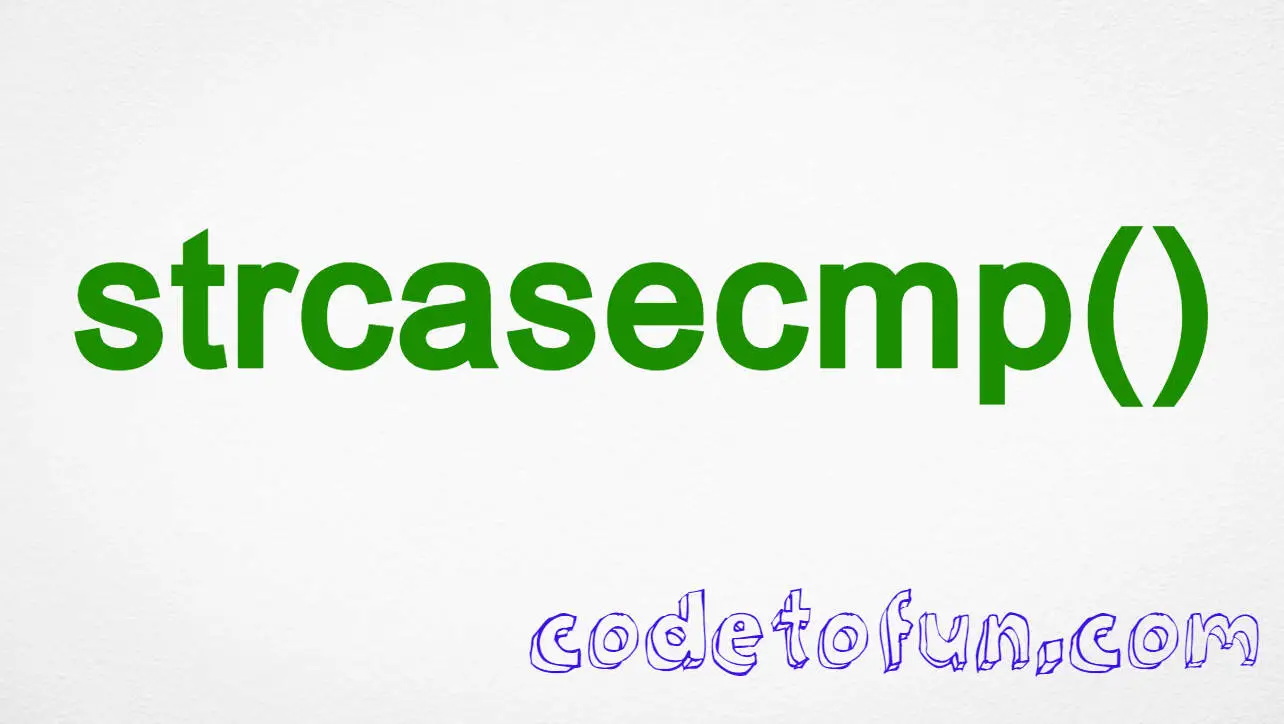
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Perform Matrix Subtraction
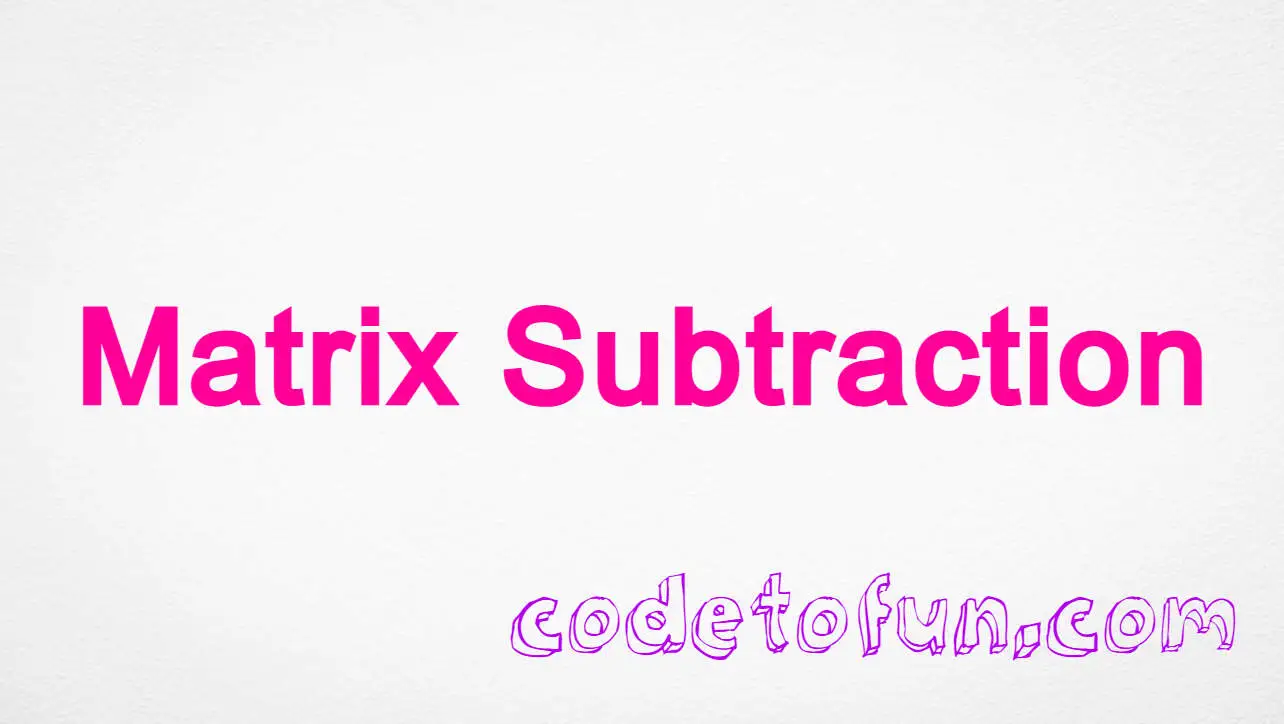
Photo Credit to CodeToFun
π Introduction
Matrix subtraction is a fundamental operation in linear algebra and computer science.
Matrix subtraction involves subtracting each element of one matrix from the corresponding element of another matrix of the same order.
In this tutorial, we'll explore a C program that performs matrix subtraction.
In the provided C program, we'll perform matrix subtraction for 3x3 matrices as an example. You can easily modify the program to work with matrices of different sizes.
π Example
Let's dive into the C code that performs matrix subtraction.
#include <stdio.h>
// Function to perform matrix subtraction
void subtractMatrices(int mat1[3][3], int mat2[3][3], int result[3][3]) {
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
result[i][j] = mat1[i][j] - mat2[i][j];
}
}
}
// Function to display a matrix
void displayMatrix(int matrix[3][3]) {
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
printf("%d\t", matrix[i][j]);
}
printf("\n");
}
}
// Driver program
int main() {
// Sample matrices for subtraction
int matrix1[3][3] = {
{5, 8, 2},
{7, 4, 9},
{3, 6, 1}
};
int matrix2[3][3] = {
{3, 1, 7},
{6, 9, 2},
{8, 5, 4}
};
int resultMatrix[3][3];
// Perform matrix subtraction
subtractMatrices(matrix1, matrix2, resultMatrix);
// Display matrices and result
printf("Matrix 1:\n");
displayMatrix(matrix1);
printf("\nMatrix 2:\n");
displayMatrix(matrix2);
printf("\nResultant Matrix (Matrix1 - Matrix2):\n");
displayMatrix(resultMatrix);
return 0;
}
π» Testing the Program
Feel free to replace the sample matrices with your own 3x3 matrices in the main function to test the program with different input.
Matrix 1: 5 8 2 7 4 9 3 6 1 Matrix 2: 3 1 7 6 9 2 8 5 4 Resultant Matrix (Matrix1 - Matrix2): 2 7 -5 1 -5 7 -5 1 -3
Compile and run the program to see the result of the matrix subtraction.
π§ How the Program Works
- The program defines a function subtractMatrices that takes two 3x3 matrices as input and calculates their subtraction, storing the result in another matrix.
- It also defines a function displayMatrix to print a matrix.
- In the main function, sample 3x3 matrices matrix1 and matrix2 are defined, and matrix subtraction is performed using the subtractMatrices function.
- The result is displayed using the displayMatrix function.
π§ Understanding the Concept of Matrix Subtraction
Matrix subtraction is performed element-wise, subtracting the corresponding elements of one matrix from the elements of another matrix. The result is a new matrix of the same order.
In the provided example, the element in the first row and first column of the result matrix is obtained by subtracting the element in the first row and first column of Matrix 2 from the element in the first row and first column of Matrix 1, and so on.
π Conclusion
Matrix subtraction is a fundamental operation in linear algebra and computer science. This C program provides a practical implementation of matrix subtraction and serves as a foundation for more advanced matrix operations.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
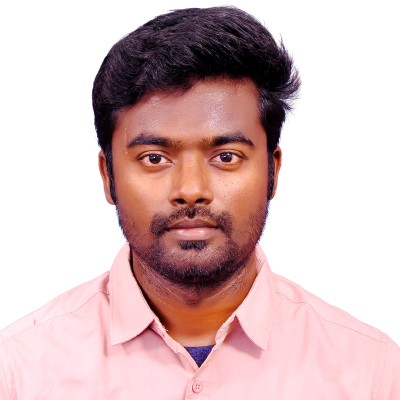
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
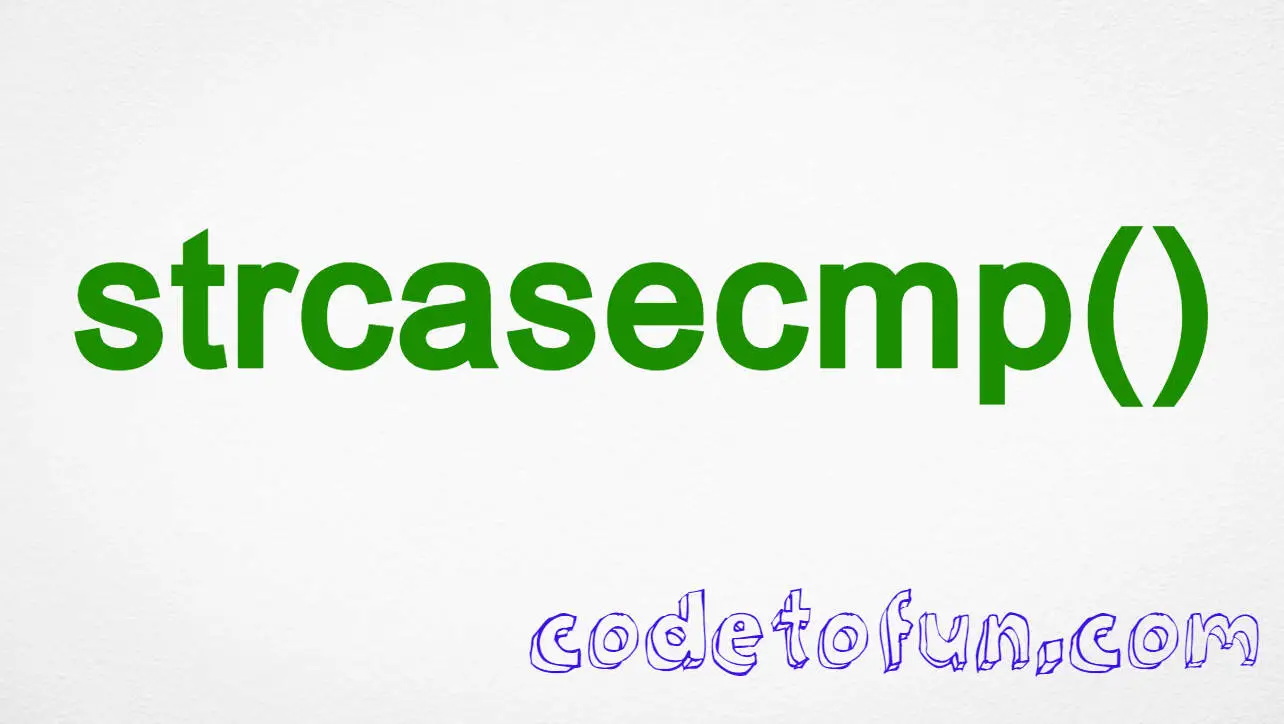
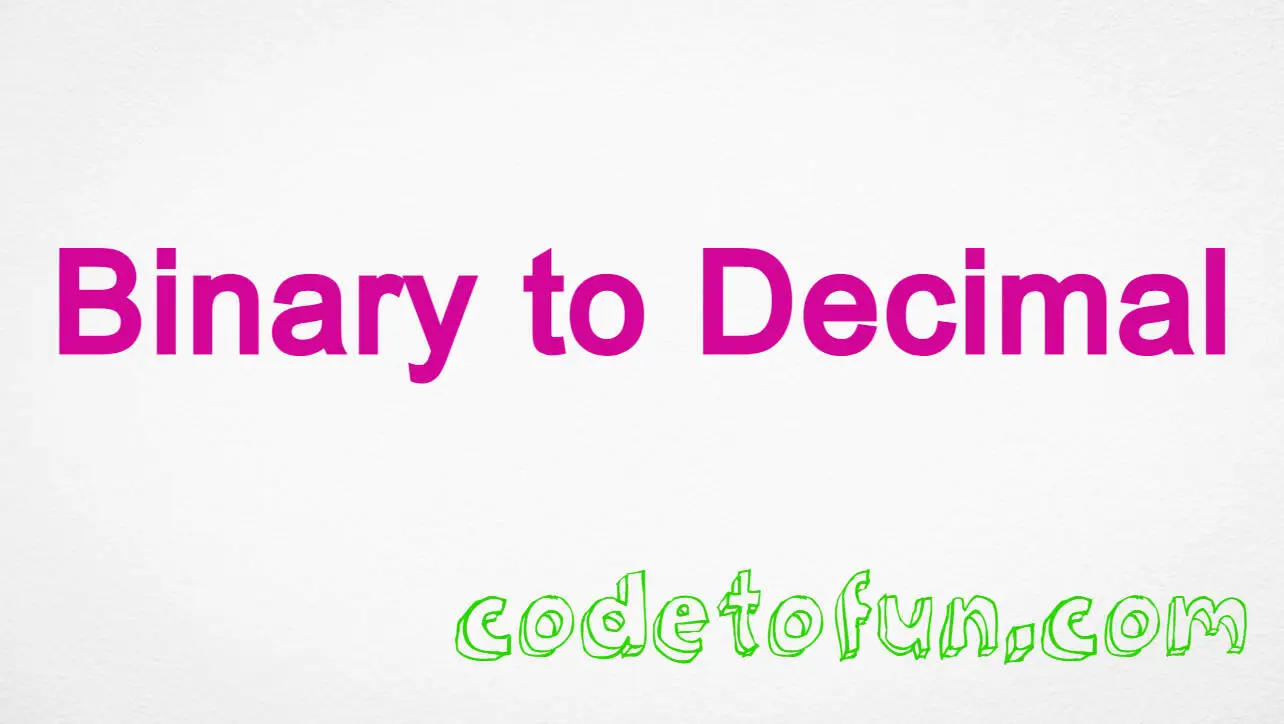
C Program to Converter a Binary to Decimal
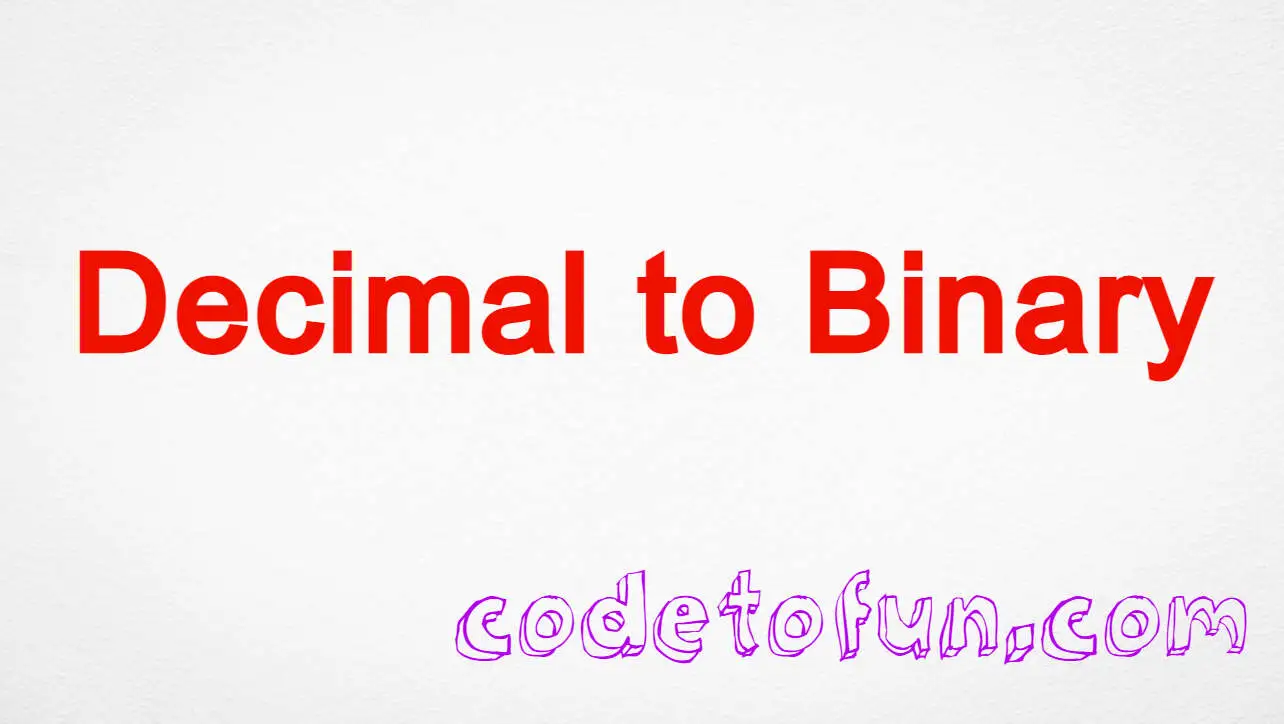
C Program to Converter a Decimal to Binary
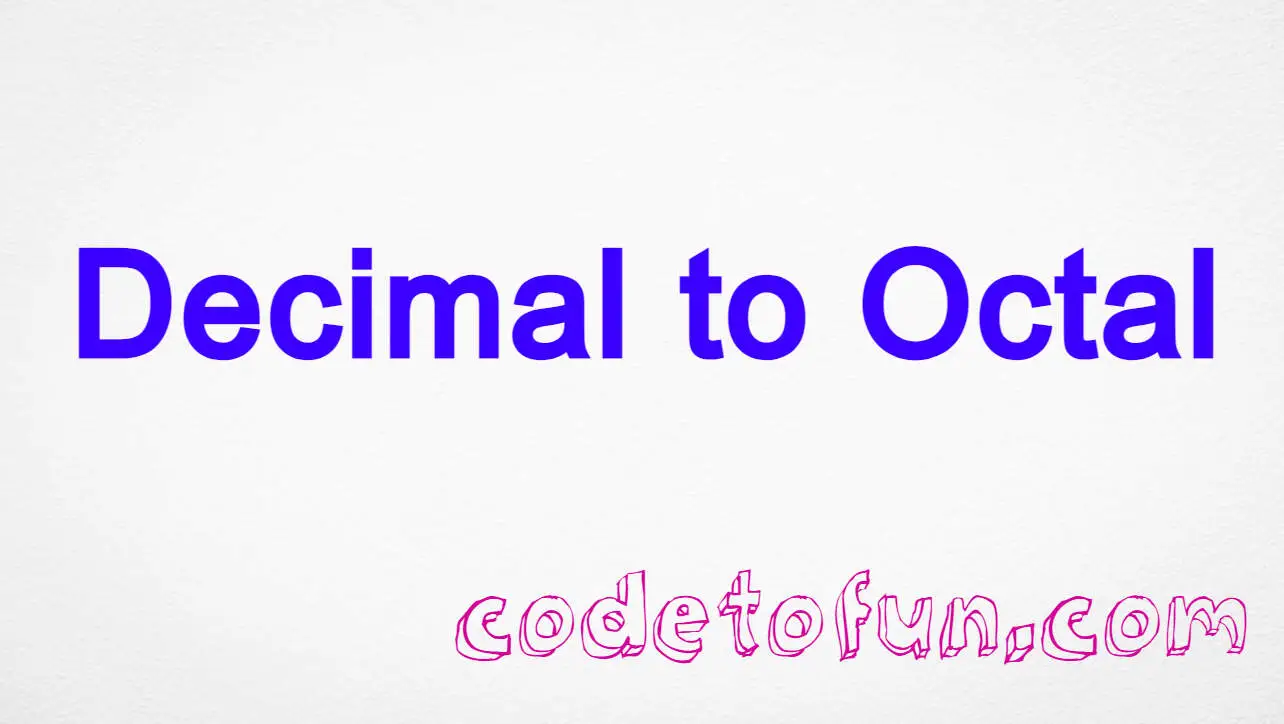
C Program to Converter a Decimal to Octal
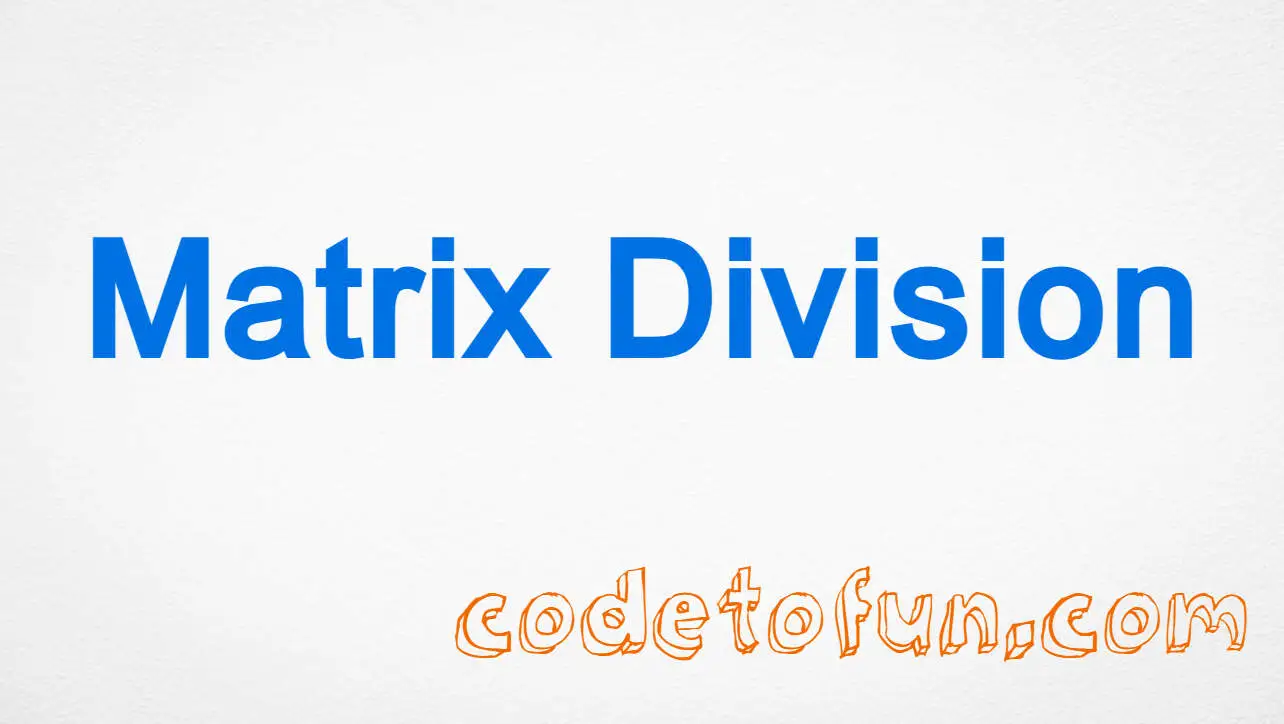
C Program to Perform Matrix Division
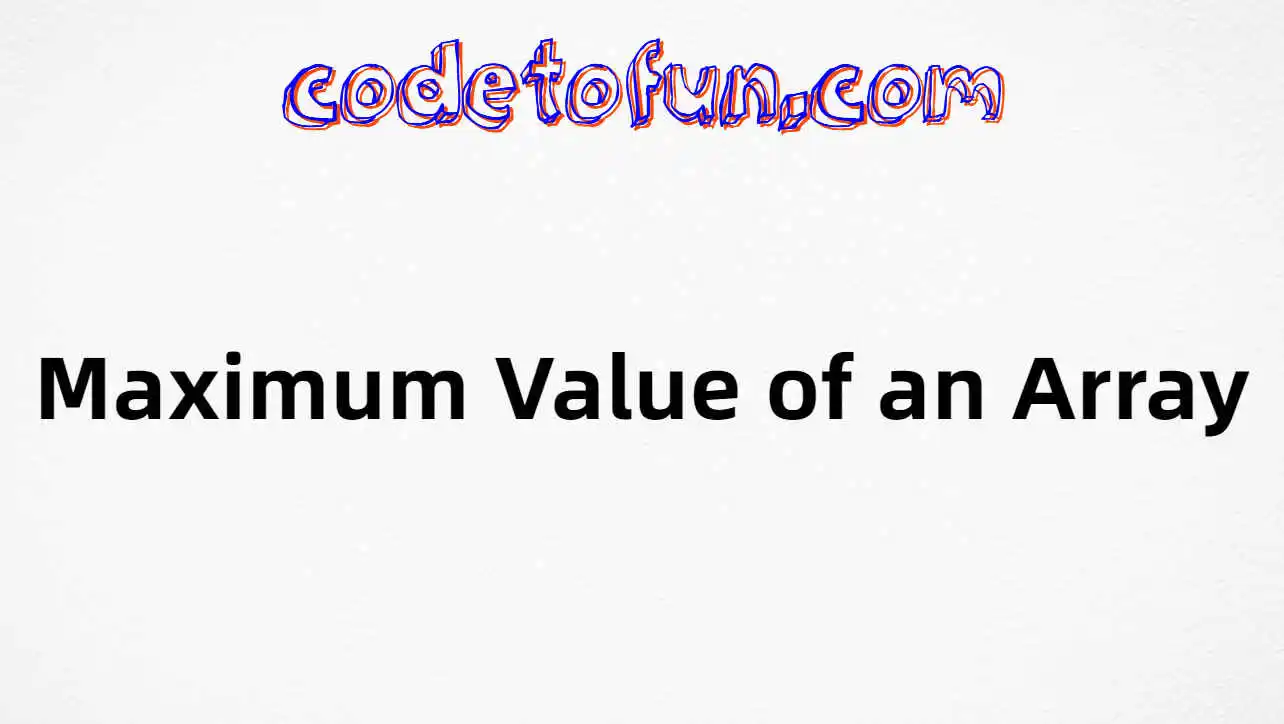
C Program to find Maximum Value of an Array
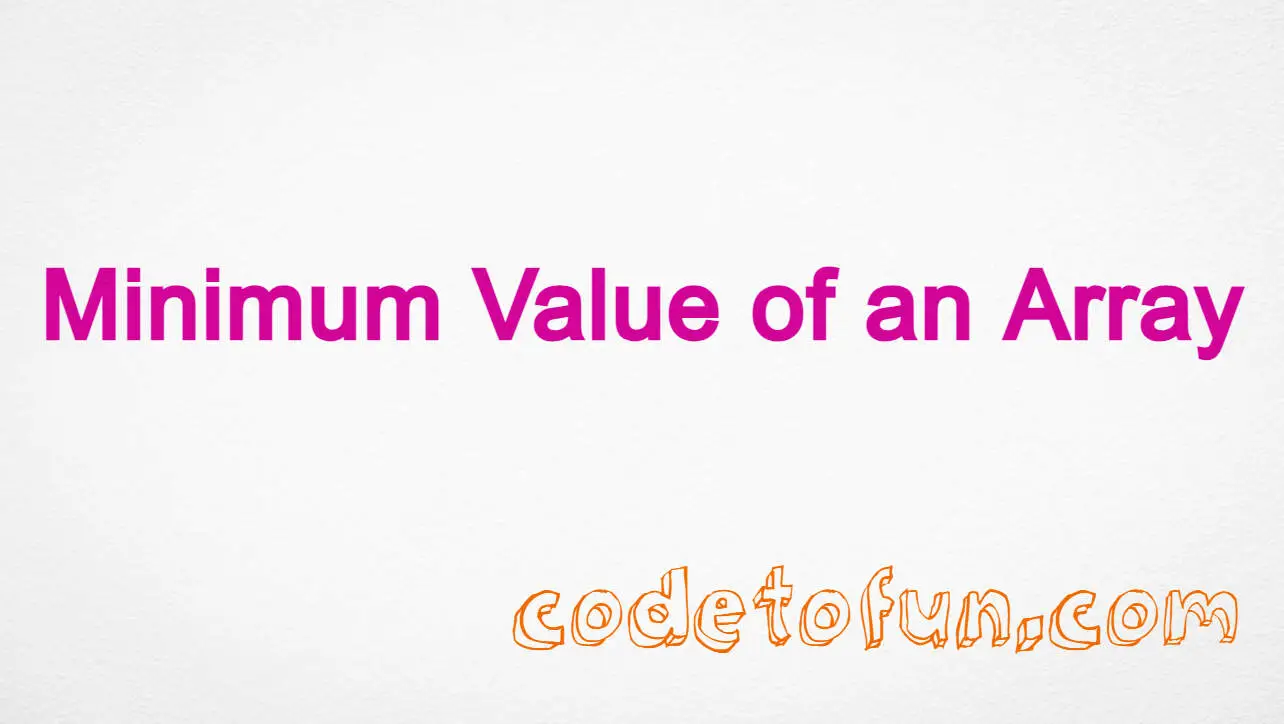
If you have any doubts regarding this article (C Program to Perform Matrix Subtraction), please comment here. I will help you immediately.