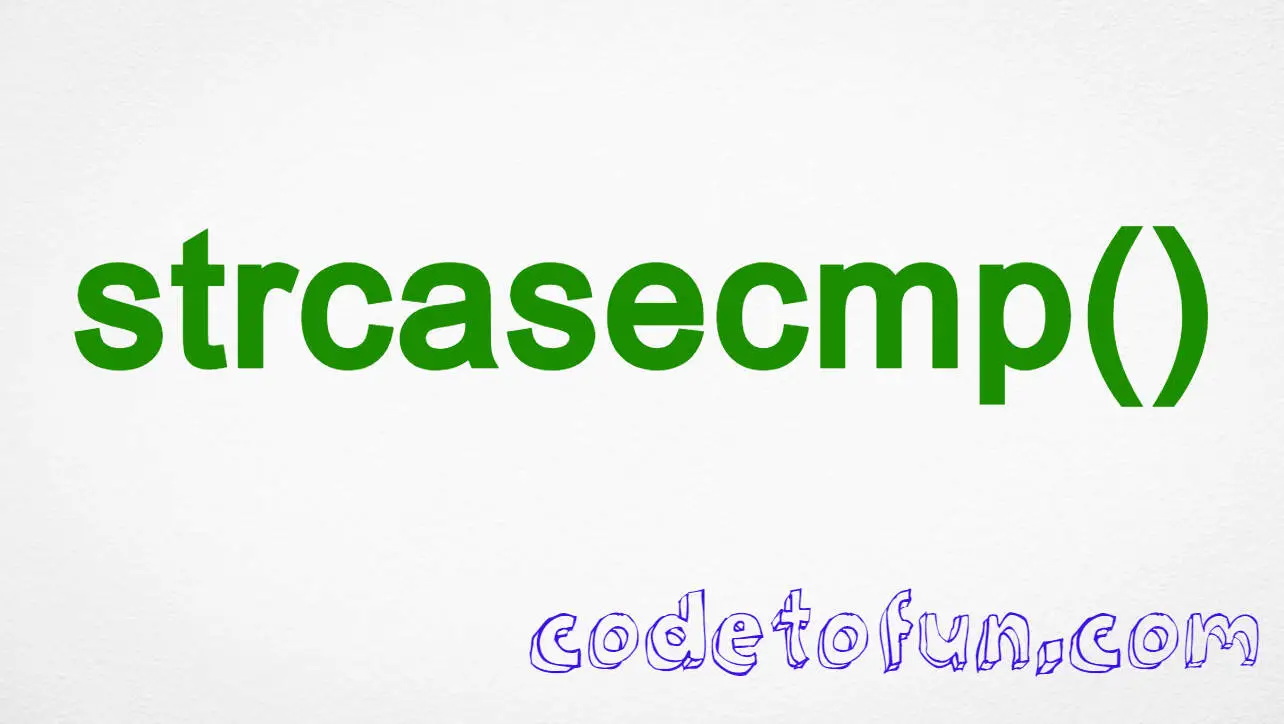
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strtok() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, string manipulation is a common task, and the strtok()
function is a powerful tool for tokenizing strings.
The strtok()
function is part of the C Standard Library (string.h) and is used to break a string into a series of tokens based on a specified set of delimiters.
In this tutorial, we'll explore the usage and functionality of the strtok()
function in C.
đĄ Syntax
The syntax for the strtok()
function is as follows:
char *strtok(char *str, const char *delimiters);
- str: The string to be tokenized. For the first call, this should be the string to tokenize. For subsequent calls, this argument should be NULL.
- delimiters: A null-terminated string containing delimiter characters. These characters are used to determine the boundaries of the tokens.
đ Example
Let's dive into an example to illustrate how the strtok()
function works.
#include <stdio.h>
#include <string.h>
int main() {
char sentence[] = "This is a sample sentence.";
const char delimiters[] = " ";
// Tokenize the sentence
char * token = strtok(sentence, delimiters);
// Loop through the tokens
while (token != NULL) {
printf("Token: %s\n", token);
token = strtok(NULL, delimiters);
}
return 0;
}
đģ Output
Token: This Token: is Token: a Token: sample Token: sentence.
đ§ How the Program Works
In this example, the strtok()
function is used to tokenize the sentence "This is a sample sentence." based on space (' ') delimiters.
âŠī¸ Return Value
The strtok()
function returns a pointer to the next token found in the string. If no more tokens are found, it returns NULL.
đ Common Use Cases
The strtok()
function is particularly useful when you need to process a string token by token, such as parsing input data or breaking down sentences into individual words.
đ Notes
- The
strtok()
function modifies the input string by replacing the delimiter characters with null characters (\0) to separate the tokens. - Be cautious when using
strtok()
in multithreaded applications, as it is not thread-safe. Consider using the strtok_r() function in such cases. - If the input string contains consecutive delimiters,
strtok()
considers them as a single delimiter.
đĸ Optimization
The strtok()
function is efficient for tokenizing strings, but for more complex string processing tasks, consider using more advanced string manipulation functions provided by the C Standard Library.
đ Conclusion
The strtok()
function in C is a valuable tool for breaking strings into tokens based on specified delimiters. It provides a flexible and efficient way to process and analyze string data.
Feel free to experiment with different strings and delimiters to understand the behavior of the strtok()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
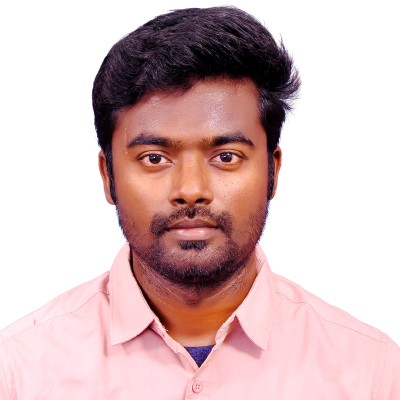
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
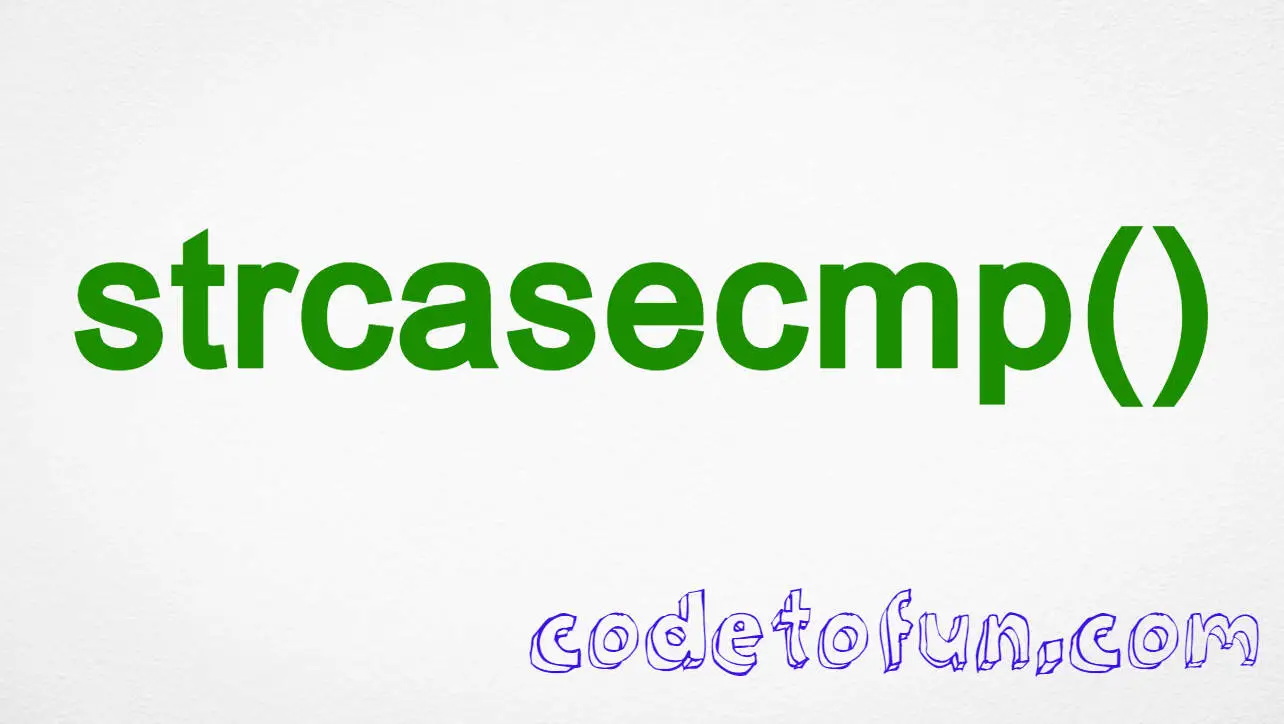
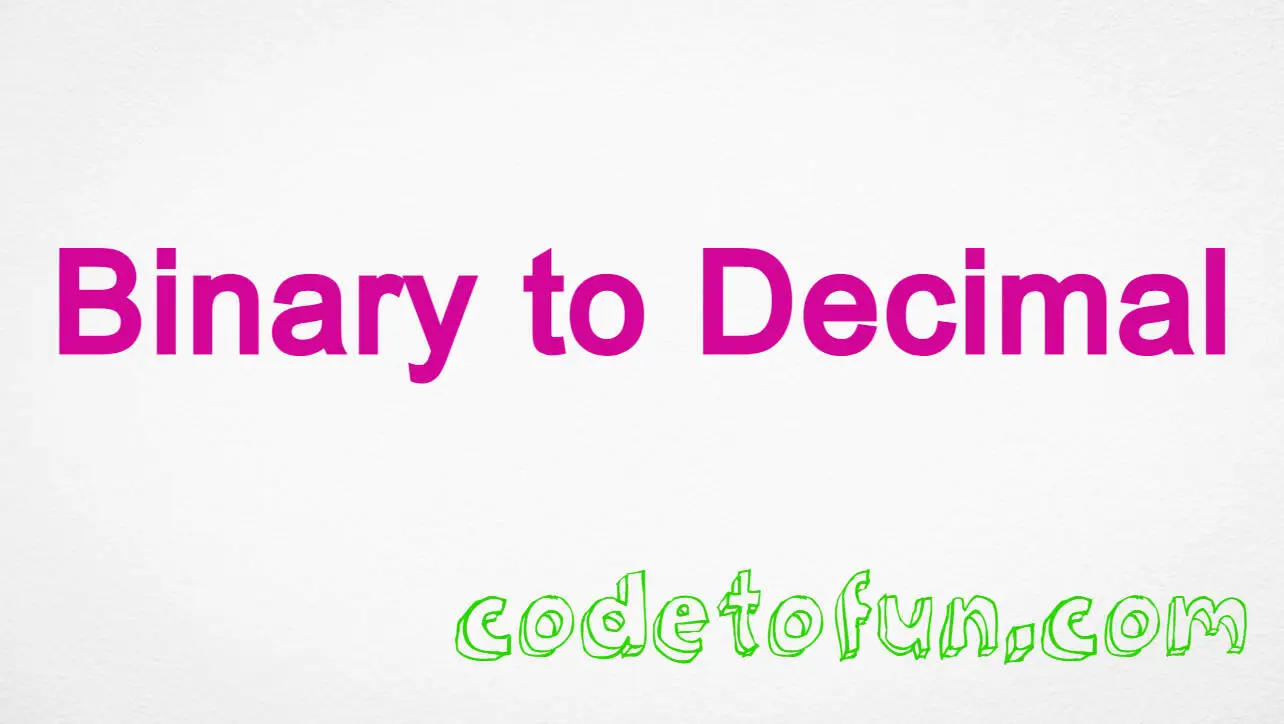
C Program to Converter a Binary to Decimal
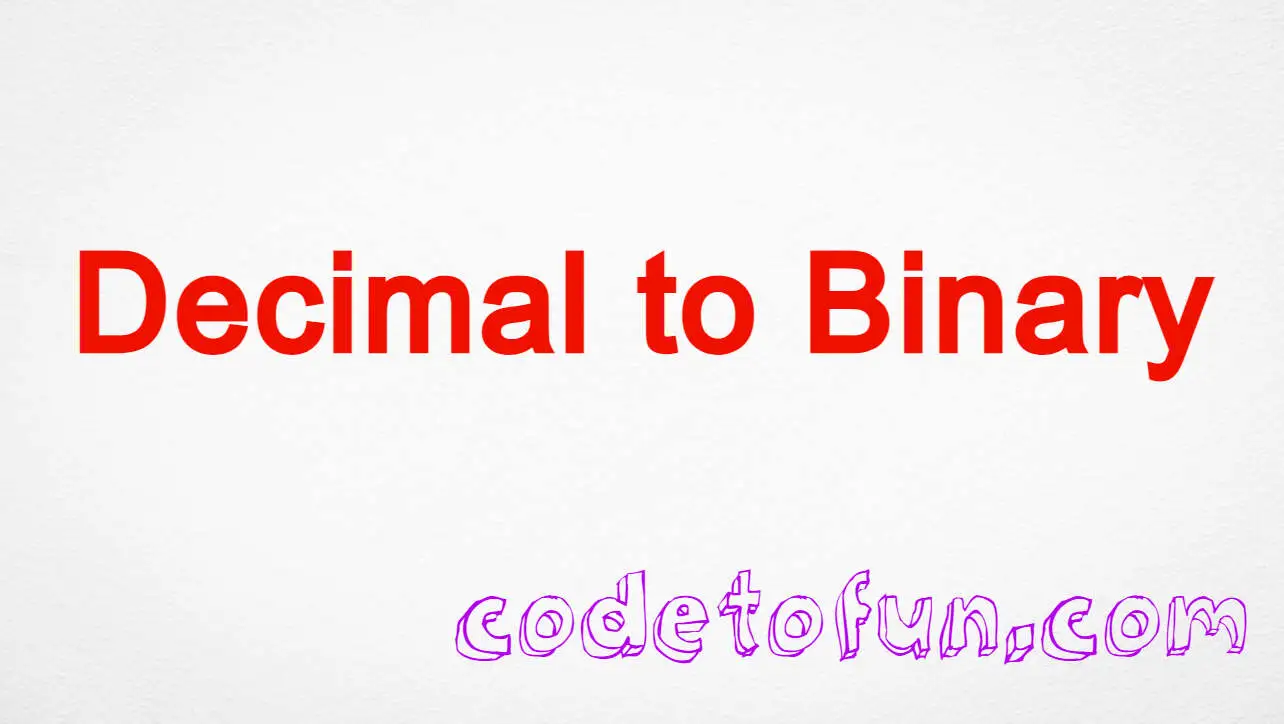
C Program to Converter a Decimal to Binary
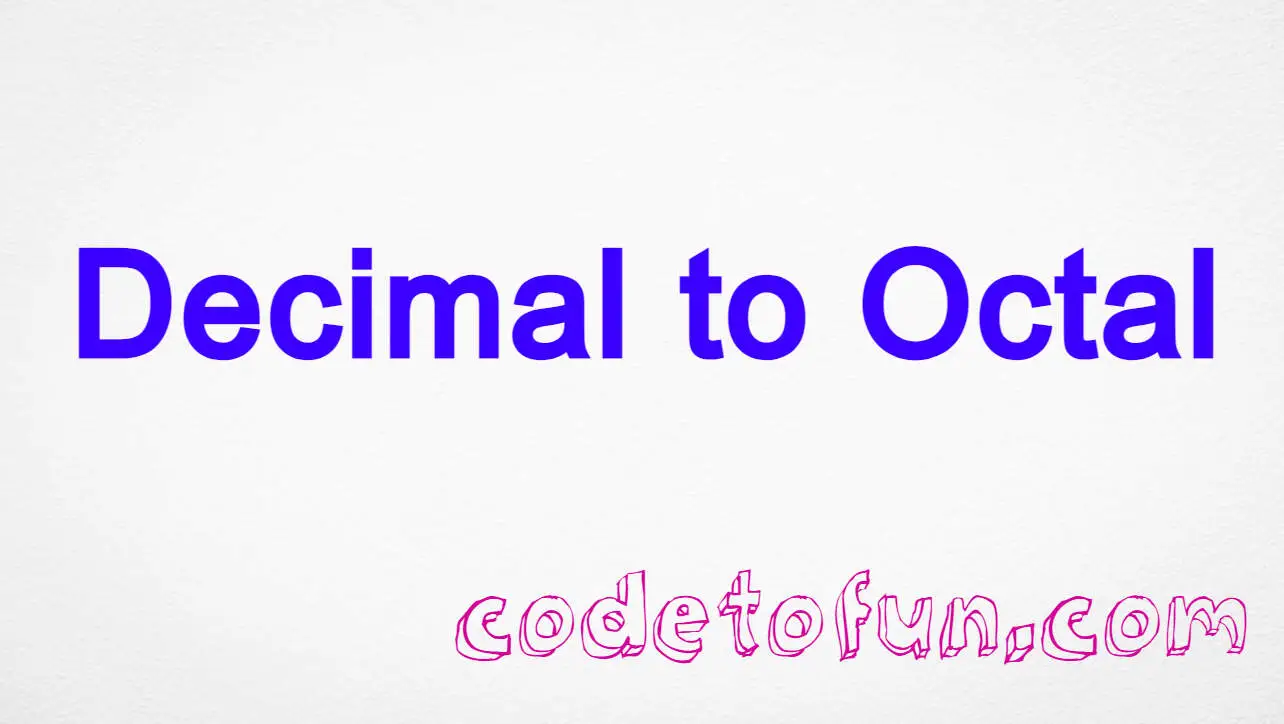
C Program to Converter a Decimal to Octal
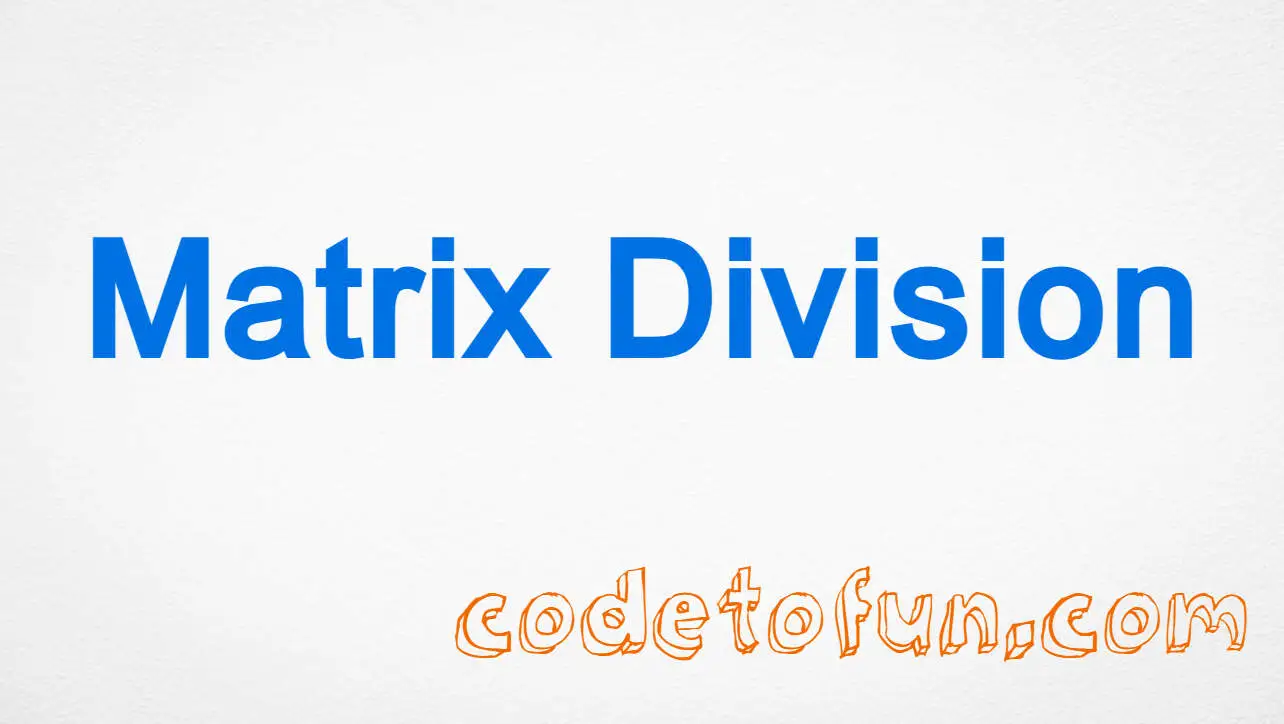
C Program to Perform Matrix Division
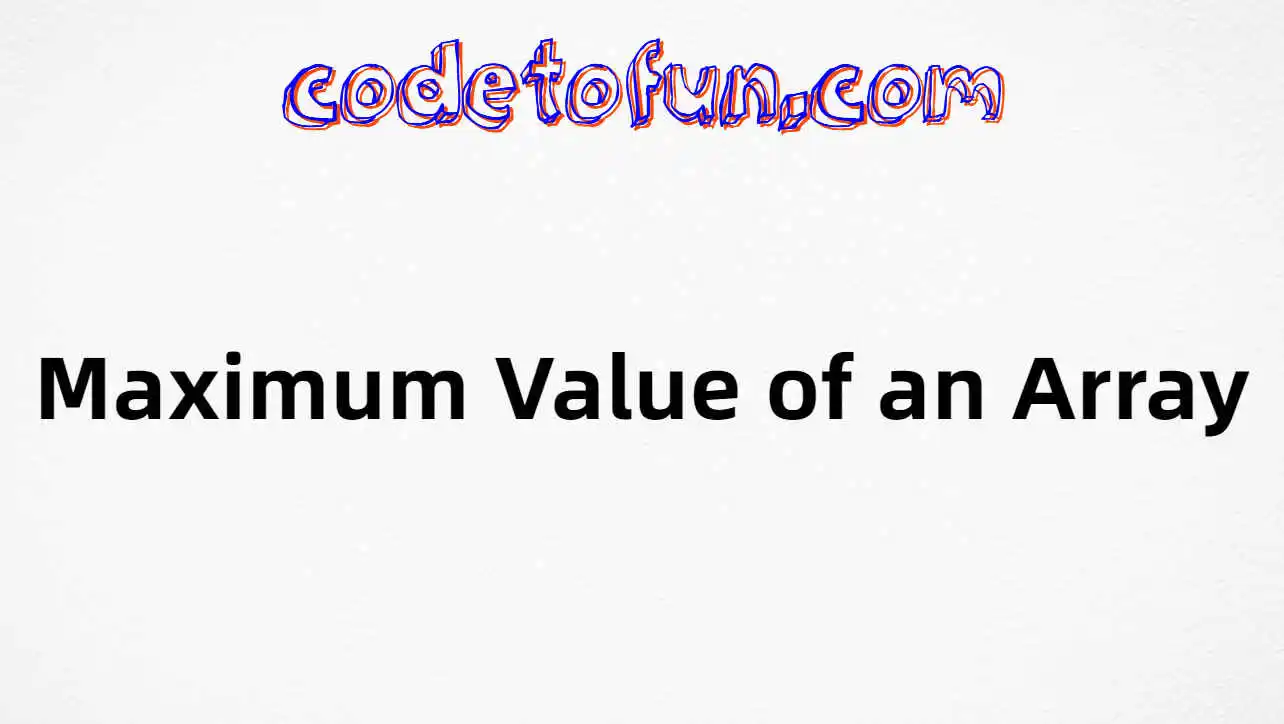
C Program to find Maximum Value of an Array
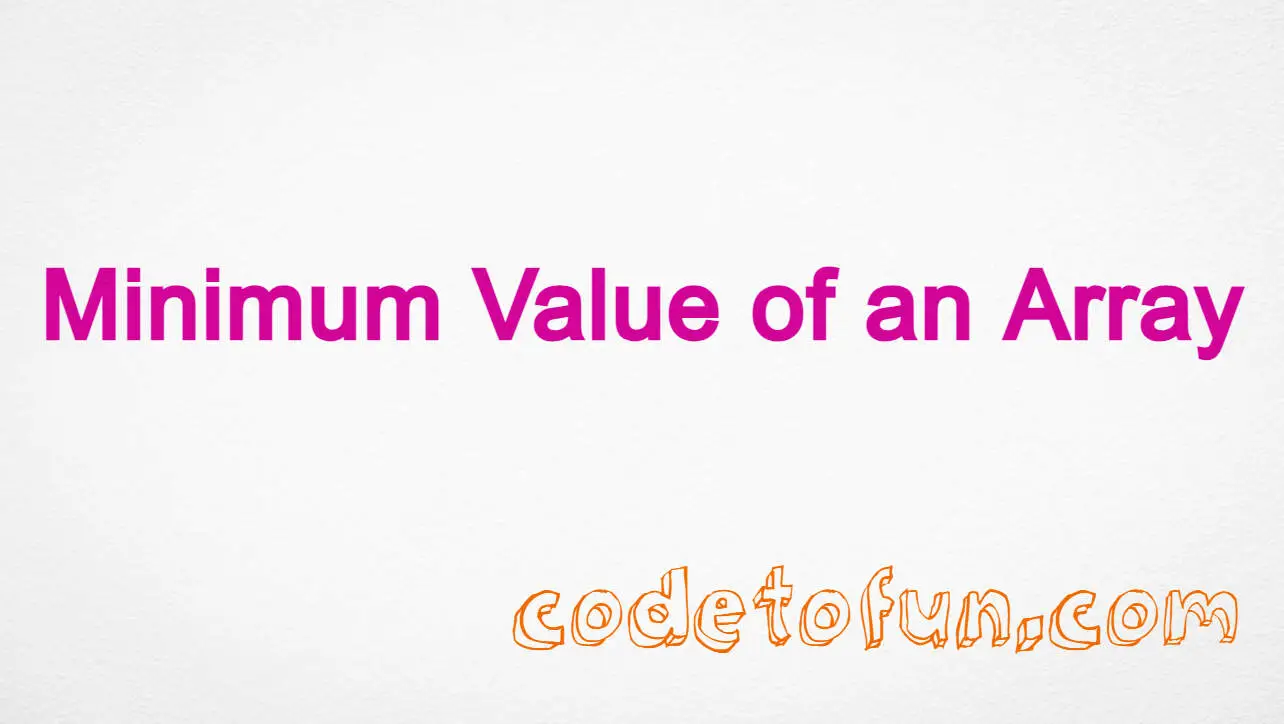
If you have any doubts regarding this article (C strtok() Function) please comment here. I will help you immediately.