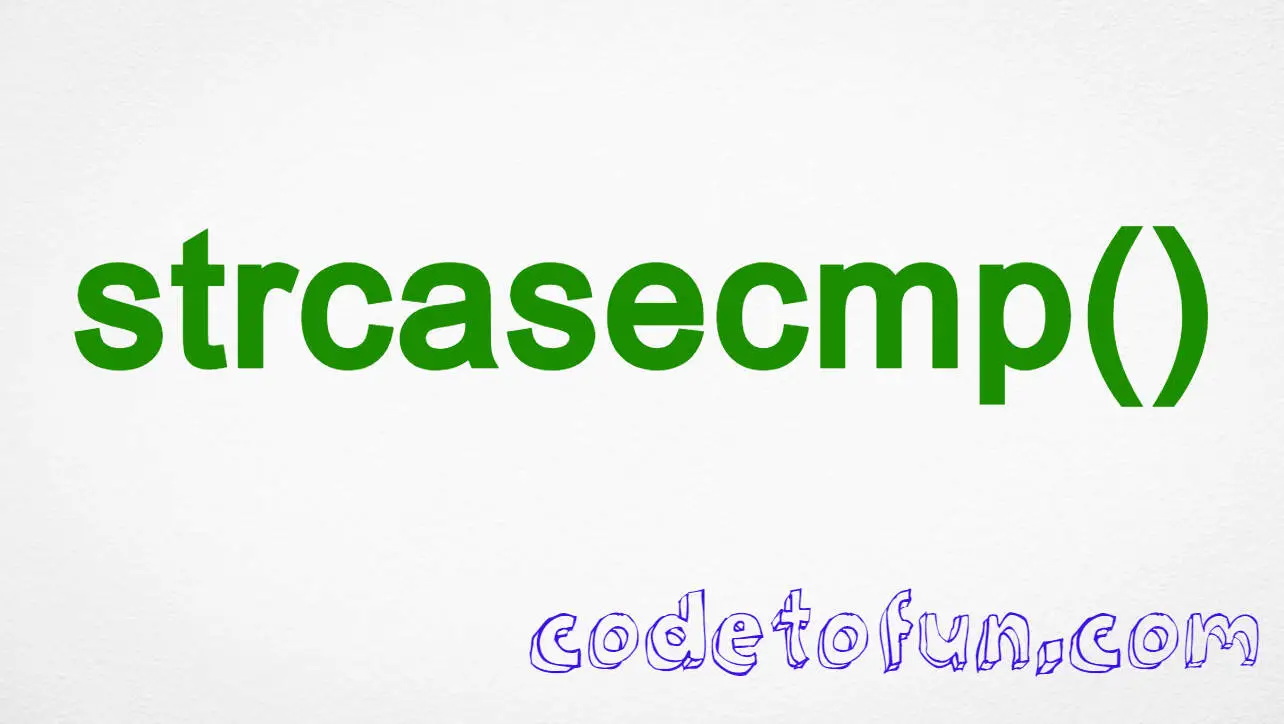
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strrchr() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In the realm of C programming, working with strings is a common and essential task.
The strrchr()
function is part of the C Standard Library and is used to find the last occurrence of a character in a given string.
In this tutorial, we'll explore the usage and functionality of the strrchr()
function in C.
đĄ Syntax
The strrchr()
function has the following signature:
char *strrchr(const char *str, int character);
- str: The string in which the search will be performed.
- character: The character to be located. This is passed as an integer but is commonly specified as a character literal.
đ Example
Let's dive into an example to illustrate how the strrchr()
function works.
#include <stdio.h>
#include <string.h>
int main() {
const char * text = "Hello, C Programming!";
char searchChar = 'o';
// Find the last occurrence of 'o' in the string
char * result = strrchr(text, searchChar);
// Output the result
if (result != NULL) {
printf("Last occurrence of '%c': %s\n", searchChar, result);
} else {
printf("'%c' not found in the string.\n", searchChar);
}
return 0;
}
đģ Output
Last occurrence of 'o': ogramming!
đ§ How the Program Works
In this example, the strrchr()
function is used to find the last occurrence of the character 'o' in the string "Hello, C Programming!" and then prints the result.
âŠī¸ Return Value
The strrchr()
function returns a pointer to the last occurrence of the specified character in the string. If the character is not found, it returns NULL.
đ Common Use Cases
The strrchr()
function is particularly useful when you need to locate the position of the last occurrence of a specific character in a string. It can be used for tasks such as extracting the file extension from a file path or parsing data.
đ Notes
- The character to be located is passed as an integer, but it is typically specified using character literal syntax, such as o.
- The function is case-sensitive, so 'o' and 'O' are considered different characters.
đĸ Optimization
The strrchr()
function is optimized for efficiency. However, if you know that the character you are searching for is the null terminator \0 or the last character in the string, using other functions like strlen() may provide a faster alternative.
đ Conclusion
The strrchr()
function in C is a valuable tool for finding the last occurrence of a character in a string. It provides flexibility in string manipulation tasks and is a fundamental component of C string handling.
Feel free to experiment with different strings and characters to deepen your understanding of the strrchr()
function. Happy coding!
đ¨âđģ Join our Community:
Author
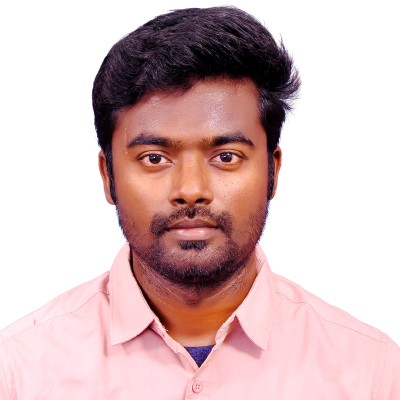
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
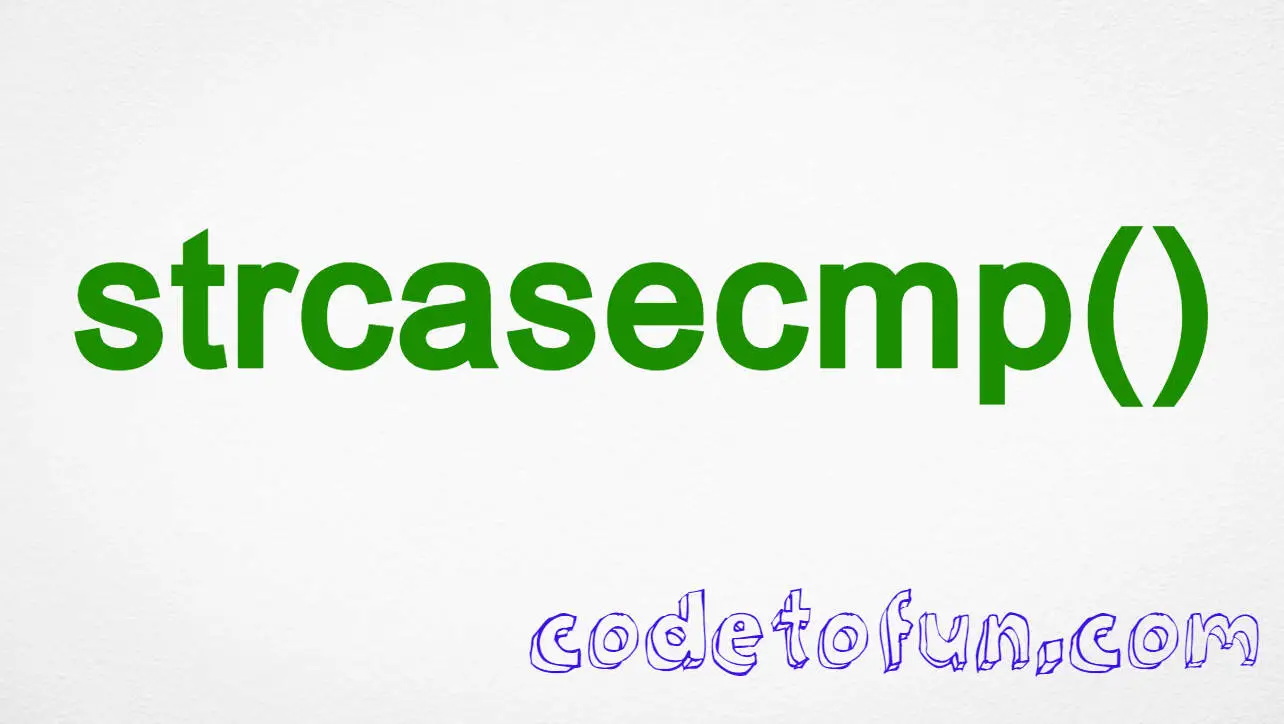
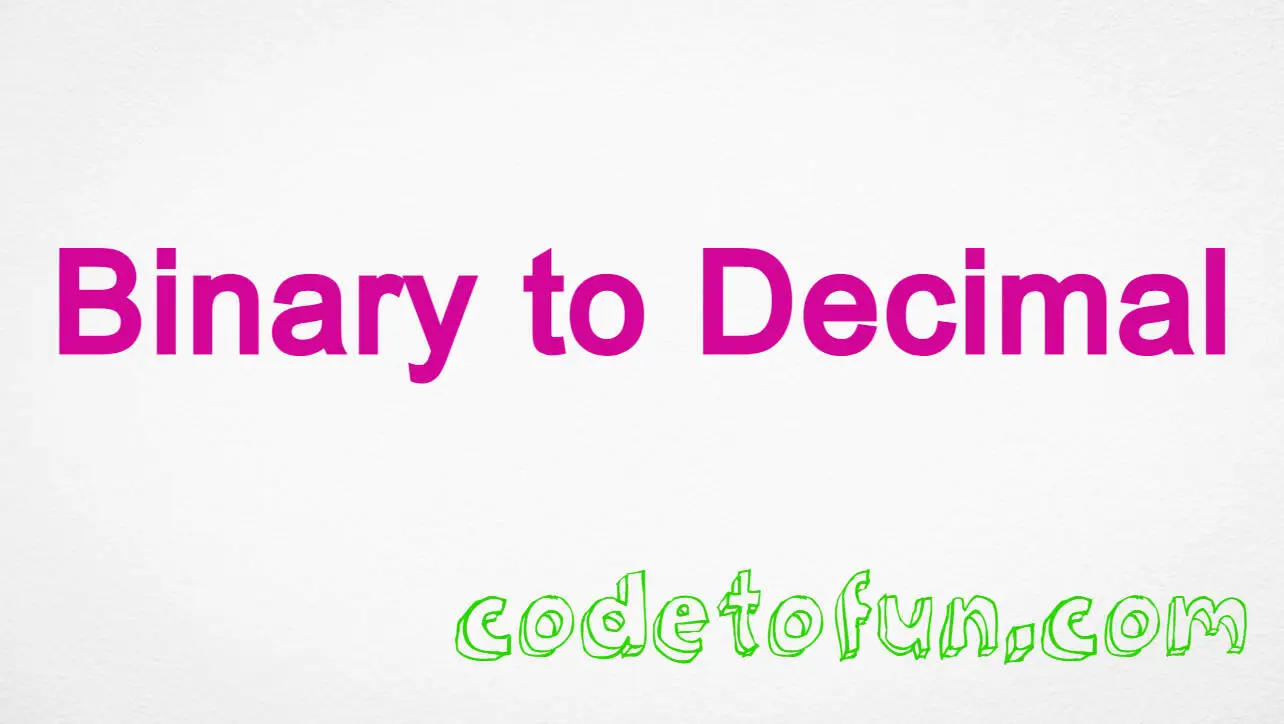
C Program to Converter a Binary to Decimal
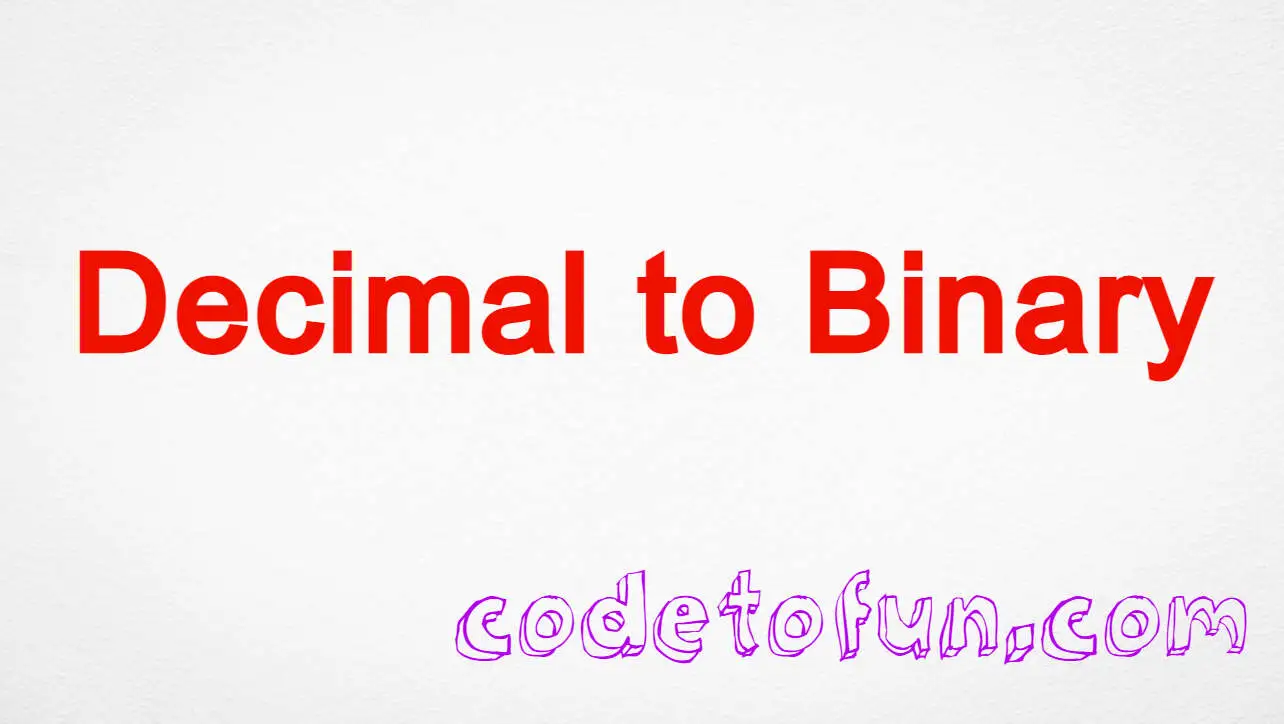
C Program to Converter a Decimal to Binary
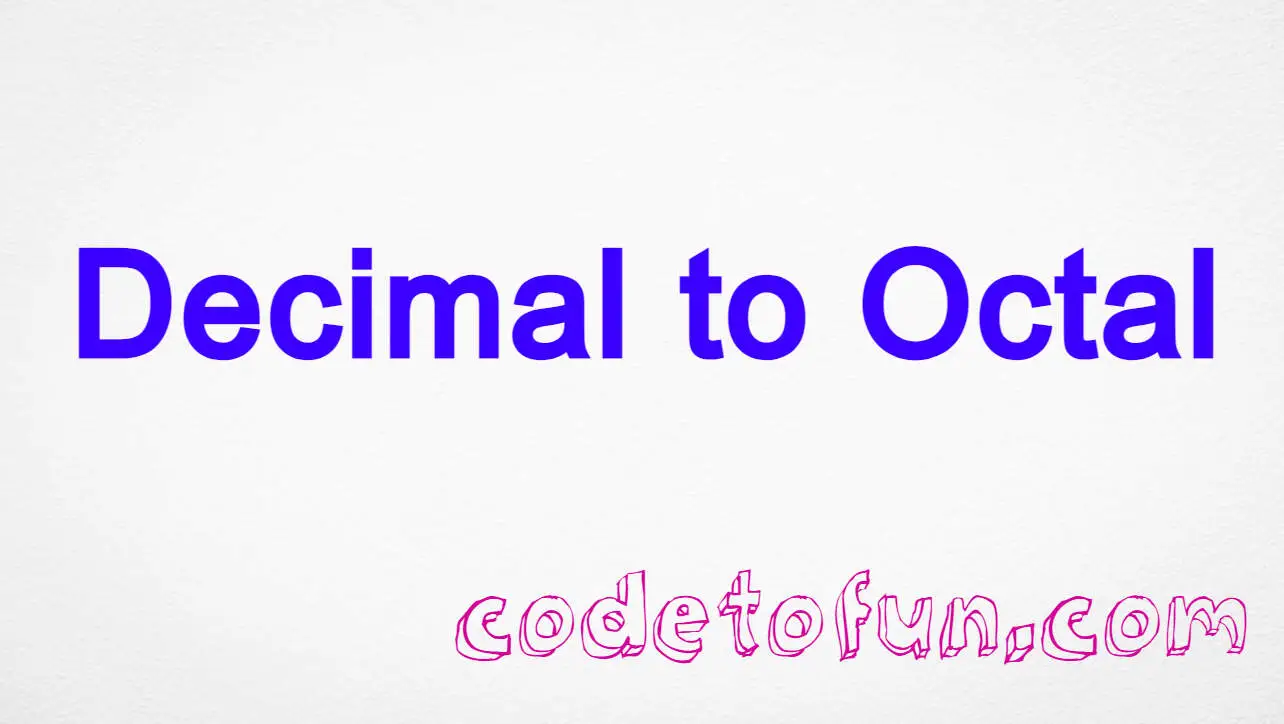
C Program to Converter a Decimal to Octal
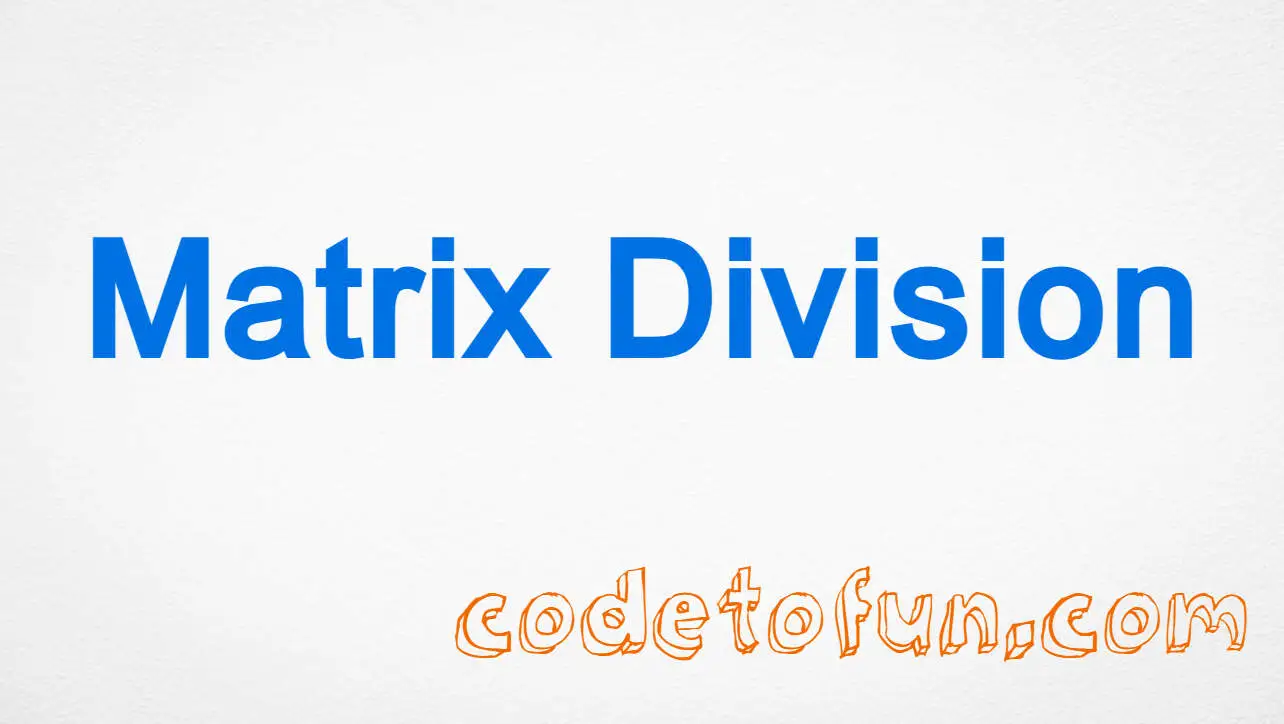
C Program to Perform Matrix Division
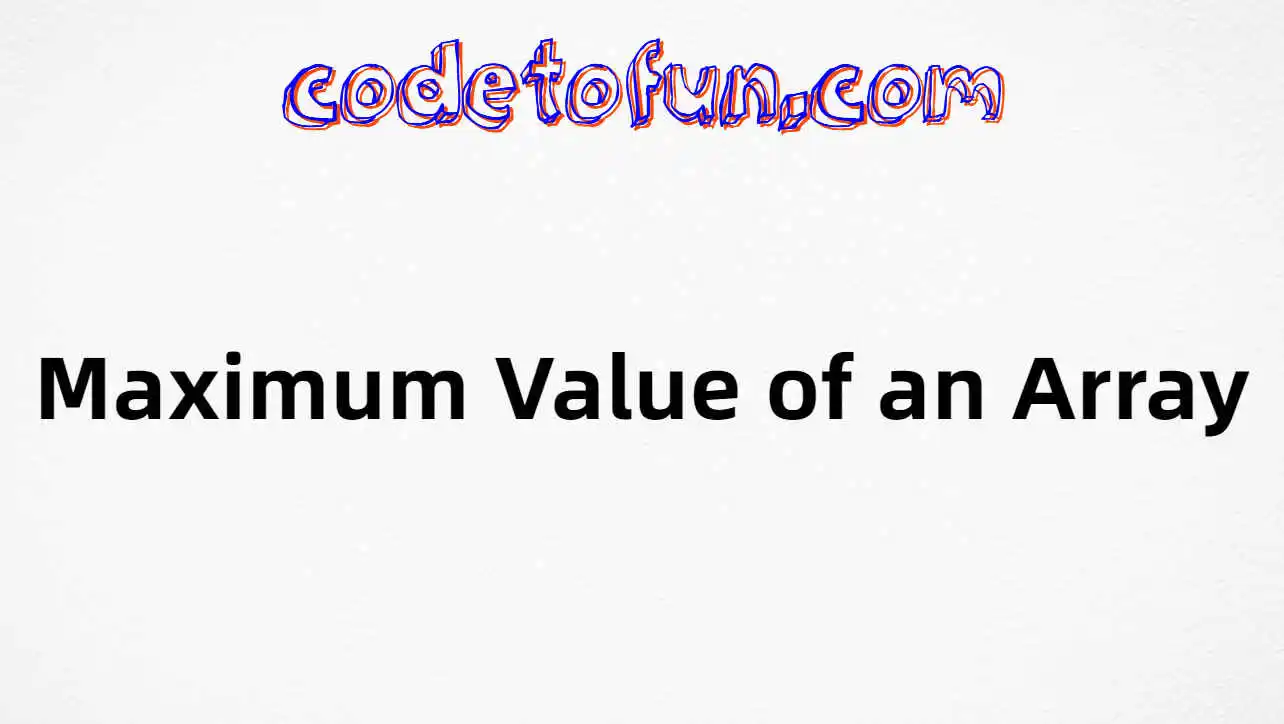
C Program to find Maximum Value of an Array
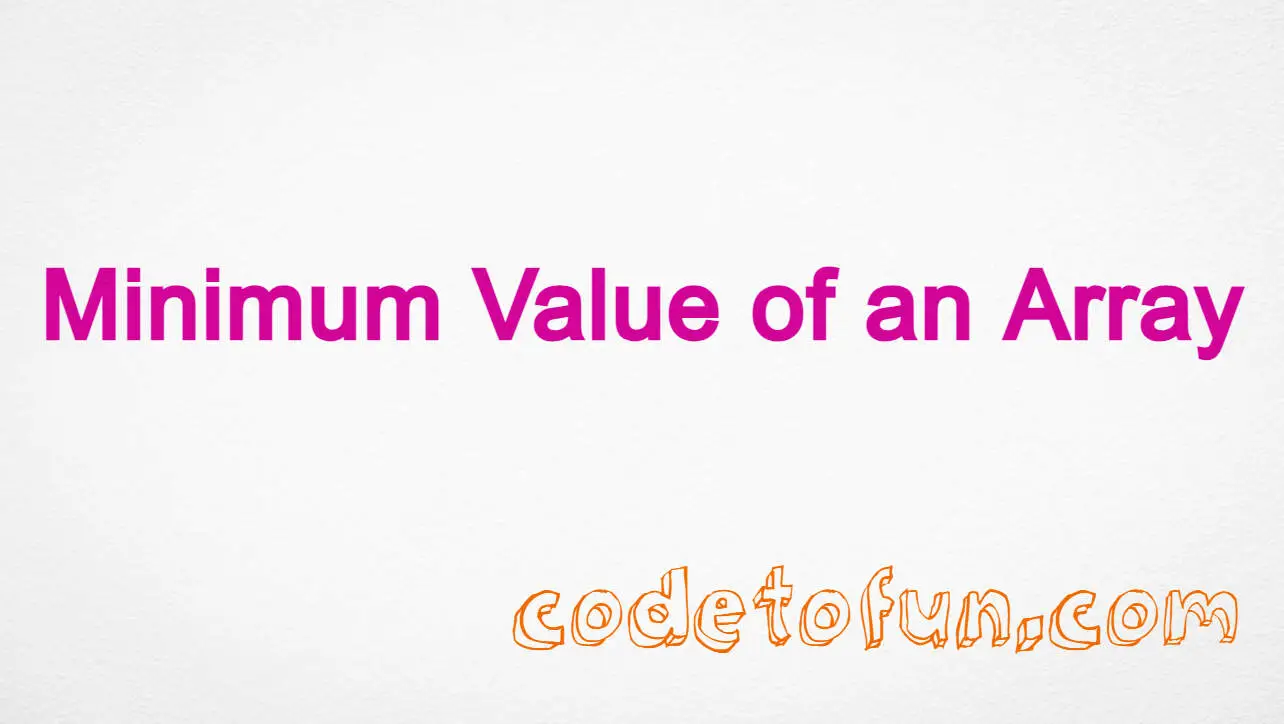
If you have any doubts regarding this article (C strrchr() Function) please comment here. I will help you immediately.