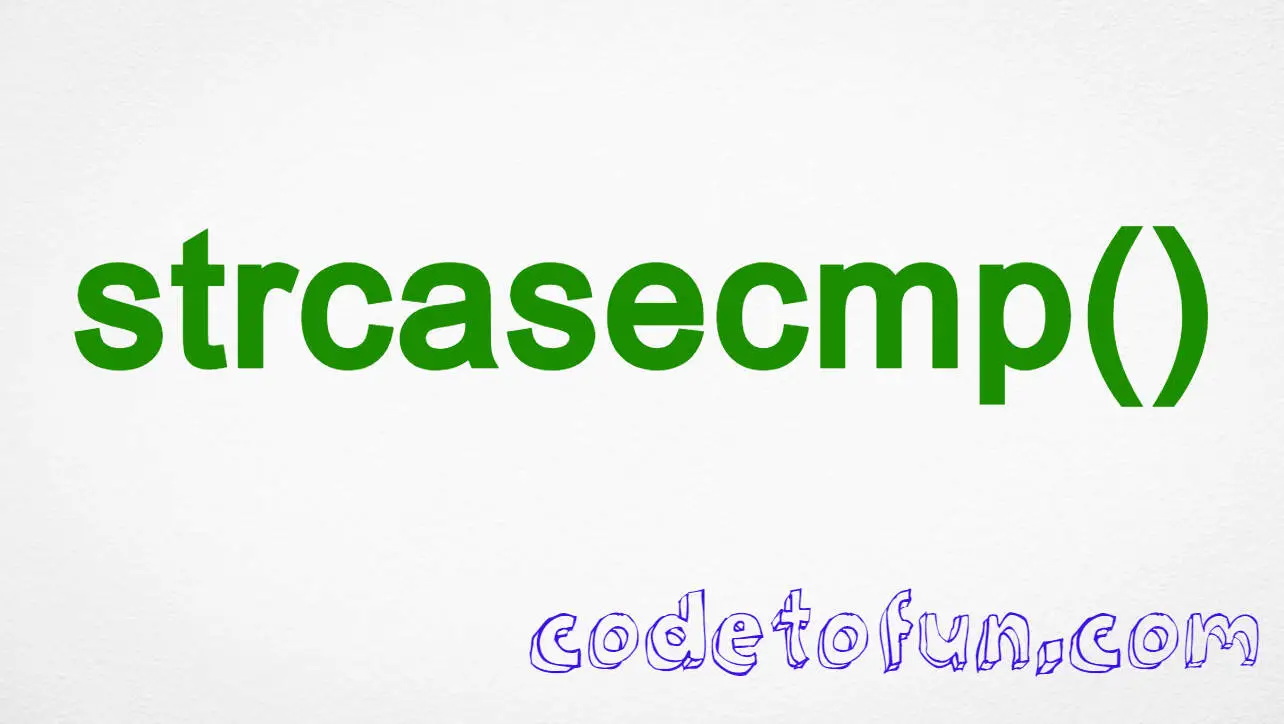
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to perform Matrix Transpose
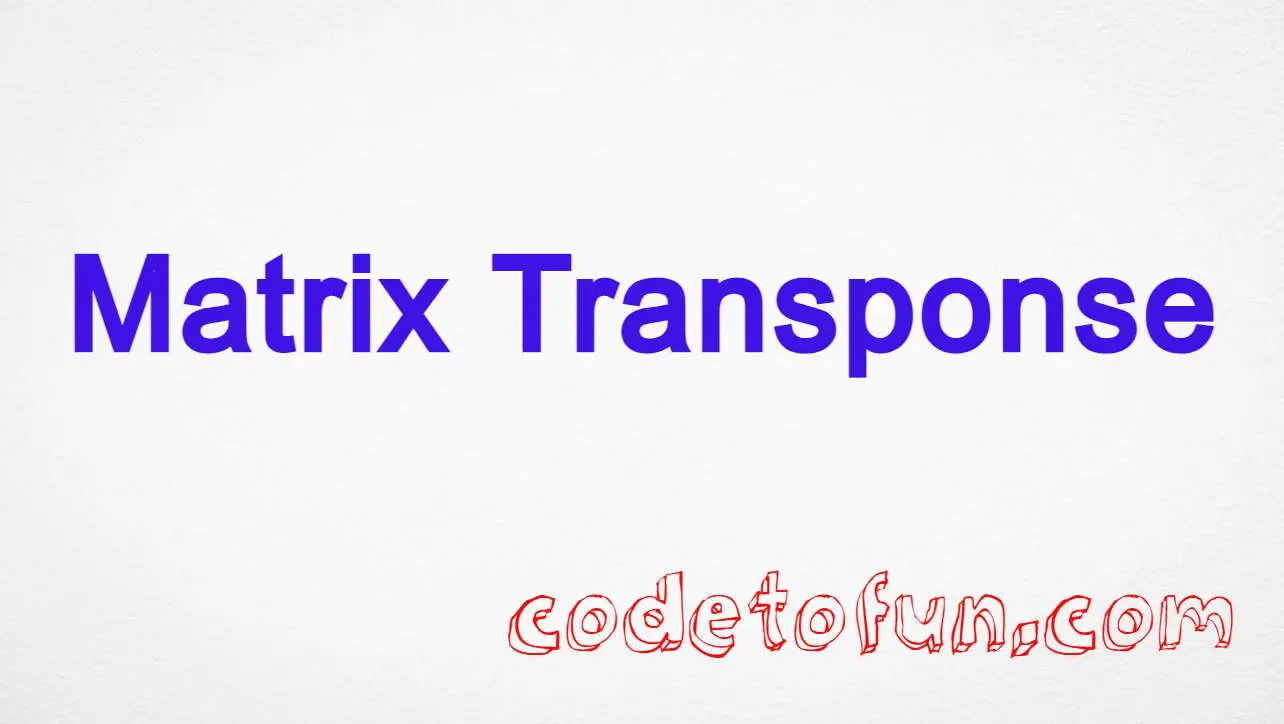
Photo Credit to CodeToFun
π Introduction
Matrix operations are fundamental in various fields of computer science and mathematics. One common operation is the transpose of a matrix.
Transposing a matrix involves swapping its rows with columns, resulting in a new matrix where the rows of the original matrix become the columns, and vice versa.
In this tutorial, we'll explore a simple yet effective C program that performs the transpose operation on a given matrix.
Understanding and implementing matrix operations is essential for various applications, including graphics, scientific computing, and data manipulation.
π Example
Now, let's look at the C code that performs matrix transpose.
#include <stdio.h>
// Function to perform matrix transpose
void transposeMatrix(int matrix[10][10], int rows, int cols) {
int transposedMatrix[10][10];
// Performing the transpose operation
for (int i = 0; i < cols; ++i) {
for (int j = 0; j < rows; ++j) {
transposedMatrix[i][j] = matrix[j][i];
}
}
// Displaying the transposed matrix
printf("Transposed Matrix:\n");
for (int i = 0; i < cols; ++i) {
for (int j = 0; j < rows; ++j) {
printf("%d\t", transposedMatrix[i][j]);
}
printf("\n");
}
}
// Driver program
int main() {
// Replace these values with your matrix dimensions and elements
int matrix[10][10] = {
{1, 2, 3},
{4, 5, 6}
};
int rows = 2;
int cols = 3;
// Call the function to perform matrix transpose
transposeMatrix(matrix, rows, cols);
return 0;
}
π» Testing the Program
To test the program with a different matrix, replace the values of matrix, rows, and cols in the main function.
Transposed Matrix: 1 4 2 5 3 6
Compile and run the program to see the transposed matrix.
π§ How the Program Works
- The program defines a function transposeMatrix that takes a matrix, number of rows, and number of columns as input and computes the transpose of the matrix.
- Inside the function, it uses nested loops to swap the rows with columns and stores the result in a new matrix transposedMatrix.
- The transposed matrix is then displayed.
π§ Understanding the Concept of Matrix Transpose
Matrix transpose is a fundamental operation where the rows and columns of a matrix are swapped. If a matrix has dimensions m×n, its transpose will have dimensions n×m. The element at position (i, j) in the original matrix becomes the element at position (j,i) in the transposed matrix.
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing optimizations based on the specific requirements of your application.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
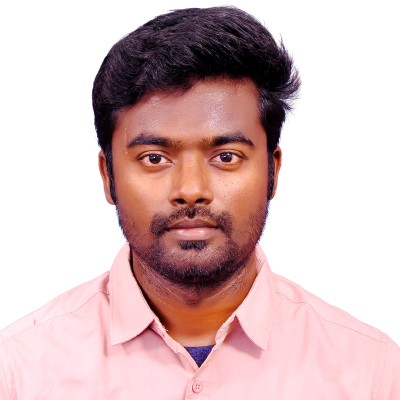
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
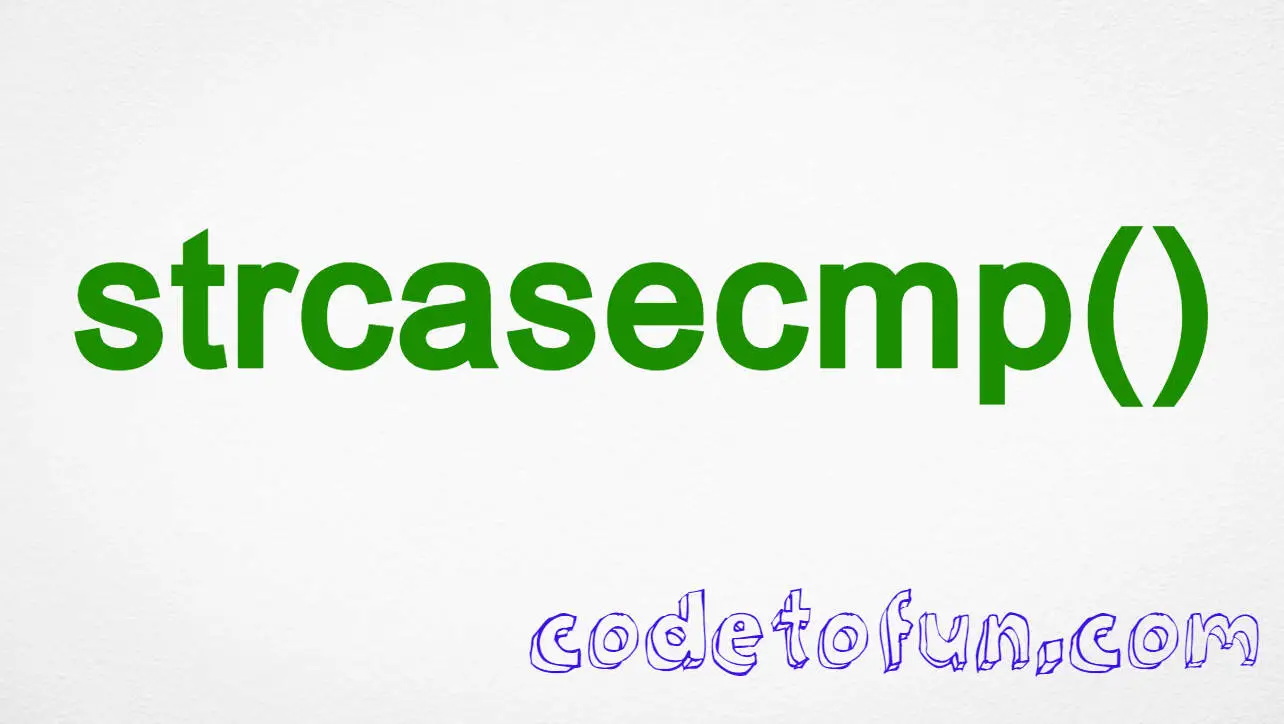
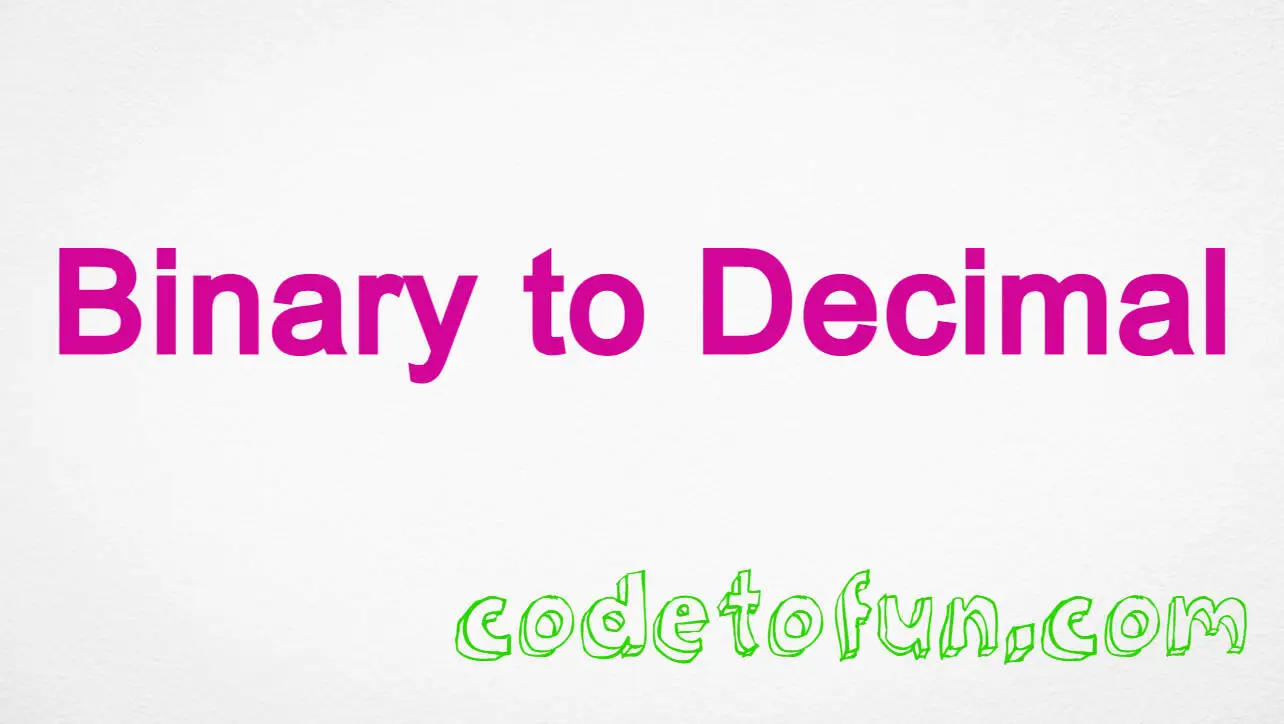
C Program to Converter a Binary to Decimal
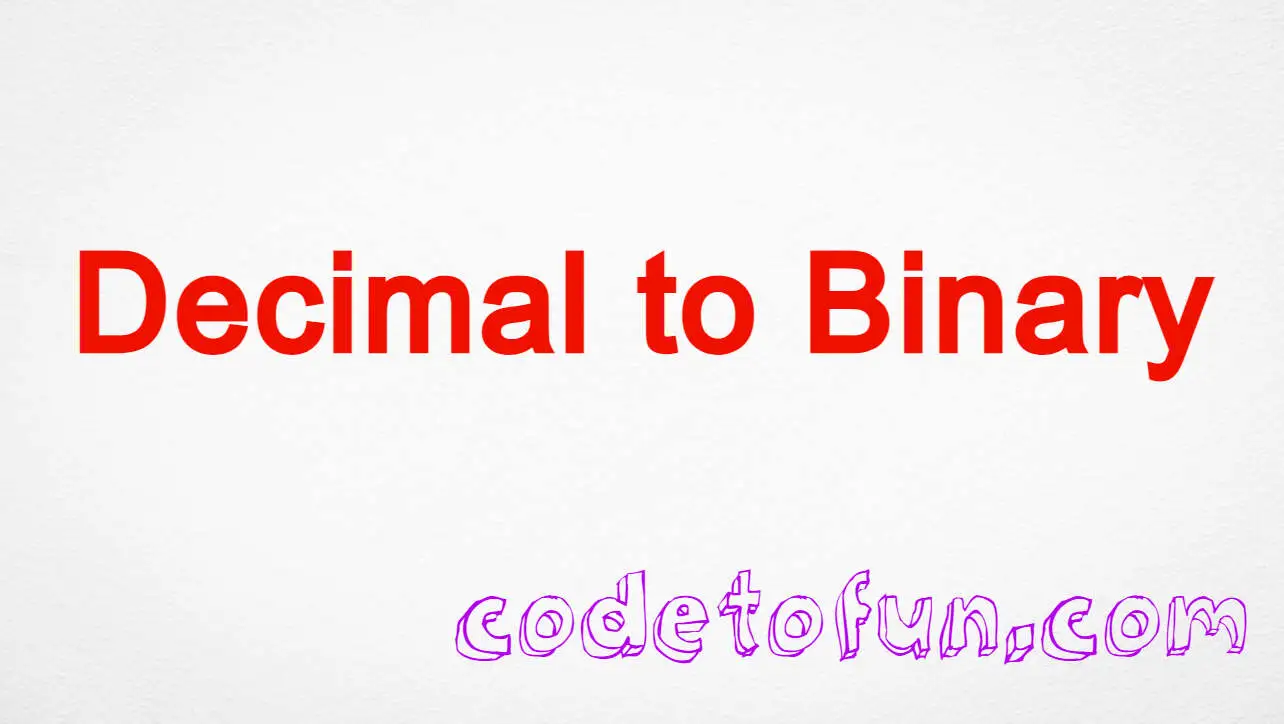
C Program to Converter a Decimal to Binary
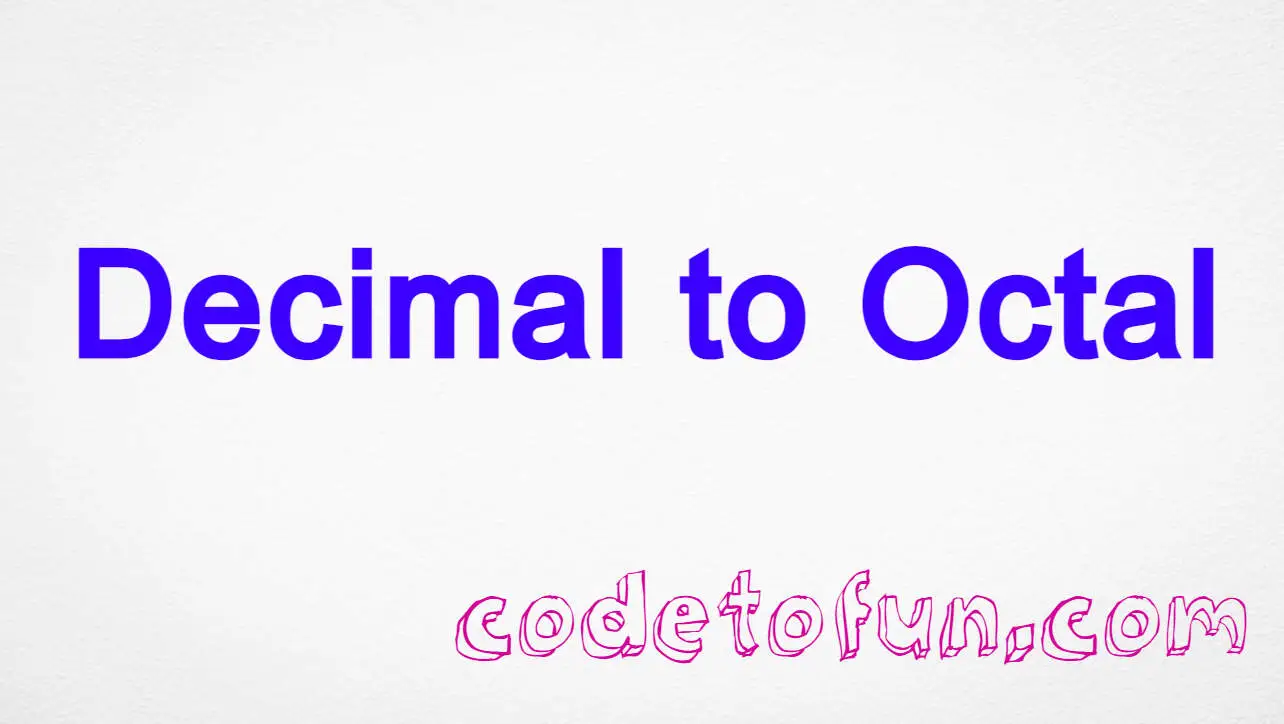
C Program to Converter a Decimal to Octal
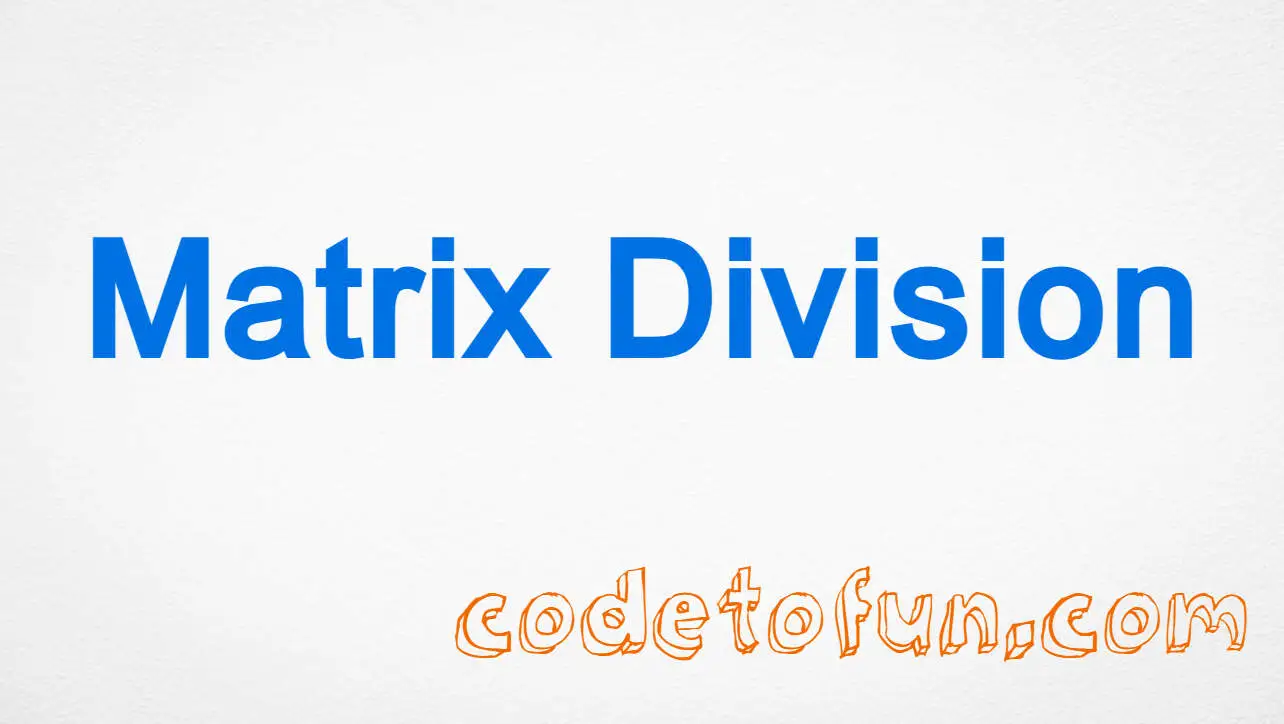
C Program to Perform Matrix Division
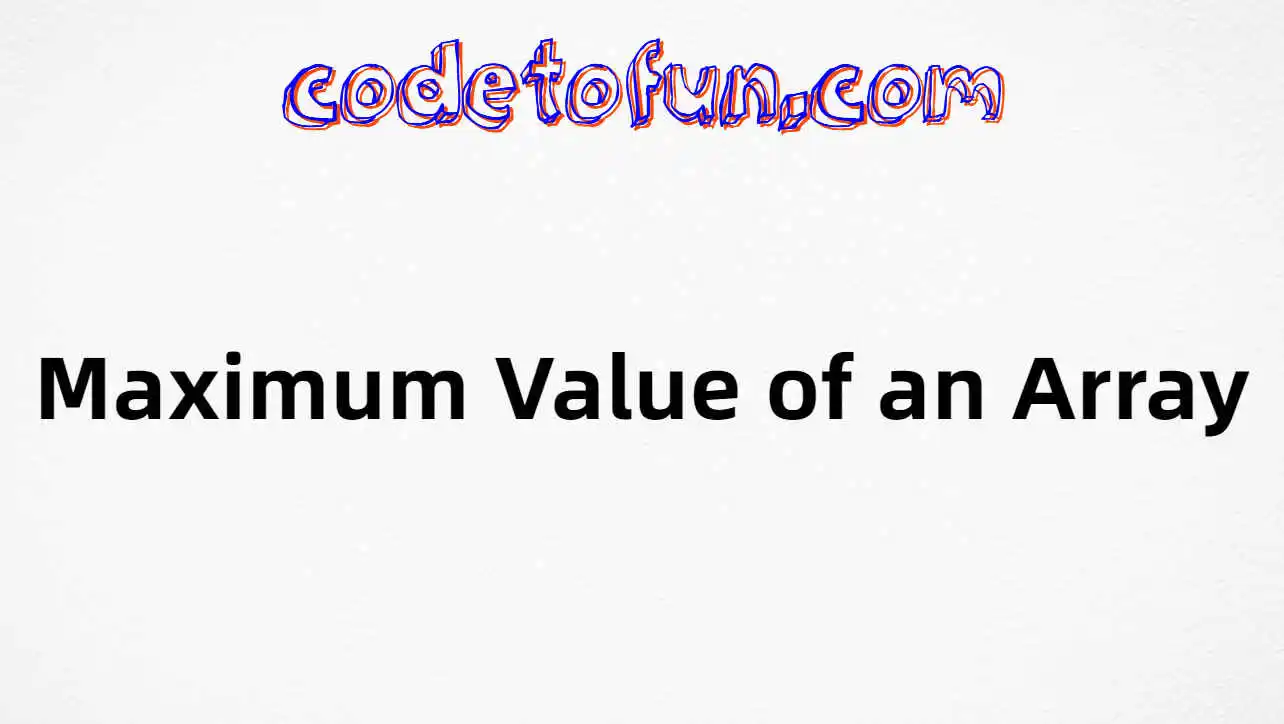
C Program to find Maximum Value of an Array
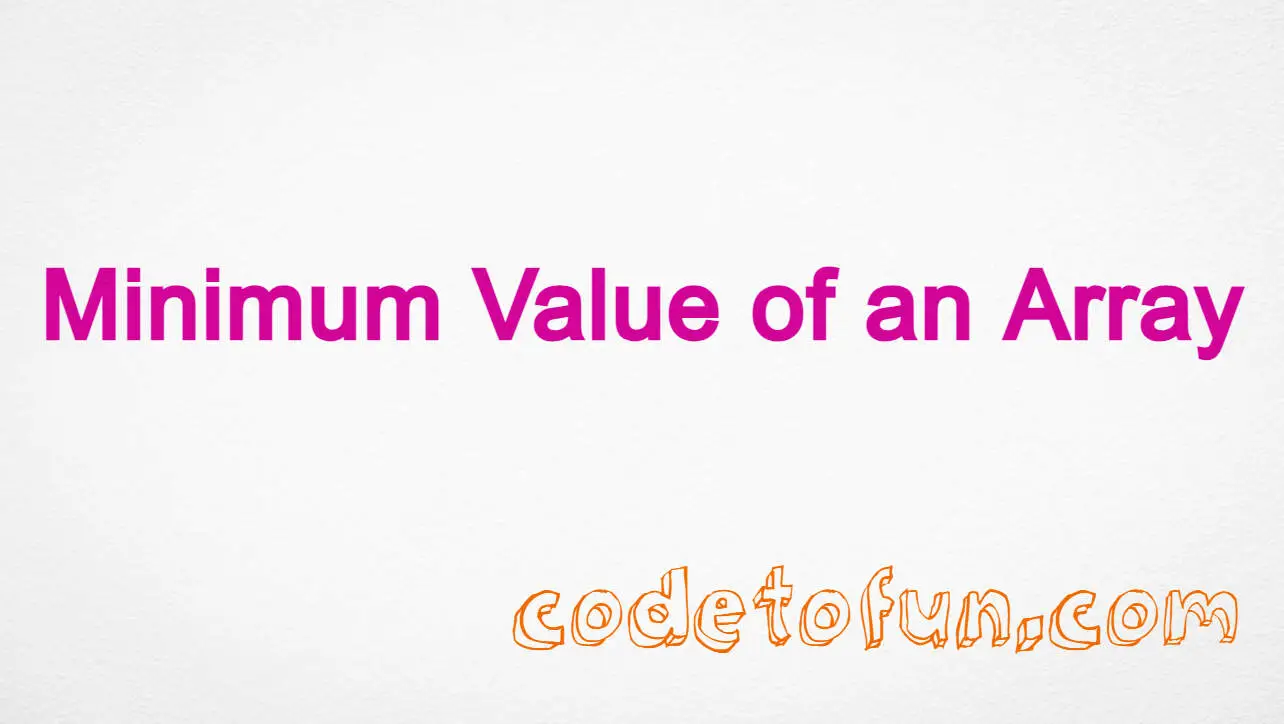
If you have any doubts regarding this article (C Program to perform Matrix Transpose), please comment here. I will help you immediately.