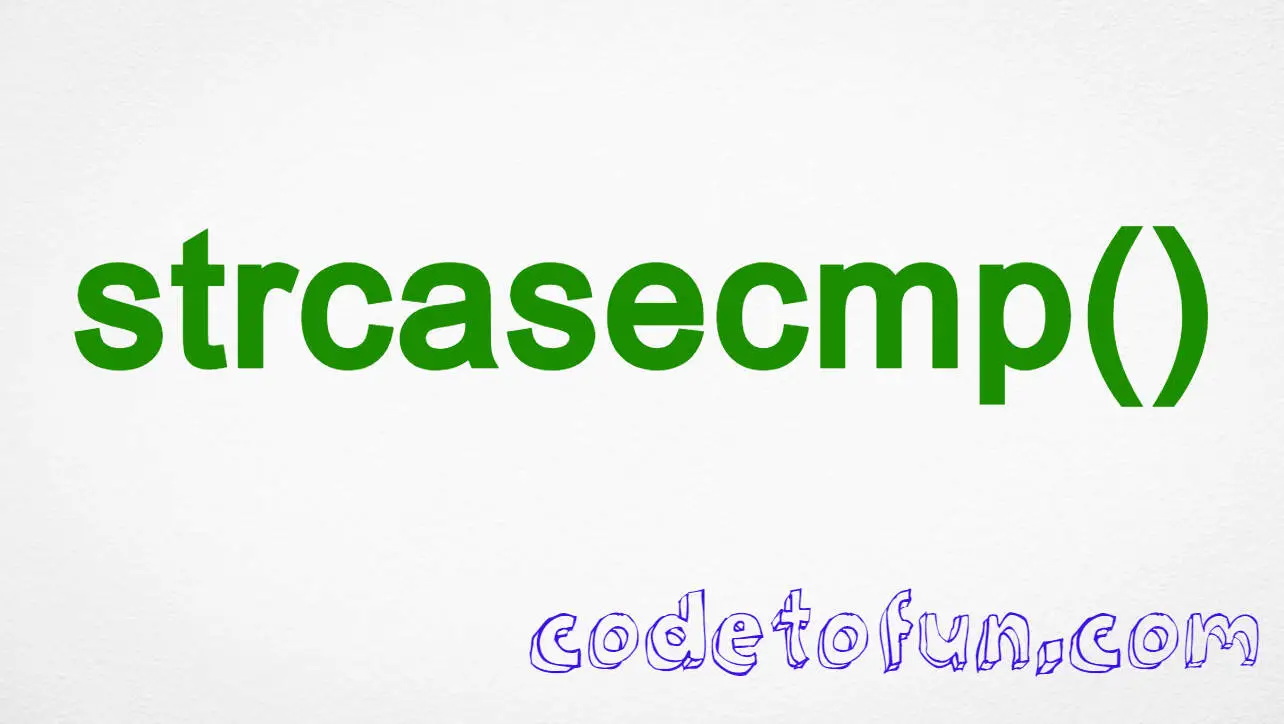
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Check Triangular Number
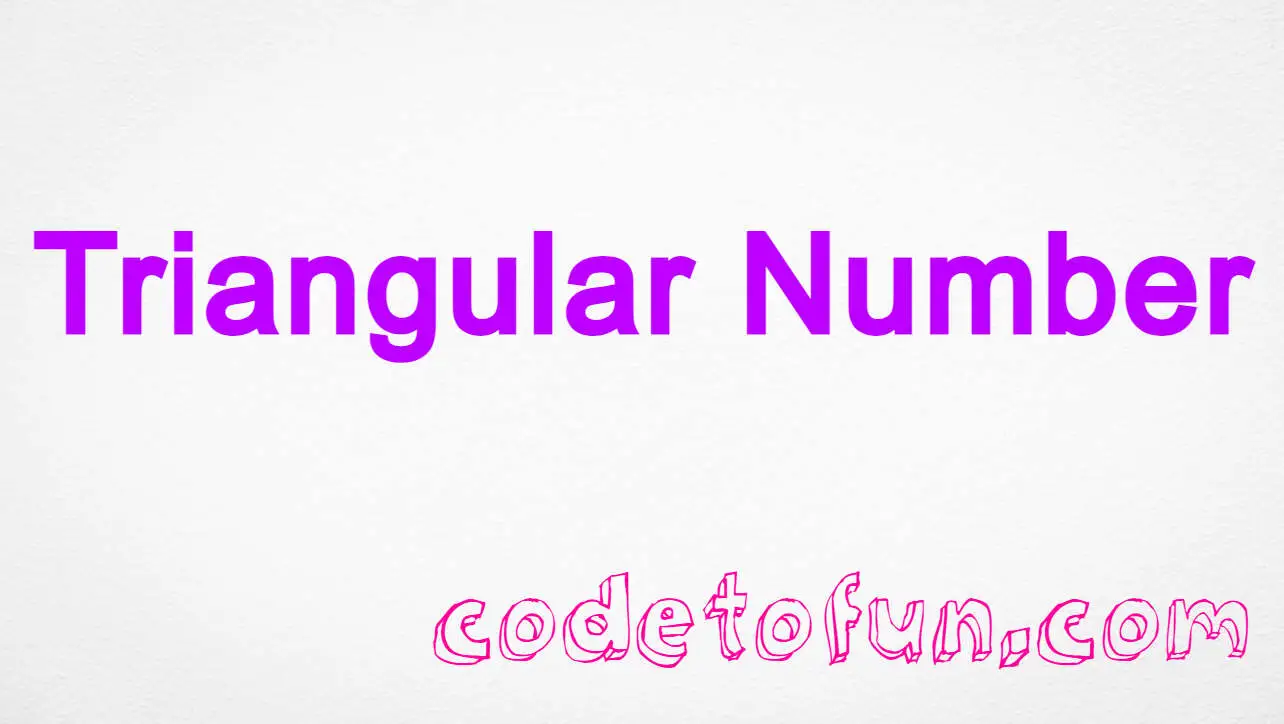
Photo Credit to CodeToFun
π Introduction
In the realm of programming, various mathematical properties of numbers often pique our interest. One such property is whether a number is a triangular number.
A triangular number or triangle number is the sum of the natural numbers up to a certain value.
In this tutorial, we'll explore a C program to check if a given number is a triangular number.
π Example
Let's delve into the C code that checks whether a given number is a triangular number.
#include <stdio.h>
#include <math.h>
// Function to check if a number is a triangular number
int isTriangularNumber(int num) {
// Formula to check triangular number
int n = (int) sqrt(2 * num);
return (n * (n + 1)) / 2 == num;
}
// Driver program
int main() {
// Replace this value with your desired number
int number = 10;
// Check if the number is a triangular number
if (isTriangularNumber(number)) {
printf("%d is a triangular number.\n", number);
} else {
printf("%d is not a triangular number.\n", number);
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the main function.
10 is a triangular number.
Compile and run the program to check if the given number is a triangular number.
π§ How the Program Works
- The program defines a function isTriangularNumber that checks if a given number is a triangular number.
- Inside the main function, replace the value of number with the desired number to check.
- The program then calls the isTriangularNumber function and prints whether the number is a triangular number or not.
π Between the Given Range
Let's take a look at the C code that checks and displays triangular numbers in the range of 1 to 50.
#include <stdio.h>
// Function to check if a number is triangular
int isTriangular(int num) {
int sum = 0, n = 1;
while (sum < num) {
sum += n;
++n;
}
return (sum == num);
}
// Driver program
int main() {
printf("Triangular Numbers in the Range 1 to 50:\n");
for (int i = 1; i <= 50; ++i) {
if (isTriangular(i)) {
printf("%d ", i);
}
}
return 0;
}
π» Testing the Program
Triangular Numbers in the Range 1 to 50: 1 3 6 10 15 21 28 36 45
Compile and run the program to see the triangular numbers in the range of 1 to 50.
π§ How the Program Works
- The program defines a function isTriangular that checks if a given number is triangular.
- Inside the function, it uses a while loop to find the triangular number by iteratively adding natural numbers.
- The main function iterates through the numbers from 1 to 50 and prints those that are triangular.
π§ Understanding the Concept of Triangular Numbers
Before delving into the code, let's take a moment to understand the concept of triangular numbers.
A triangular number is the sum of the natural numbers up to a certain value, and it can be represented by the formula Tn = n * (n + 1) / 2, where n is a non-negative integer.
For example, T3 = 1 + 2 + 3 = 6 is a triangular number.
π’ Optimizing the Program
While the provided program is effective, you can explore and implement optimizations based on the properties of triangular numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
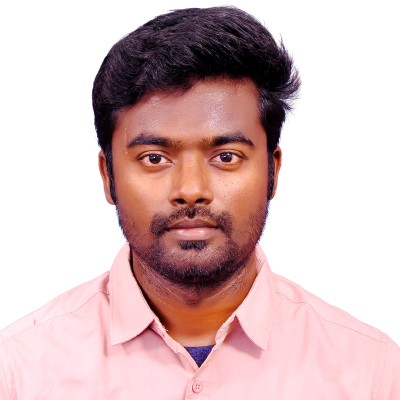
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
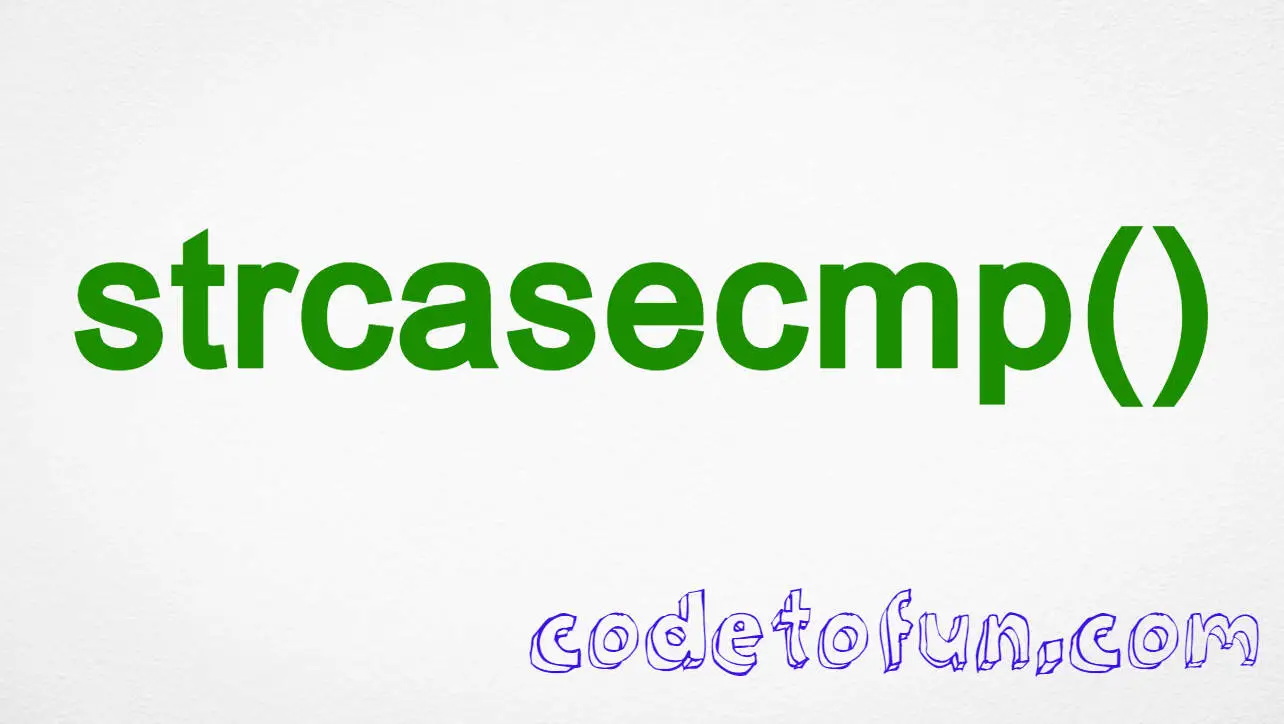
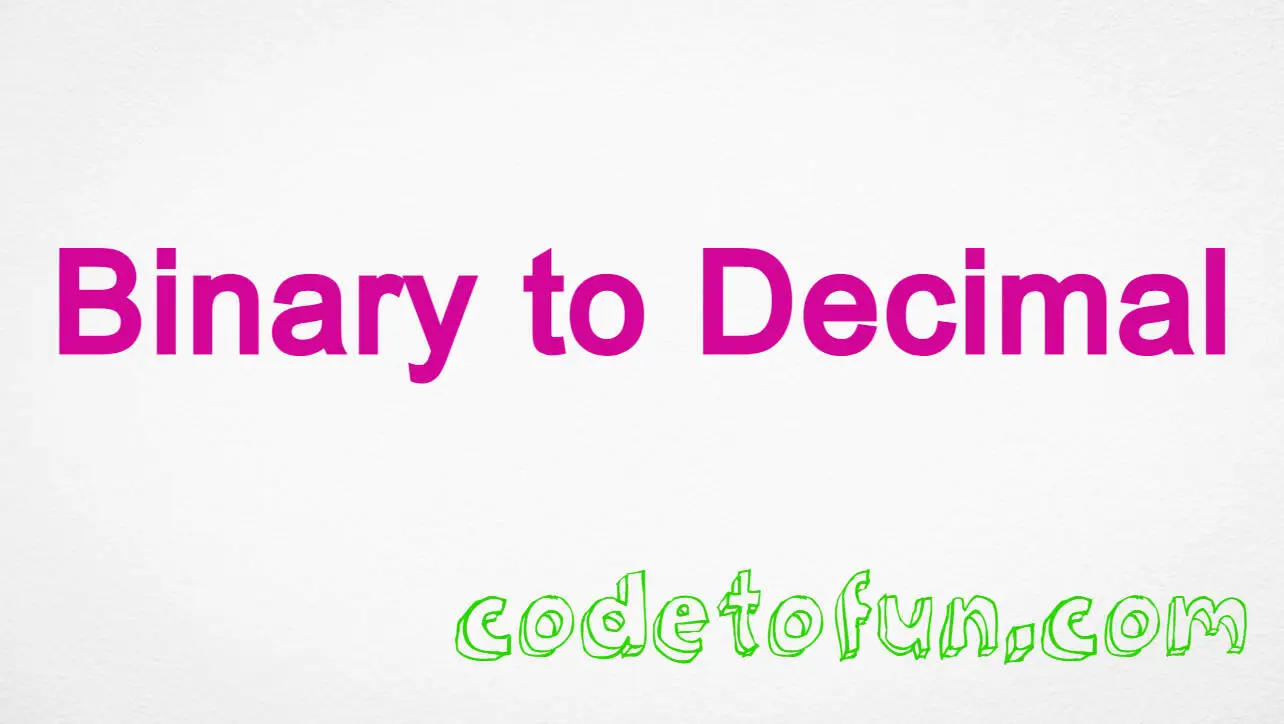
C Program to Converter a Binary to Decimal
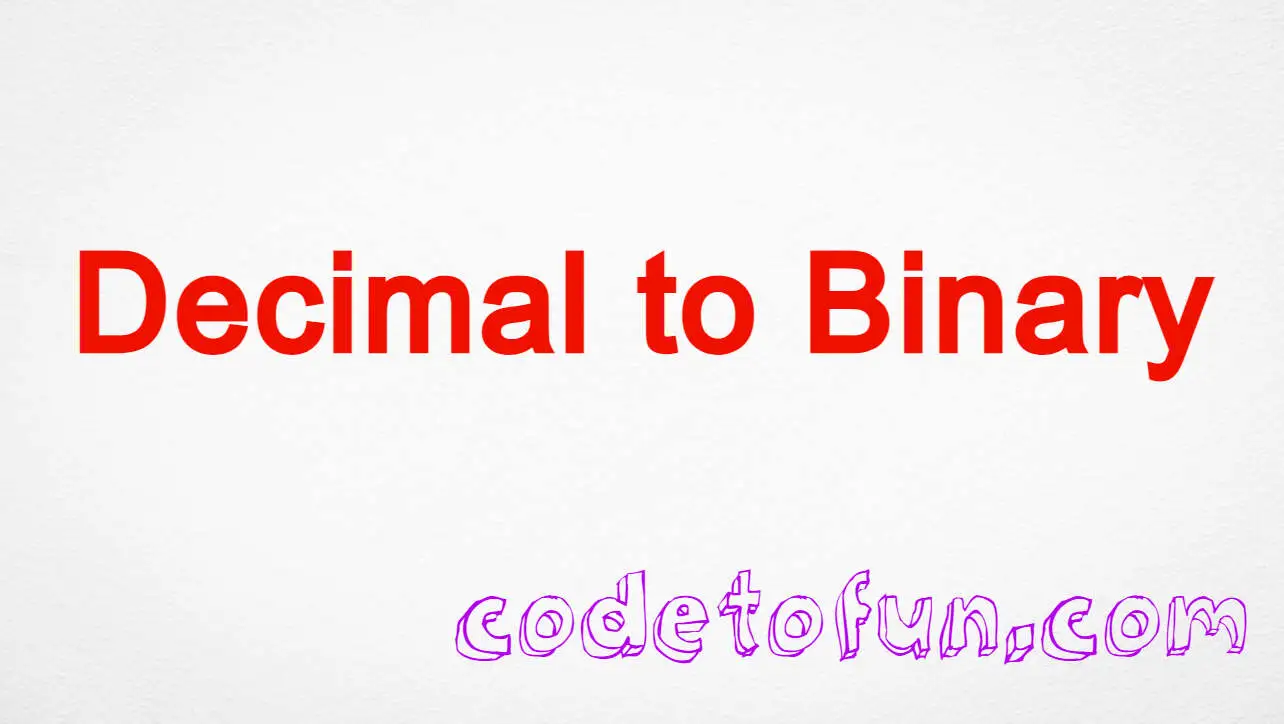
C Program to Converter a Decimal to Binary
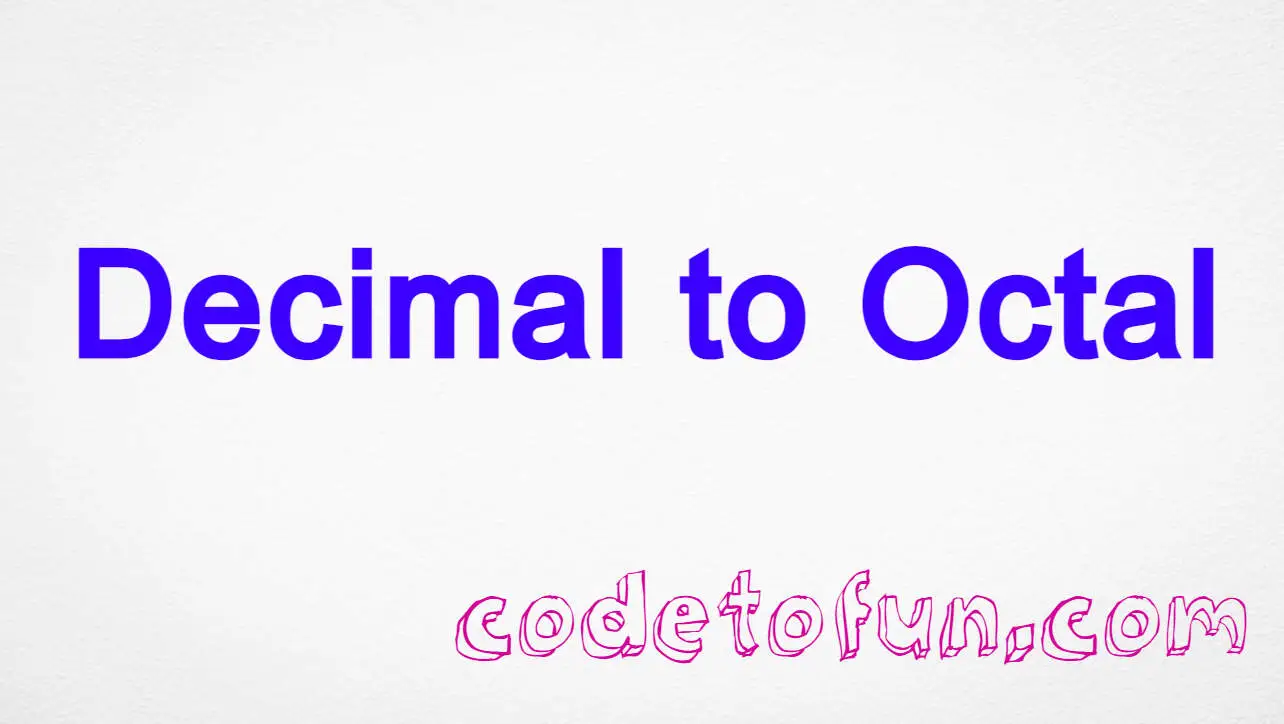
C Program to Converter a Decimal to Octal
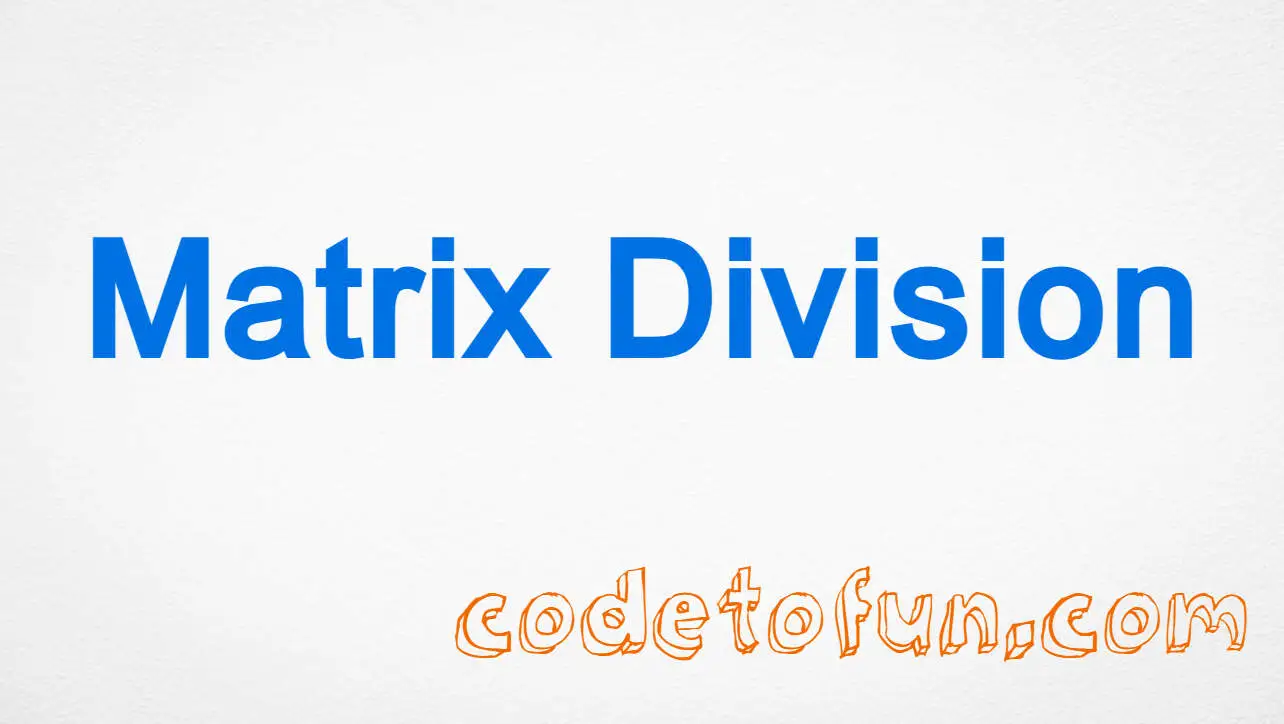
C Program to Perform Matrix Division
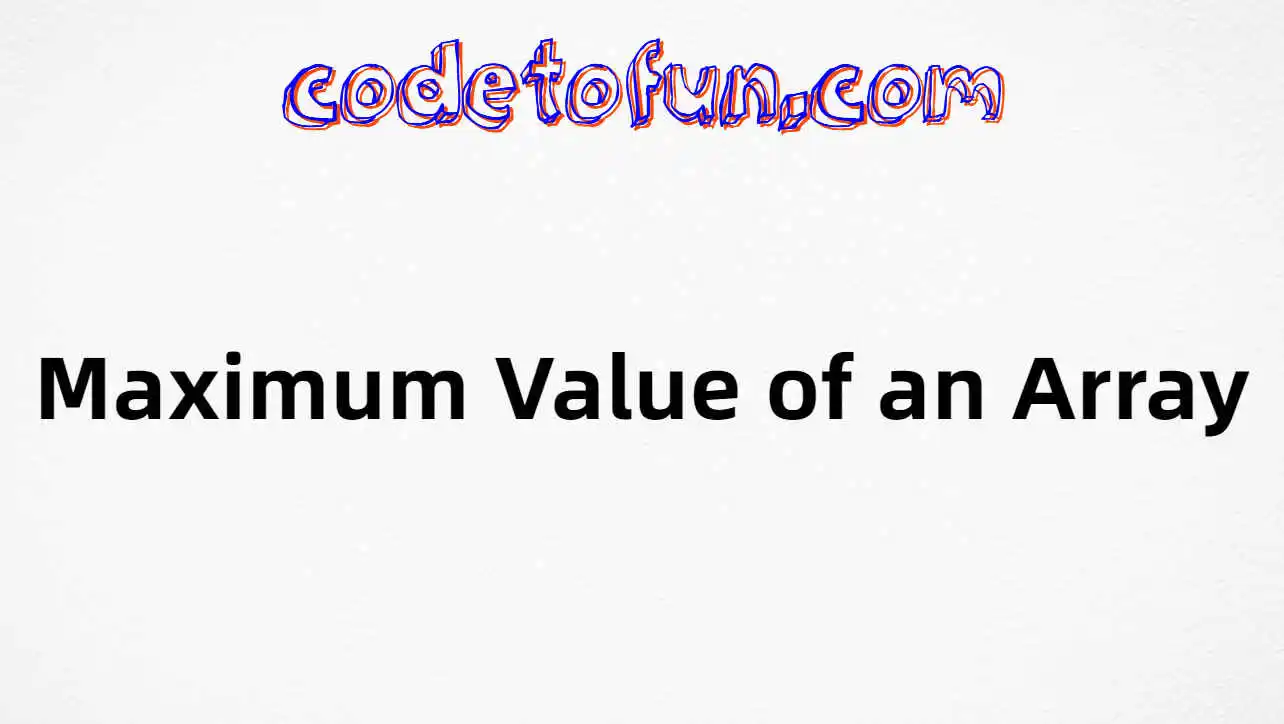
C Program to find Maximum Value of an Array
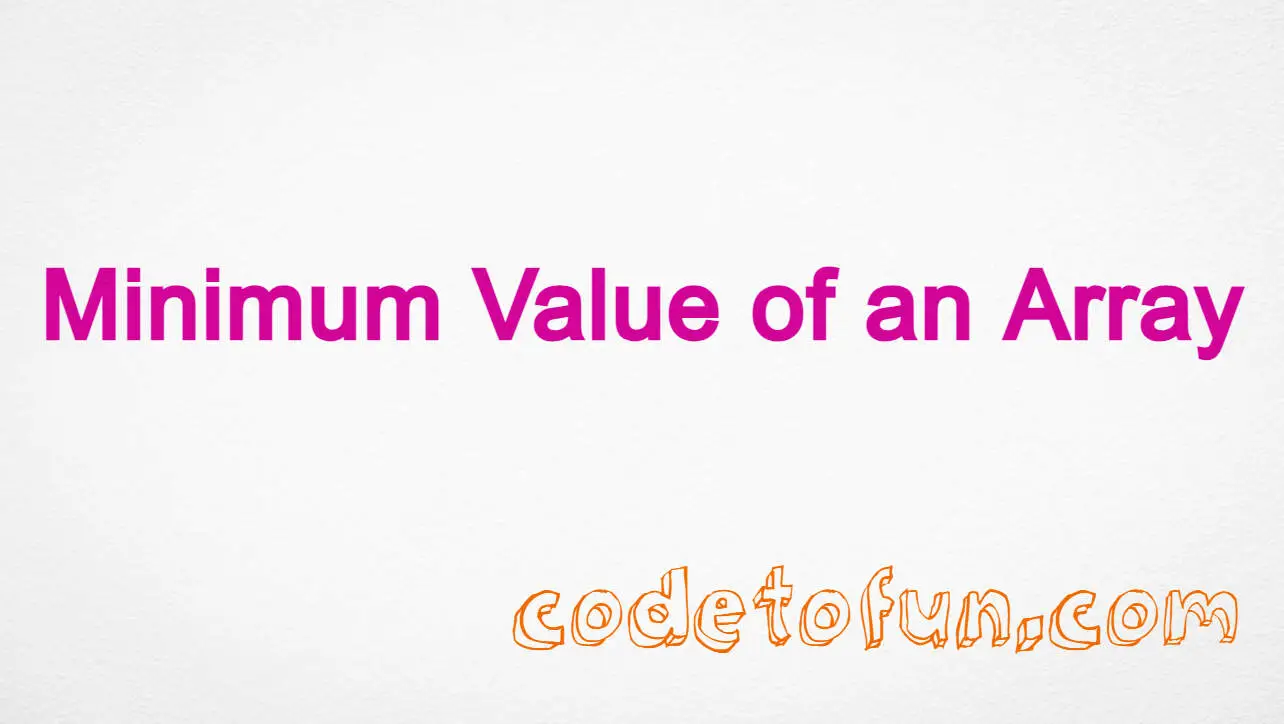
If you have any doubts regarding this article (C Program to Check Triangular Number), please comment here. I will help you immediately.