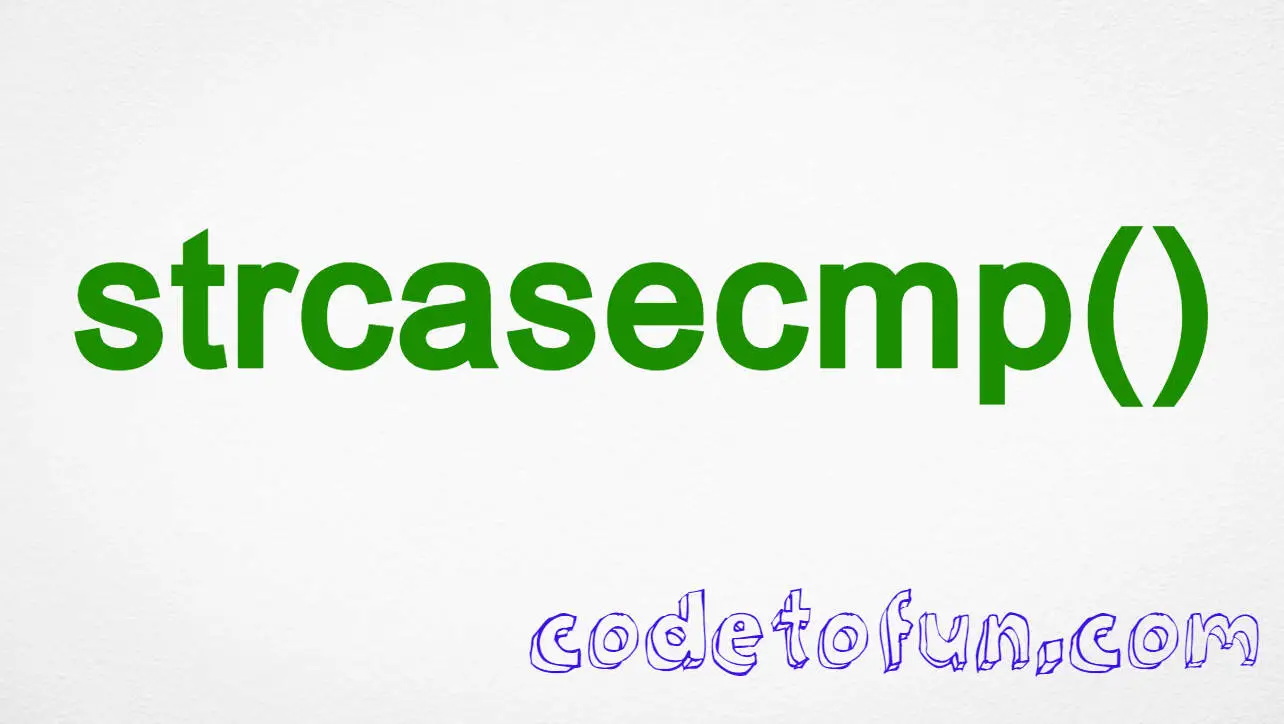
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strcasecmp() Function
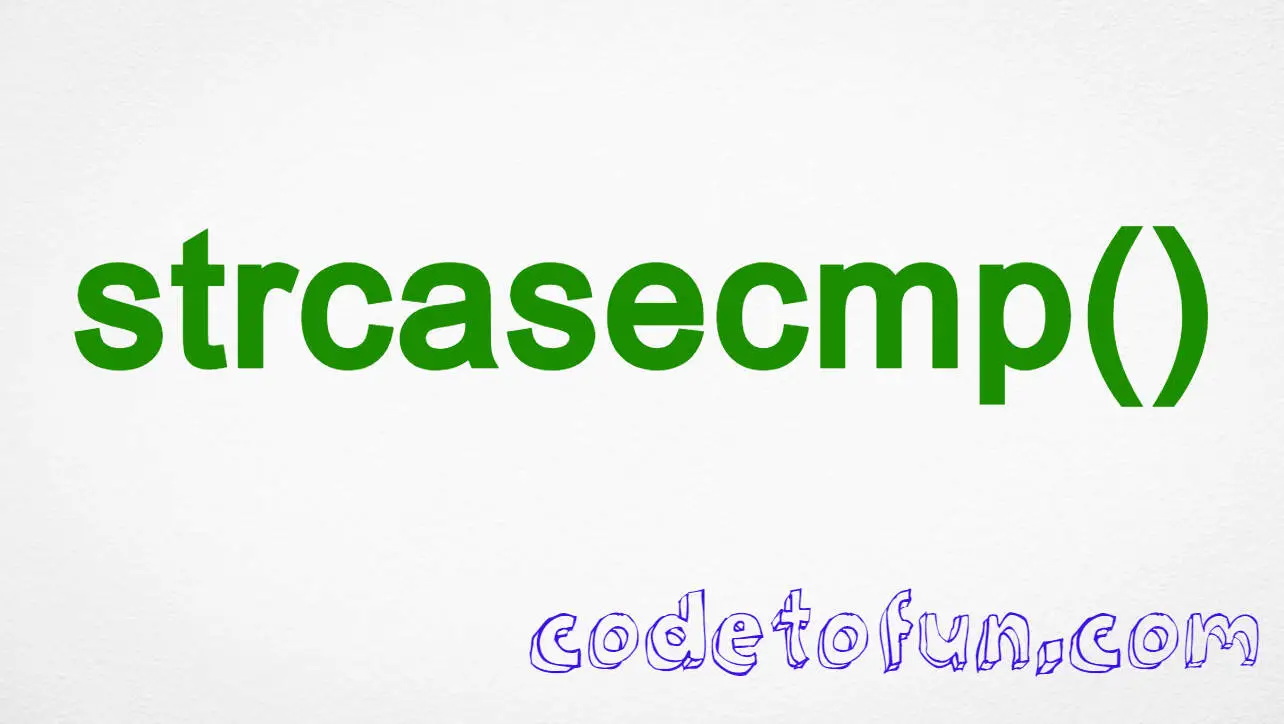
Photo Credit to CodeToFun
đ Introduction
In C programming, comparing strings is a common operation, and there are standard functions for this task.
However, for case-insensitive string comparison, the standard library provides strcasecmp()
in POSIX systems and _stricmp() in Windows.
In this tutorial, we'll focus on the strcasecmp()
function and explore its usage and functionality.
đĄ Syntax
The signature of the strcasecmp()
function is as follows:
int strcasecmp(const char *s1, const char *s2);
This function takes two strings, s1 and s2, and performs a case-insensitive comparison.
đ Example
Let's delve into an example to illustrate how the strcasecmp()
function works.
#include <stdio.h>
#include <strings.h>
int main() {
const char * str1 = "Hello, World!";
const char * str2 = "hello, world!";
// Compare strings case-insensitively
int result = strcasecmp(str1, str2);
// Output the result
if (result == 0) {
printf("The strings are equal.\n");
} else {
printf("The strings are not equal.\n");
}
return 0;
}
đģ Output
The strings are equal.
đ§ How the Program Works
In this example, the strcasecmp()
function compares the strings "Hello, World!" and "hello, world!" case-insensitively and prints the result.
âŠī¸ Return Value
The strcasecmp()
function returns an integer less than, equal to, or greater than zero if s1 is found, respectively, to be less than, to match, or be greater than s2. The comparison is case-insensitive.
đ Common Use Cases
The strcasecmp()
function is particularly useful when you need to compare strings in a case-insensitive manner. It simplifies tasks such as sorting strings or searching for a specific string regardless of case.
đ Notes
- Ensure that your compiler and system support the
strcasecmp()
function. In Windows environments, consider using _stricmp() instead.
đĸ Optimization
The strcasecmp()
function is optimized for case-insensitive string comparison. However, for performance-critical applications, you may want to explore alternative approaches or consider implementing your optimized version based on specific requirements.
đ Conclusion
The strcasecmp()
function in C is a valuable tool for case-insensitive string comparison. It provides a standardized and efficient way to perform this task, enhancing the flexibility and readability of your code.
Feel free to experiment with different strings and explore the behavior of the strcasecmp()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
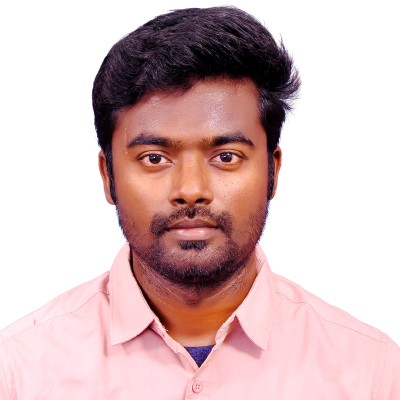
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
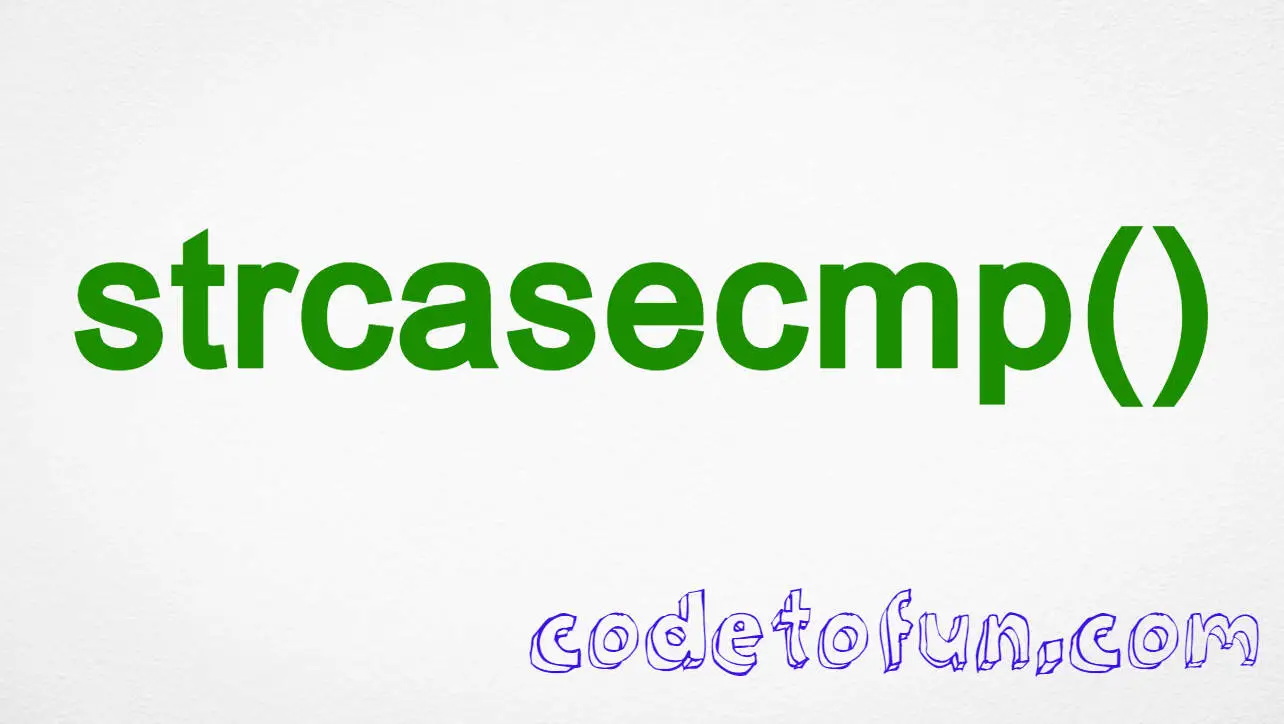
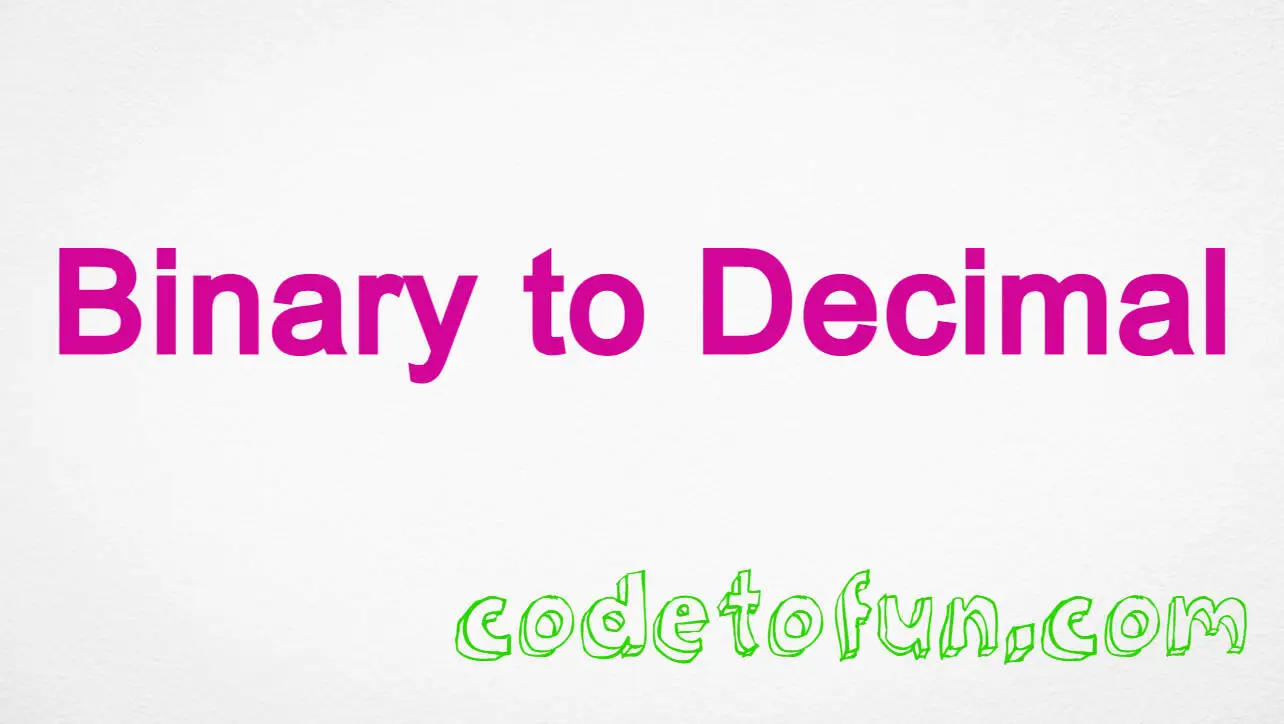
C Program to Converter a Binary to Decimal
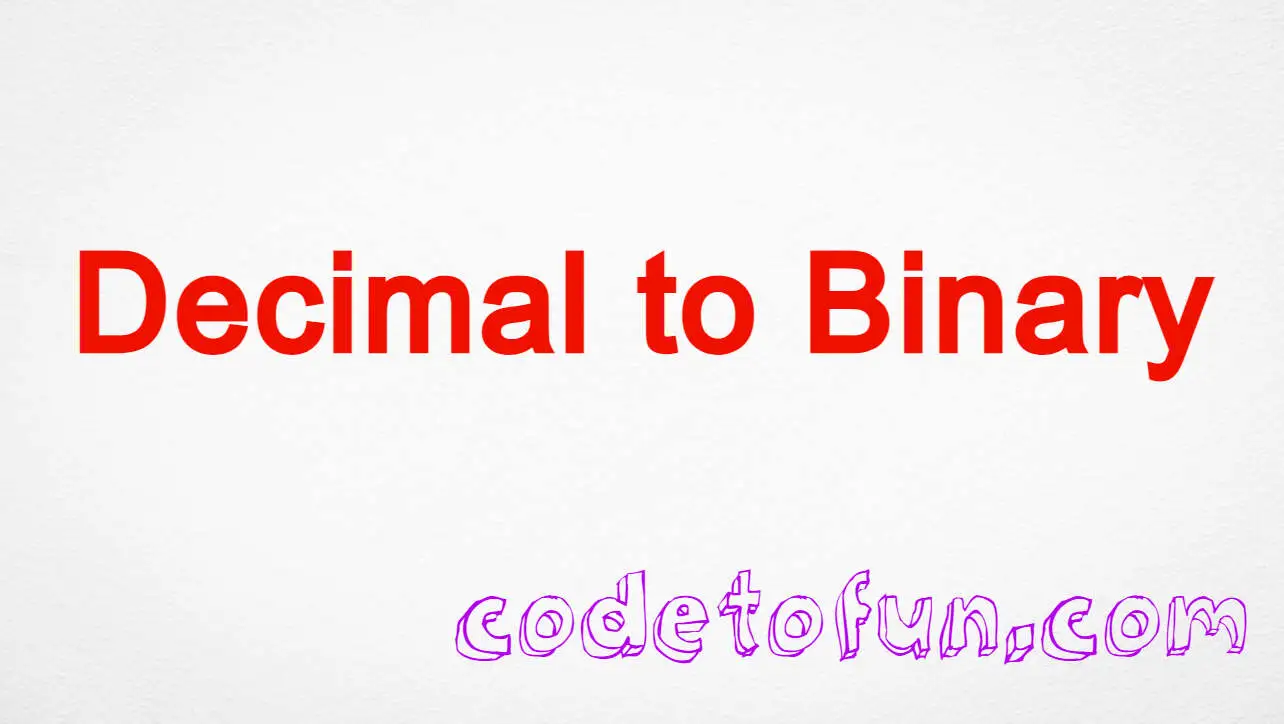
C Program to Converter a Decimal to Binary
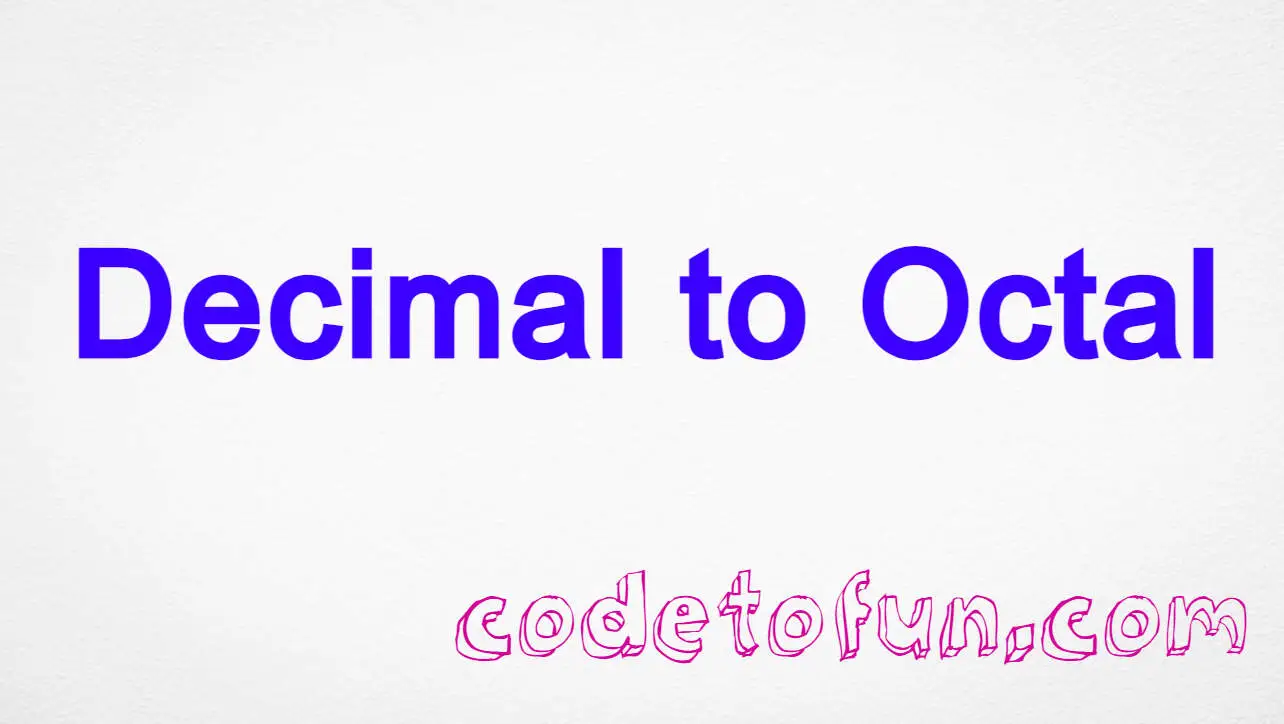
C Program to Converter a Decimal to Octal
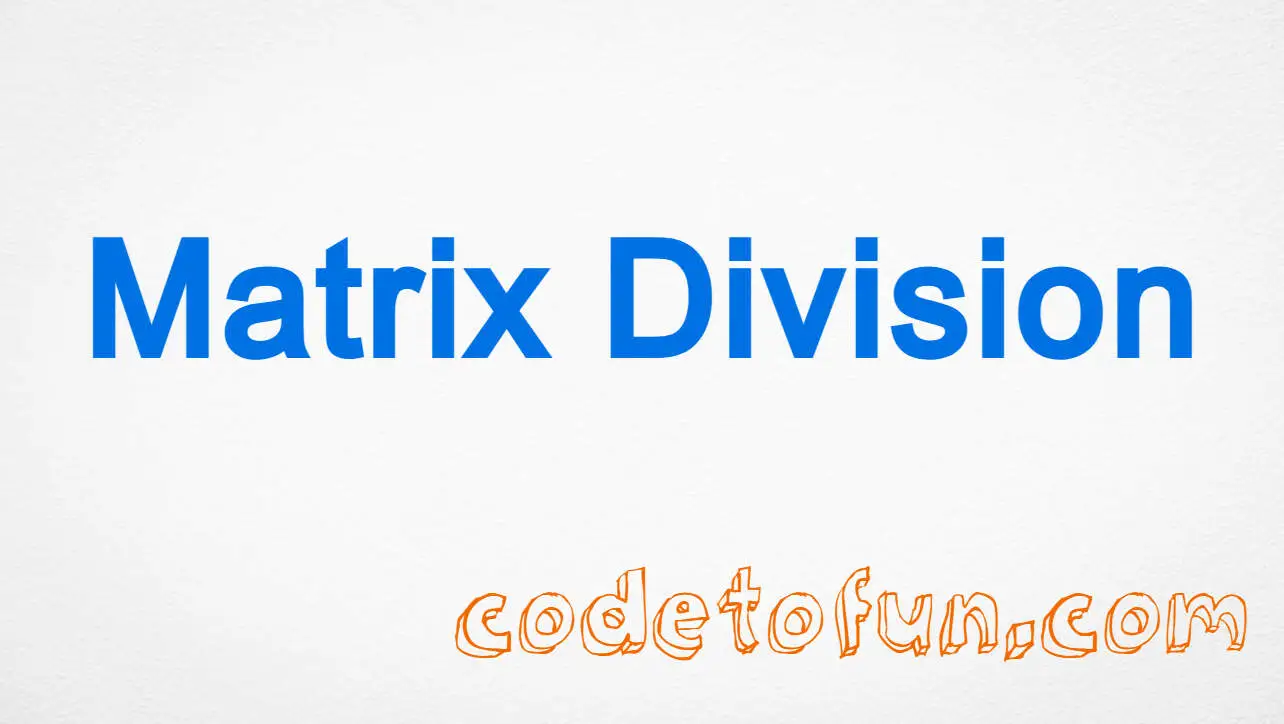
C Program to Perform Matrix Division
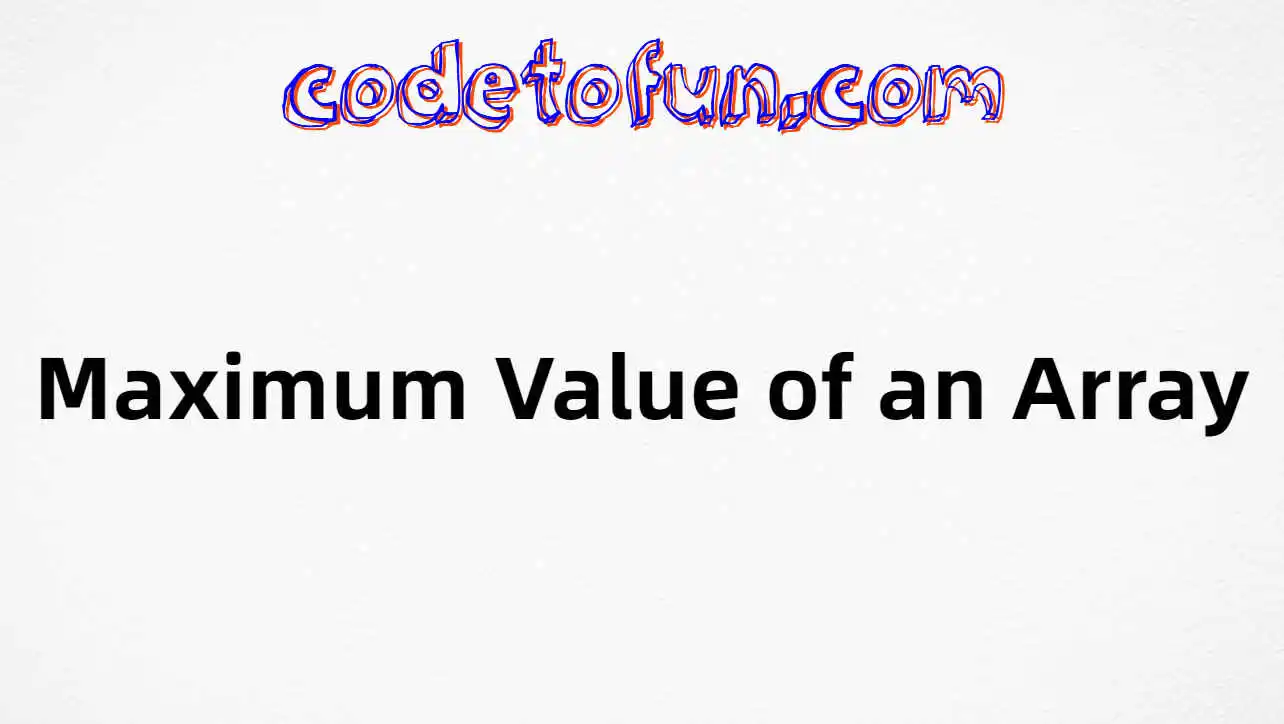
C Program to find Maximum Value of an Array
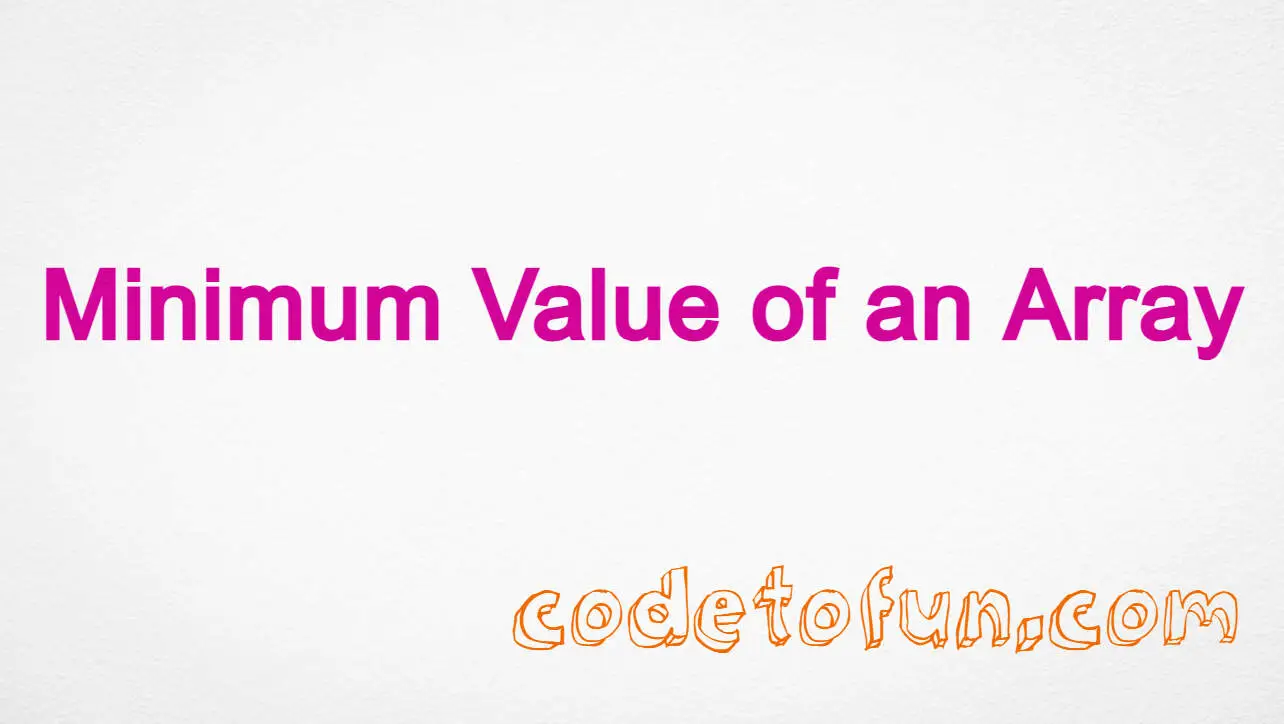
If you have any doubts regarding this article (C strcasecmp() Function), please comment here. I will help you immediately.