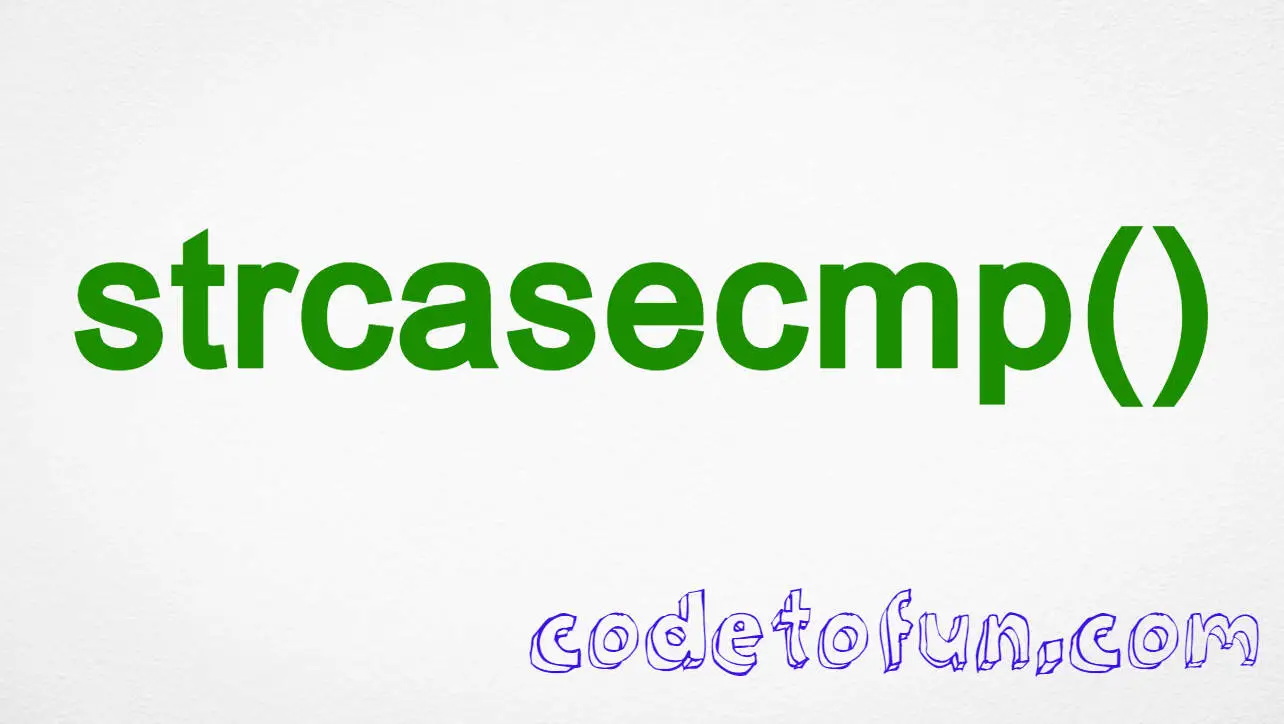
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strchr() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In the C programming language, strings are handled as arrays of characters, and various functions are available to manipulate and search within them.
The strchr()
function is one such function that searches for the first occurrence of a character in a given string and returns a pointer to that character.
In this tutorial, we'll explore the usage and functionality of the strchr()
function in C.
đĄ Syntax
The syntax for the strchr()
function is as follows:
char *strchr(const char *str, int character);
- str: The pointer to the null-terminated string in which the search will be performed.
- character: The character to be located. This is passed as an integer, but it is commonly specified using single quotes (e.g., a).
đ Example
Let's dive into an example to illustrate how the strchr()
function works.
#include <stdio.h>
int main() {
const char * sampleString = "Hello, C Programming!";
char searchChar = 'C';
// Find the first occurrence of 'C' in the string
char * result = strchr(sampleString, searchChar);
if (result != NULL) {
printf("Character '%c' found at position: %ld\n", searchChar, result - sampleString);
} else {
printf("Character '%c' not found in the string.\n", searchChar);
}
return 0;
}
đģ Output
Character 'C' found at position: 7
đ§ How the Program Works
In this example, the strchr()
function is used to find the first occurrence of the character 'C' in the string "Hello, C Programming!".
âŠī¸ Return Value
The strchr()
function returns a pointer to the first occurrence of the specified character in the given string. If the character is not found, it returns NULL.
đ Common Use Cases
The strchr()
function is particularly useful when you need to locate the position of a specific character within a string. It is commonly used in tasks such as parsing strings or extracting information.
đ Notes
- The
strchr()
function is case-sensitive. It differentiates between uppercase and lowercase letters. - If you need a case-insensitive search, you can use the
strchr()
function in combination with functions like tolower() or toupper() to convert characters to a consistent case.
đĸ Optimization
The strchr()
function is optimized for efficiency, and no specific optimizations are typically needed. It is a standard library function that provides a reliable and fast way to search for characters in strings.
đ Conclusion
The strchr()
function in C is a valuable tool for searching for the first occurrence of a character in a string. It is versatile and widely used in various string manipulation tasks.
Feel free to experiment with different strings and characters to deepen your understanding of the strchr()
function. Happy coding!
đ¨âđģ Join our Community:
Author
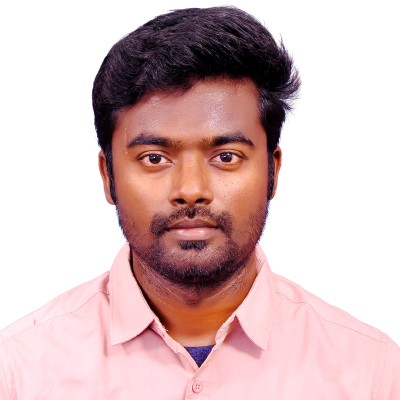
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
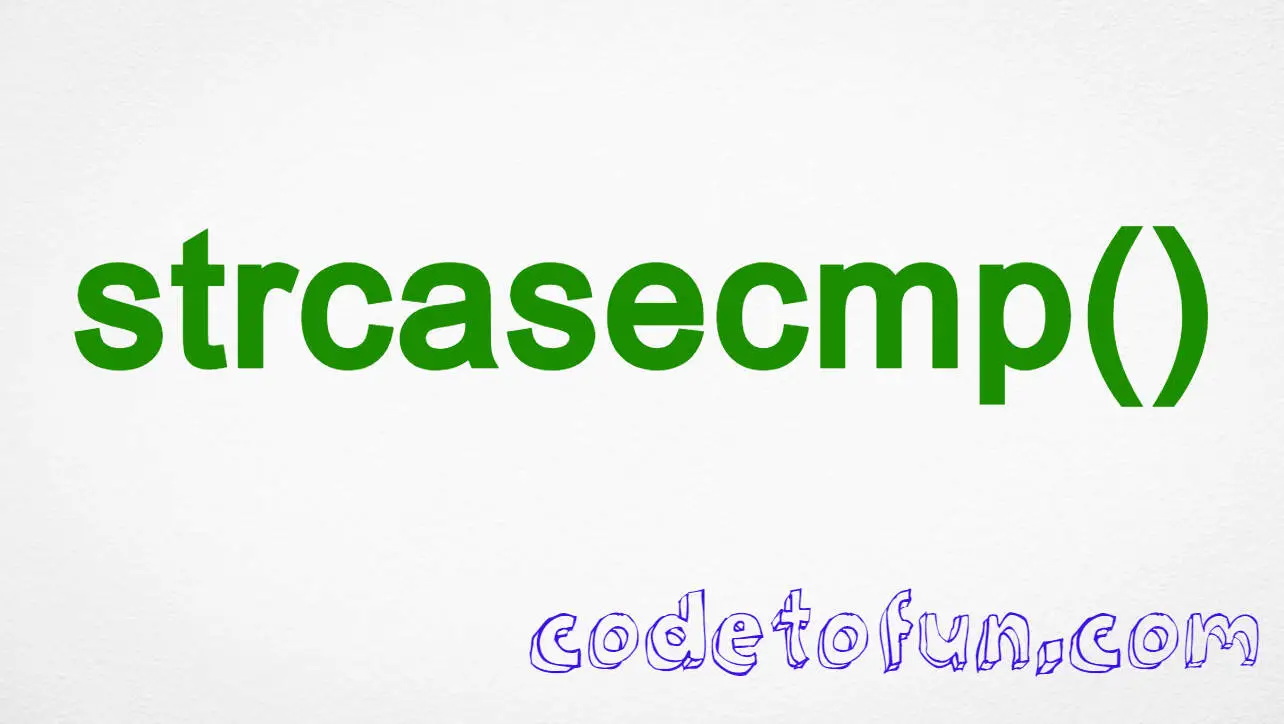
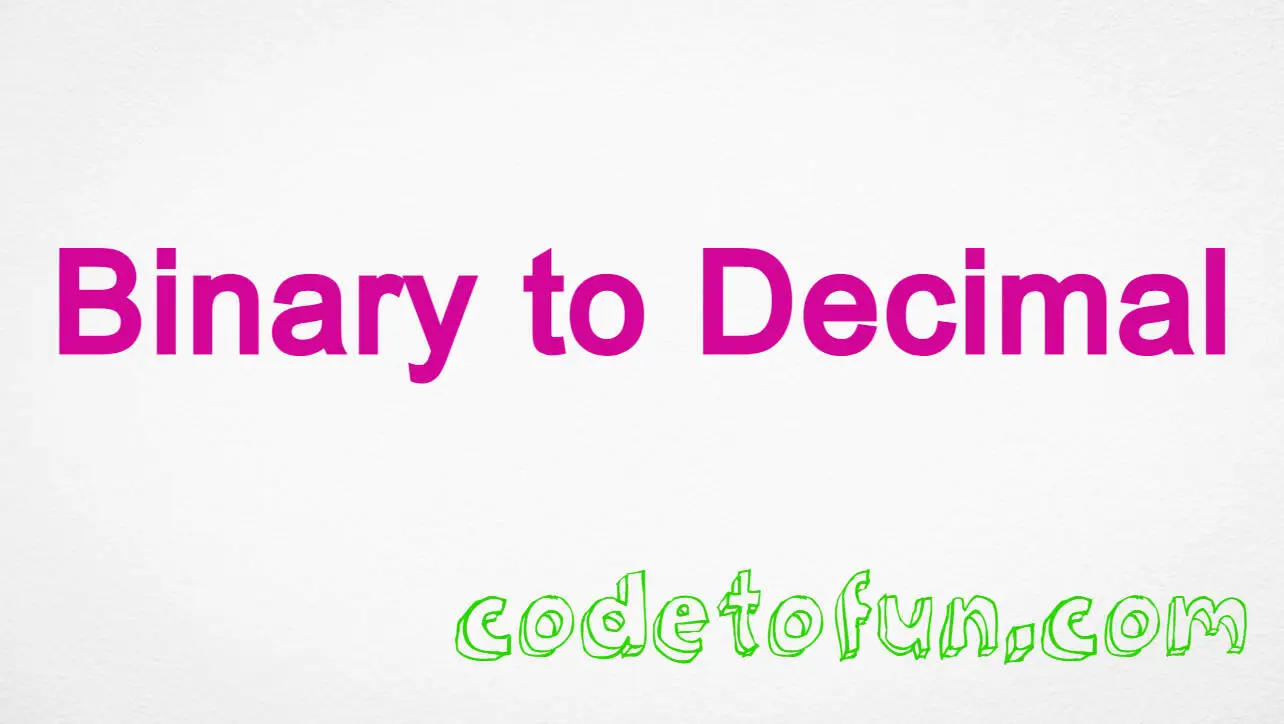
C Program to Converter a Binary to Decimal
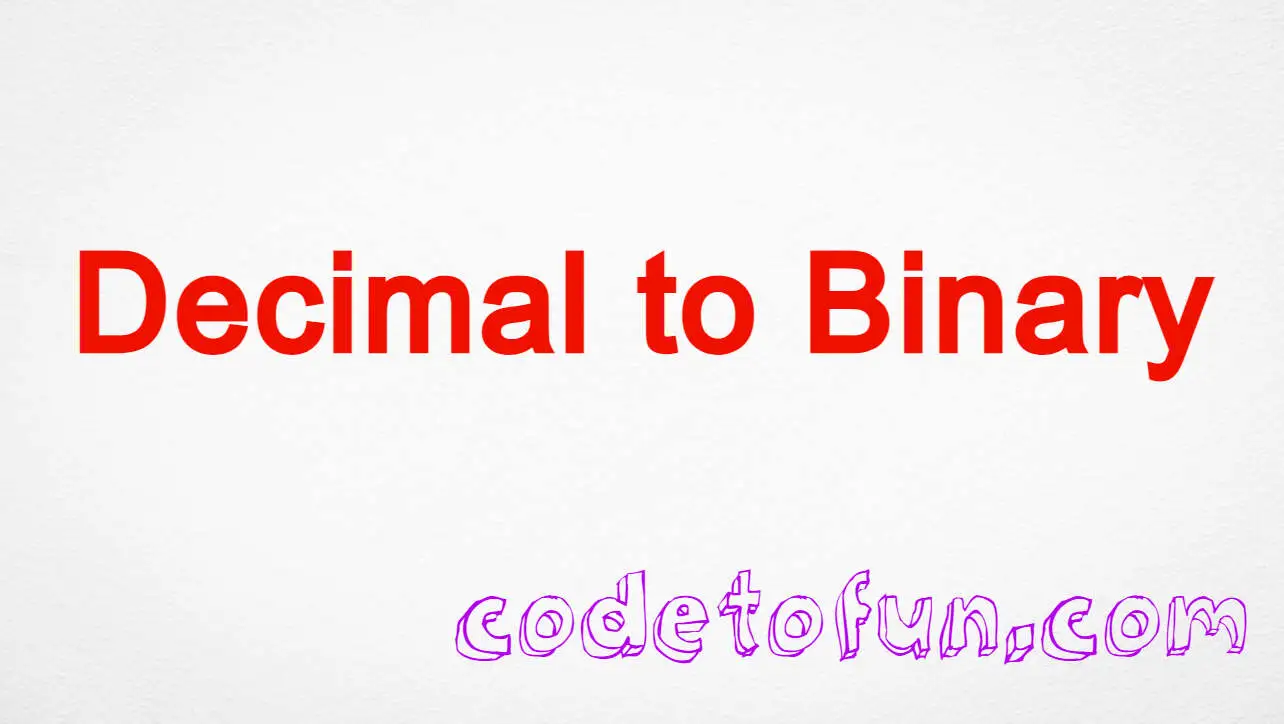
C Program to Converter a Decimal to Binary
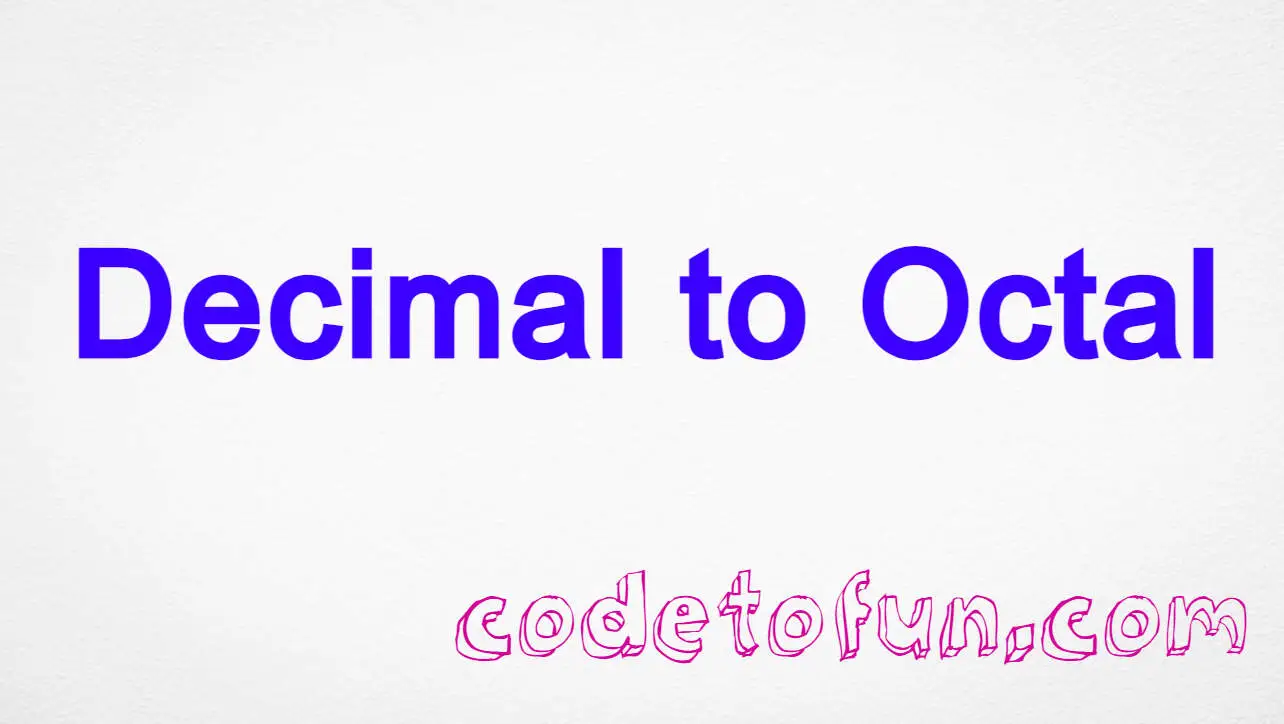
C Program to Converter a Decimal to Octal
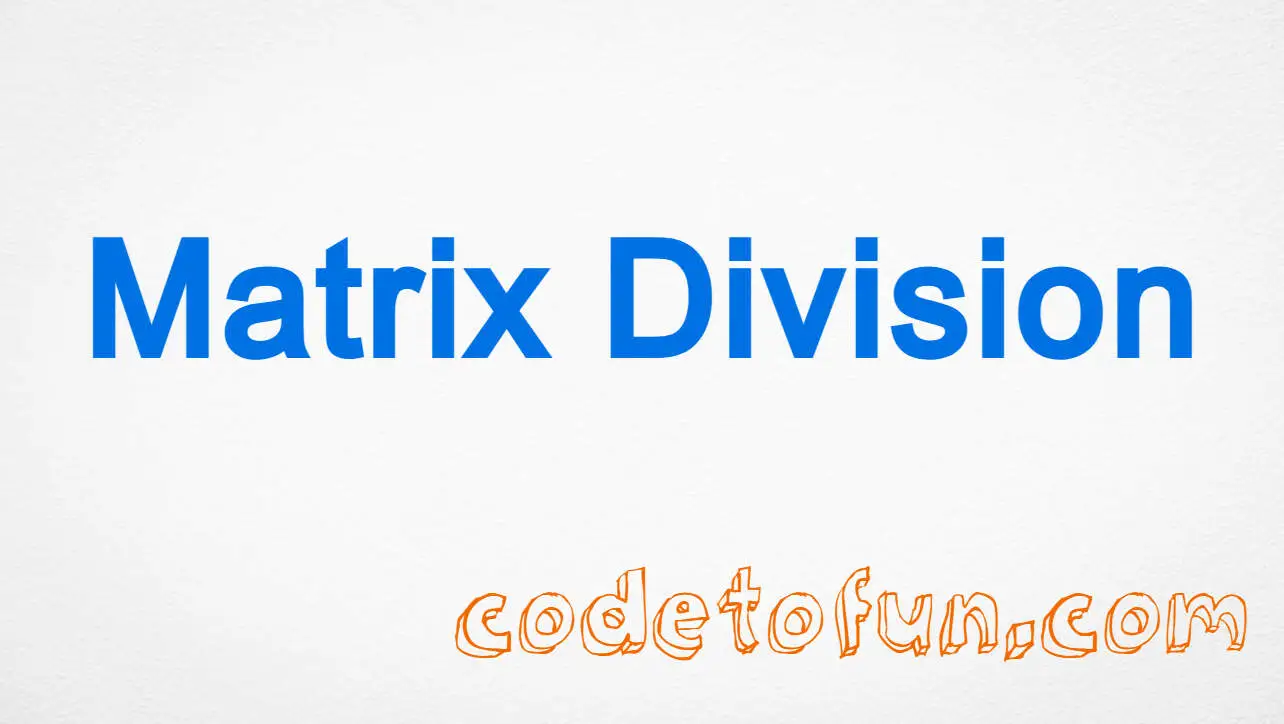
C Program to Perform Matrix Division
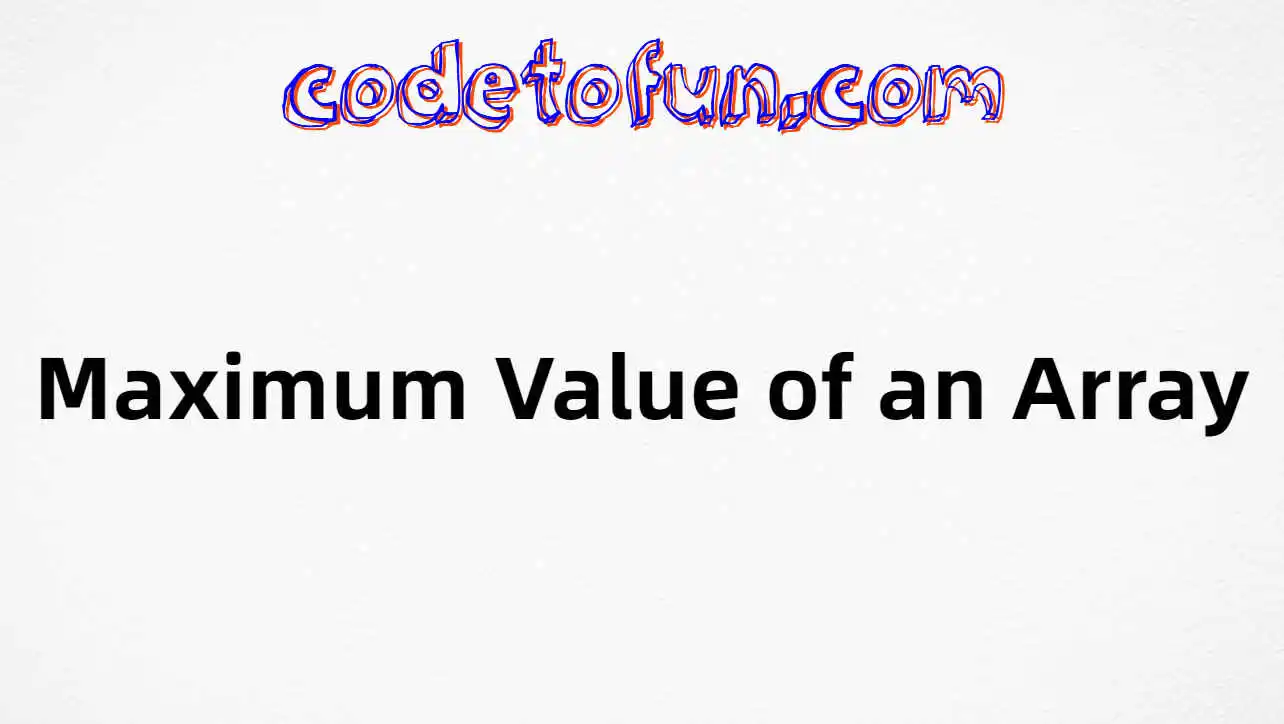
C Program to find Maximum Value of an Array
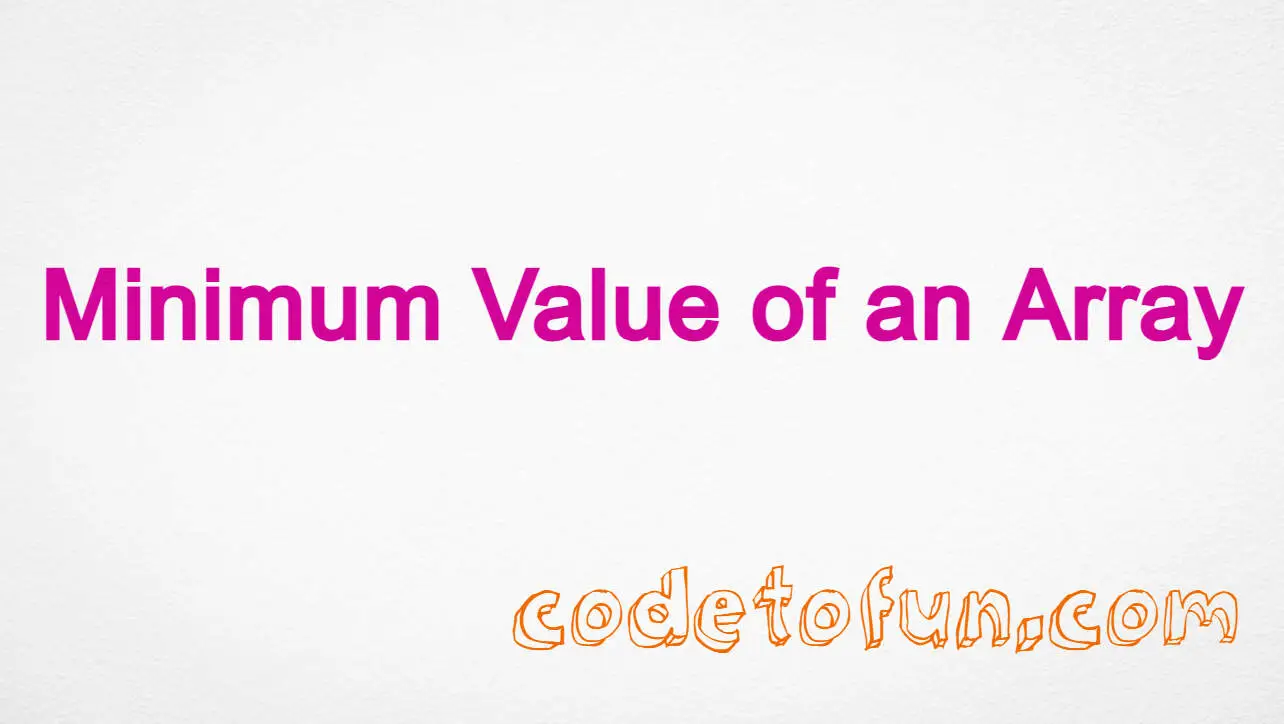
If you have any doubts regarding this article (C strchr() Function) please comment here. I will help you immediately.