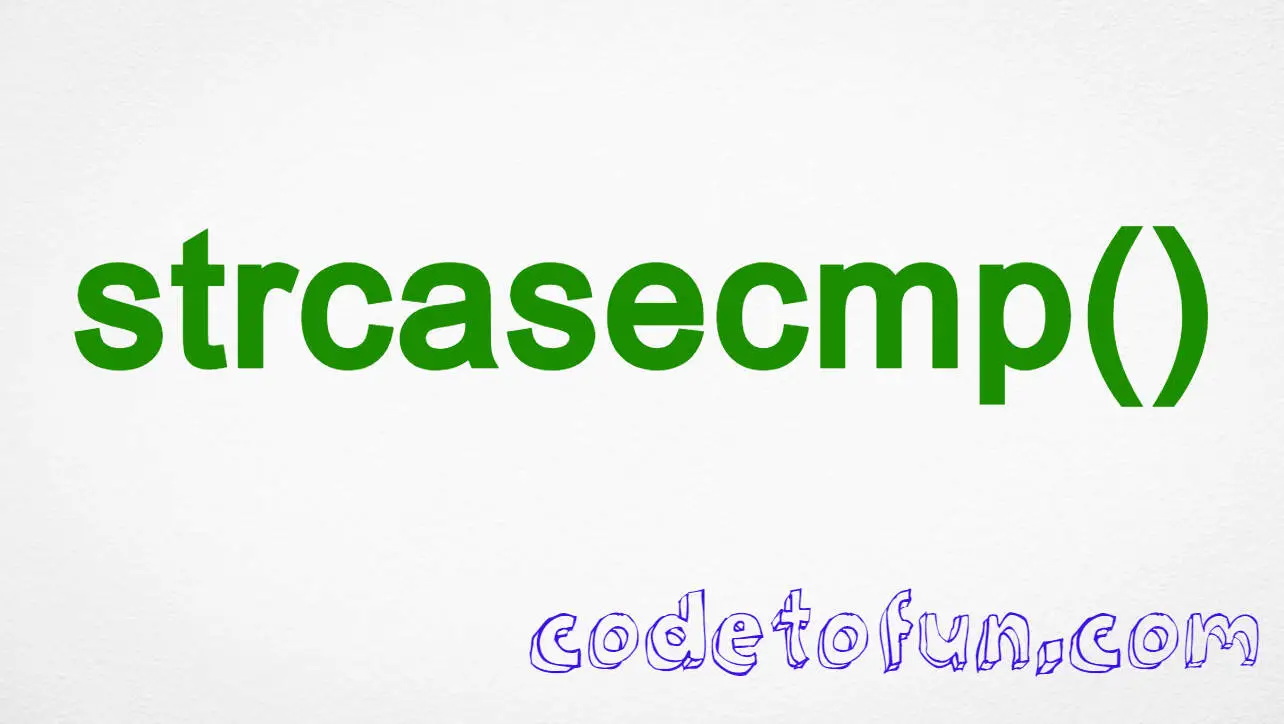
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strcat() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, working with strings is a common and fundamental task.
The strcat()
function, short for "string concatenate," is a standard library function that allows you to concatenate (append) one string to another.
In this tutorial, we'll explore the usage and functionality of the strcat()
function in C.
đĄ Syntax
The signature of the strcat()
function is as follows:
char *strcat(char *dest, const char *src);
This function takes two arguments: dest, which is the destination string (the string to which the source string will be appended), and src, the source string (the string that will be appended to the destination).
đ Example
Let's delve into an example to illustrate how the strcat()
function works.
#include <stdio.h>
#include <string.h>
int main() {
char destination[20] = "Hello, ";
const char * source = "World!";
// Concatenate strings
strcat(destination, source);
// Output the result
printf("Concatenated String: %s\n", destination);
return 0;
}
đģ Output
Concatenated String: Hello, World!
đ§ How the Program Works
In this example, the strcat()
function appends the string "World!" to the existing string "Hello, " in the destination array.
âŠī¸ Return Value
The strcat()
function returns a pointer to the destination string (dest). It is essential to note that the resulting concatenated string is stored in the destination string itself.
đ Common Use Cases
The strcat()
function is particularly useful when you need to combine two strings to create a single, longer string. It is commonly used in scenarios where you want to build strings dynamically during runtime.
đ Notes
- Ensure that the destination string (dest) has sufficient space to accommodate the concatenated result. Overlapping strings should be avoided.
- The
strcat()
function does not perform boundary checking, and care must be taken to avoid buffer overflow.
đĸ Optimization
While the strcat()
function is a standard and efficient way to concatenate strings, consider using safer alternatives like strncat() if you need to control the number of characters appended or if you want to ensure null-termination in all cases.
đ Conclusion
The strcat()
function in C is a powerful tool for string concatenation. It simplifies the process of combining strings, making it easier to manipulate and construct dynamic strings in your programs.
Feel free to experiment with different strings and explore the behavior of the strcat()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
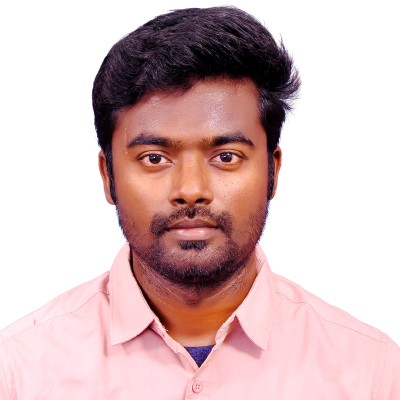
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
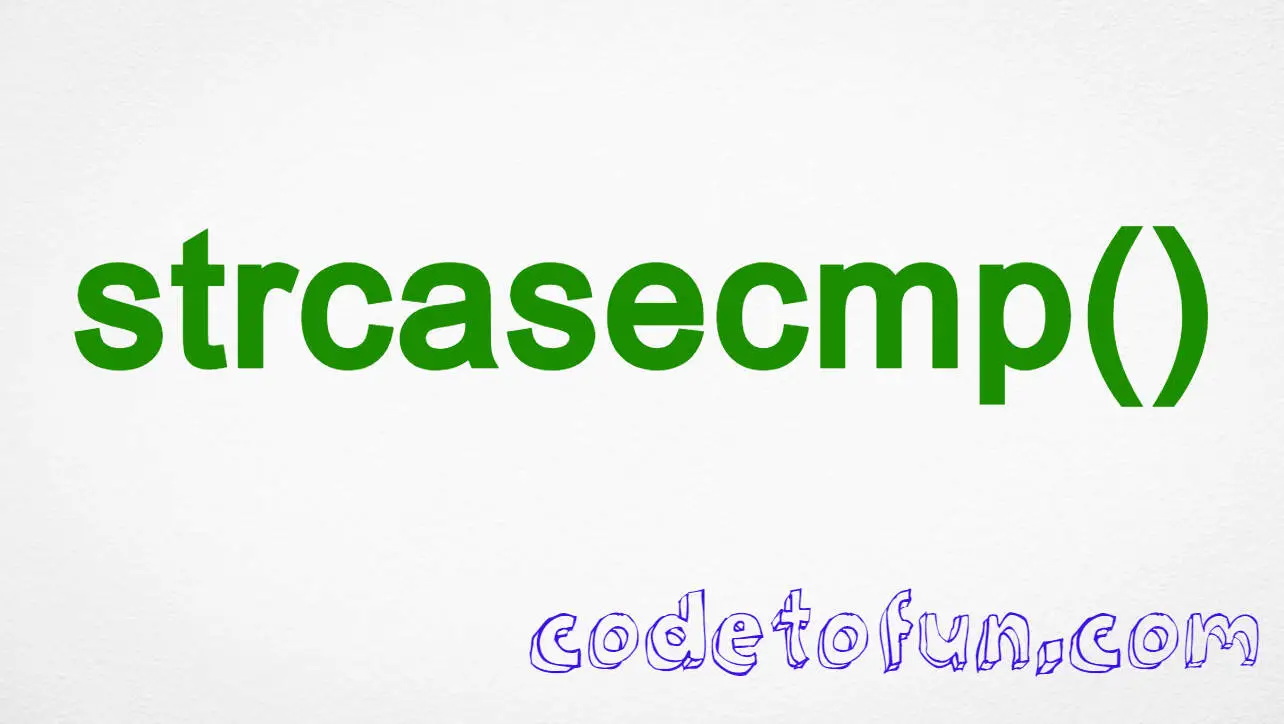
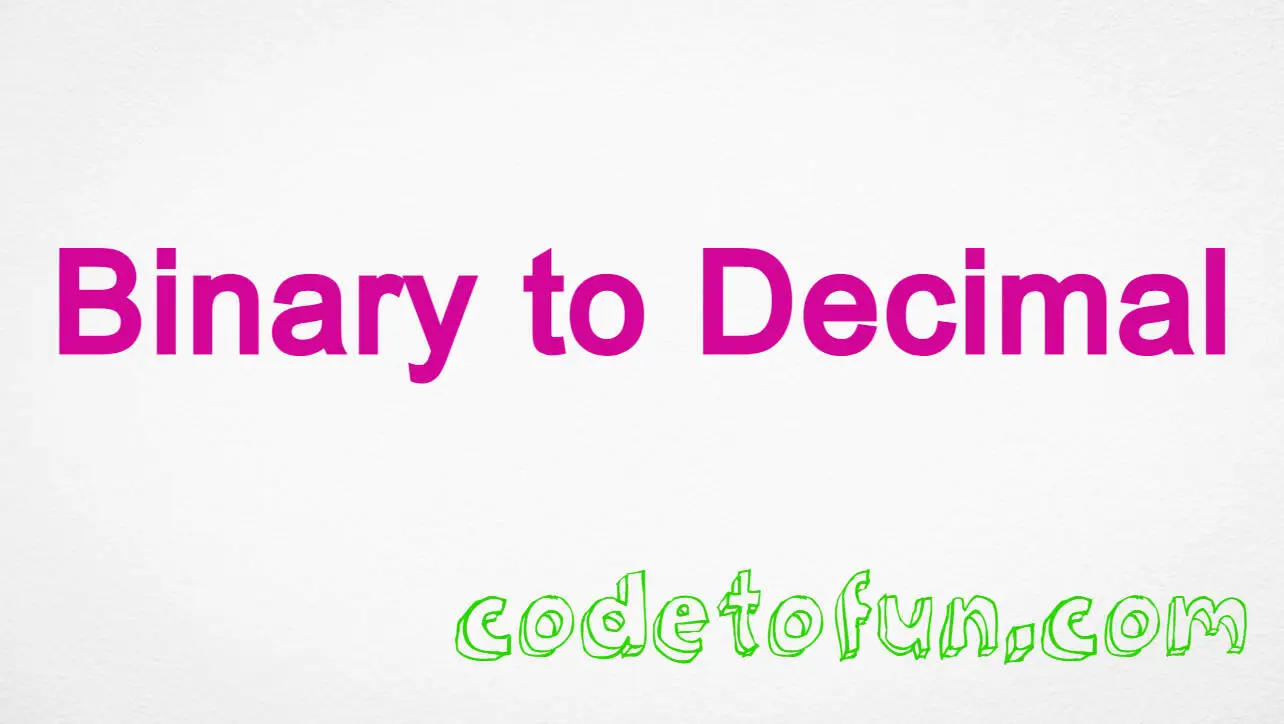
C Program to Converter a Binary to Decimal
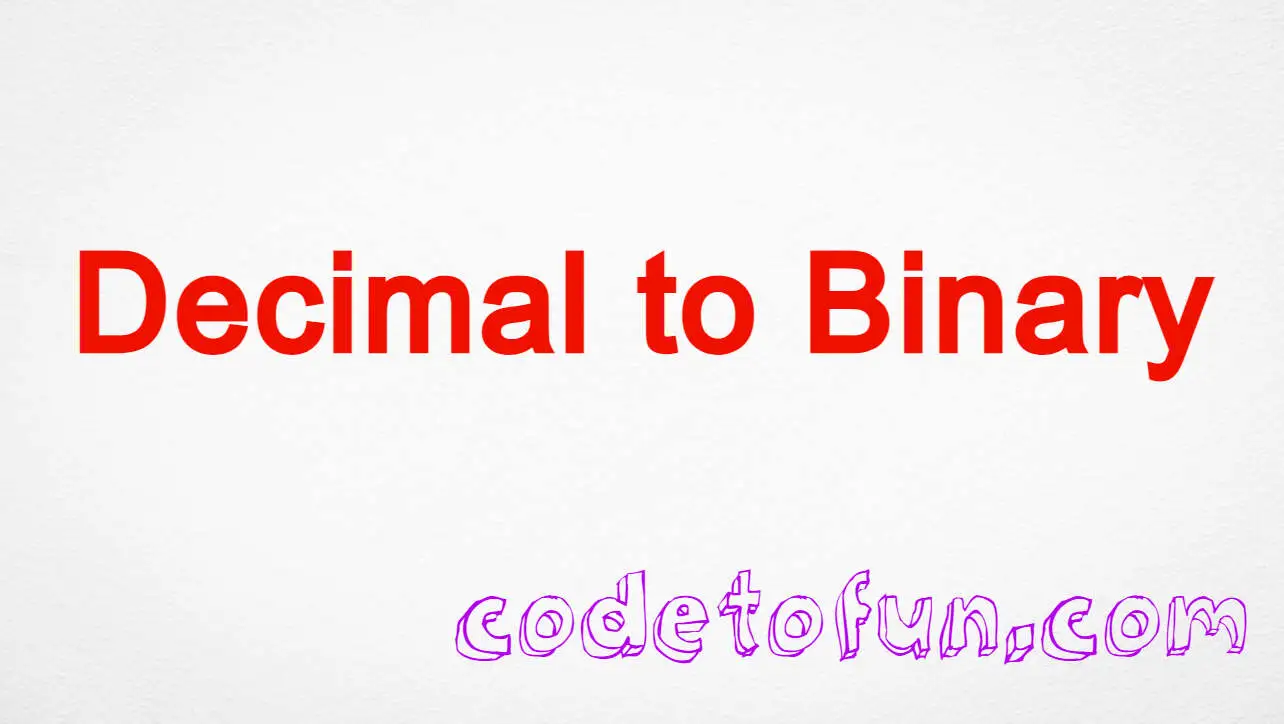
C Program to Converter a Decimal to Binary
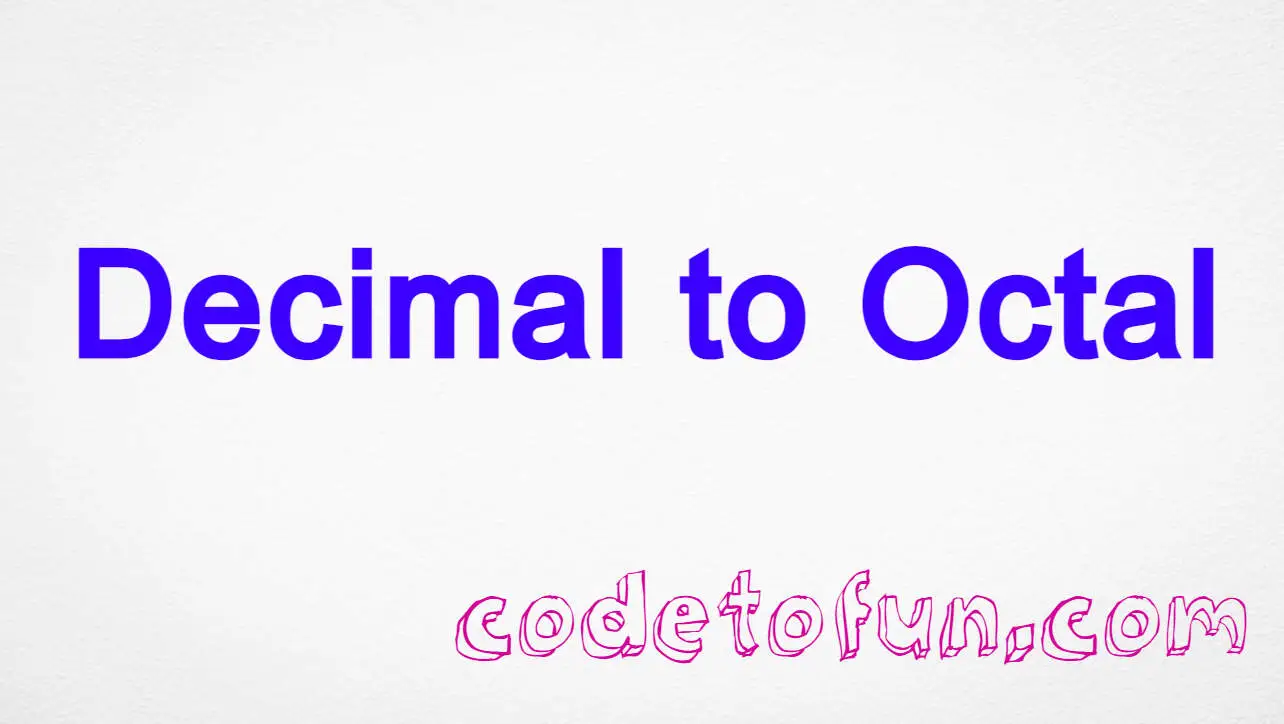
C Program to Converter a Decimal to Octal
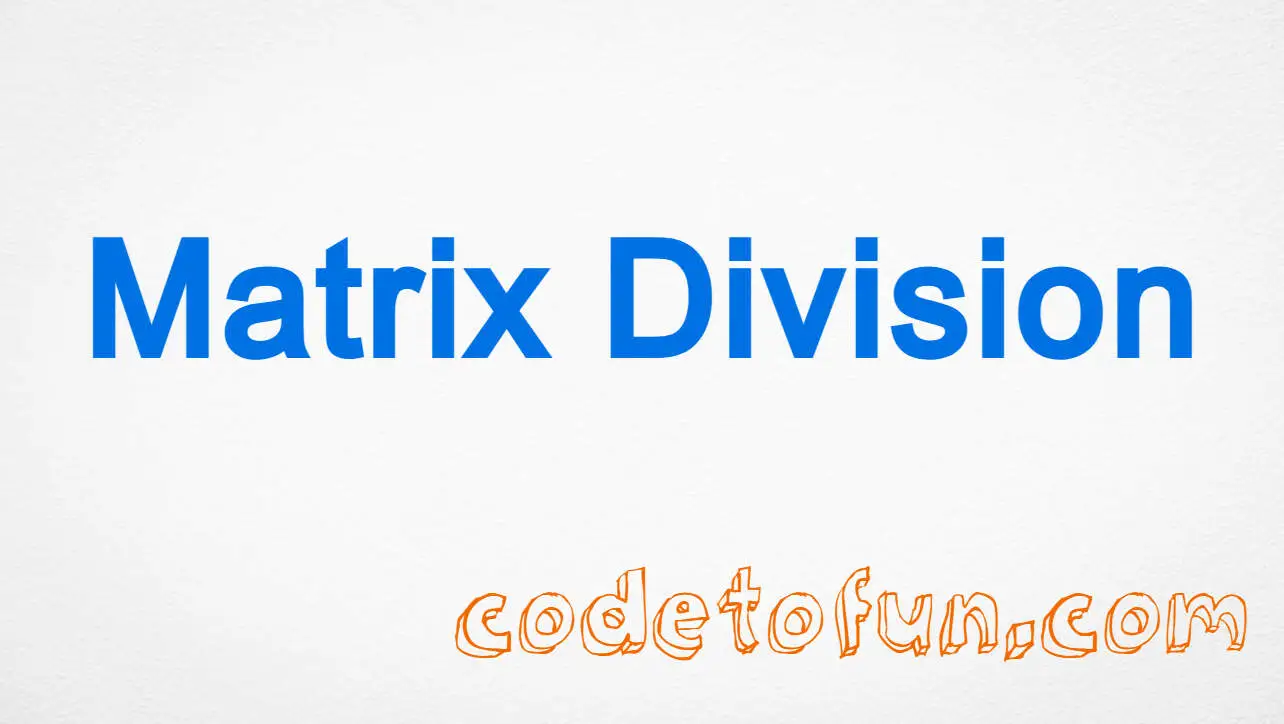
C Program to Perform Matrix Division
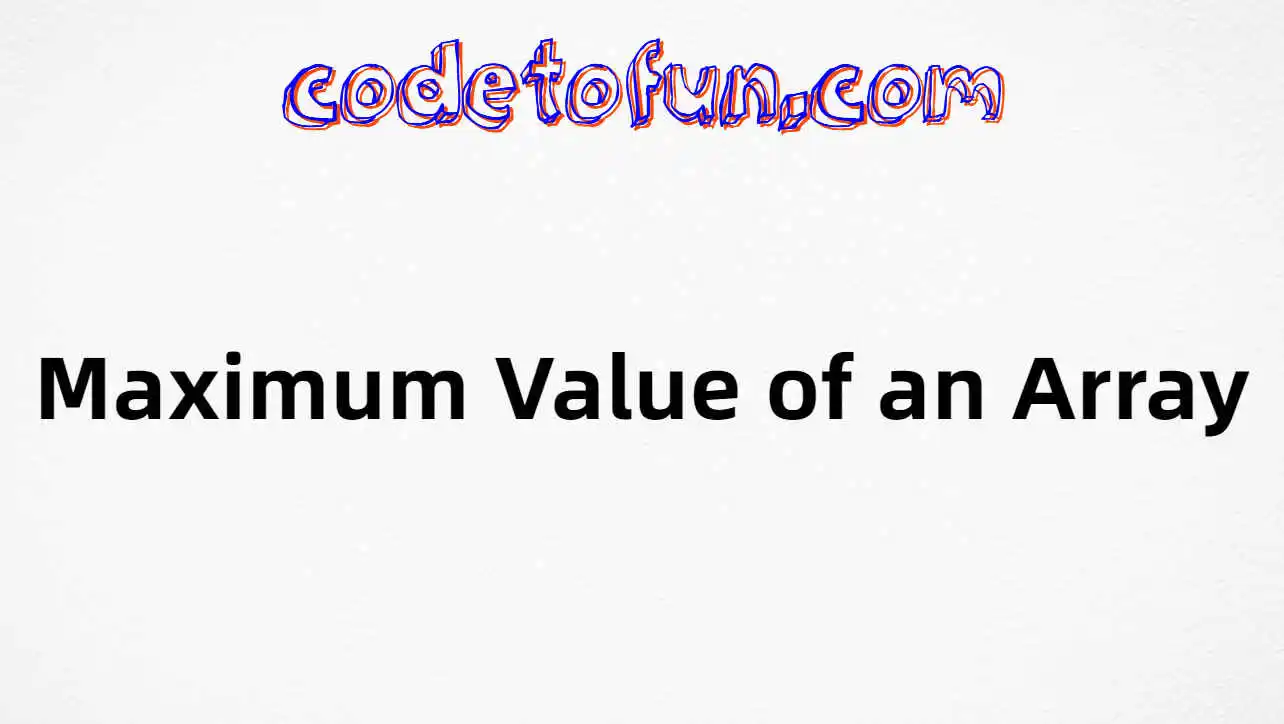
C Program to find Maximum Value of an Array
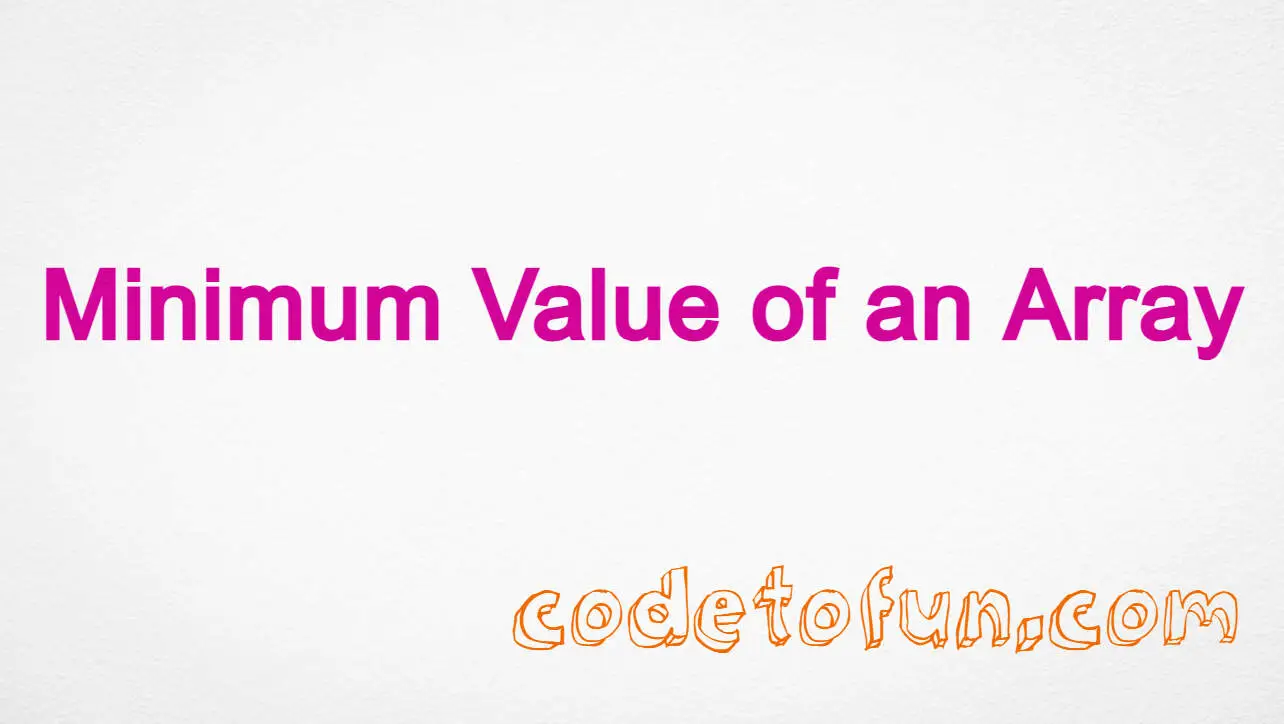
If you have any doubts regarding this article (C strcat() Function) please comment here. I will help you immediately.