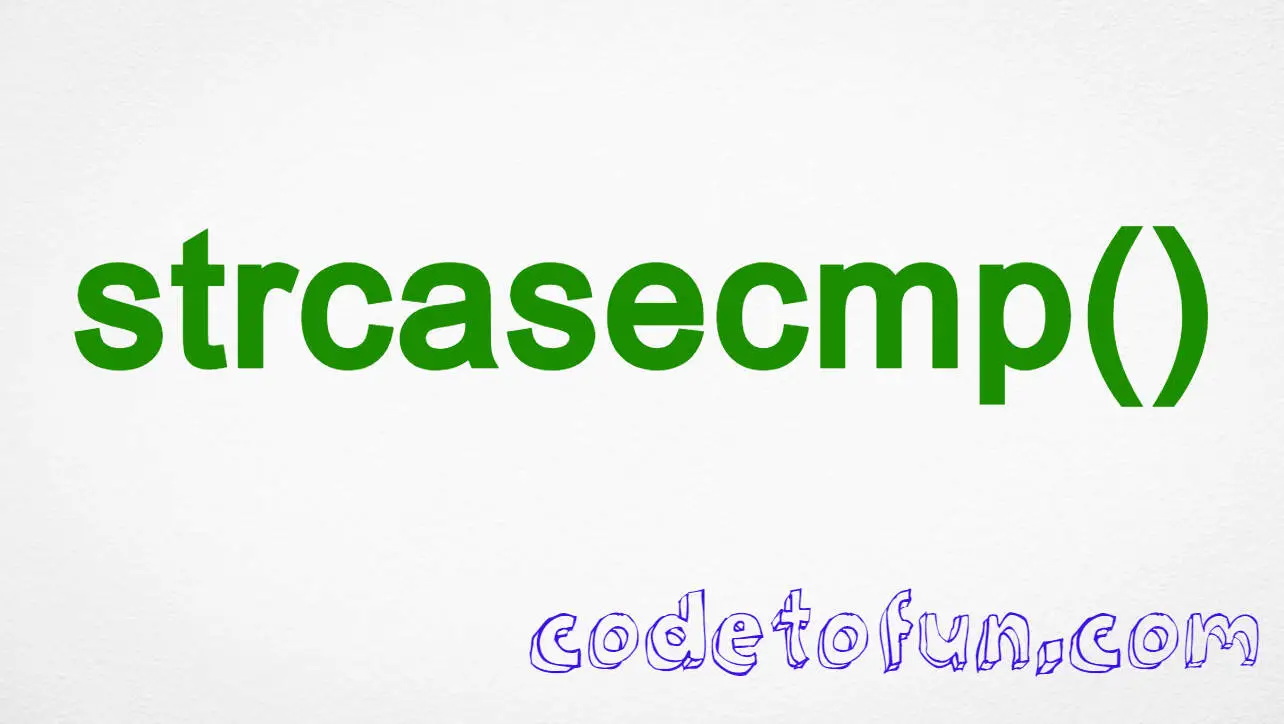
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to find Minimum Value of an Array
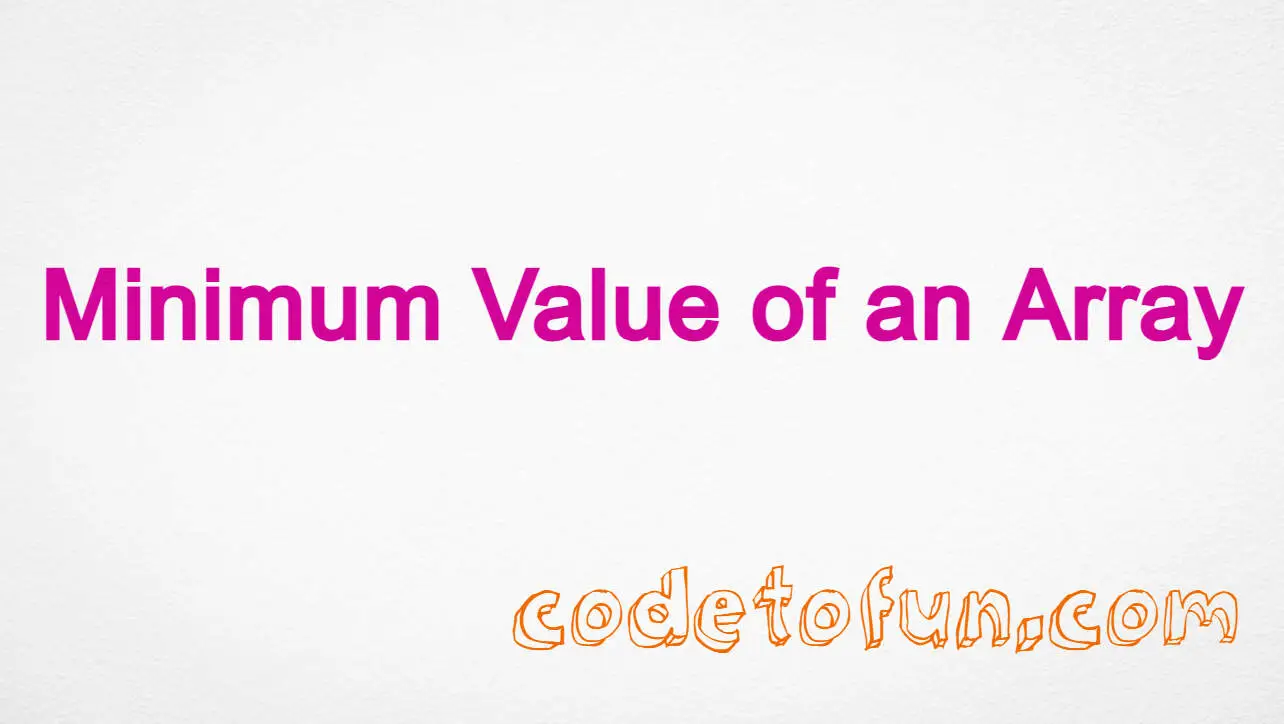
Photo Credit to CodeToFun
π Introduction
In the world of programming, manipulating arrays is a fundamental skill. One common task is finding the minimum value within an array.
This involves iterating through the array elements and identifying the smallest value present.
In this tutorial, we will walk through a C program that efficiently finds the minimum value of an array..
π Example
Let's dive into the C code to achieve this functionality.
#include <stdio.h>
// Function to find the minimum value in an array
int findMinValue(int arr[], int size) {
// Assume the first element is the minimum
int minValue = arr[0];
// Iterate through the array to find the minimum value
for (int i = 1; i < size; ++i) {
if (arr[i] < minValue) {
minValue = arr[i];
}
}
return minValue;
}
// Driver program
int main() {
// Replace these values with your array
int array[] = {12, 5, 7, 3, 2, 8, 10};
// Calculate the size of the array
int size = sizeof(array) / sizeof(array[0]);
// Call the function to find the minimum value
int minValue = findMinValue(array, size);
// Print the result
printf("The minimum value in the array is: %d\n", minValue);
return 0;
}
π» Testing the Program
To test the program with a different array, simply replace the values in the array declaration in the main function.
The minimum value in the array is: 2
Compile and run the program to see the minimum value of the array.
π§ How the Program Works
- The program defines a function findMinValue that takes an array and its size as input and returns the minimum value.
- Inside the function, it assumes the first element of the array is the minimum value.
- It then iterates through the array, updating the minimum value whenever a smaller element is encountered.
- The driver program initializes an array and calculates its size before calling the function and printing the result.
π§ Understanding the Concept of Minimum Value of an Array
Before diving into the code, let's understand the concept of finding the minimum value in an array.
The minimum value is the smallest element present in the array. The algorithm iterates through the array elements, updating the minimum value whenever a smaller element is encountered.
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing optimizations, such as using additional data structures or algorithms, depending on the specific requirements and constraints of your application.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
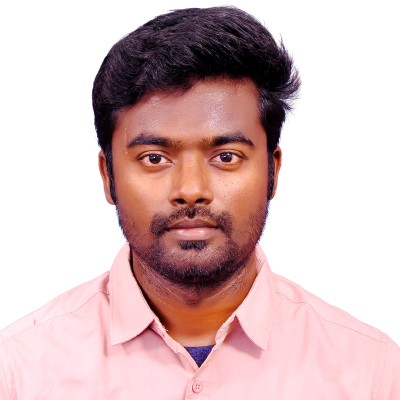
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
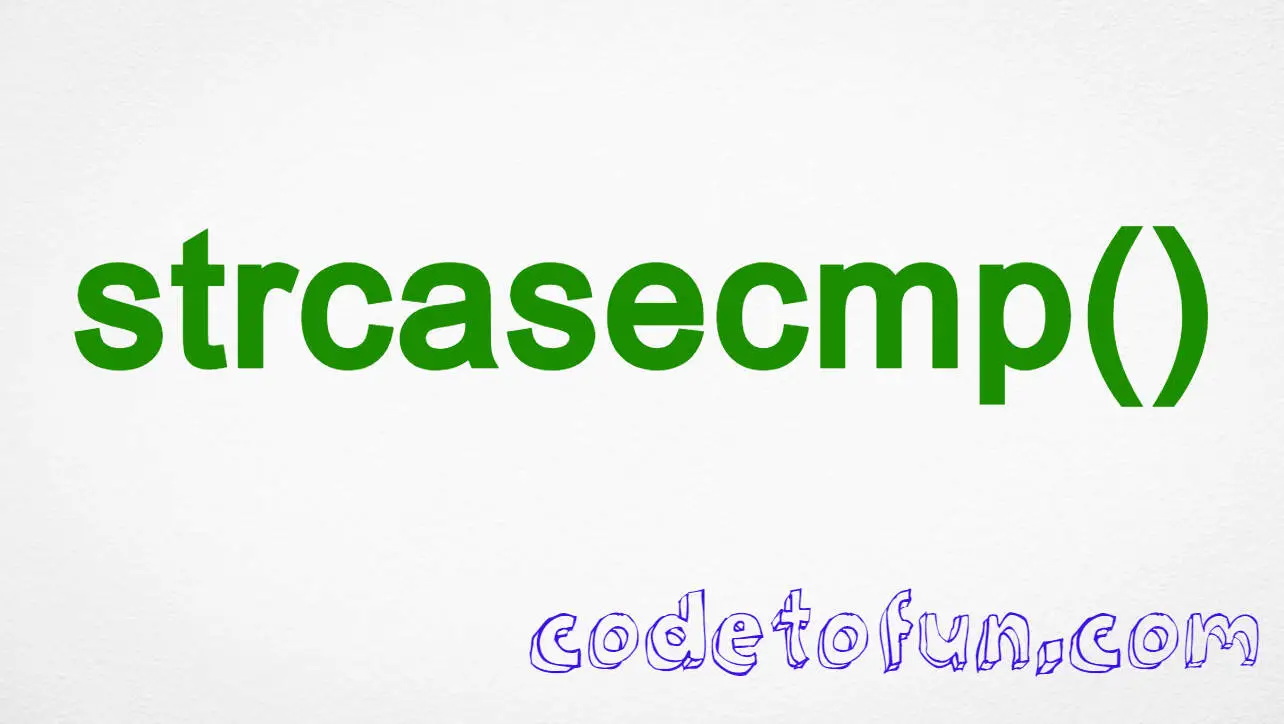
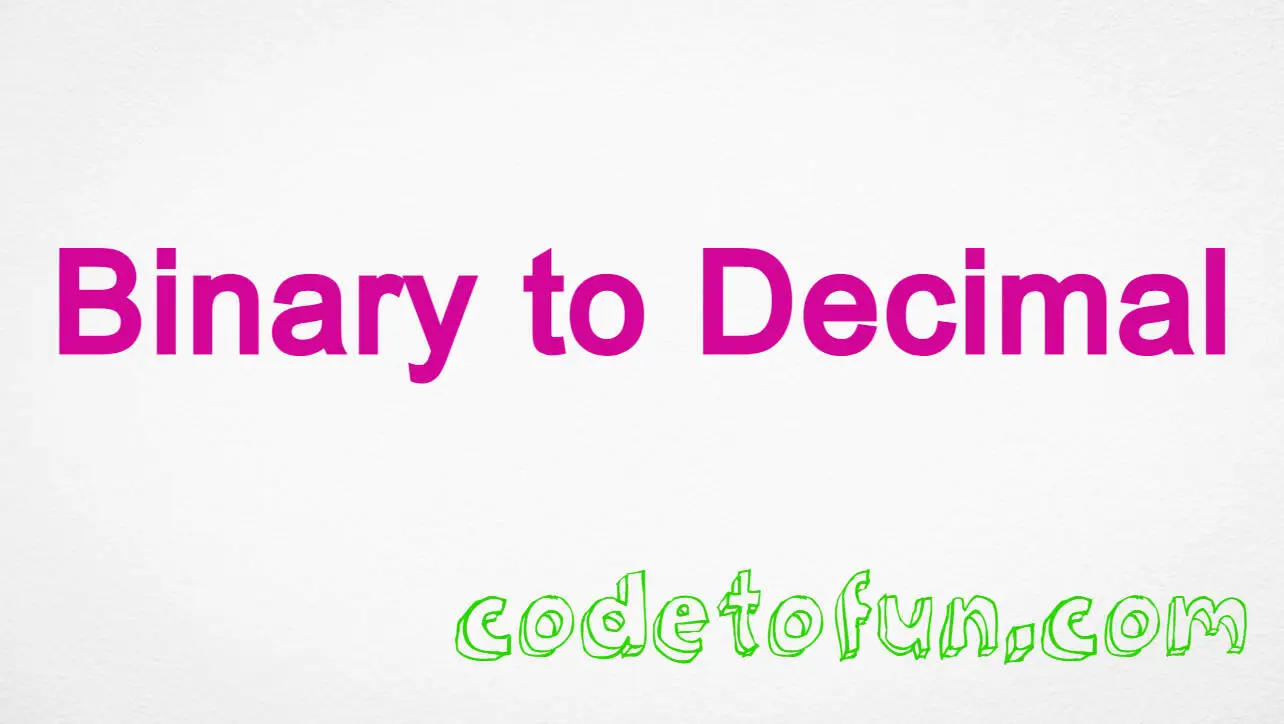
C Program to Converter a Binary to Decimal
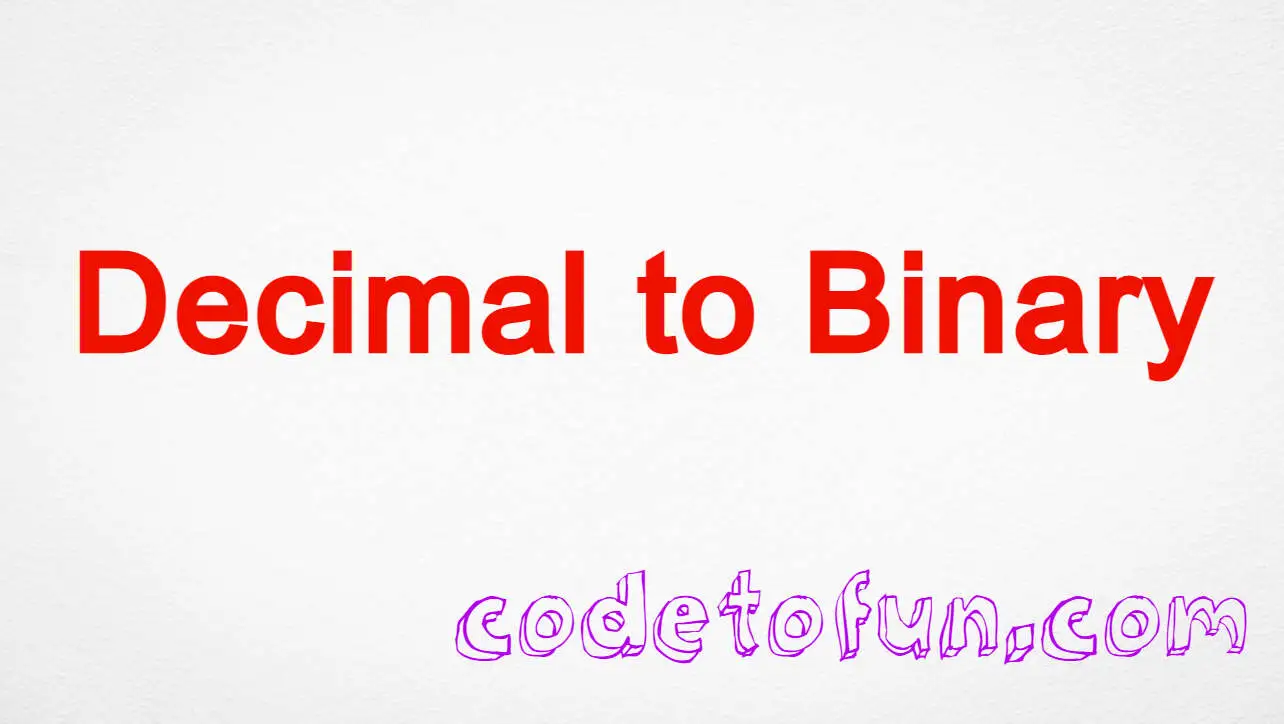
C Program to Converter a Decimal to Binary
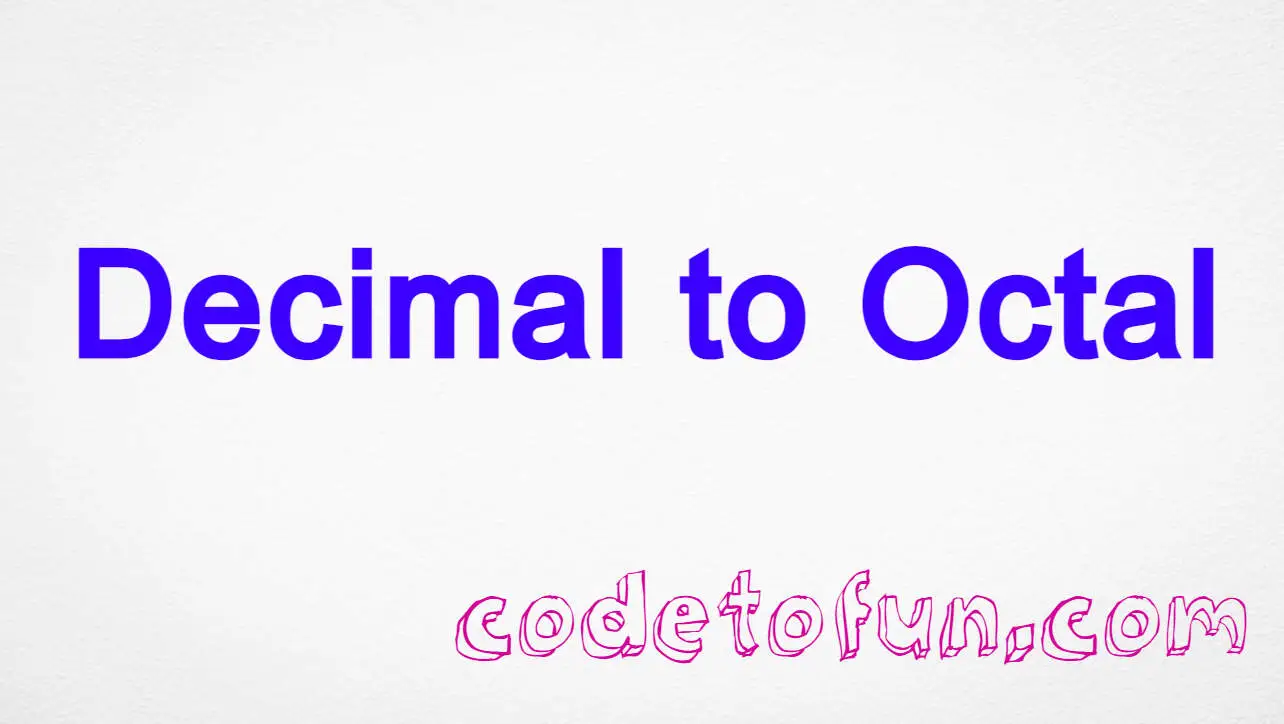
C Program to Converter a Decimal to Octal
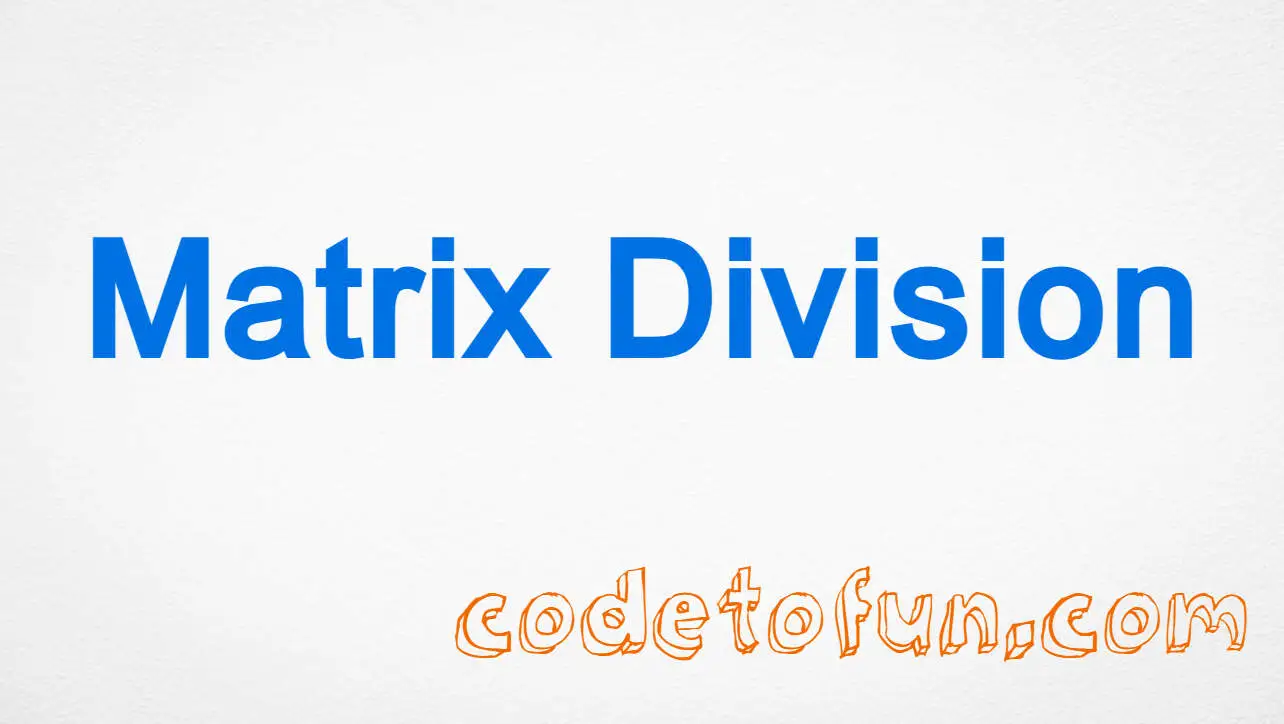
C Program to Perform Matrix Division
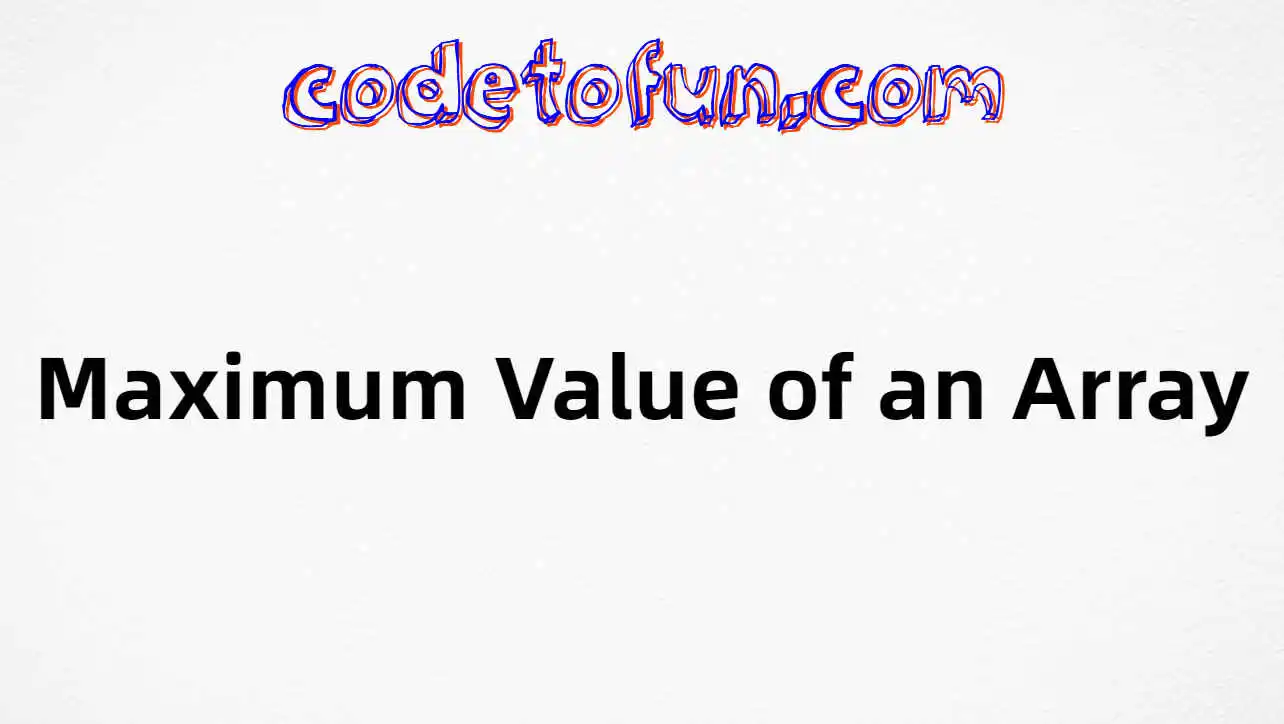
C Program to find Maximum Value of an Array
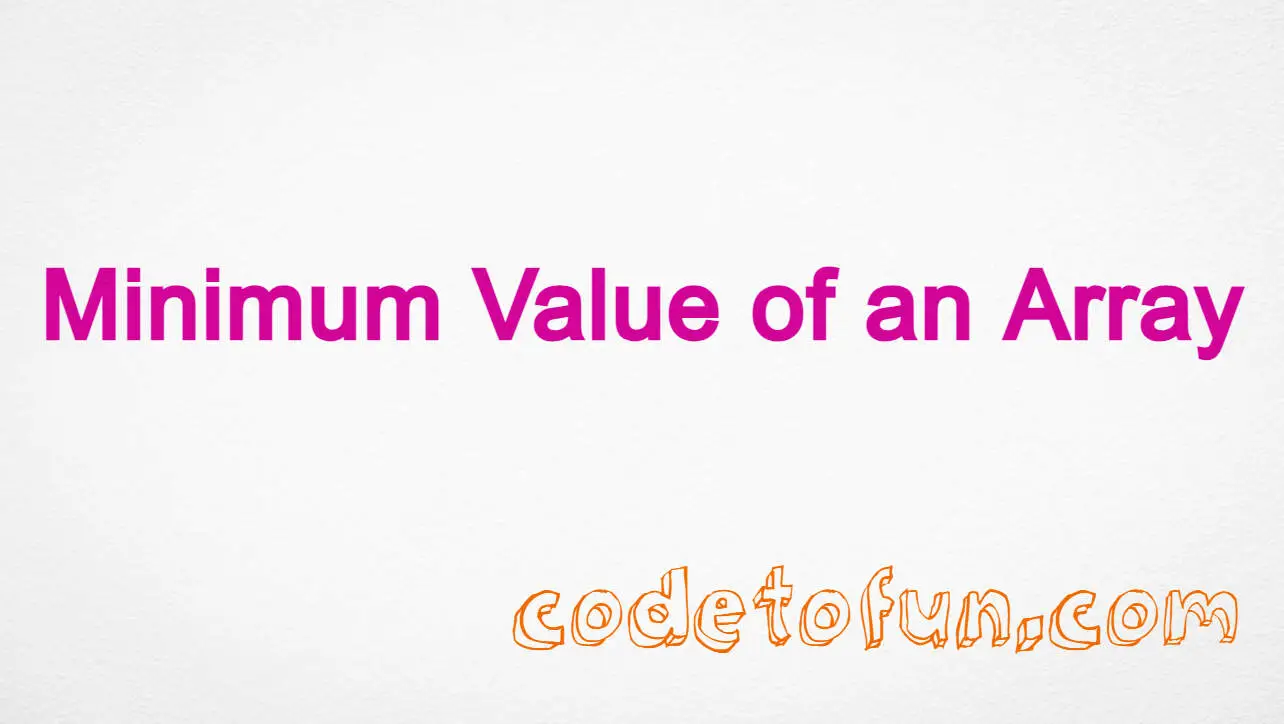
If you have any doubts regarding this article (C Program to find Minimum Value of an Array), please comment here. I will help you immediately.