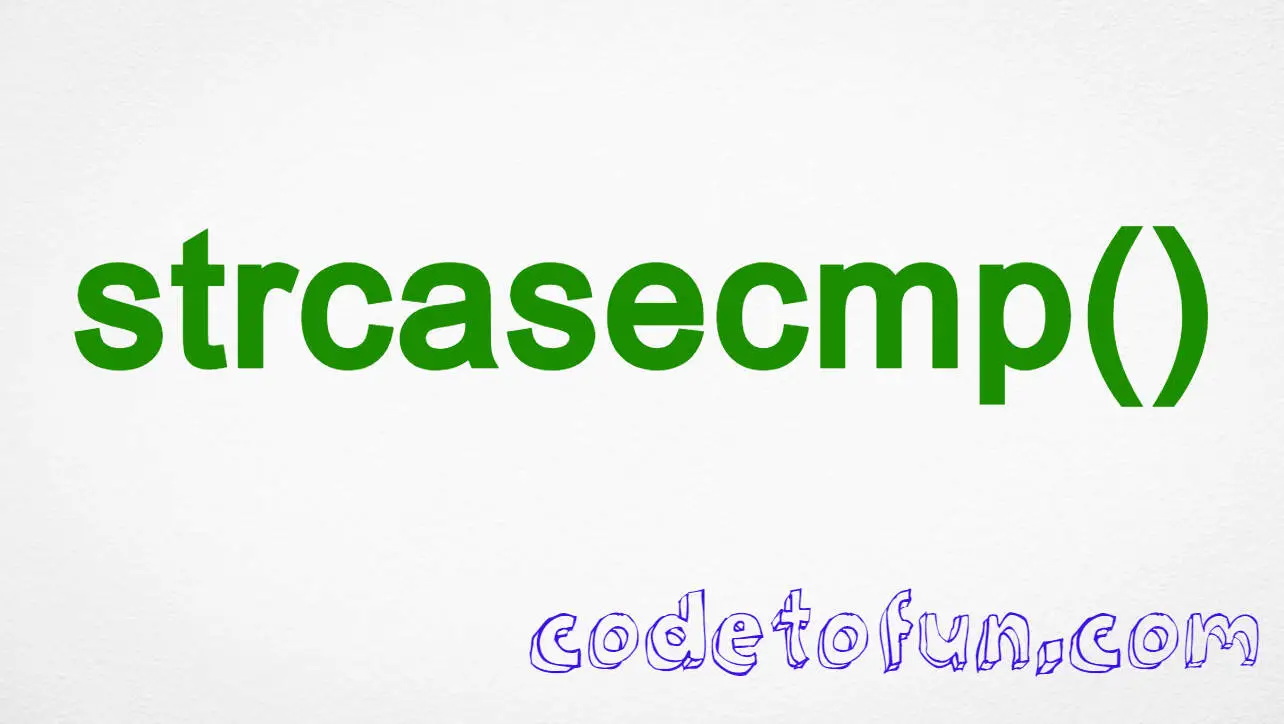
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Perform Matrix Multiplication
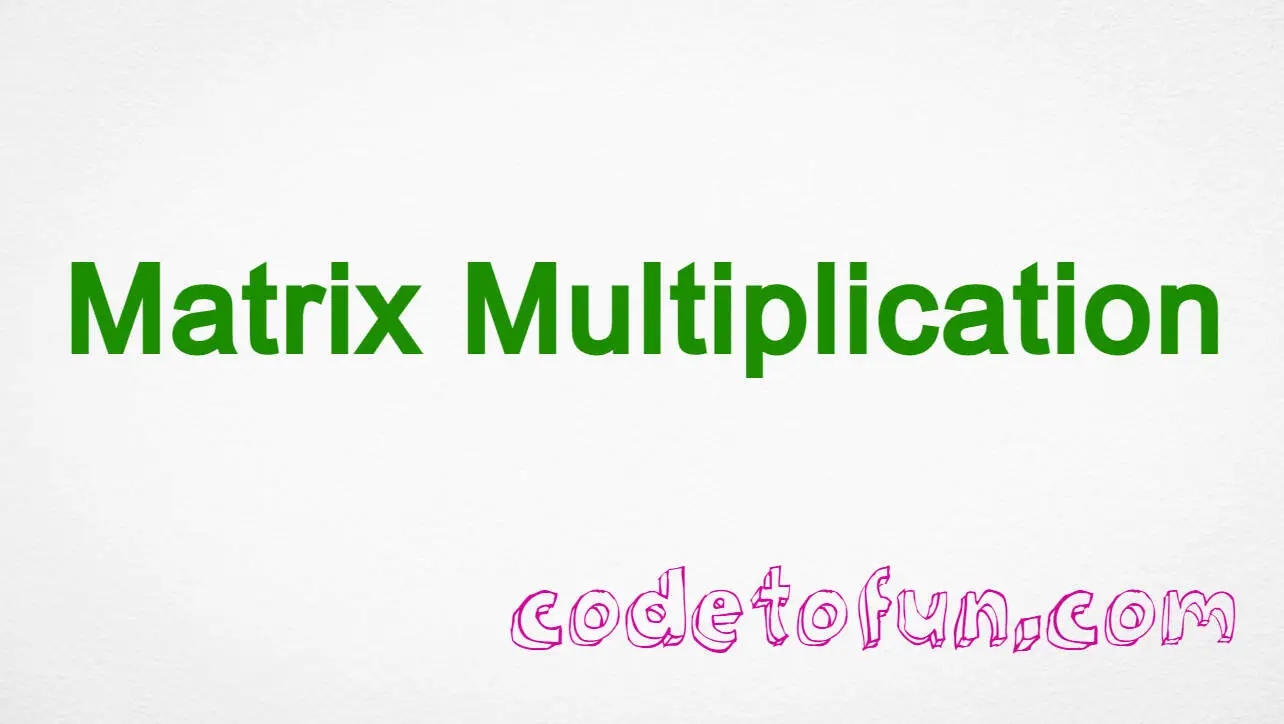
Photo Credit to CodeToFun
π Introduction
Matrix multiplication is a fundamental operation in linear algebra and computer science. It involves multiplying two matrices to produce a third matrix.
In this tutorial, we will explore a C program that performs matrix multiplication.
We'll break down the logic step by step and provide a sample implementation.
π Example
Let's dive into the C code that performs matrix multiplication.
#include <stdio.h>
#define MAX_SIZE 3
// Function to perform matrix multiplication
void multiplyMatrices(int firstMatrix[MAX_SIZE][MAX_SIZE], int secondMatrix[MAX_SIZE][MAX_SIZE], int result[MAX_SIZE][MAX_SIZE]) {
int i, j, k;
// Initialize the result matrix with zeros
for (i = 0; i < MAX_SIZE; ++i) {
for (j = 0; j < MAX_SIZE; ++j) {
result[i][j] = 0;
}
}
// Perform matrix multiplication
for (i = 0; i < MAX_SIZE; ++i) {
for (j = 0; j < MAX_SIZE; ++j) {
for (k = 0; k < MAX_SIZE; ++k) {
result[i][j] += firstMatrix[i][k] * secondMatrix[k][j];
}
}
}
}
// Function to display a matrix
void displayMatrix(int matrix[MAX_SIZE][MAX_SIZE]) {
int i, j;
for (i = 0; i < MAX_SIZE; ++i) {
for (j = 0; j < MAX_SIZE; ++j) {
printf("%d\t", matrix[i][j]);
}
printf("\n");
}
}
// Driver program
int main() {
int firstMatrix[MAX_SIZE][MAX_SIZE] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int secondMatrix[MAX_SIZE][MAX_SIZE] = {
{9, 8, 7},
{6, 5, 4},
{3, 2, 1}
};
int result[MAX_SIZE][MAX_SIZE];
// Call the function to perform matrix multiplication
multiplyMatrices(firstMatrix, secondMatrix, result);
// Display the matrices
printf("First Matrix:\n");
displayMatrix(firstMatrix);
printf("\nSecond Matrix:\n");
displayMatrix(secondMatrix);
printf("\nResult Matrix:\n");
displayMatrix(result);
return 0;
}
π» Testing the Program
Feel free to replace the sample matrices with your own 3x3 matrices in the main function to test the program with different input.
First Matrix: 1 2 3 4 5 6 7 8 9 Second Matrix: 9 8 7 6 5 4 3 2 1 Result Matrix: 30 24 18 84 69 54 138 114 90
Compiler and run the program to see the result of the matrix multiplication.
π§ How the Program Works
- The program defines a function multiplyMatrices that takes two matrices (firstMatrix and secondMatrix) and computes their product, storing the result in the result matrix.
- It initializes the result matrix with zeros and uses three nested loops to perform the matrix multiplication.
- The displayMatrix function is used to display the contents of a matrix.
- In the main function, sample input matrices (firstMatrix and secondMatrix) are provided, and the program calls the multiplication function.
- The program then displays the original matrices and the result matrix.
π§ Understanding the Concept of Matrix Multiplication
Matrix multiplication is defined as follows: given two matrices A (of dimensions m x n) and B (of dimensions n x p), the product matrix C (of dimensions m x p) is obtained by multiplying each element of a row in matrix A by the corresponding element of a column in matrix B and summing up the results.
π Conclusion
Matrix multiplication is a powerful operation used in various fields, including graphics, physics simulations, and machine learning.
Understanding the logic behind matrix multiplication and implementing it in C provides a foundational understanding of linear algebra in computer science.
Feel free to experiment with different matrix sizes and values to further explore the capabilities of this program. Happy coding!
π¨βπ» Join our Community:
Author
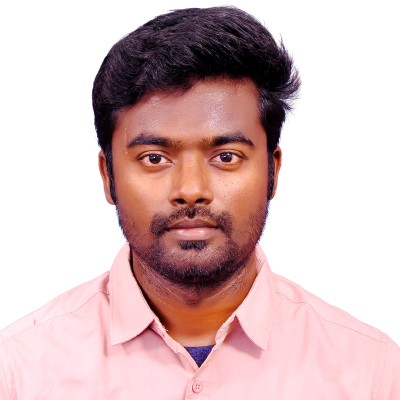
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
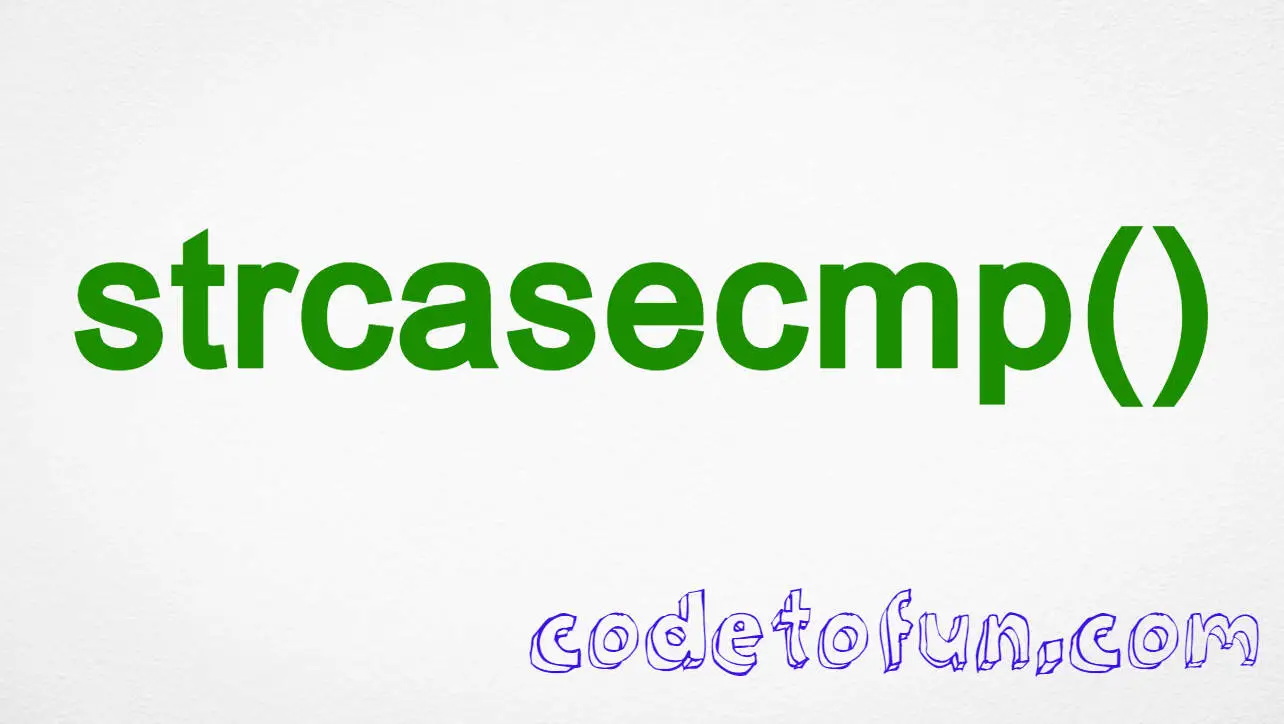
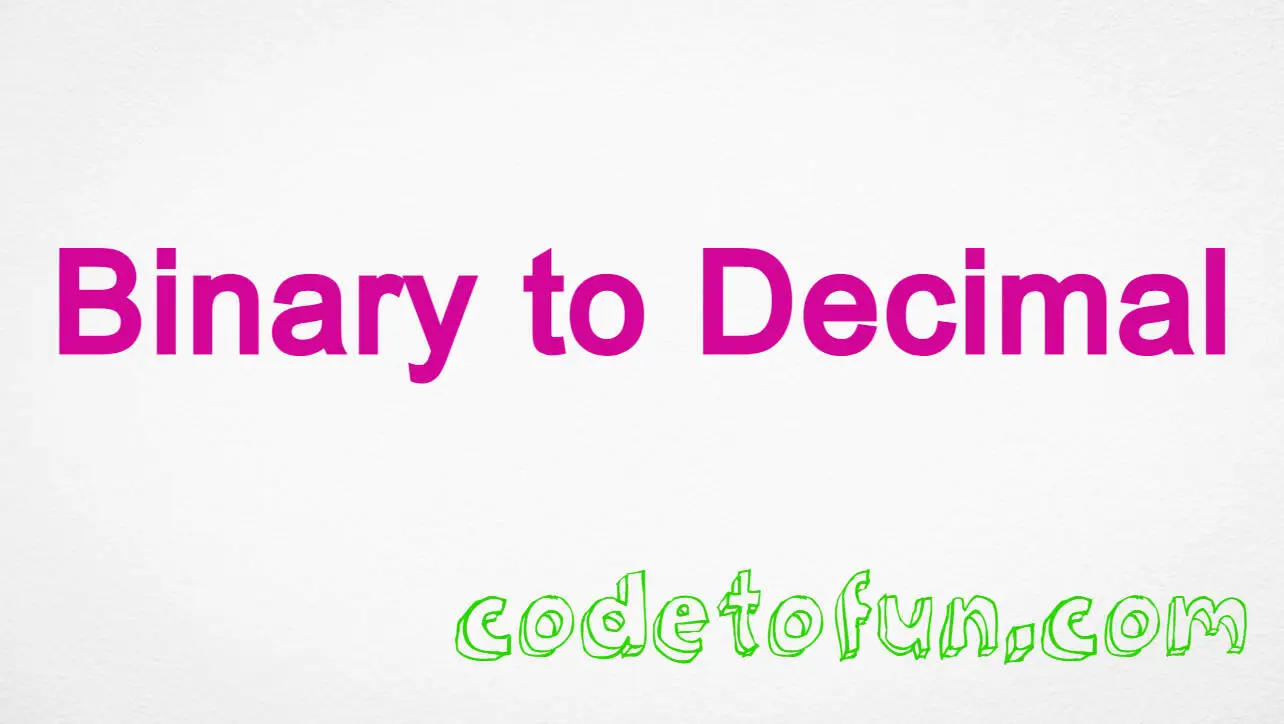
C Program to Converter a Binary to Decimal
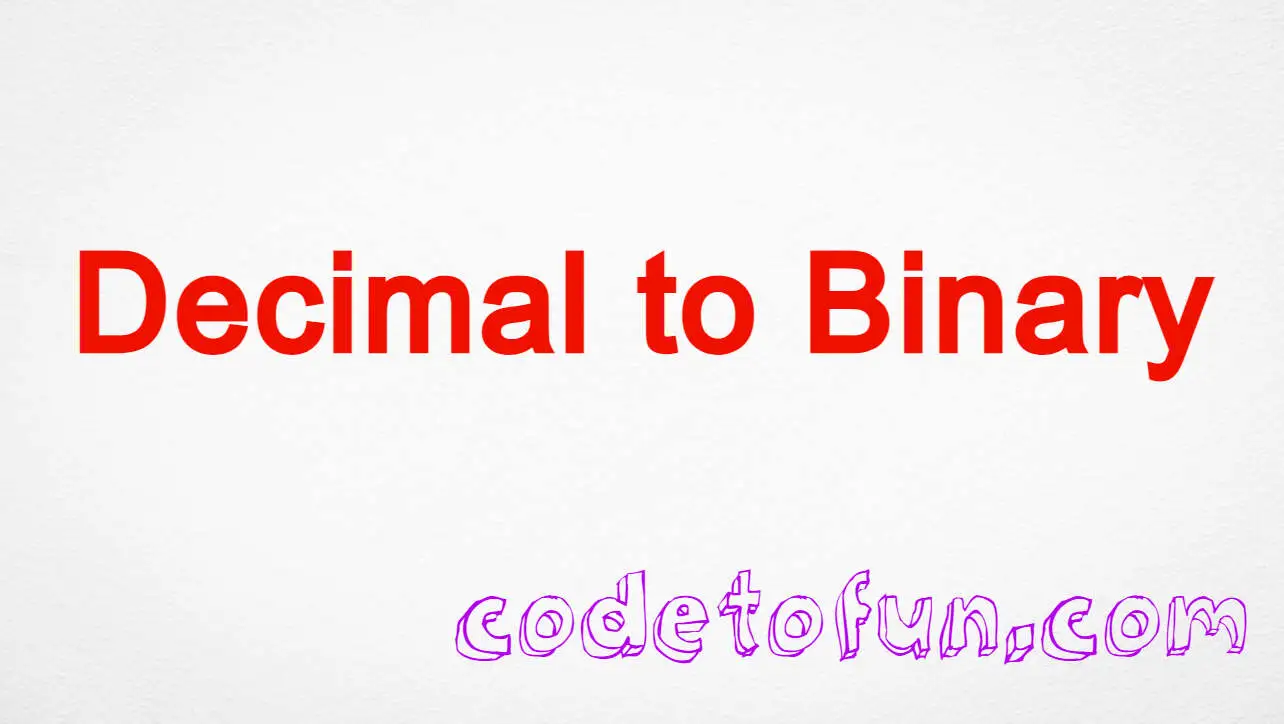
C Program to Converter a Decimal to Binary
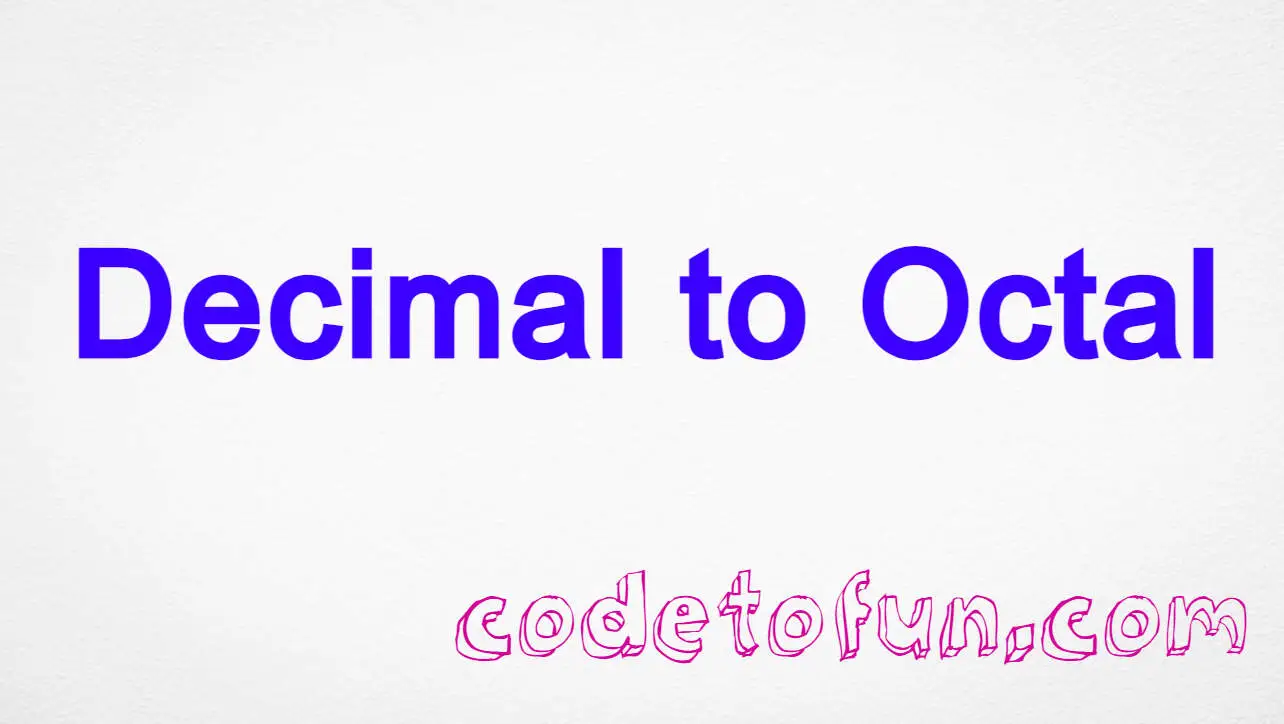
C Program to Converter a Decimal to Octal
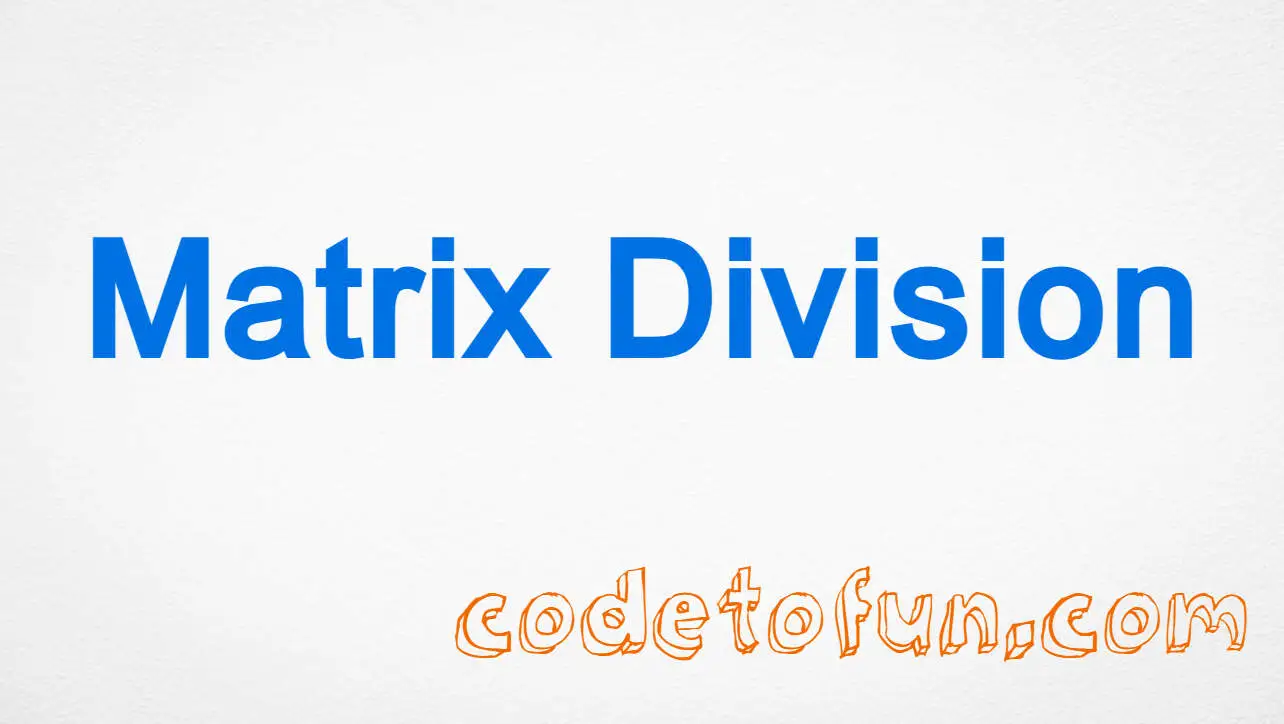
C Program to Perform Matrix Division
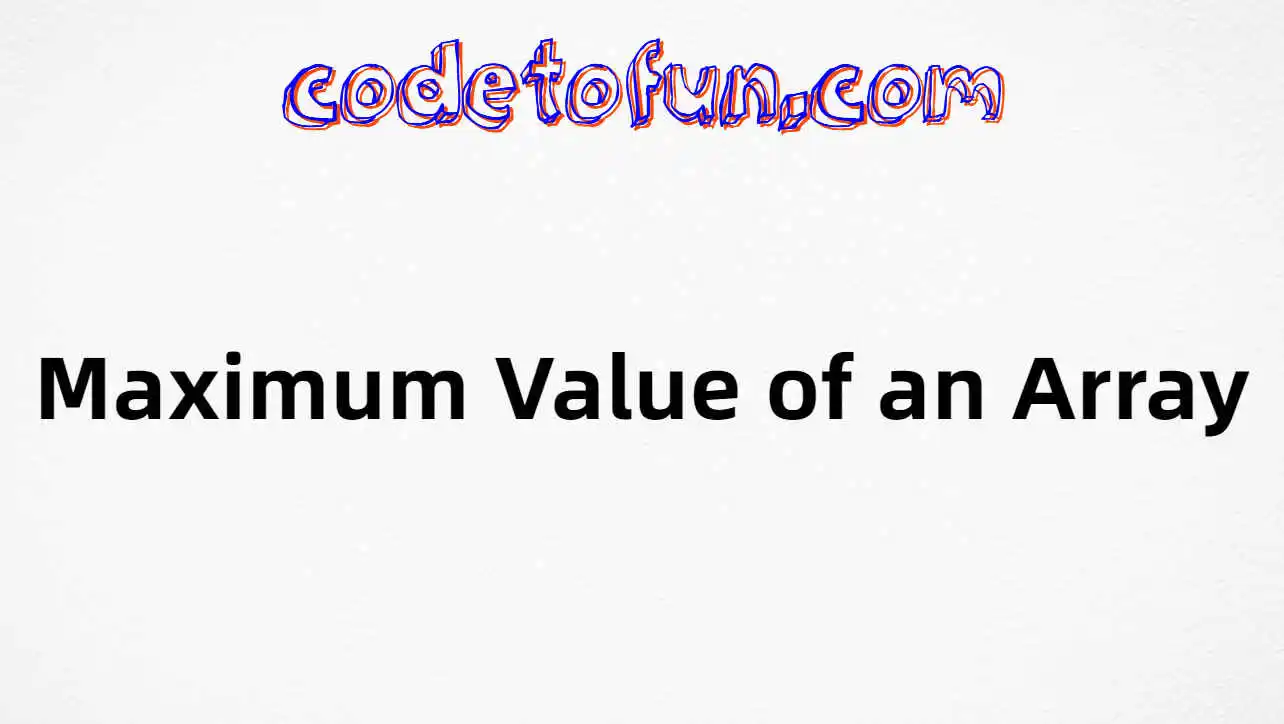
C Program to find Maximum Value of an Array
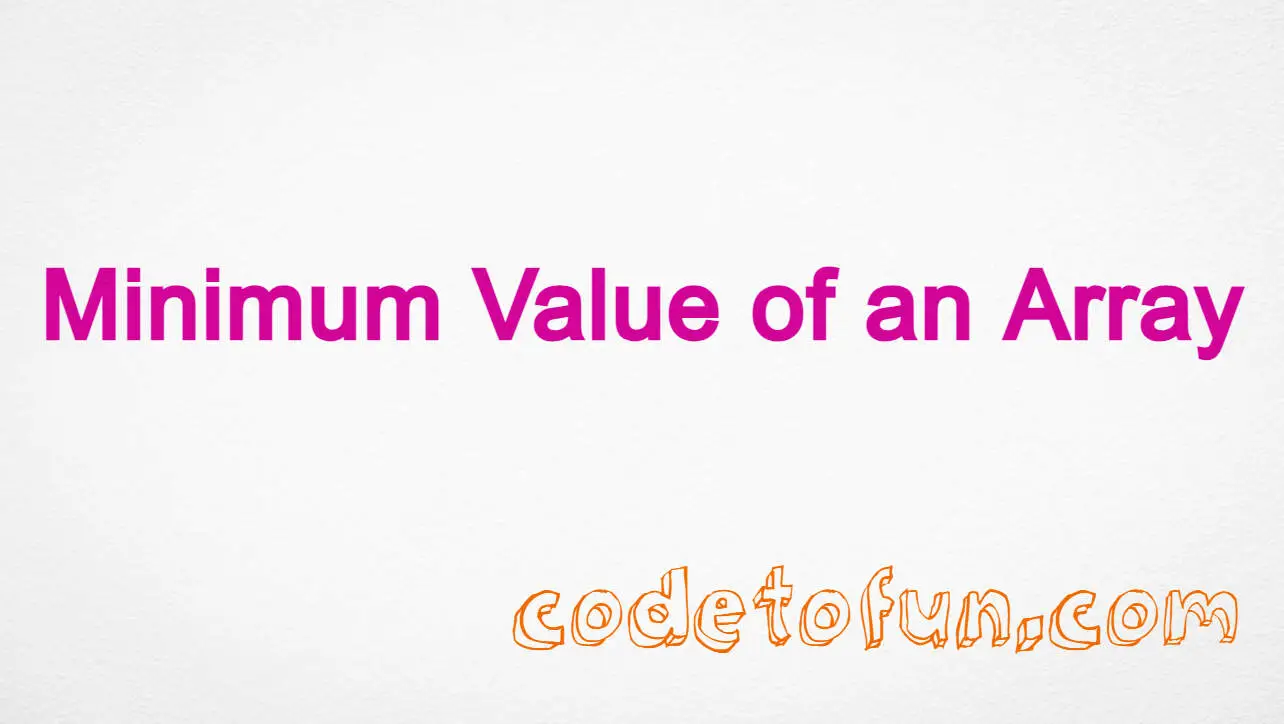
If you have any doubts regarding this article (C Program to Perform Matrix Multiplication), please comment here. I will help you immediately.