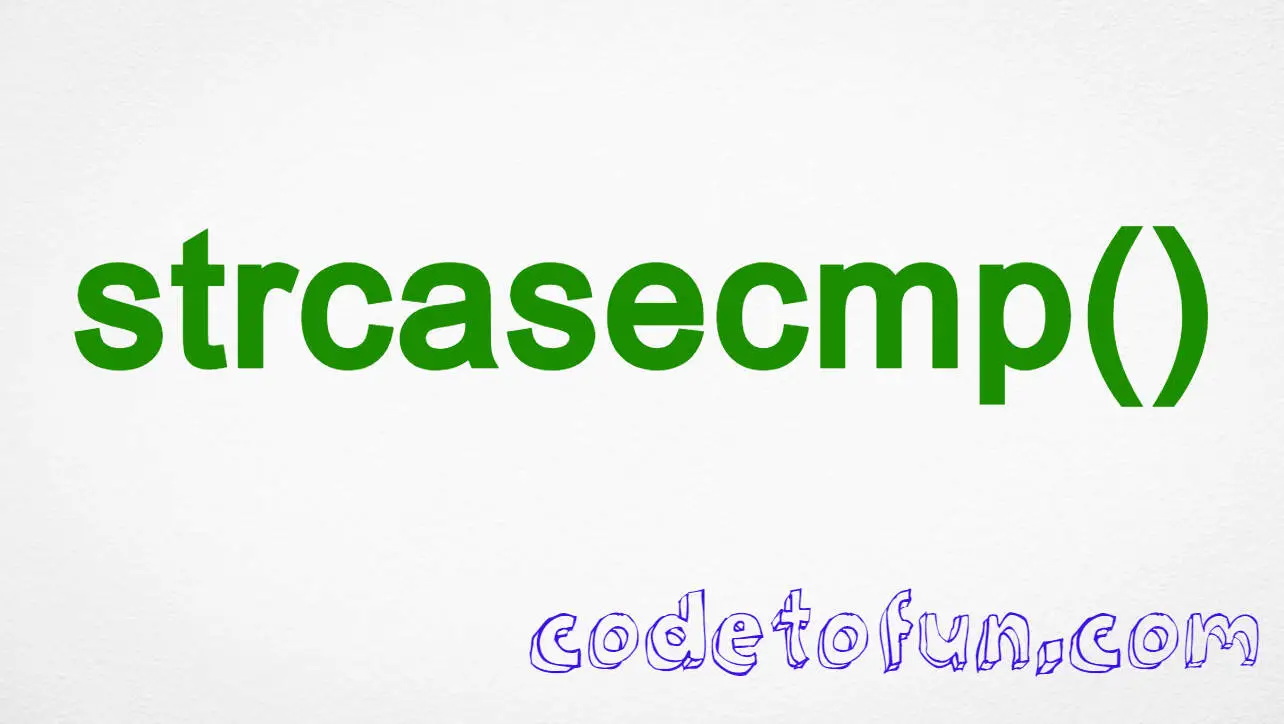
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strncat() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, manipulating strings often involves concatenation, and the strncat()
function is a useful tool for this task.
The strncat()
function is part of the C Standard Library, and it is used to concatenate a specified number of characters from one string to the end of another.
In this tutorial, we'll explore the usage and functionality of the strncat()
function.
đĄ Syntax
The signature of the strncat()
function is as follows:
char *strncat(char *dest, const char *src, size_t n);
This function appends at most n characters from the string pointed to by src to the end of the string pointed to by dest.
đ Example
Let's delve into an example to illustrate how the strncat()
function works.
#include <stdio.h>
#include <string.h>
int main() {
// Destination string with enough space for concatenation
char dest[20] = "Hello, ";
// Source string
const char * src = "world!";
// Concatenate at most 5 characters from src to dest
strncat(dest, src, 5);
// Output the result
printf("Concatenated string: %s\n", dest);
return 0;
}
đģ Output
Concatenated string: Hello, world
đ§ How the Program Works
In this example, the strncat()
function concatenates at most 5 characters from the source string "world!" to the end of the destination string "Hello, ".
âŠī¸ Return Value
The strncat()
function returns a pointer to the resulting concatenated string (dest).
đ Common Use Cases
The strncat()
function is useful when you want to concatenate a specified number of characters from one string to another. It helps prevent buffer overflows by allowing you to control the maximum number of characters appended.
đ Notes
- Ensure that the destination string (dest) has enough space to accommodate the concatenated characters.
- The
strncat()
function does not check for the length of the destination string, so it's crucial to provide sufficient space.
đĸ Optimization
The strncat()
function is optimized for efficient string concatenation. However, it's essential to manage buffer sizes carefully to prevent memory-related issues.
đ Conclusion
The strncat()
function in C is a valuable tool for controlled string concatenation. It provides a flexible and efficient way to concatenate a specified number of characters from one string to another.
Feel free to experiment with different strings, adjust the number of characters to concatenate, and explore the behavior of the strncat()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
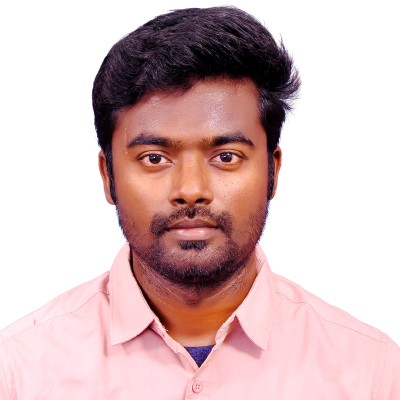
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
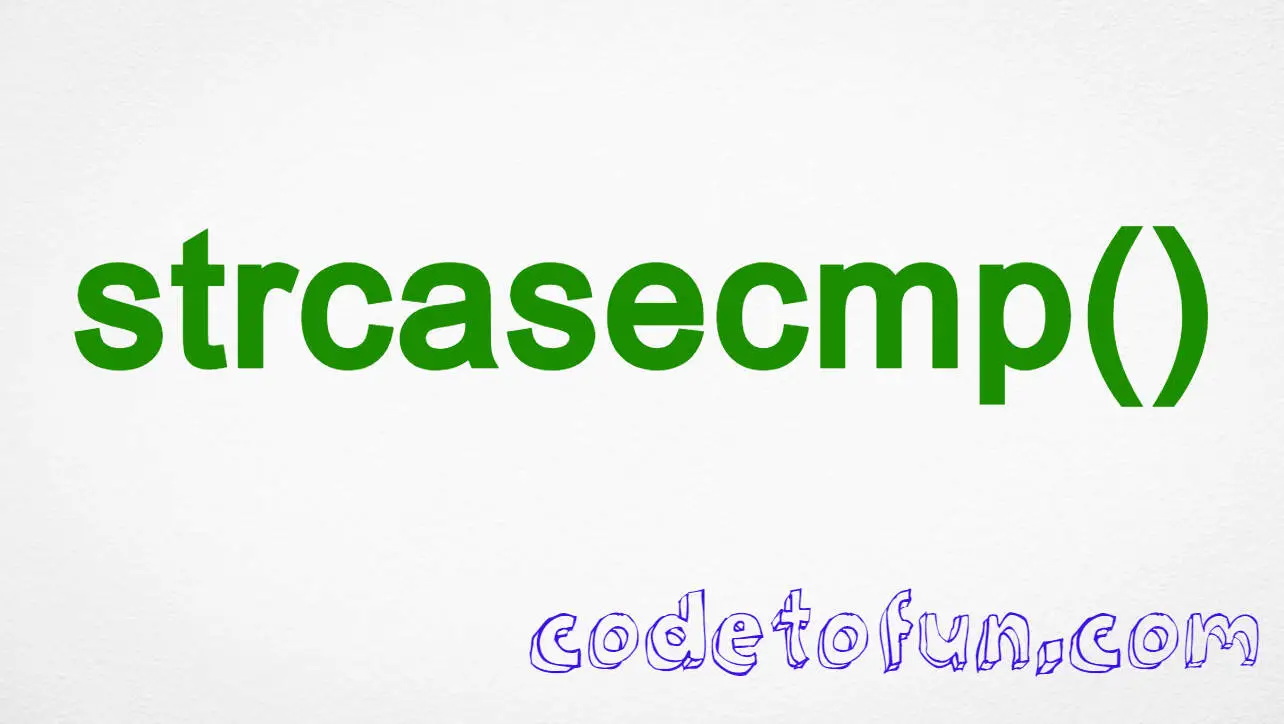
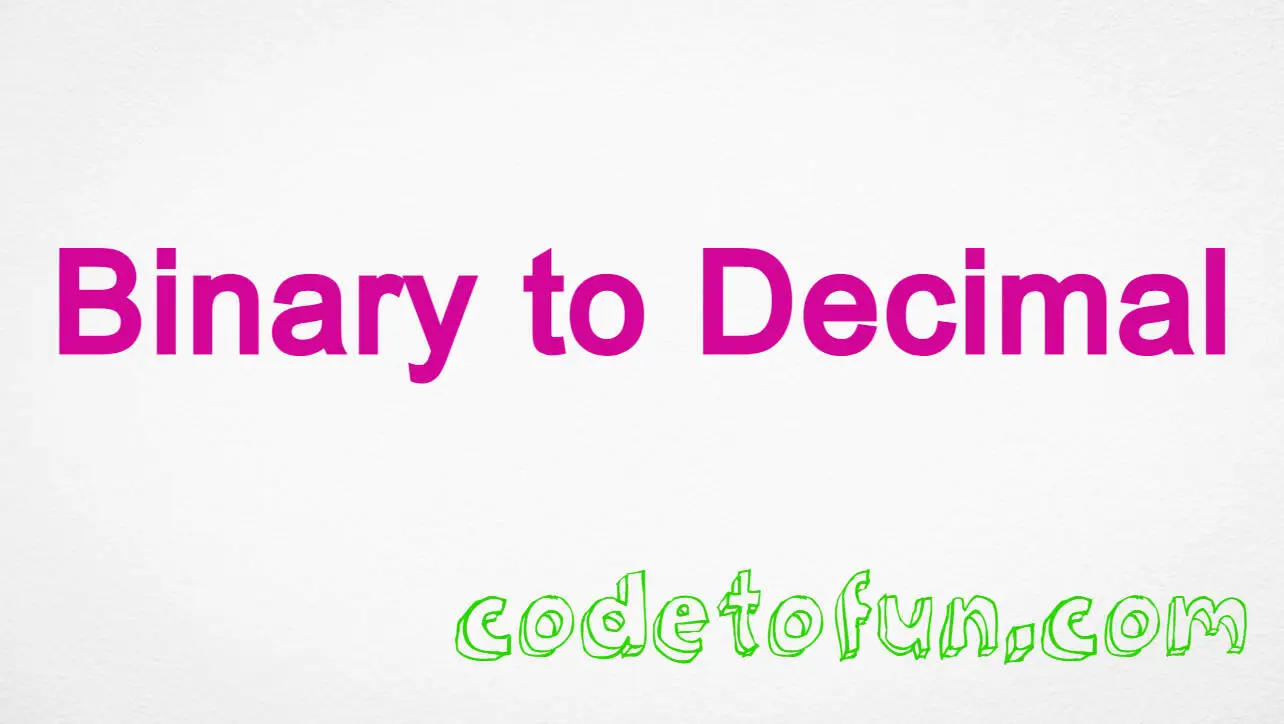
C Program to Converter a Binary to Decimal
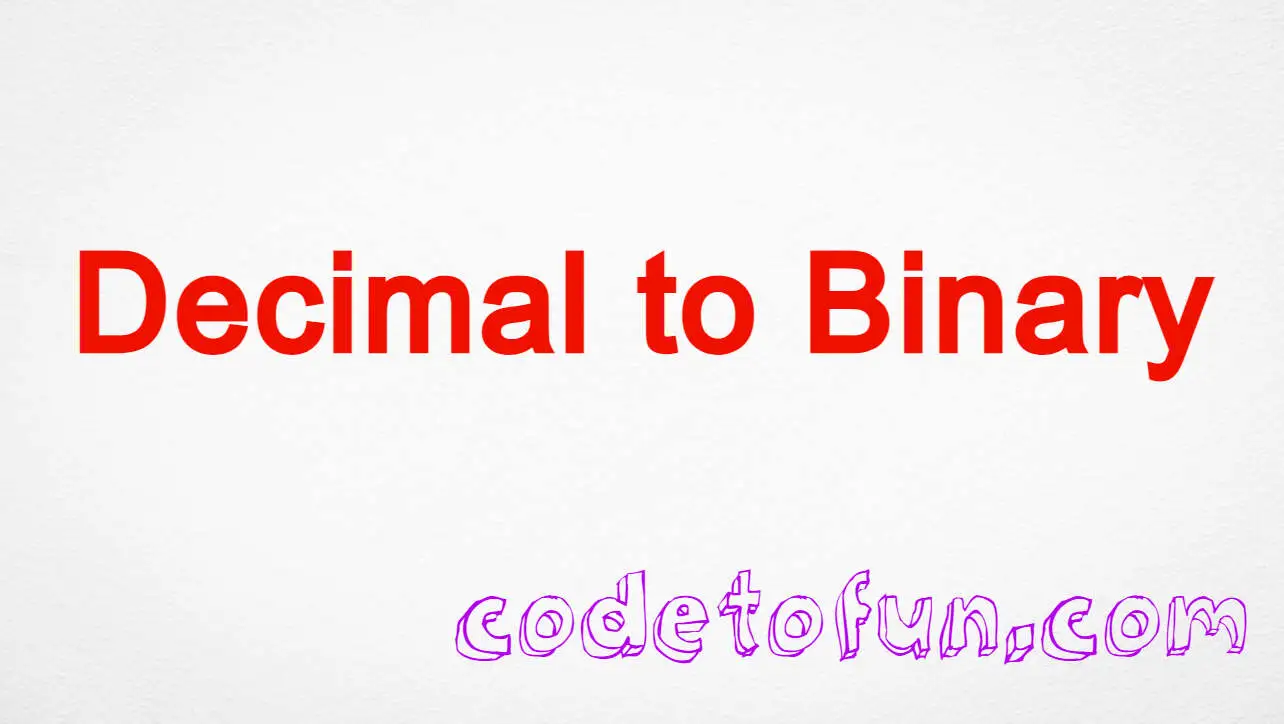
C Program to Converter a Decimal to Binary
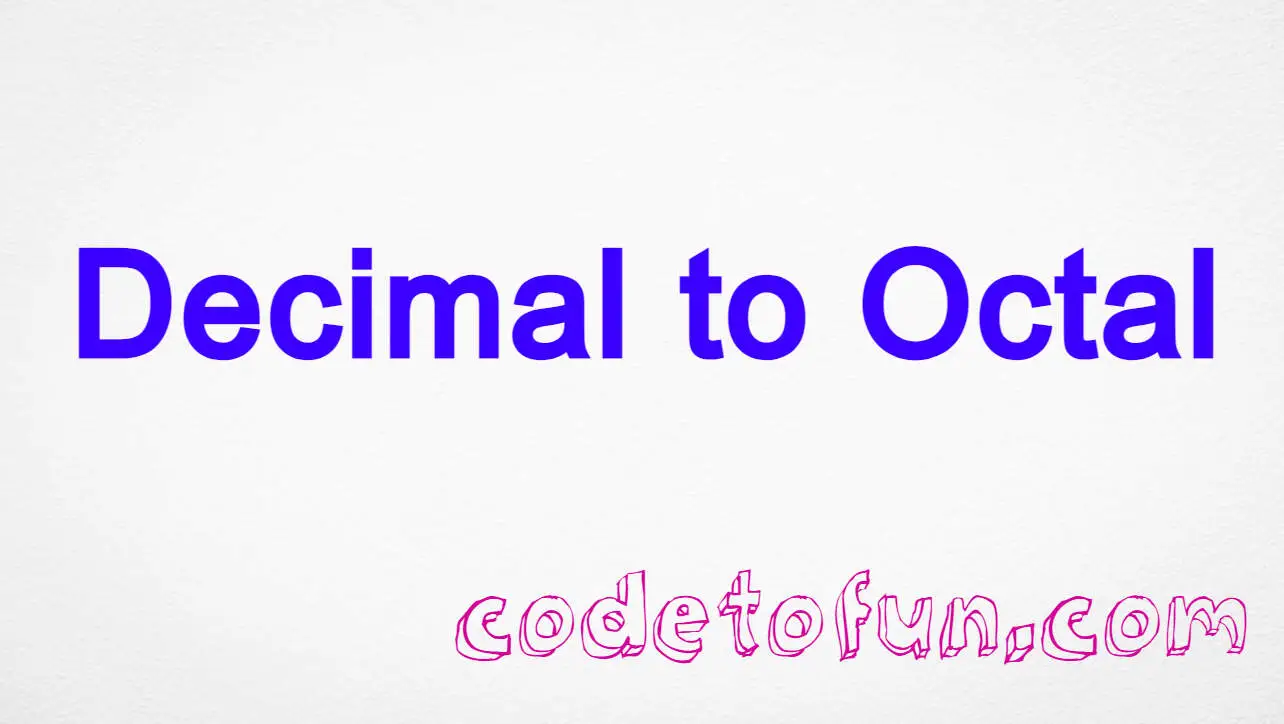
C Program to Converter a Decimal to Octal
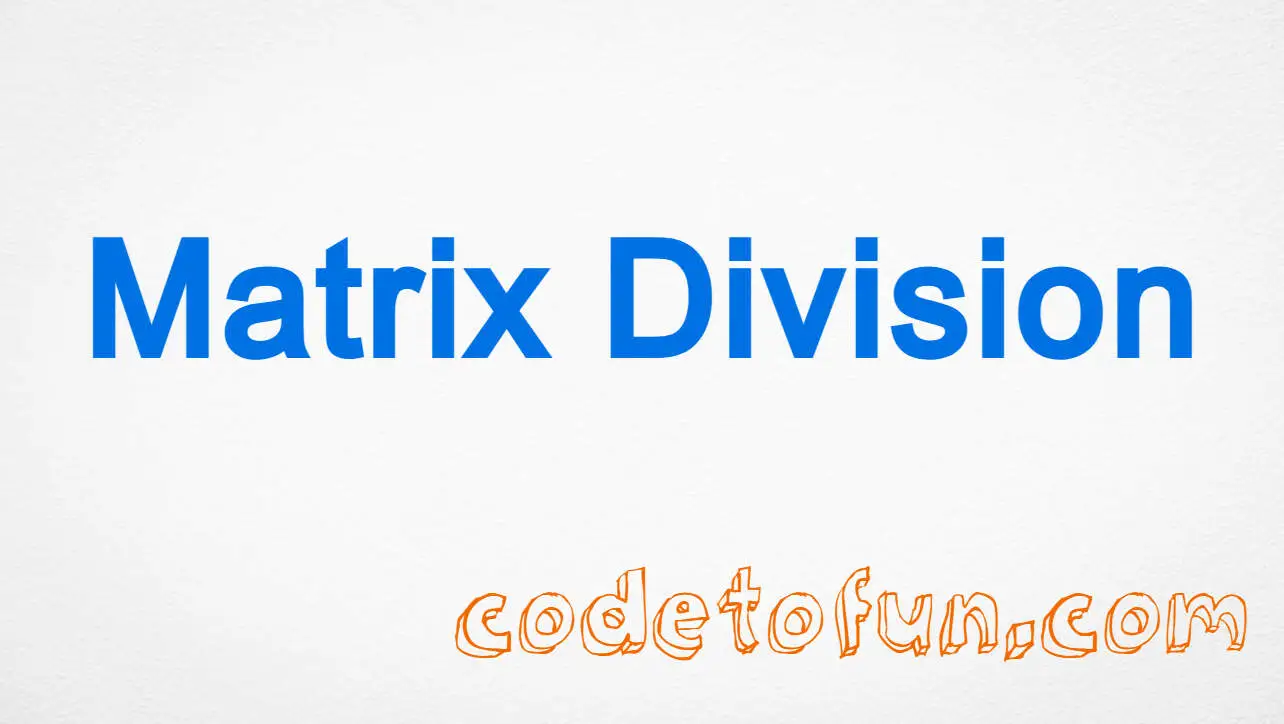
C Program to Perform Matrix Division
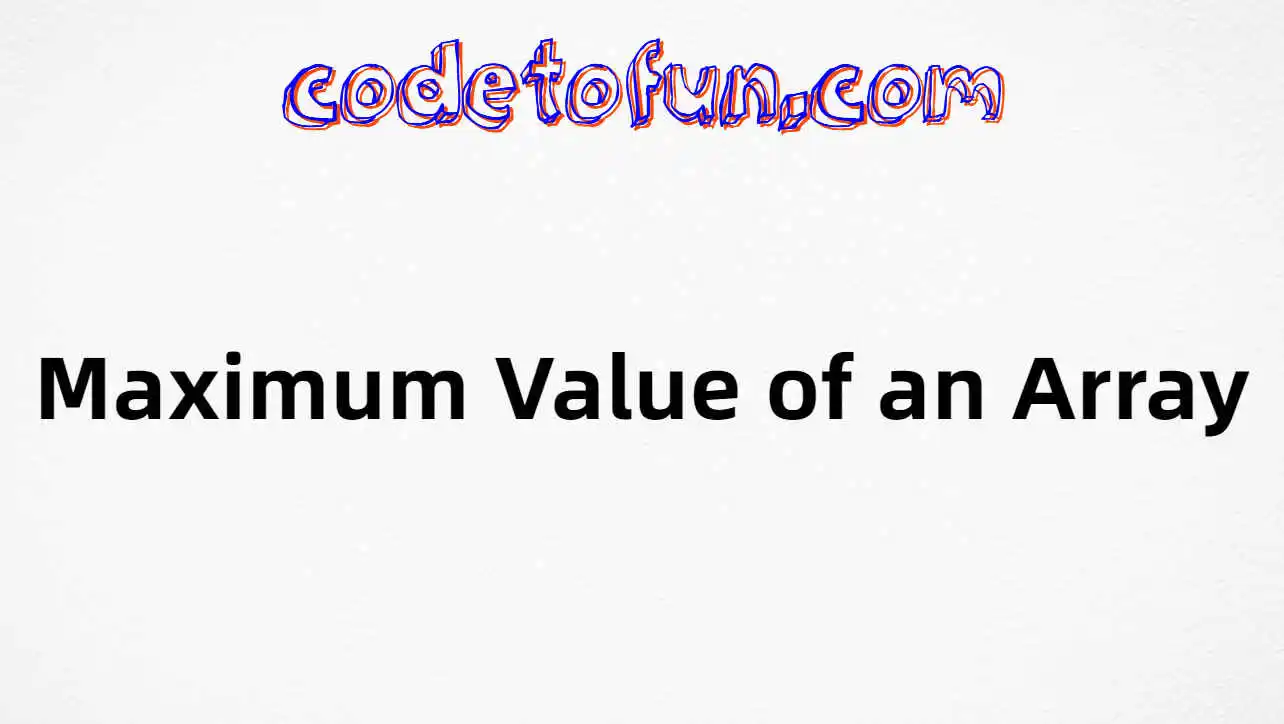
C Program to find Maximum Value of an Array
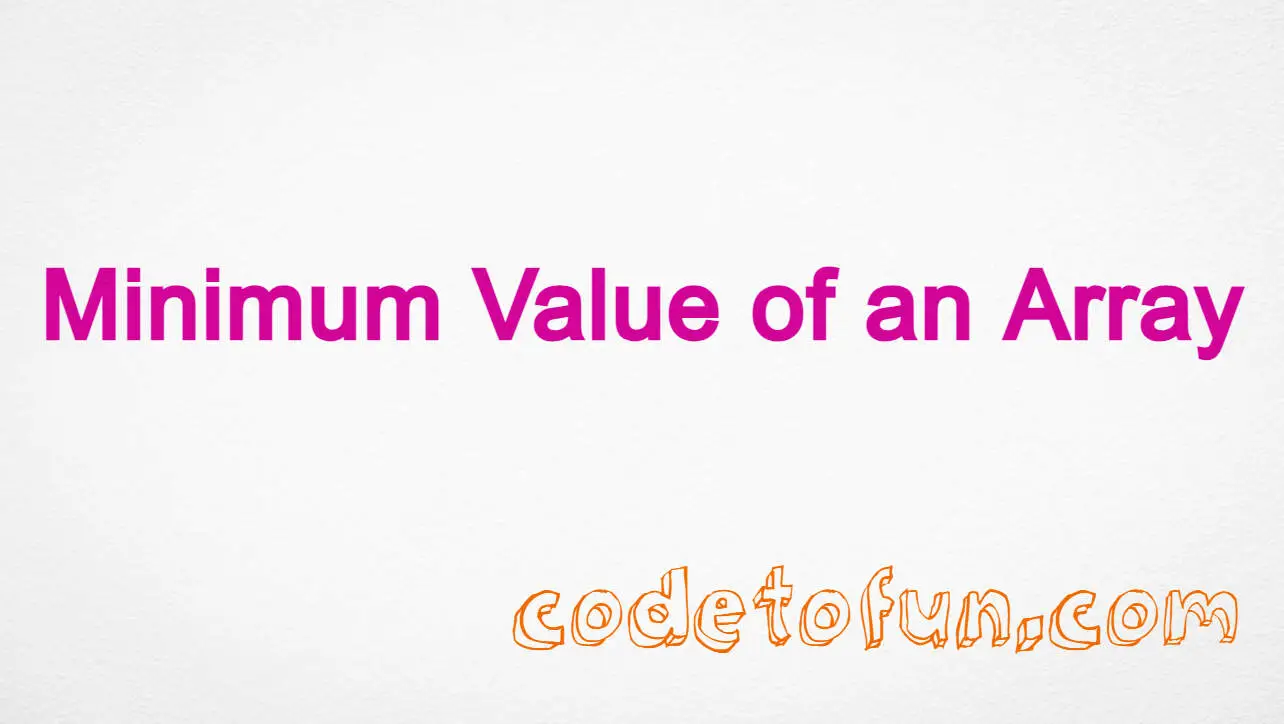
If you have any doubts regarding this article (C strncat() Function) please comment here. I will help you immediately.