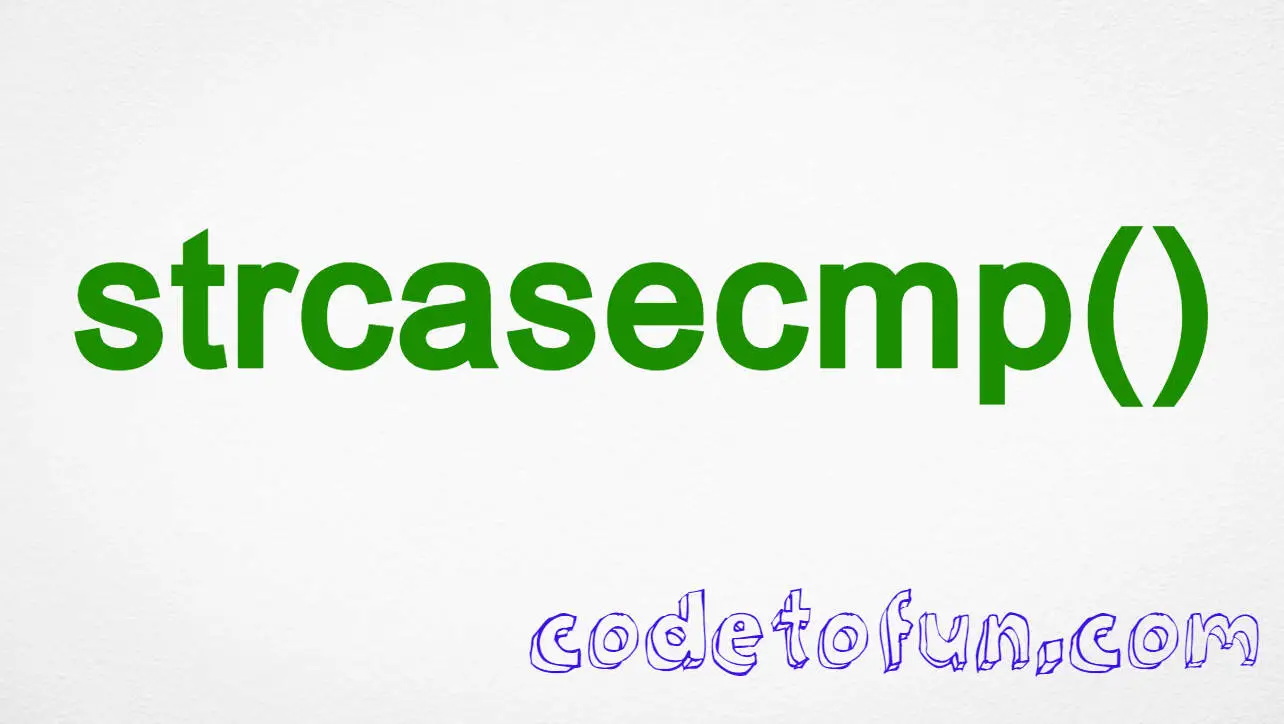
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Check Power of 2
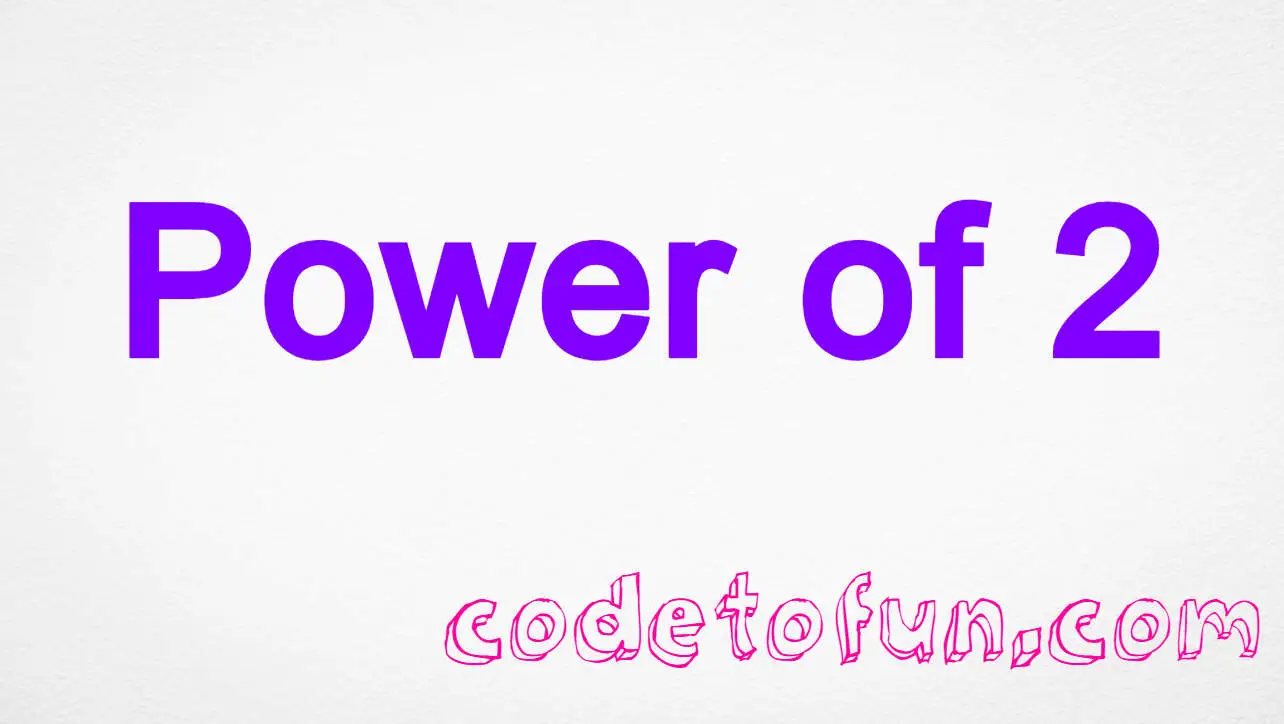
Photo Credit to CodeToFun
π Introduction
In the realm of programming, efficiency and optimization are key considerations. One common optimization involves determining whether a given number is a power of 2.
Checking if a number is a power of 2 is a fundamental operation with applications in various domains.
In this tutorial, we'll explore a C program that efficiently checks whether a given number is a power of 2.
π Example
Let's delve into the C code that accomplishes this functionality.
#include <stdio.h>
// Function to check if a number is a power of 2
int isPowerOfTwo(int num) {
// A number is a power of 2 if and only if it has a single set bit.
// Using bitwise AND operation to check this condition.
return (num > 0) && ((num & (num - 1)) == 0);
}
// Driver program
int main() {
// Replace this value with your desired number
int number = 16;
// Check if the number is a power of 2
if (isPowerOfTwo(number)) {
printf("%d is a power of 2.\n", number);
} else {
printf("%d is not a power of 2.\n", number);
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the main function.
16 is a power of 2.
Compile and run the program to see whether the given number is a power of 2.
π§ How the Program Works
- The program defines a function isPowerOfTwo that takes an integer as input and returns 1 if the number is a power of 2 and 0 otherwise.
- Inside the function, it uses a bitwise AND operation to check if the number has a single set bit, which is a characteristic of power-of-2 numbers.
- The main function demonstrates the usage of the isPowerOfTwo function by checking if a given number is a power of 2.
π Between the Given Range
Let's dive into the C code that performs the check for powers of 2 in the range 1 to 20.
#include <stdio.h>
#include <math.h>
// Function to check if a number is a power of 2
int isPowerOfTwo(int num) {
return (num && !(num & (num - 1)));
}
// Driver program
int main() {
printf("Power of 2 in the range 1 to 20:\n");
for (int i = 1; i <= 20; ++i) {
if (isPowerOfTwo(i)) {
printf("%d ", i);
}
}
printf("\n");
return 0;
}
π» Testing the Program
The output of the program, showing powers of 2 in the range from 1 to 20, will be displayed in the following format:
Power of 2 in the range 1 to 20: 1 2 4 8 16
π§ How the Program Works
- The program defines a function isPowerOfTwo that checks whether a given number is a power of 2 using bitwise operations.
- The main function iterates through numbers from 1 to 20 and prints those that are powers of 2.
π§ Understanding the Concept of Power of 2
A number is considered a power of 2 if and only if it has a single set bit.
For example, 2, 4, 8, and 16 are powers of 2 because their binary representations have only one bit set.
Understanding this concept is crucial for efficient programming and algorithm design.
π’ Optimizing the Program
The provided program is a straightforward implementation. However, there are more advanced techniques for checking if a number is a power of 2, such as using logarithmic operations or counting the number of set bits. Depending on your specific use case, you might explore these optimizations.
Feel free to incorporate and modify this code as needed for your specific requirements. Happy coding!
π¨βπ» Join our Community:
Author
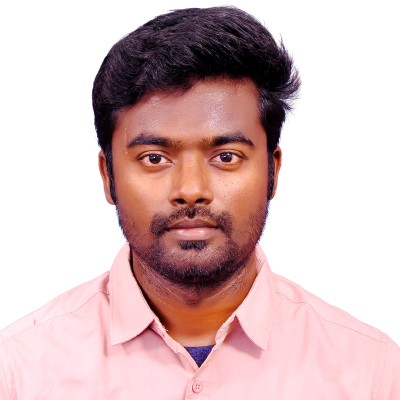
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
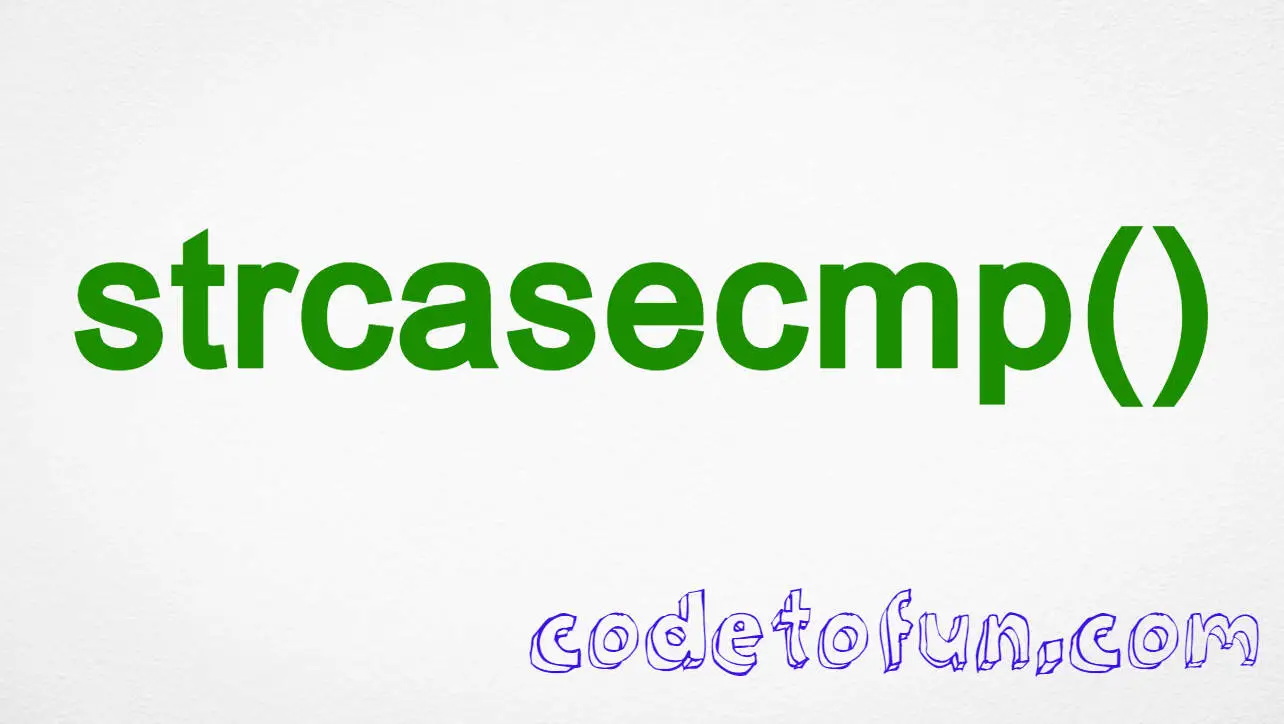
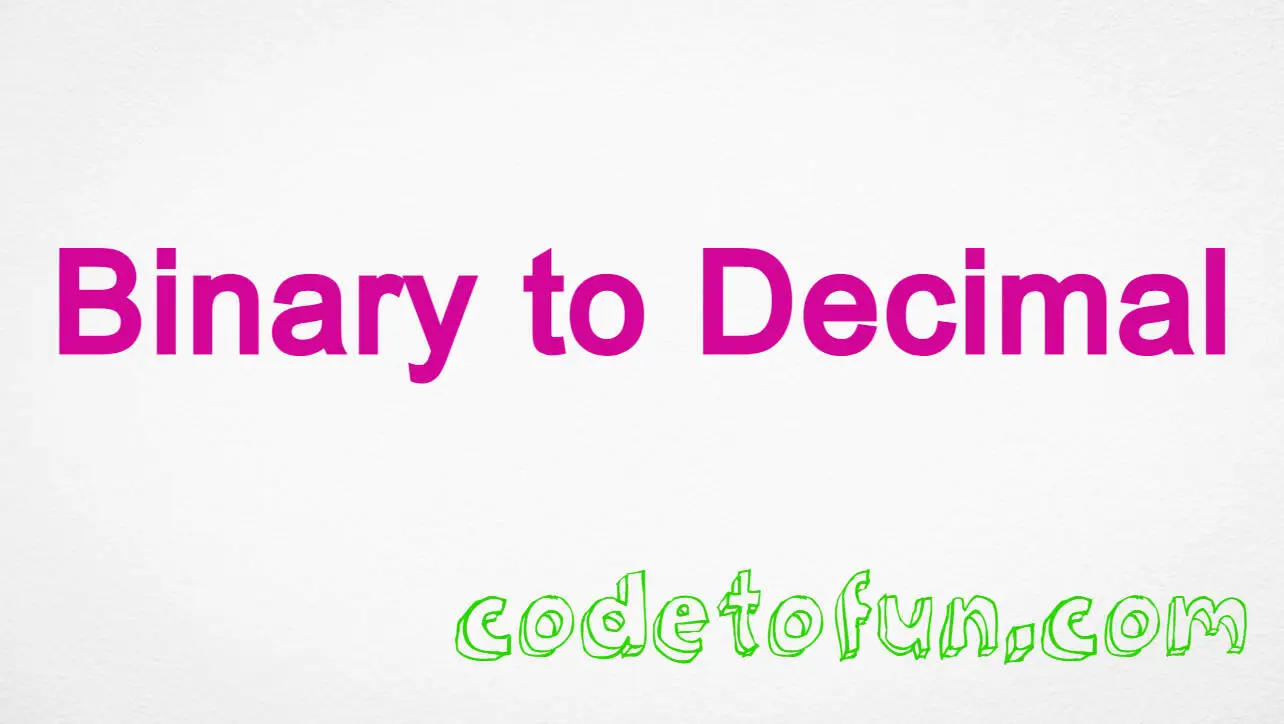
C Program to Converter a Binary to Decimal
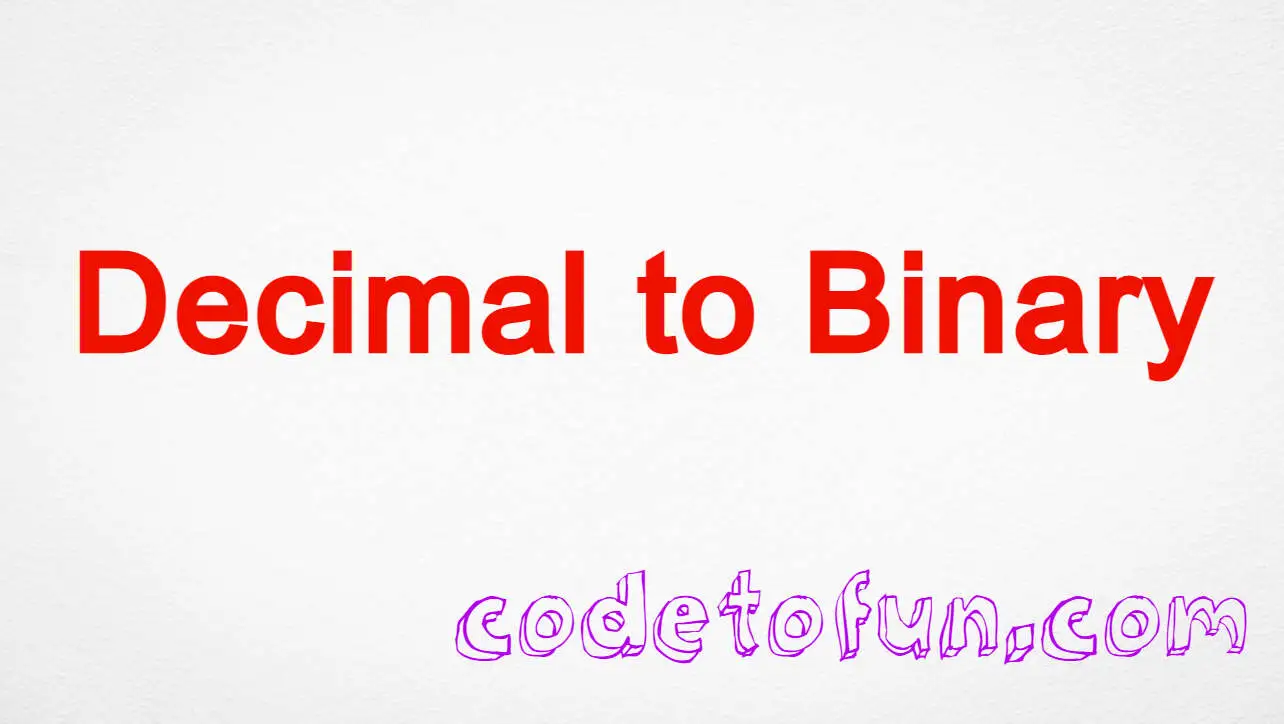
C Program to Converter a Decimal to Binary
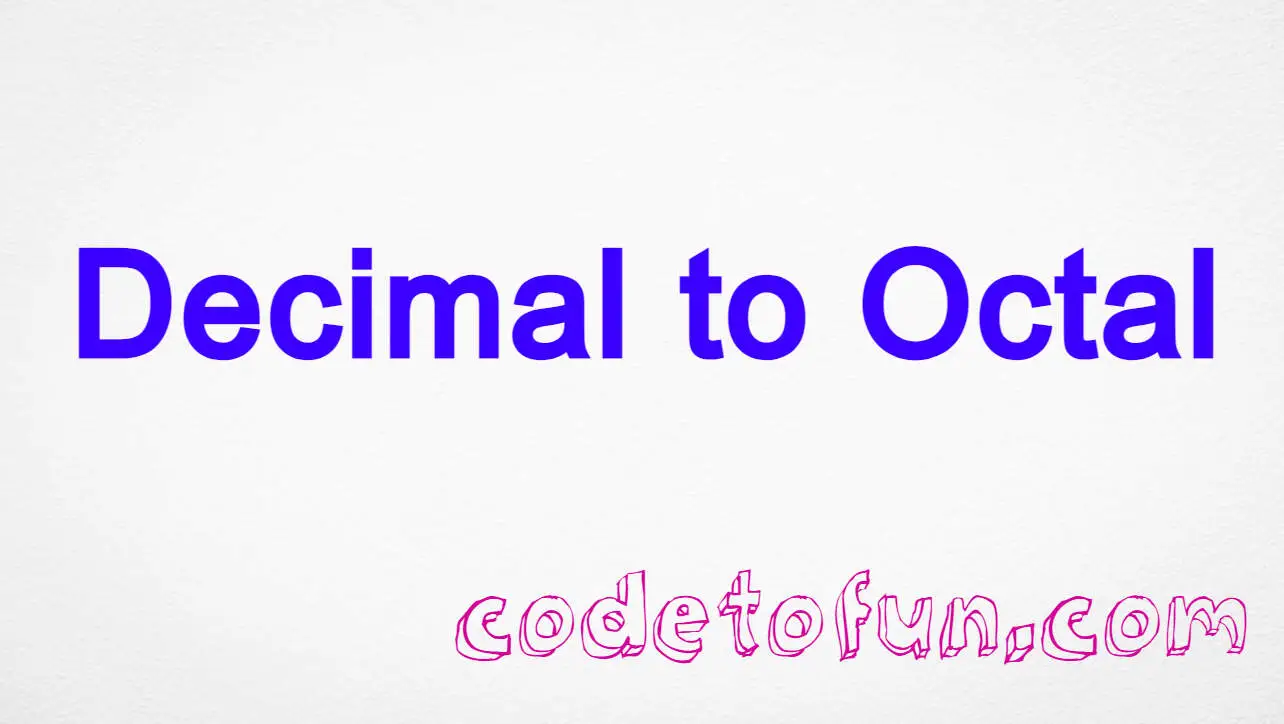
C Program to Converter a Decimal to Octal
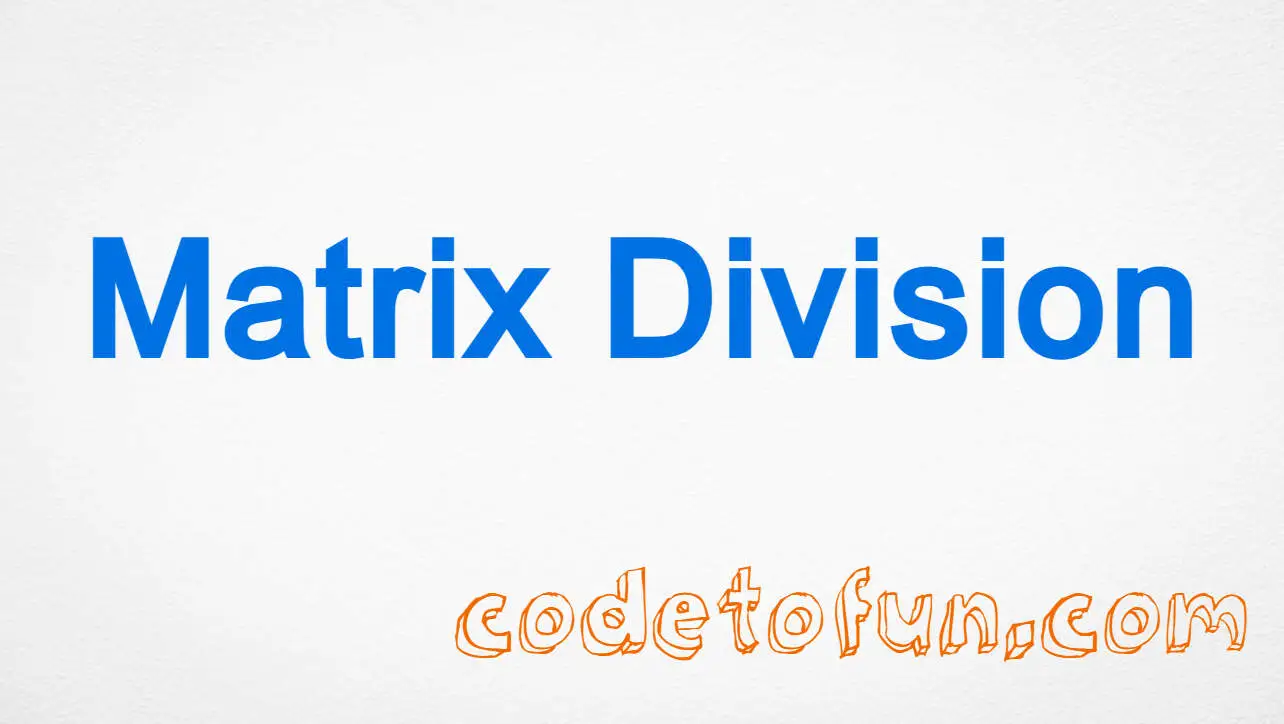
C Program to Perform Matrix Division
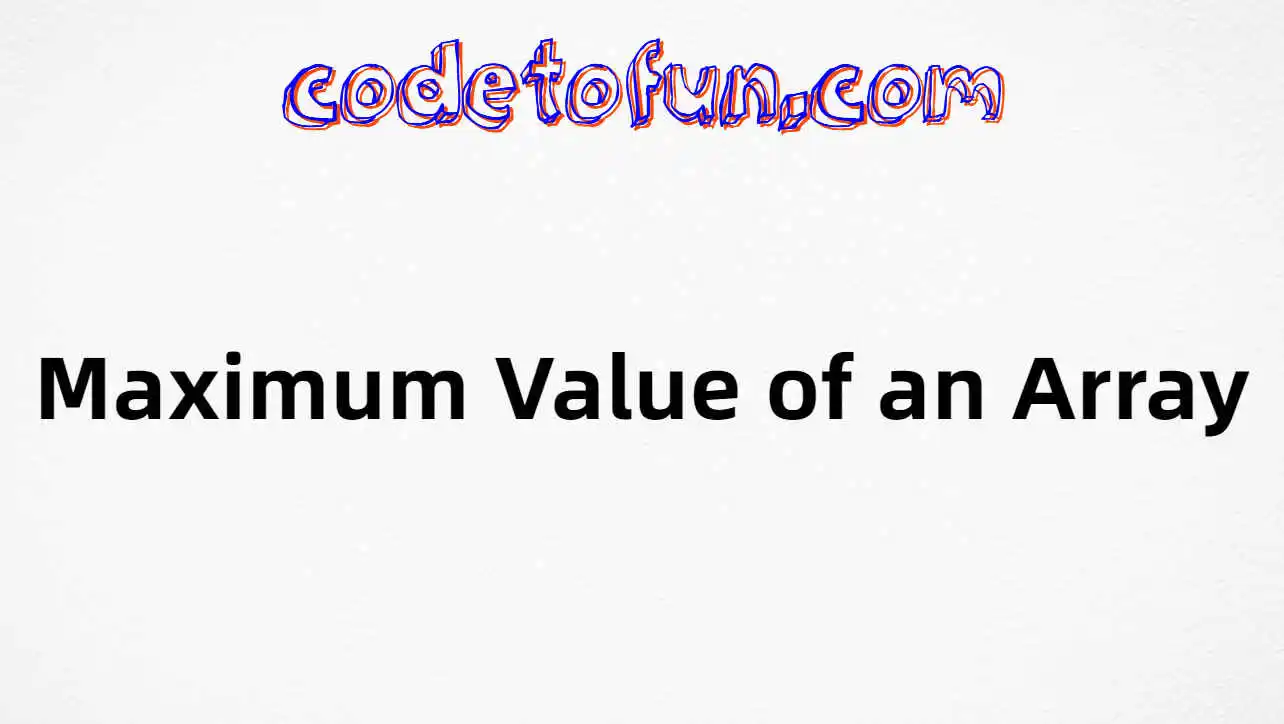
C Program to find Maximum Value of an Array
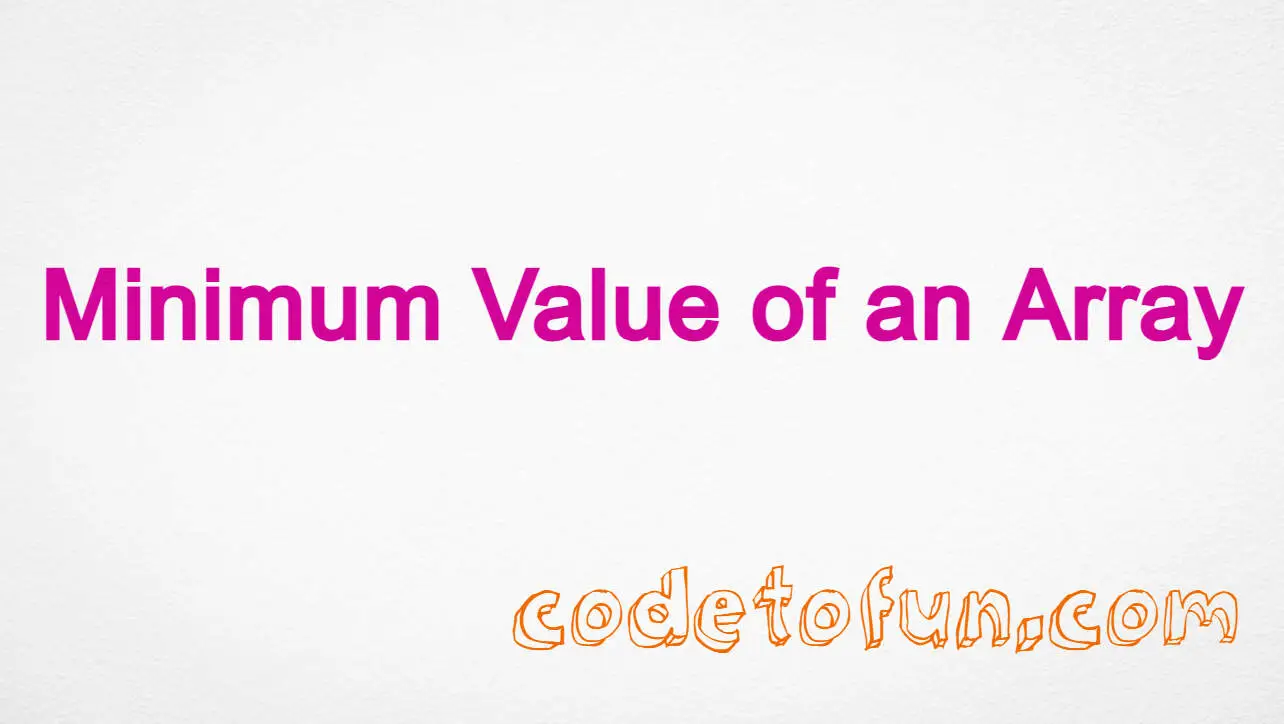
If you have any doubts regarding this article (C Program to Check Power of 2), please comment here. I will help you immediately.