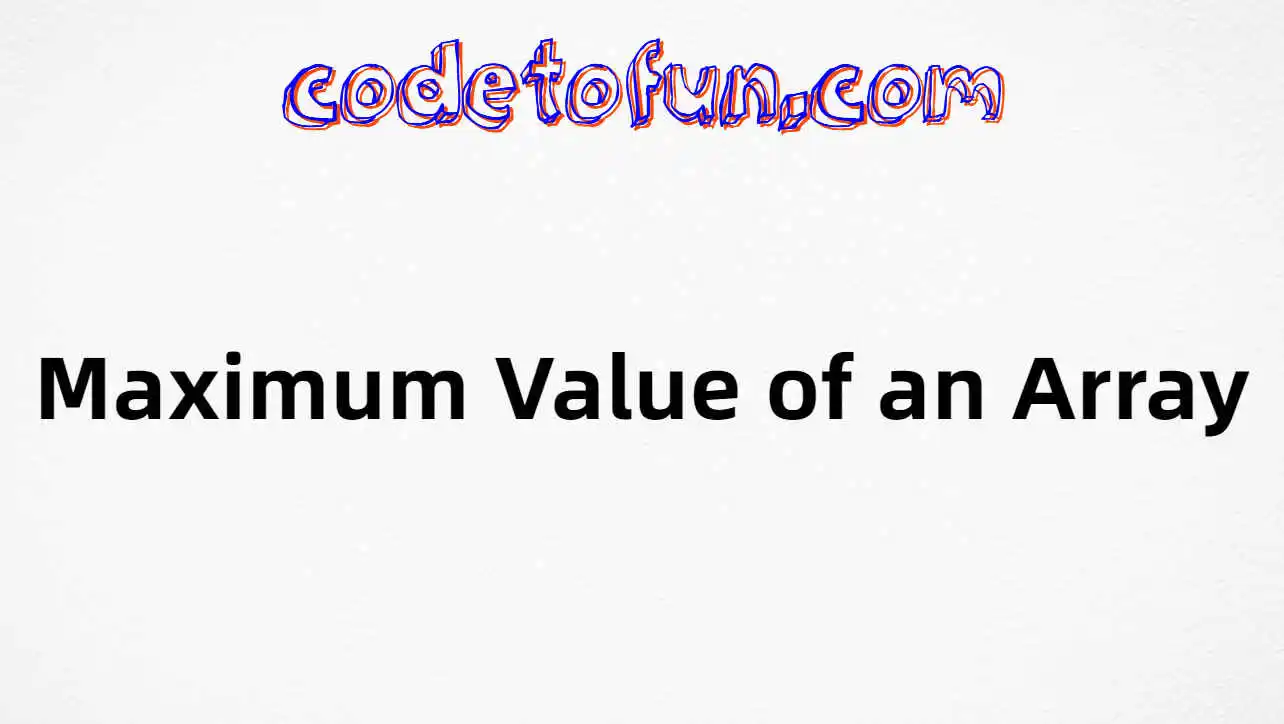
C Program to find Maximum Value of an Array
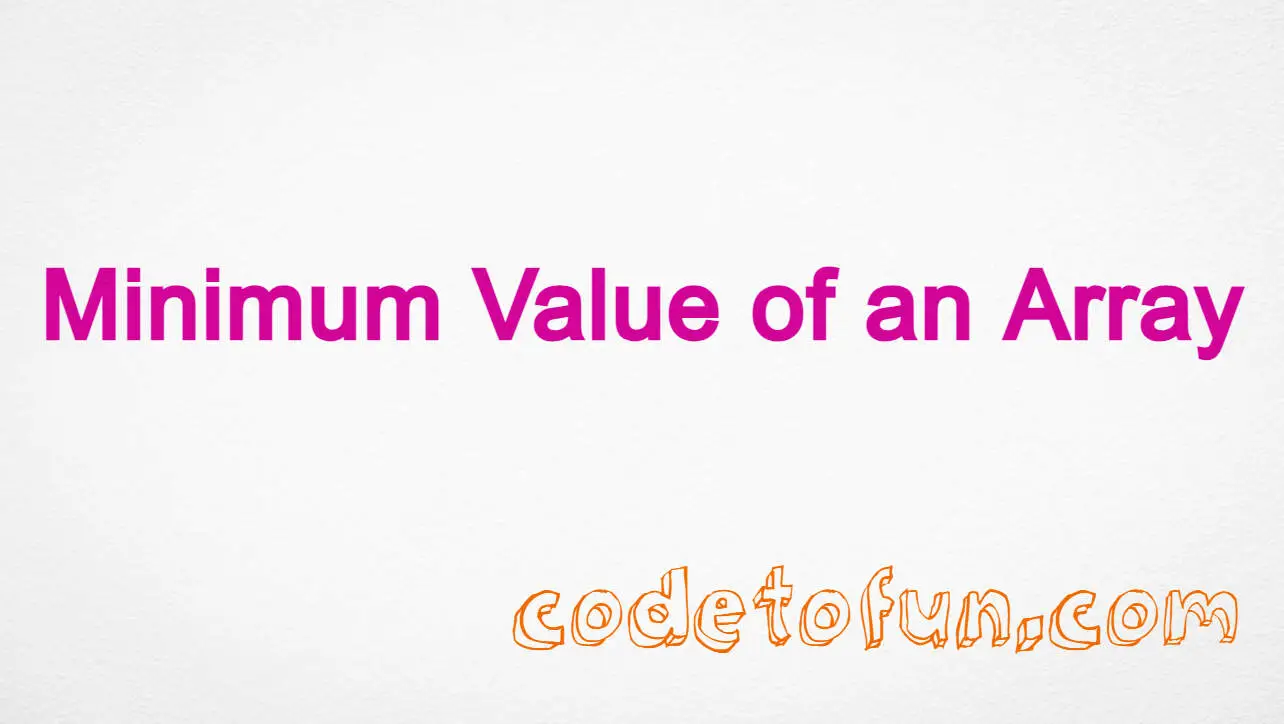
If you have any doubts regarding this article (C Program to Check Strong Number), please comment here. I will help you immediately.