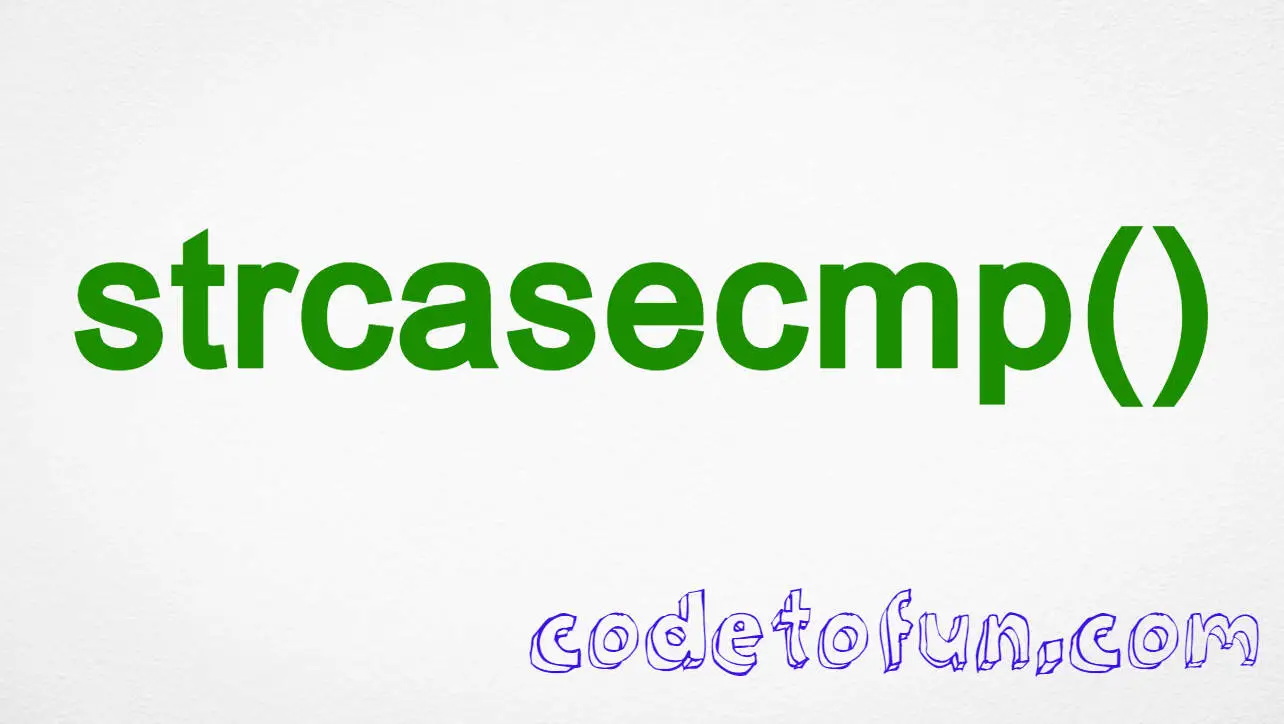
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Perform Matrix Addition
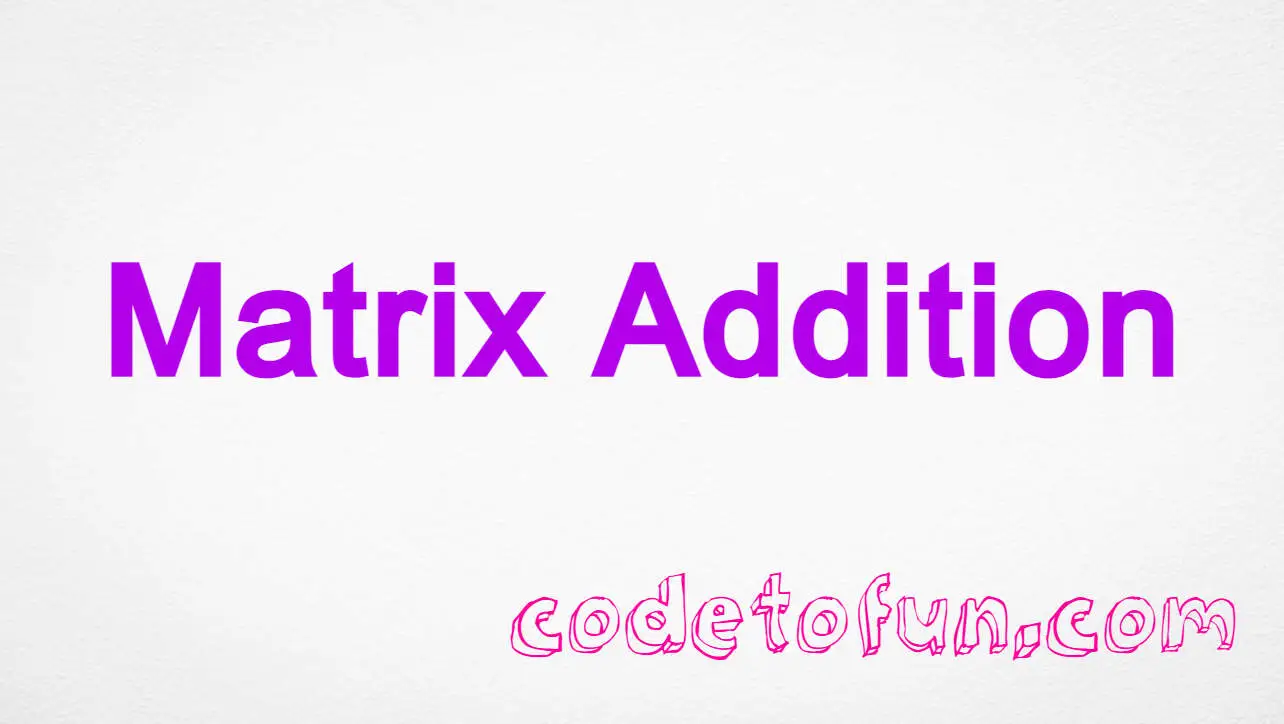
Photo Credit to CodeToFun
π Introduction
Matrix addition is a fundamental operation in linear algebra and computer science. It involves adding corresponding elements of two matrices to create a new matrix.
This operation is essential in various fields, including computer graphics, scientific computing, and data analysis.
In this tutorial, we'll explore a simple yet powerful C program that performs matrix addition.
Understanding matrix operations and implementing them in C can provide a solid foundation for more complex computations.
π Example
Let's delve into the C code that achieves matrix addition.
#include <stdio.h>
// Function to perform matrix addition
void addMatrices(int mat1[3][3], int mat2[3][3], int result[3][3]) {
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
result[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
// Function to display a matrix
void displayMatrix(int matrix[3][3]) {
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
printf("%d ", matrix[i][j]);
}
printf("\n");
}
}
// Driver program
int main() {
// Sample matrices for testing
int matrix1[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int matrix2[3][3] = {
{9, 8, 7},
{6, 5, 4},
{3, 2, 1}
};
int resultMatrix[3][3];
// Call the function to perform matrix addition
addMatrices(matrix1, matrix2, resultMatrix);
// Displaying matrices and result
printf("Matrix 1:\n");
displayMatrix(matrix1);
printf("\nMatrix 2:\n");
displayMatrix(matrix2);
printf("\nResultant Matrix:\n");
displayMatrix(resultMatrix);
return 0;
}
π» Testing the Program
Feel free to replace the sample matrices with your own 3x3 matrices in the main function to test the program with different input.
Matrix 1: 1 2 3 4 5 6 7 8 9 Matrix 2: 9 8 7 6 5 4 3 2 1 Resultant Matrix: 10 10 10 10 10 10 10 10 10
Compile and run the program to see the result of the matrix addition.
π§ How the Program Works
- The program defines a function addMatrices to add two matrices and a function displayMatrix to display a matrix.
- In the main function, sample matrices matrix1 and matrix2 are provided for testing.
- The addMatrices function is called to perform the addition, and the result is stored in resultMatrix.
- The matrices are displayed using the displayMatrix function.
π§ Understanding the Concept of Matrix Addition
Matrix addition involves adding corresponding elements of two matrices to create a new matrix.
Given two matrices A and B, the sum C is calculated as follows:
Cij = Aij + Bij.
where Cij is the element at the i-th row and j-th column of matrix C, and Aij and Bij are the corresponding elements of matrices A and B.
Matrix addition is only defined for matrices of the same size; that is, they must have the same number of rows and columns.
π’ Optimizing the Program
While the provided program is effective, there are optimizations that can be applied for larger matrices. Consider exploring techniques such as parallelization for improved performance.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
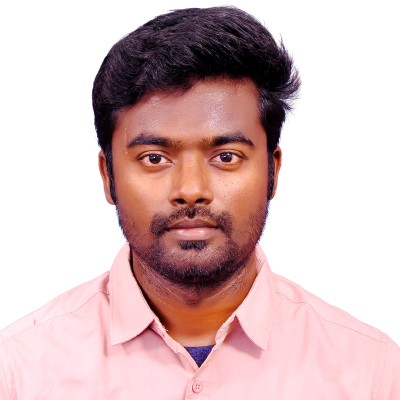
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
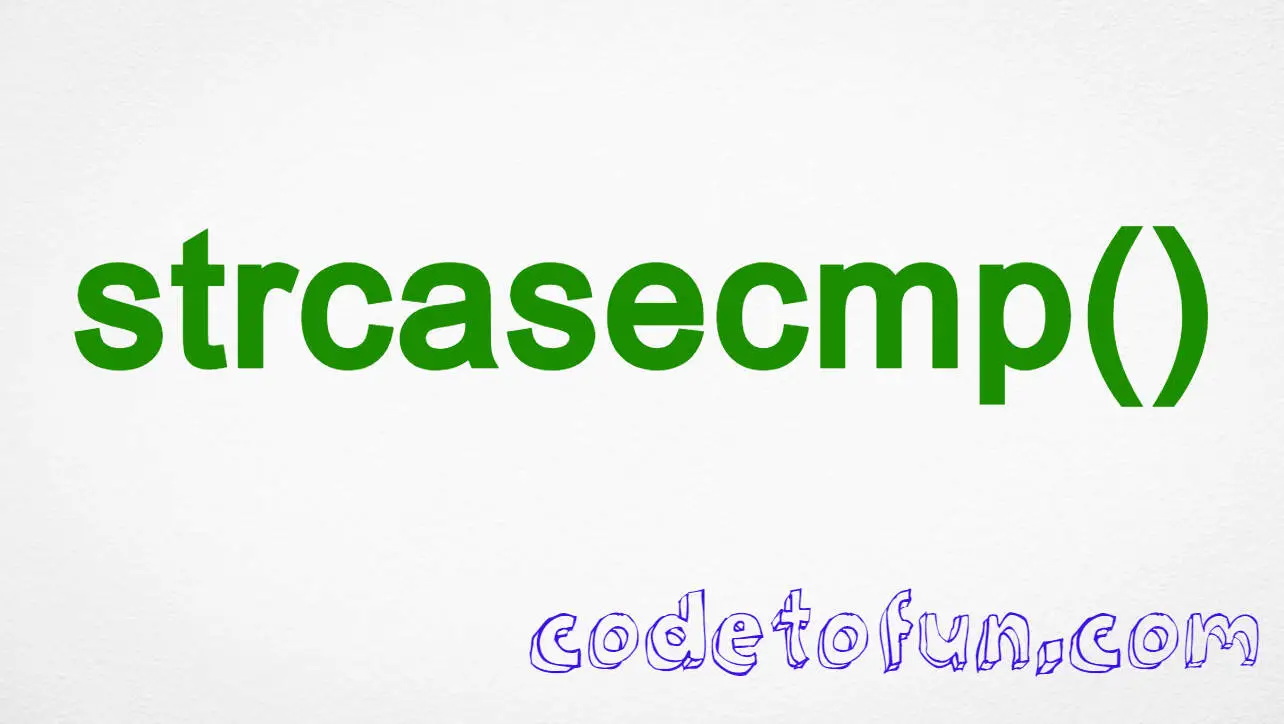
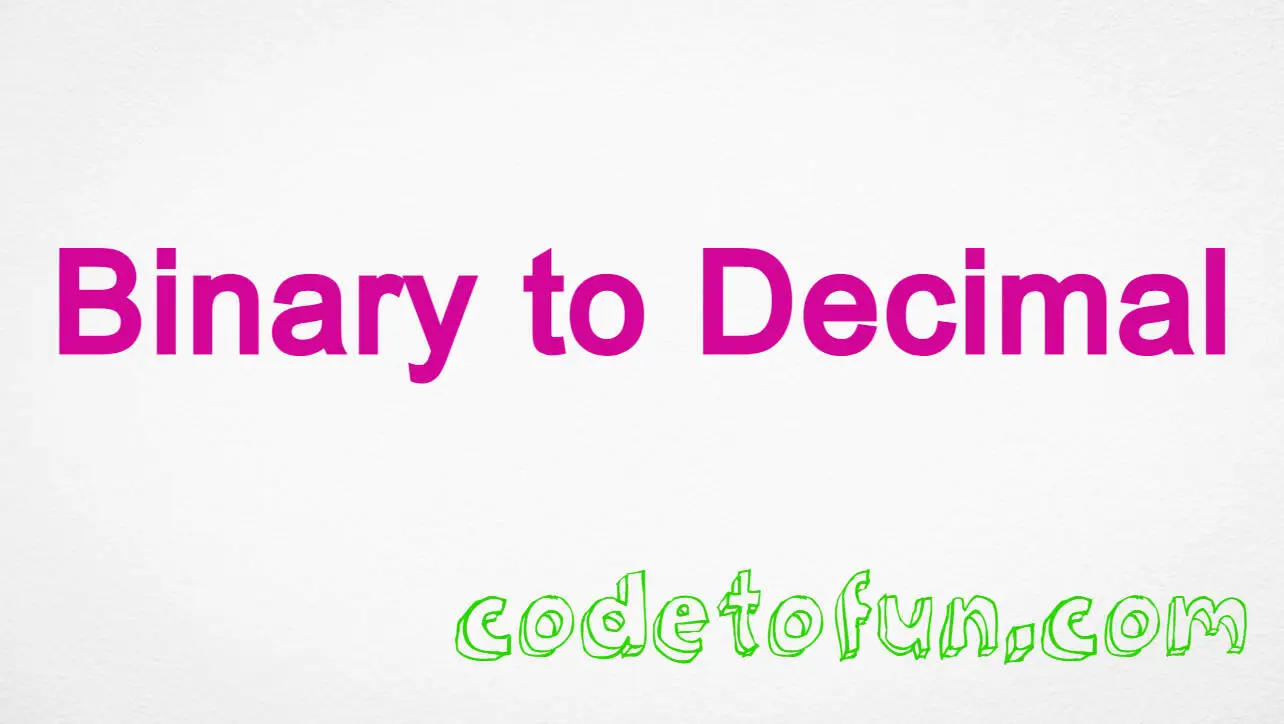
C Program to Converter a Binary to Decimal
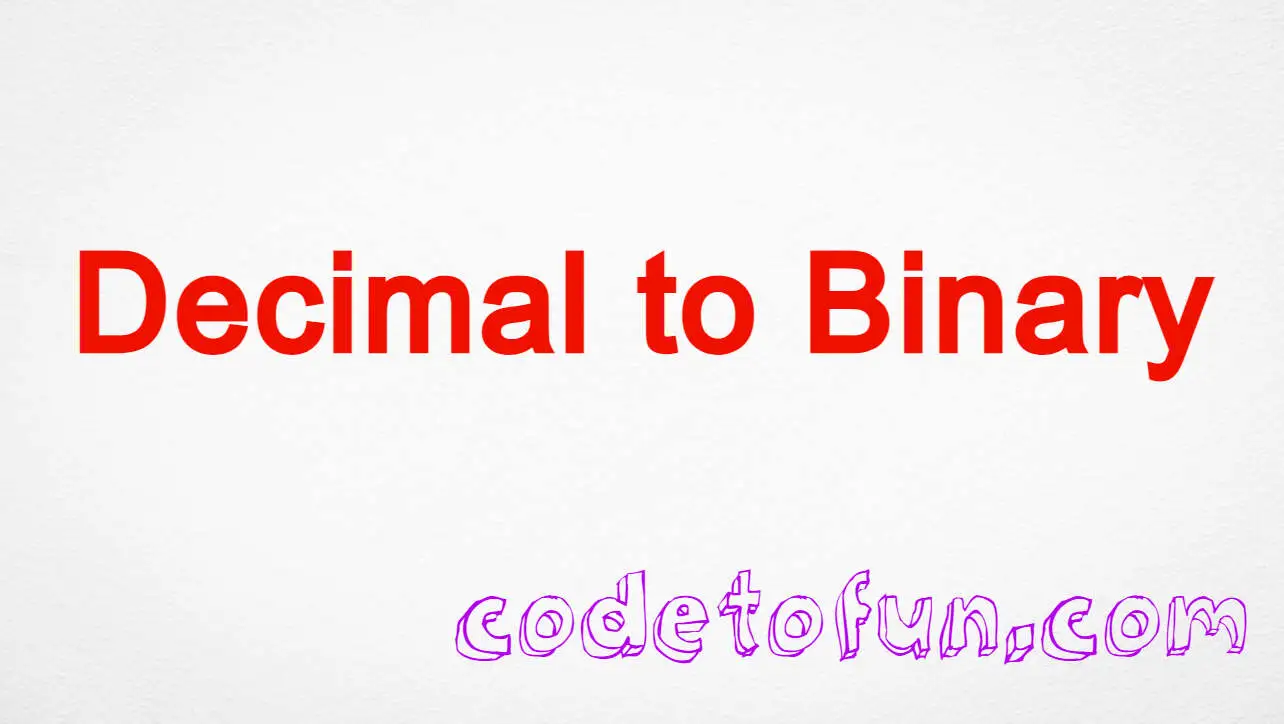
C Program to Converter a Decimal to Binary
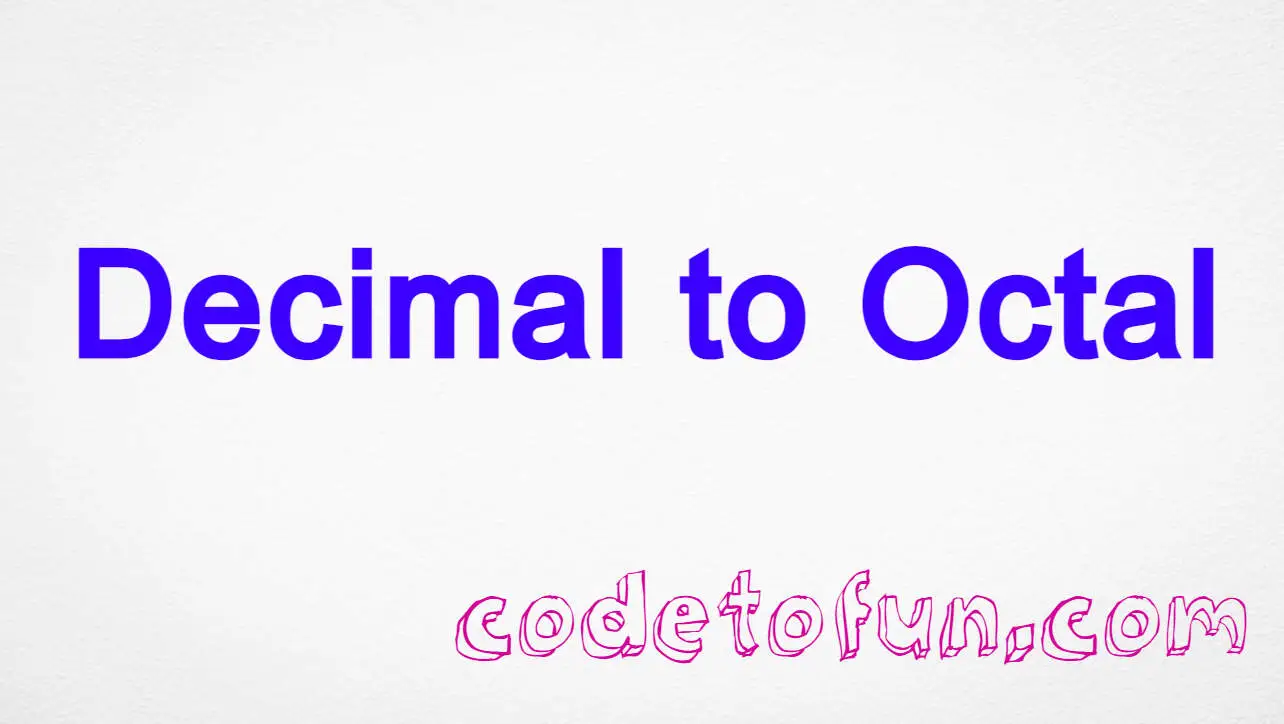
C Program to Converter a Decimal to Octal
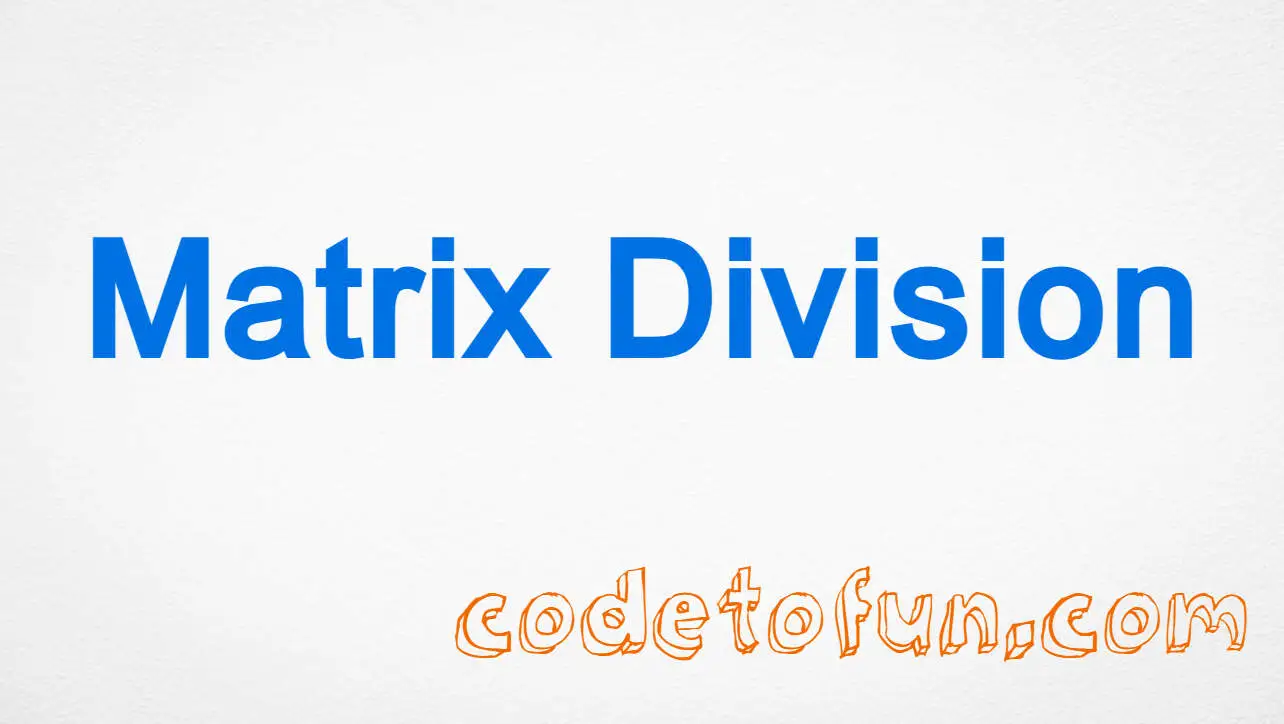
C Program to Perform Matrix Division
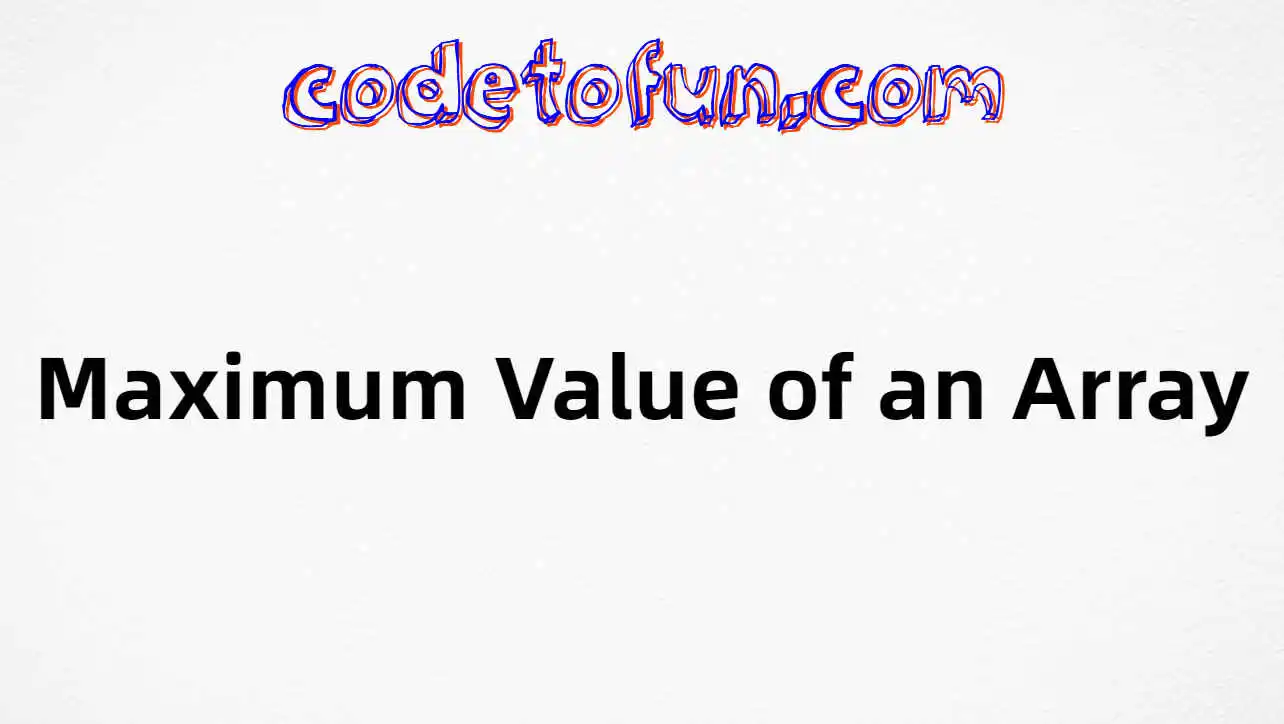
C Program to find Maximum Value of an Array
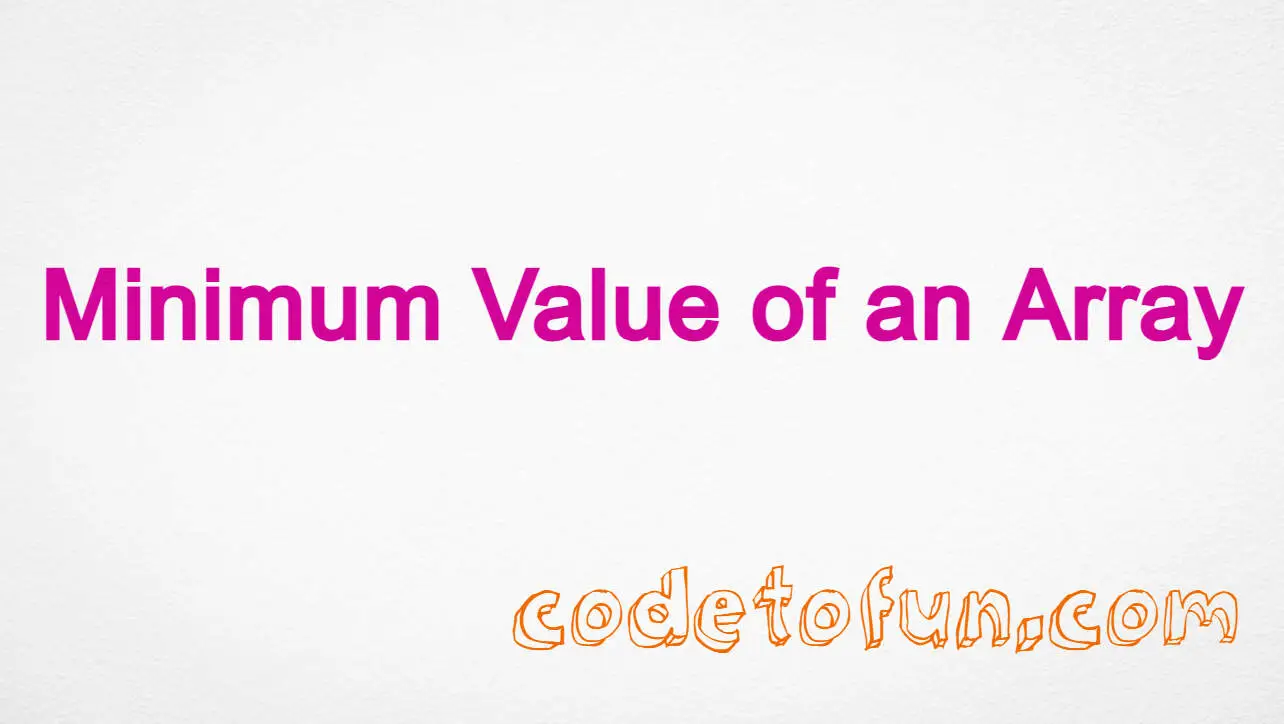
If you have any doubts regarding this article (C Program to Perform Matrix Addition), please comment here. I will help you immediately.