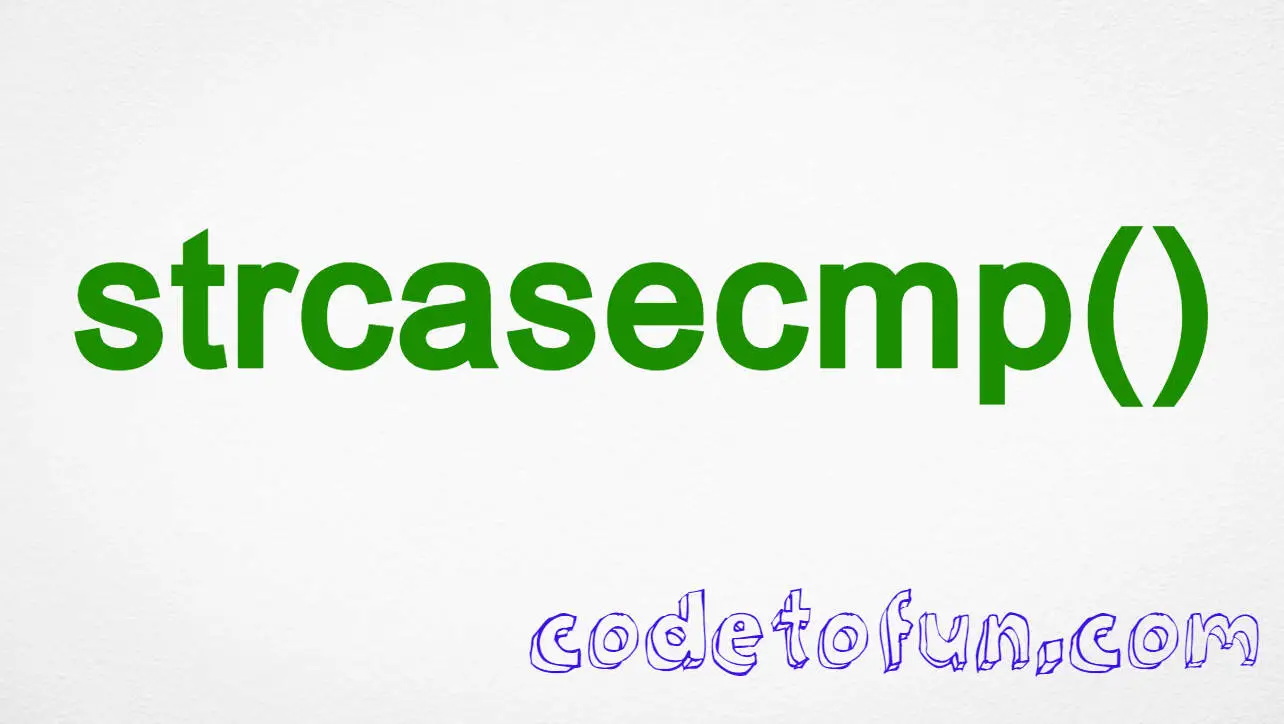
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Check Power of 3
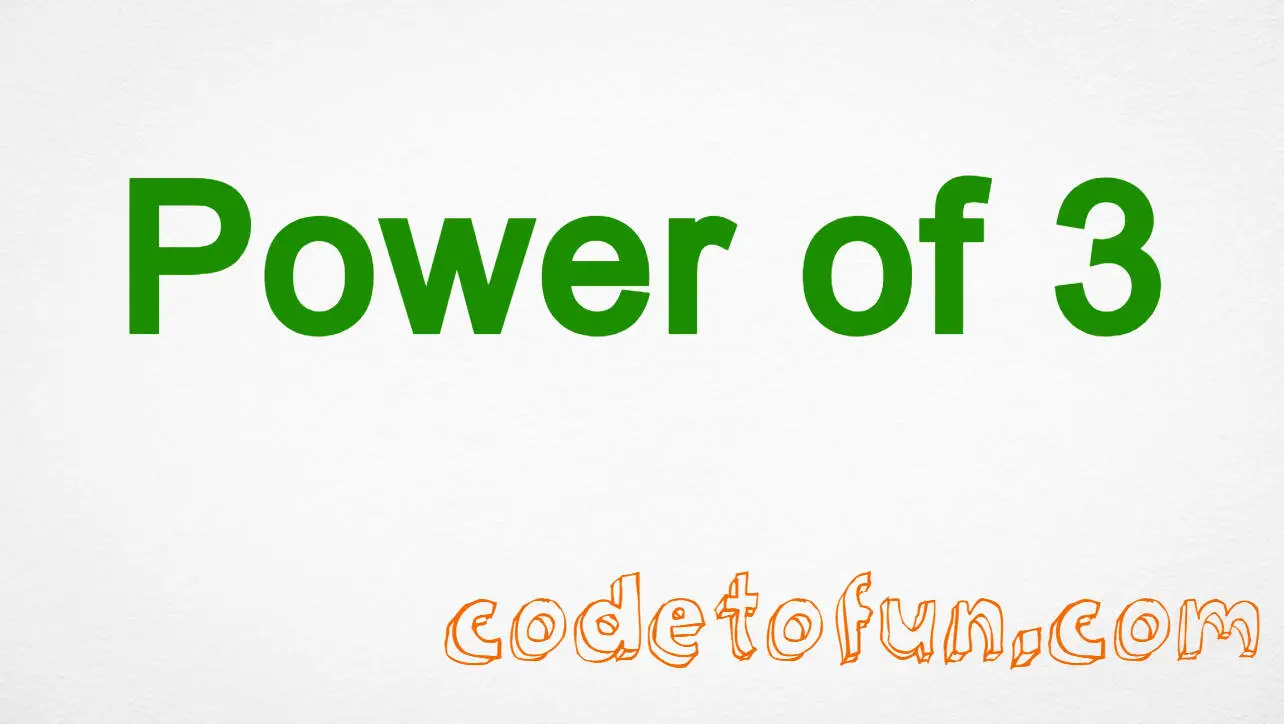
Photo Credit to CodeToFun
π Introduction
In the realm of programming, one often encounters the need to check if a given number is a power of another.
In this case, we'll explore a C program specifically designed to check if a number is a power of 3.
The logic behind this program involves repeatedly dividing the number by 3 until it becomes 1, indicating that it is a power of 3.
π Example
Let's delve into the C code that achieves this functionality.
#include <stdio.h>
// Function to check if a number is a power of 3
int isPowerOf3(int n) {
// Keep dividing the number by 3 until it is greater than 1
while (n % 3 == 0 && n > 1) {
n /= 3;
}
// If the final value is 1, the original number is a power of 3
return n == 1;
}
// Driver program
int main() {
// Replace this value with your desired number
int number = 27;
// Check if the number is a power of 3
if (isPowerOf3(number)) {
printf("%d is a power of 3\n", number);
} else {
printf("%d is not a power of 3\n", number);
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the main function.
27 is a power of 3
Compile and run the program to see if the given number is a power of 3.
π§ How the Program Works
- The program defines a function isPowerOf3 that takes an integer as input and checks if it is a power of 3.
- Inside the function, it repeatedly divides the number by 3 until it becomes greater than 1.
- If the final value is 1, the original number is a power of 3, and the function returns true; otherwise, it returns false.
- The driver program in the main function tests a specific number and prints whether it is a power of 3 or not.
π Between the Given Range
Let's take a look at the C code that checks for powers of 3 in the given range.
#include <stdio.h>
#include <stdbool.h>
// Function to check if a number is a power of 3
bool isPowerOfThree(int num) {
if (num <= 0) {
return false;
}
while (num % 3 == 0) {
num /= 3;
}
return num == 1;
}
// Driver program
int main() {
// Define the range from 1 to 20
int start = 1;
int end = 20;
printf("Power of 3 in the range %d to %d:\n", start, end);
// Check and print powers of 3 in the specified range
for (int i = start; i <= end; ++i) {
if (isPowerOfThree(i)) {
printf("%d ", i);
}
}
printf("\n");
return 0;
}
π» Testing the Program
Power of 3 in the range 1 to 20: 1 3 9
Compile and run the program to see the powers of 3 in the specified range.
π§ How the Program Works
- The program defines a function isPowerOfThree that checks whether a given number is a power of 3.
- Inside the function, it uses a while loop to repeatedly divide the number by 3 until it is no longer divisible by 3.
- The main function defines a range from 1 to 20 and prints the powers of 3 within that range.
π§ Understanding the Concept of Power of 3
Before diving into the code, let's understand the concept of a power of 3.
A number is considered a power of 3 if it can be expressed as 3^n, where n is an integer.
For example, 1, 3, 9, 27, and so on are powers of 3.
π’ Optimizing the Program
While the provided program is straightforward, there are alternative approaches to check if a number is a power of 3. Consider exploring other mathematical techniques for optimization.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
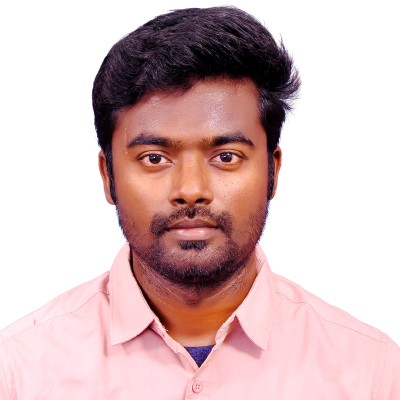
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
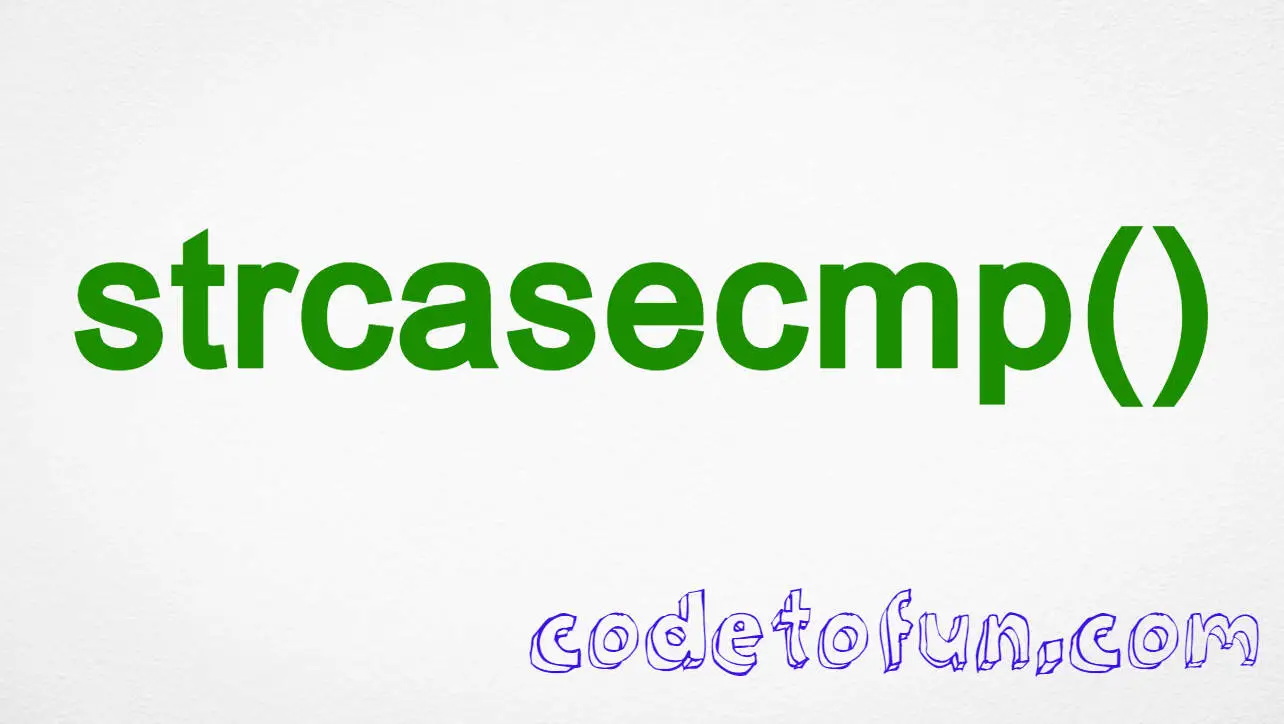
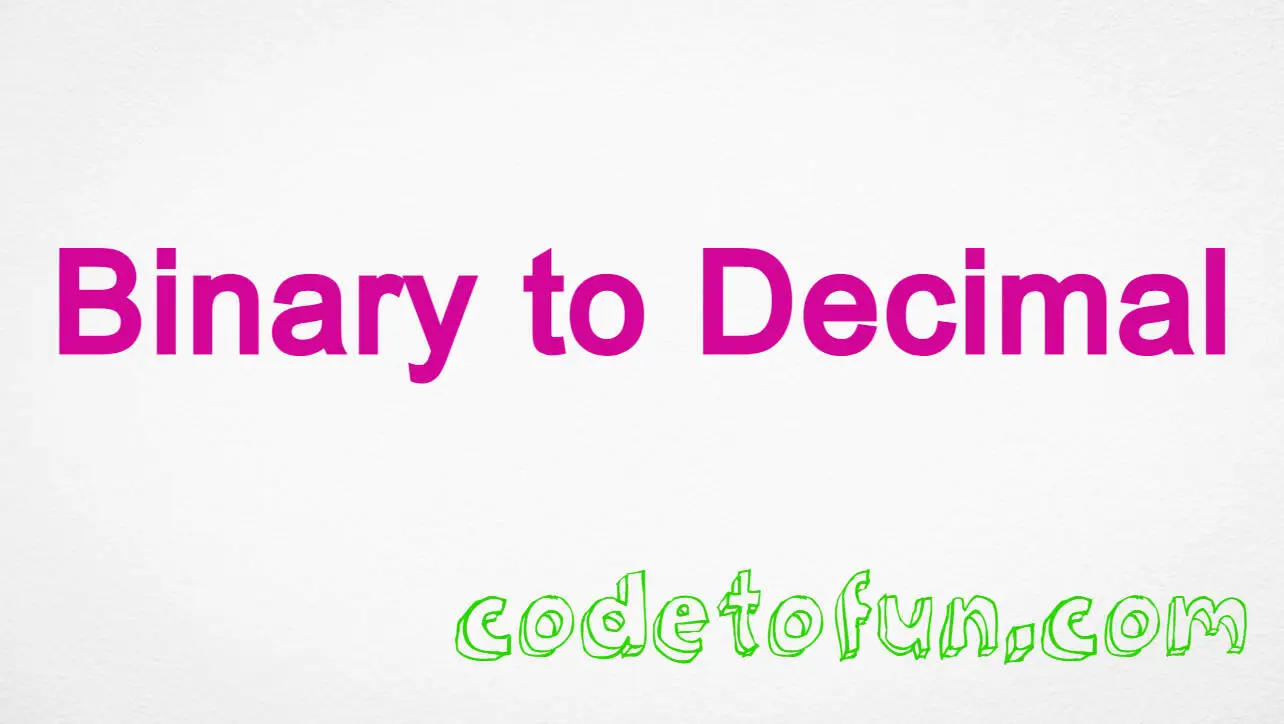
C Program to Converter a Binary to Decimal
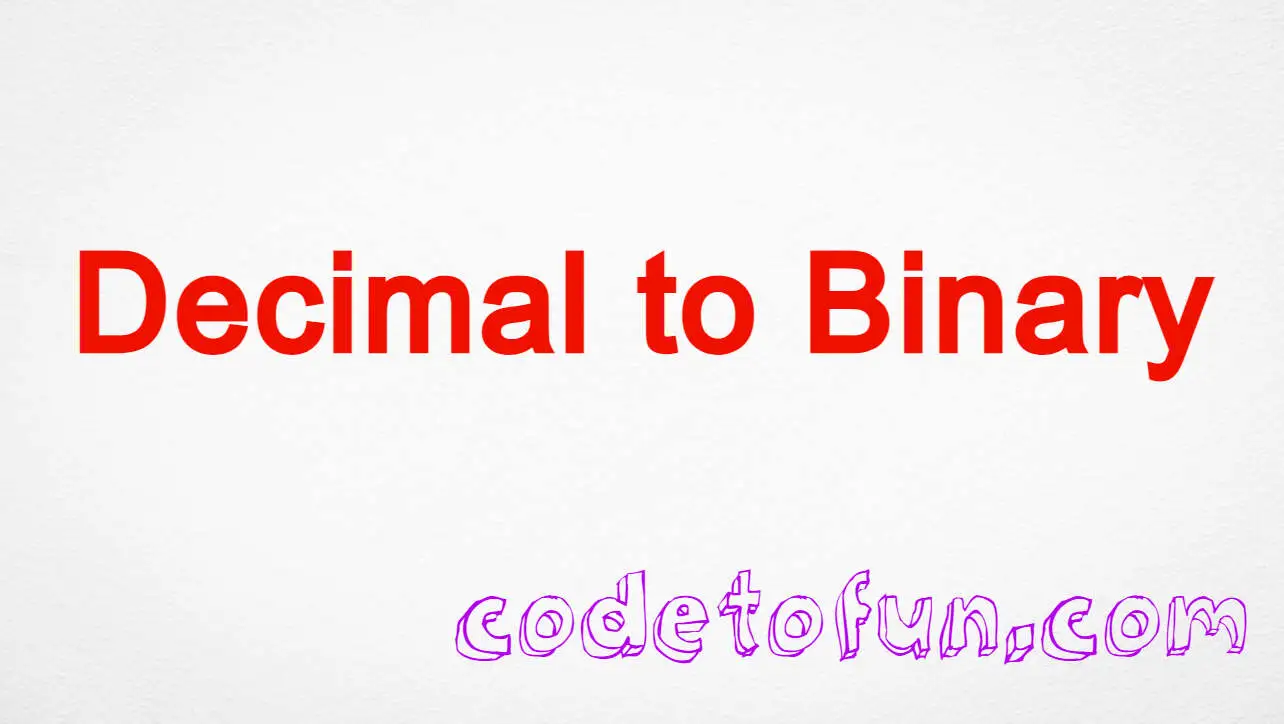
C Program to Converter a Decimal to Binary
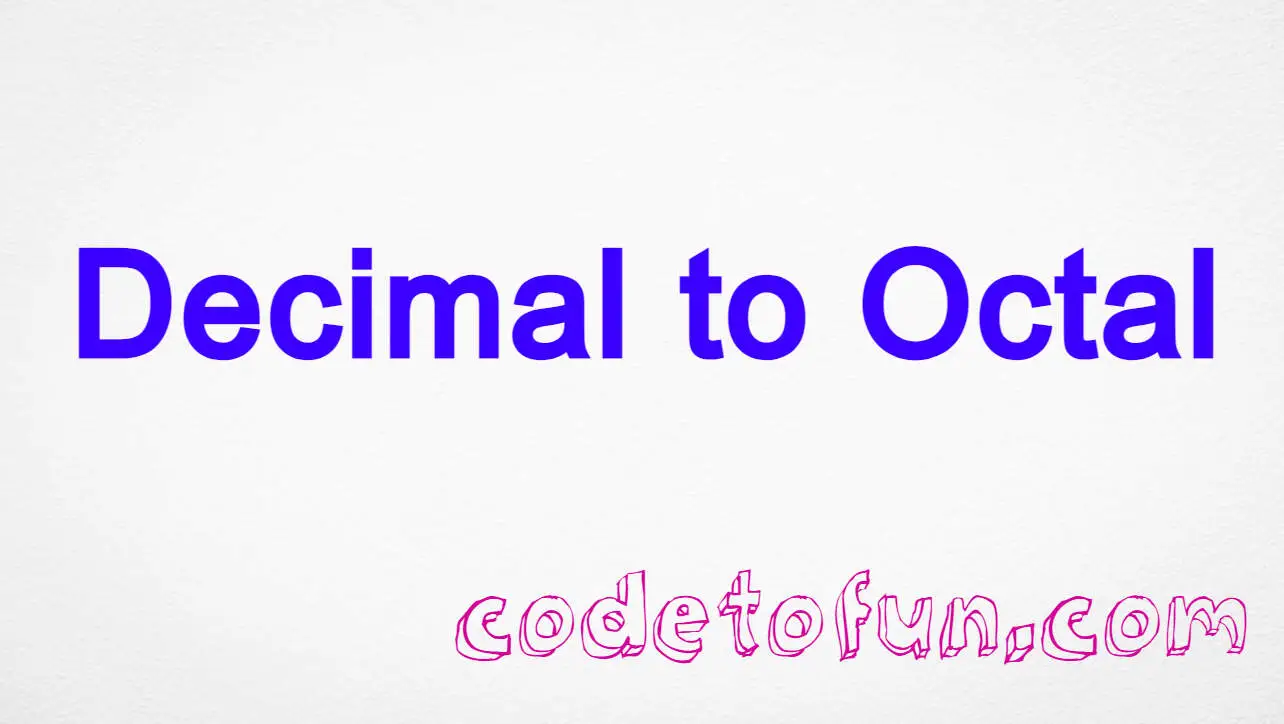
C Program to Converter a Decimal to Octal
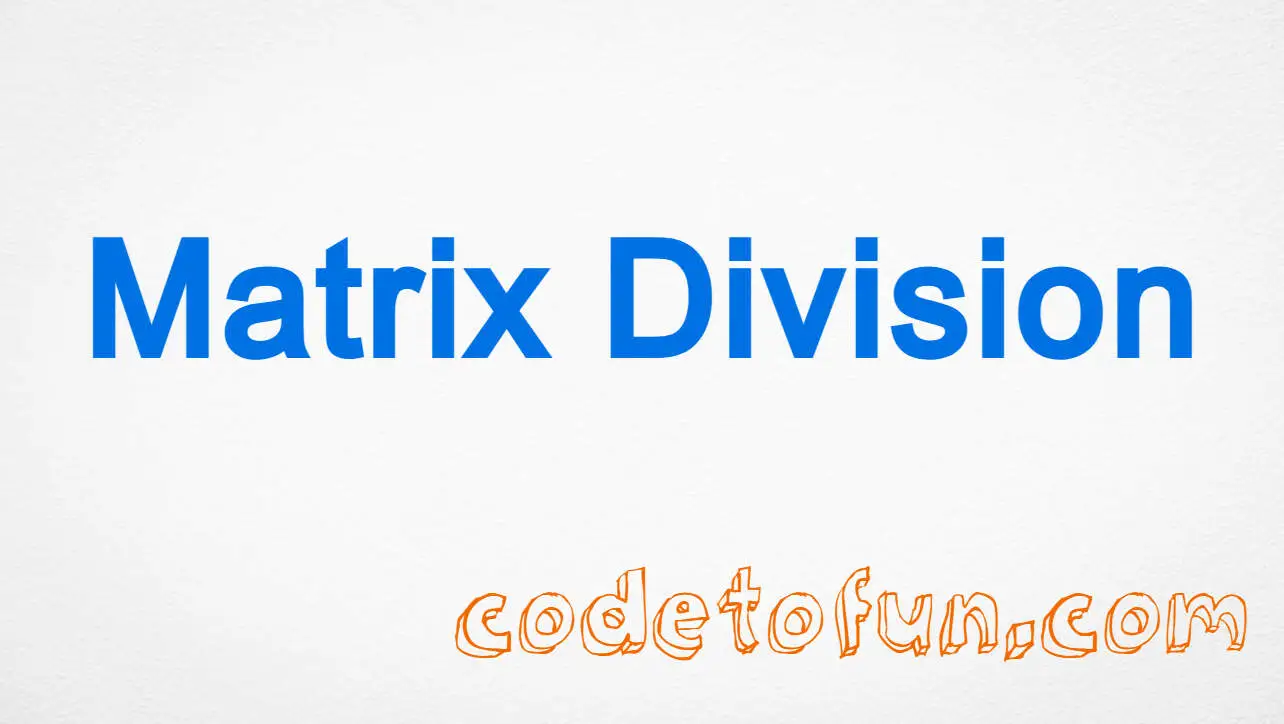
C Program to Perform Matrix Division
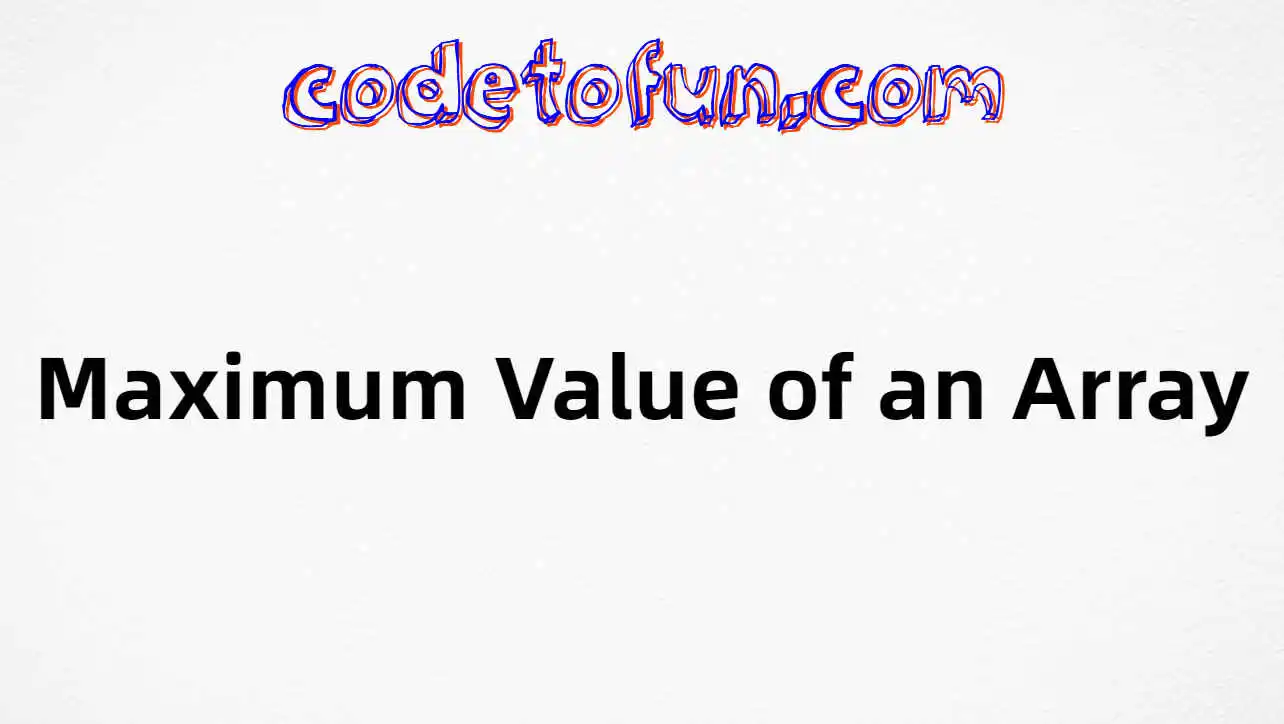
C Program to find Maximum Value of an Array
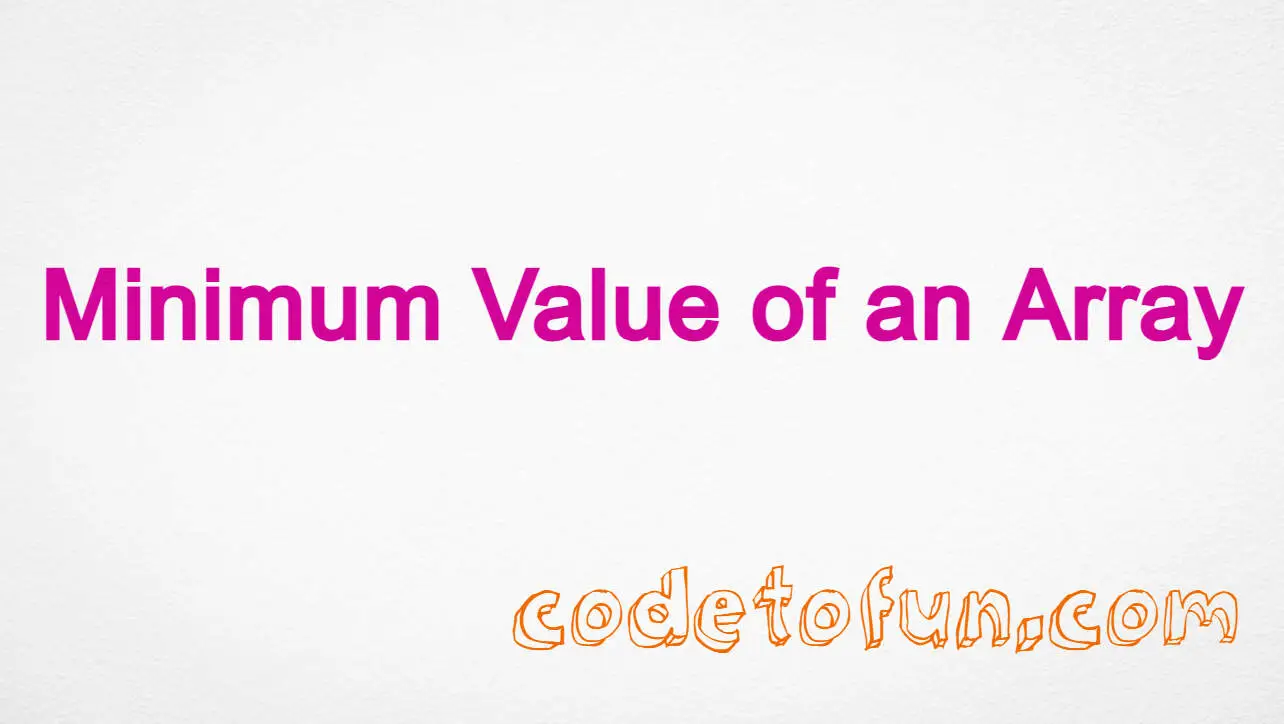
If you have any doubts regarding this article (C Program to Check Power of 3), please comment here. I will help you immediately.