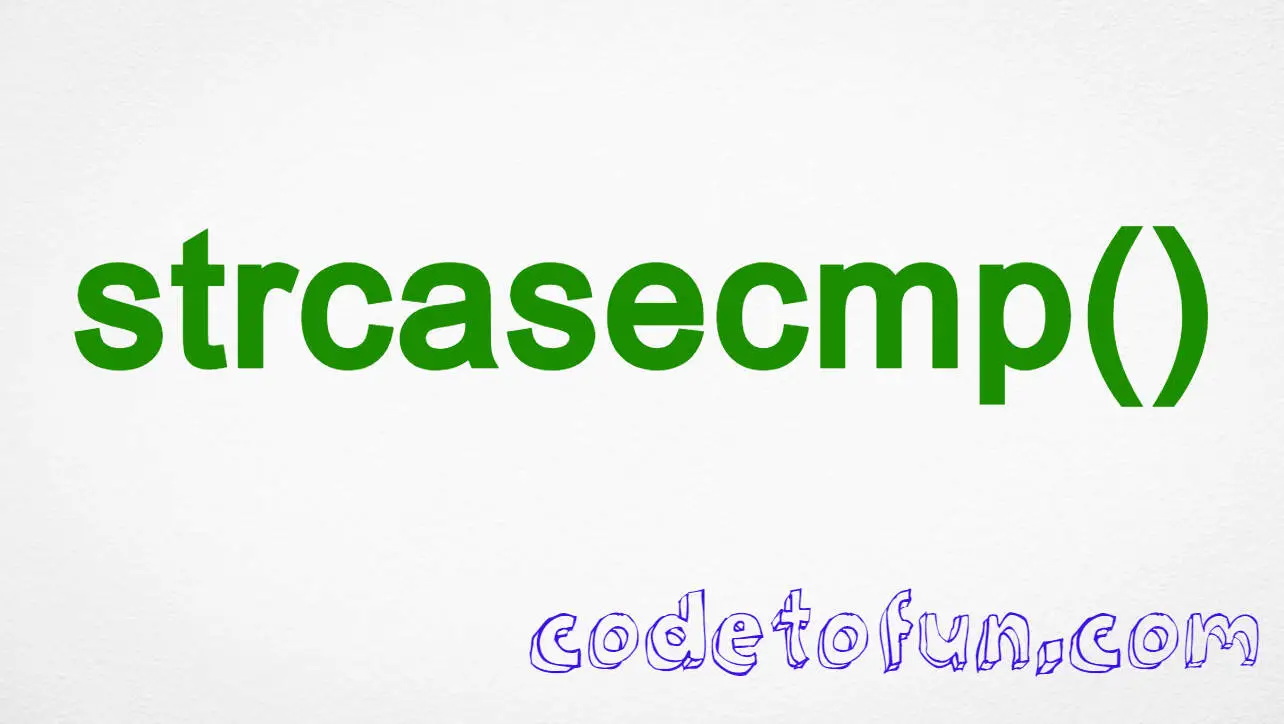
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Perform Matrix Division
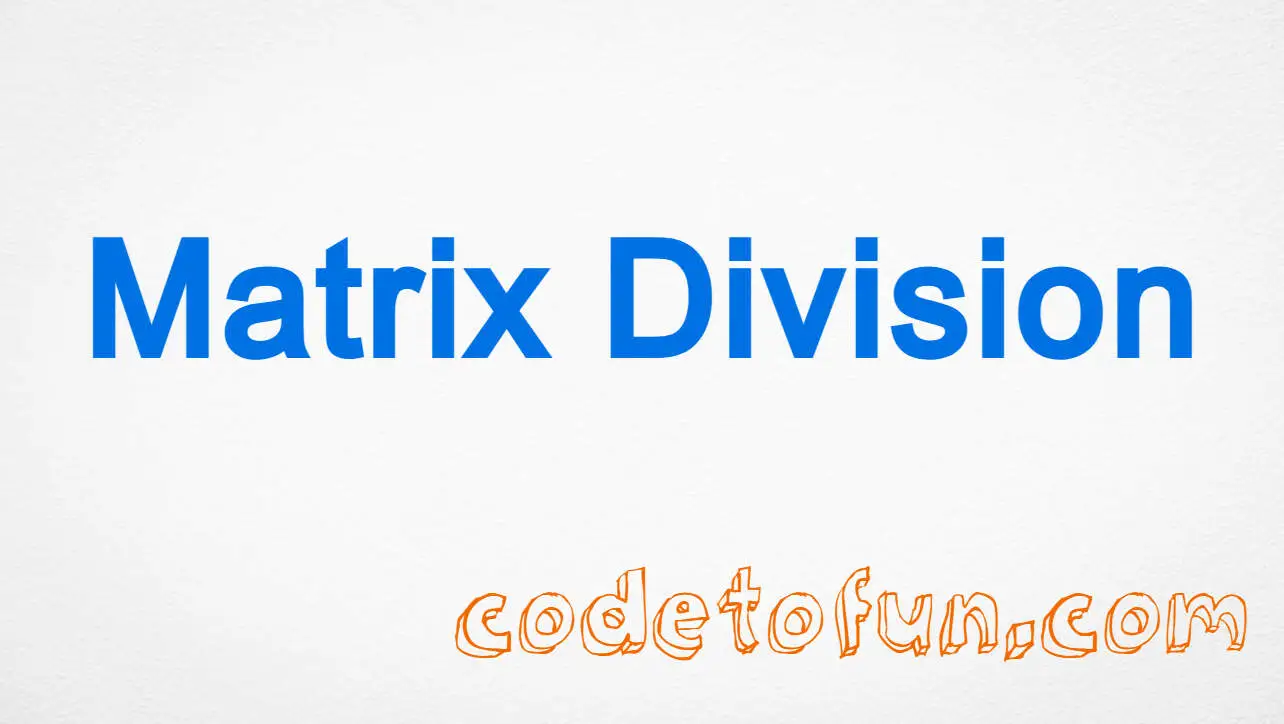
Photo Credit to CodeToFun
π Introduction
Matrix operations are fundamental in linear algebra and various scientific and engineering applications. One essential matrix operation is division.
In this tutorial, we'll explore a C program that performs matrix division, providing a foundational understanding of how to handle matrices in a programming context.
π Example
Let's delve into the C code that performs matrix division.
#include <stdio.h>
// Function to print a matrix
void printMatrix(int rows, int cols, float matrix[rows][cols]) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
printf("%0.2f\t", matrix[i][j]);
}
printf("\n");
}
}
// Function to perform matrix division
void matrixDivision(int rows, int cols, float matrixA[rows][cols], float matrixB[rows][cols]) {
float result[rows][cols];
// Check if matrixB is invertible
// Additional logic for inverse calculation is required here
// Perform matrix division
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result[i][j] = matrixA[i][j] / matrixB[i][j];
}
}
// Print the result
printf("Result of matrix division:\n");
printMatrix(rows, cols, result);
}
// Driver program
int main() {
// Define matrices A and B
float matrixA[2][2] = {{4.0, 8.0},{2.0, 6.0}};
float matrixB[2][2] = {{2.0, 4.0},{1.0, 3.0}};
// Call the function to perform matrix division
matrixDivision(2, 2, matrixA, matrixB);
return 0;
}
π» Testing the Program
To test the program with different matrices, replace the values of matrixA and matrixB in the main function.
Result of matrix division: 2.00 2.00 2.00 2.00
Compile and run the program to see the result of matrix division.
π§ How the Program Works
- The program defines a function printMatrix to print a matrix for better visualization.
- The matrixDivision function checks if matrix B is invertible (additional logic for inverse calculation is required).
- It performs matrix division element-wise and prints the result.
π§ Understanding the Concept of Matrix Division
Matrix division is not a straightforward operation like addition or multiplication.
Matrix division involves multiplying one matrix by the inverse of another. Ensure that the second matrix is invertible, and additional logic for inverse calculation may be required.
For two matrices A and B, the division A/B is equivalent to A * B^(-1), where B^(-1) is the inverse of matrix B.
π’ Optimizing the Program
This basic example assumes a 2x2 matrix for simplicity. For larger matrices, you may need to implement a robust algorithm for matrix inversion.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
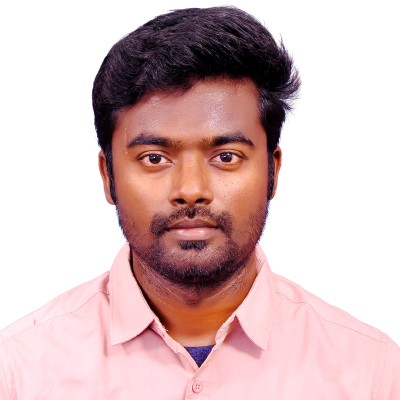
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
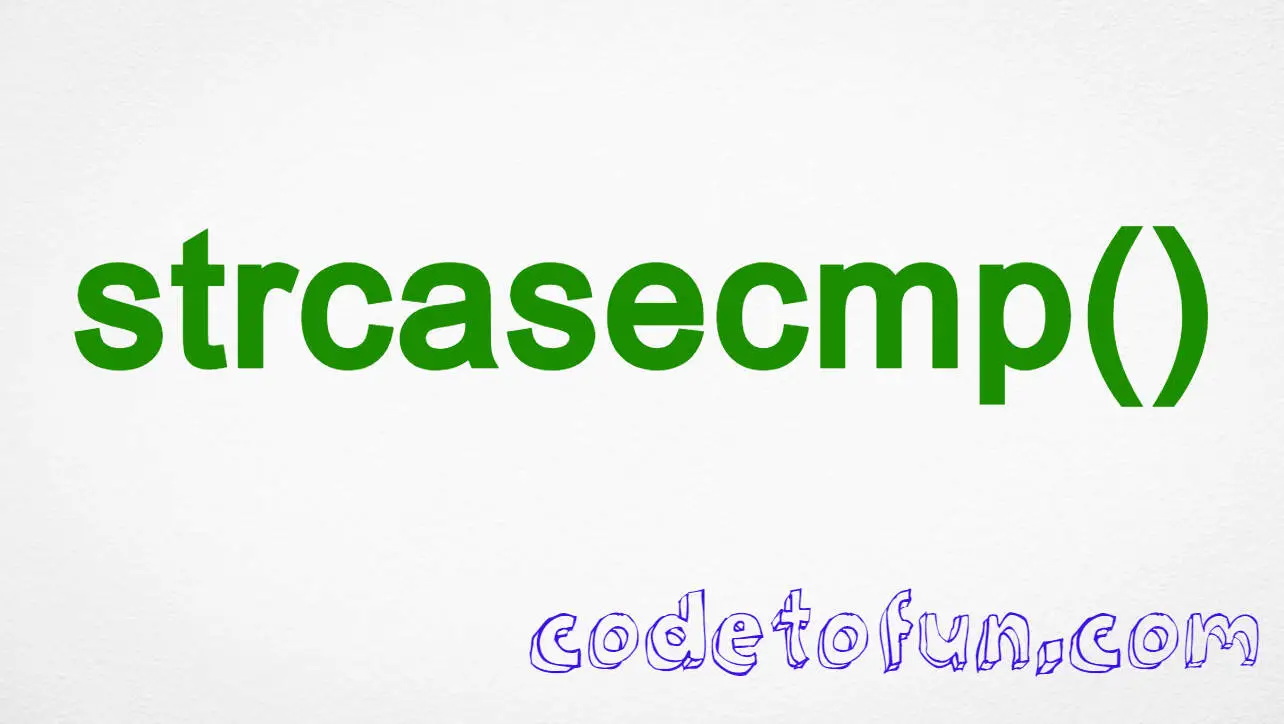
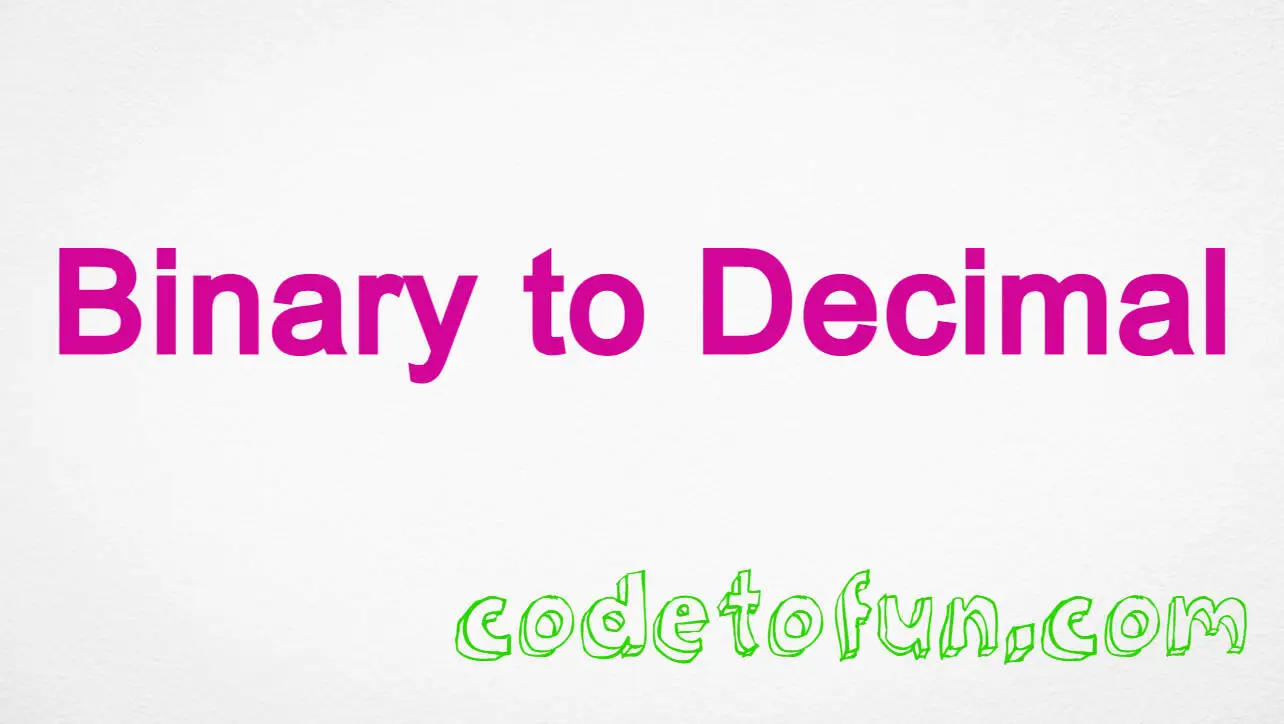
C Program to Converter a Binary to Decimal
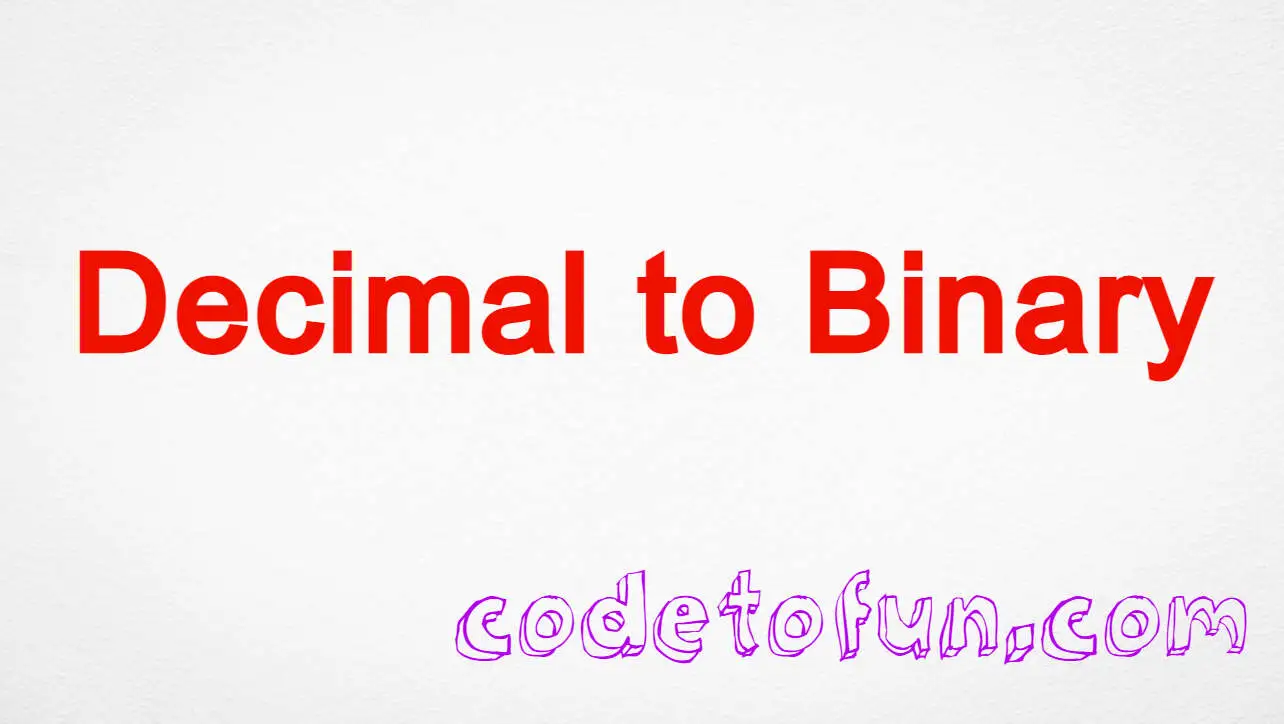
C Program to Converter a Decimal to Binary
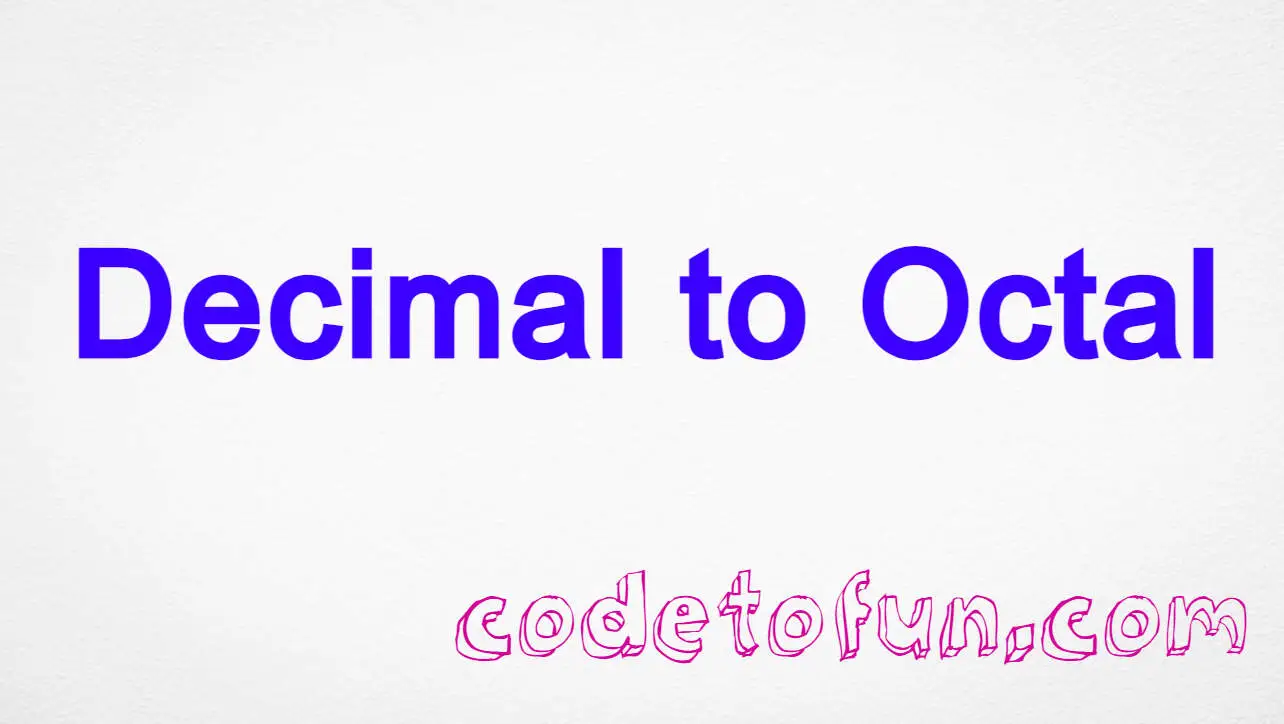
C Program to Converter a Decimal to Octal
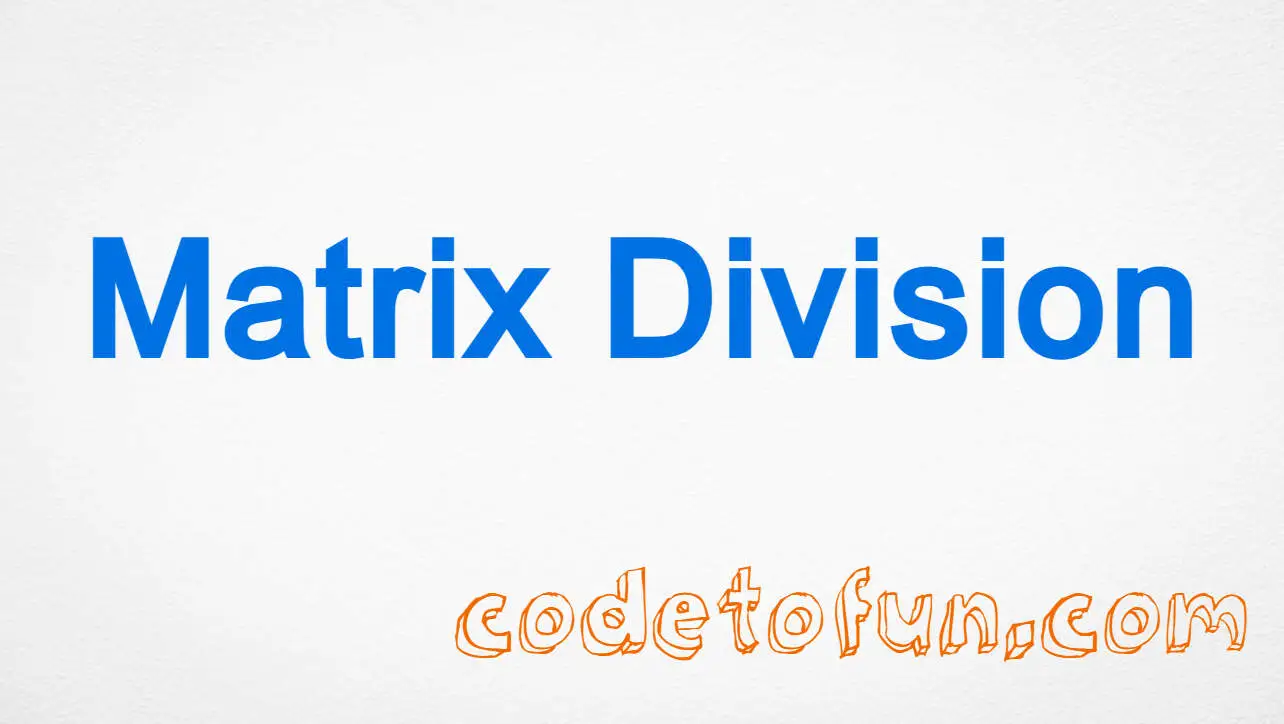
C Program to Perform Matrix Division
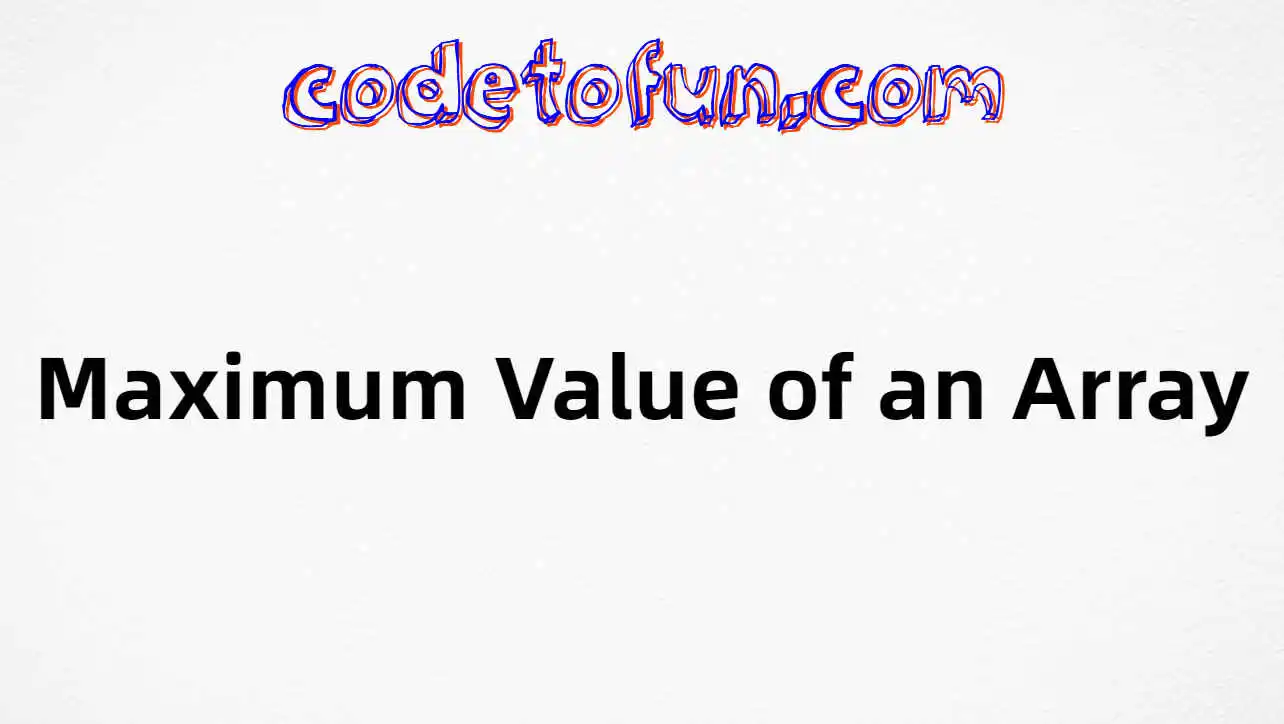
C Program to find Maximum Value of an Array
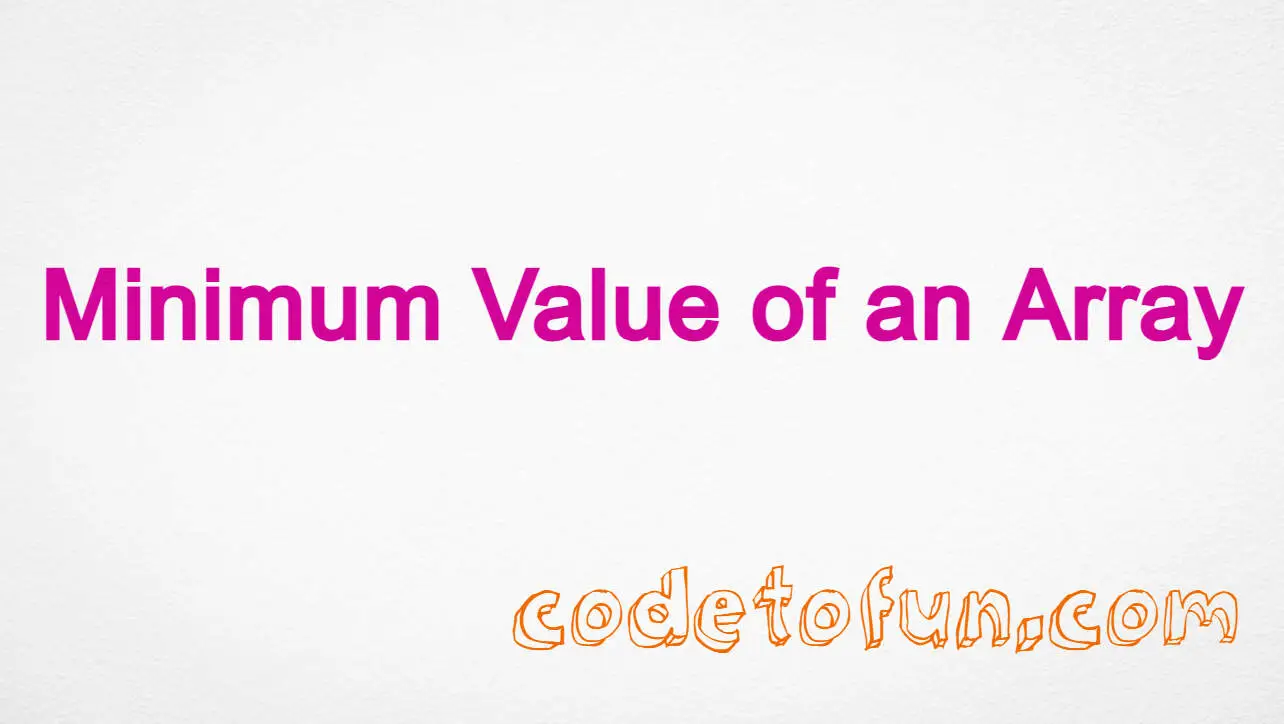
If you have any doubts regarding this article (C Program to Perform Matrix Division), please comment here. I will help you immediately.