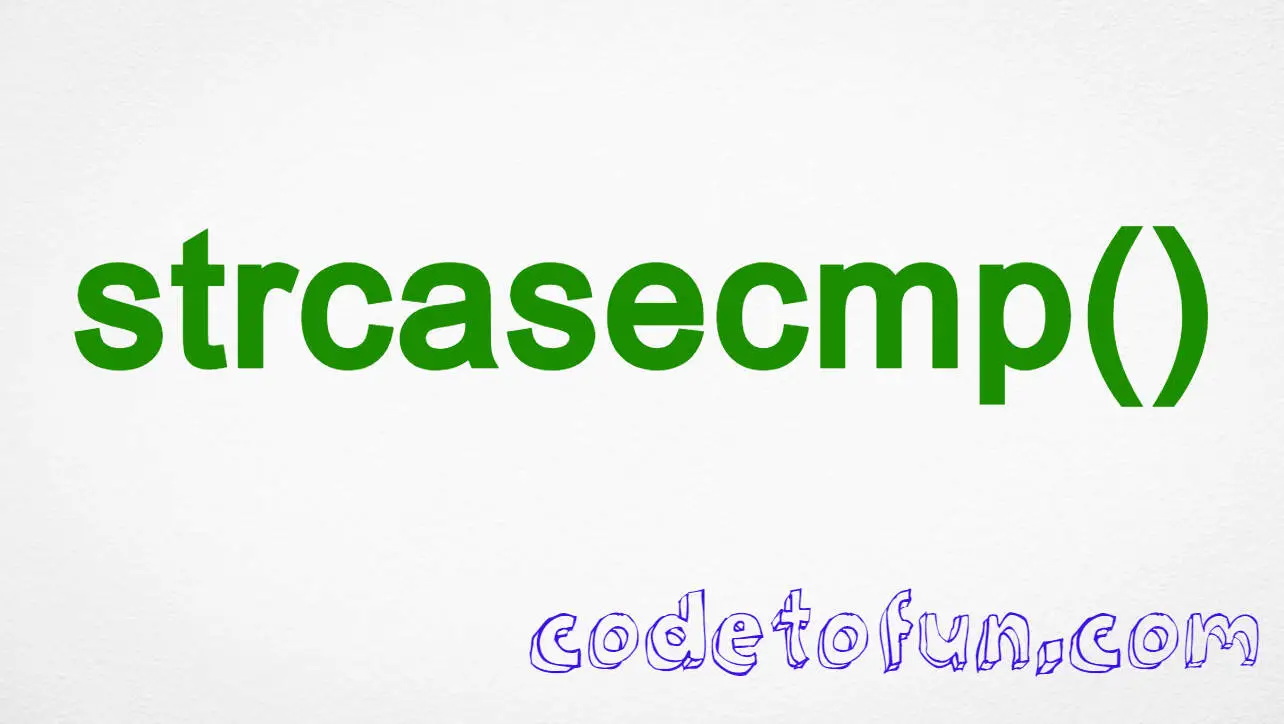
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strncpy() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, manipulating strings is a common and crucial task.
The strncpy()
function is a standard library function that copies a specified number of characters from one string to another.
In this tutorial, we'll explore the usage and functionality of the strncpy()
function in C.
đĄ Syntax
The signature of the strncpy()
function is as follows:
char *strncpy(char *dest, const char *src, size_t n);
This function copies up to n characters from the source string src to the destination string dest.
đ Example
Let's delve into an example to illustrate how the strncpy()
function works.
#include <stdio.h>
#include <string.h>
int main() {
char destination[20];
const char * source = "Hello, World!";
// Copy the first 10 characters from source to destination
strncpy(destination, source, 10);
destination[10] = '\0'; // Ensure null-termination
// Output the result
printf("Copied String: %s\n", destination);
return 0;
}
đģ Output
Copied String: Hello, Wor
đ§ How the Program Works
In this example, the strncpy()
function copies the first 10 characters from the source string "Hello, World!" to the destination string and ensures null-termination.
âŠī¸ Return Value
The strncpy()
function returns a pointer to the destination string. It is essential to note that the destination string might not be null-terminated if the number of characters copied equals the specified limit n.
đ Common Use Cases
The strncpy()
function is useful when you need to copy a specific number of characters from one string to another, providing control over the maximum length of the copied string.
đ Notes
- If the length of the source string is less than n, the remaining characters in the destination string will be padded with null characters.
- If the length of the source string is greater than or equal to n, the destination string will not be null-terminated unless explicitly done.
đĸ Optimization
The strncpy()
function is optimized for copying a specific number of characters. Ensure that the destination string is large enough to accommodate the specified number of characters and null-termination.
đ Conclusion
The strncpy()
function in C is a valuable tool for controlled string copying. It provides a way to copy a specific number of characters, allowing you to manage buffer sizes efficiently.
Feel free to experiment with different source strings, destination sizes, and copy lengths to explore the behavior of the strncpy()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
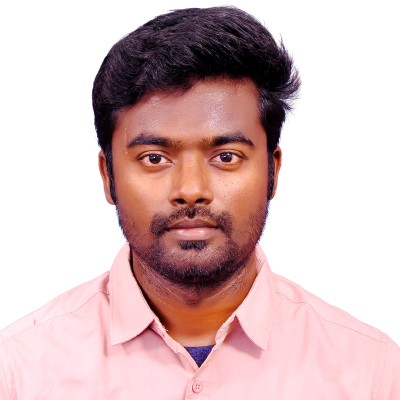
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
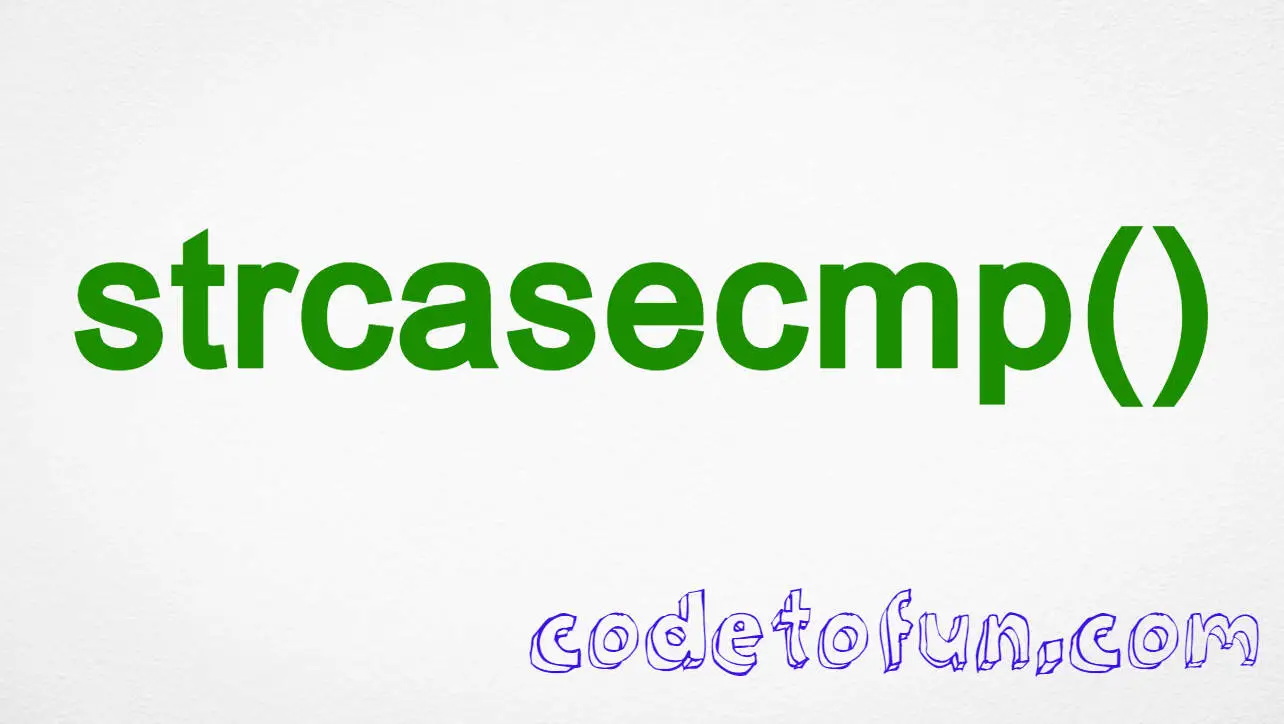
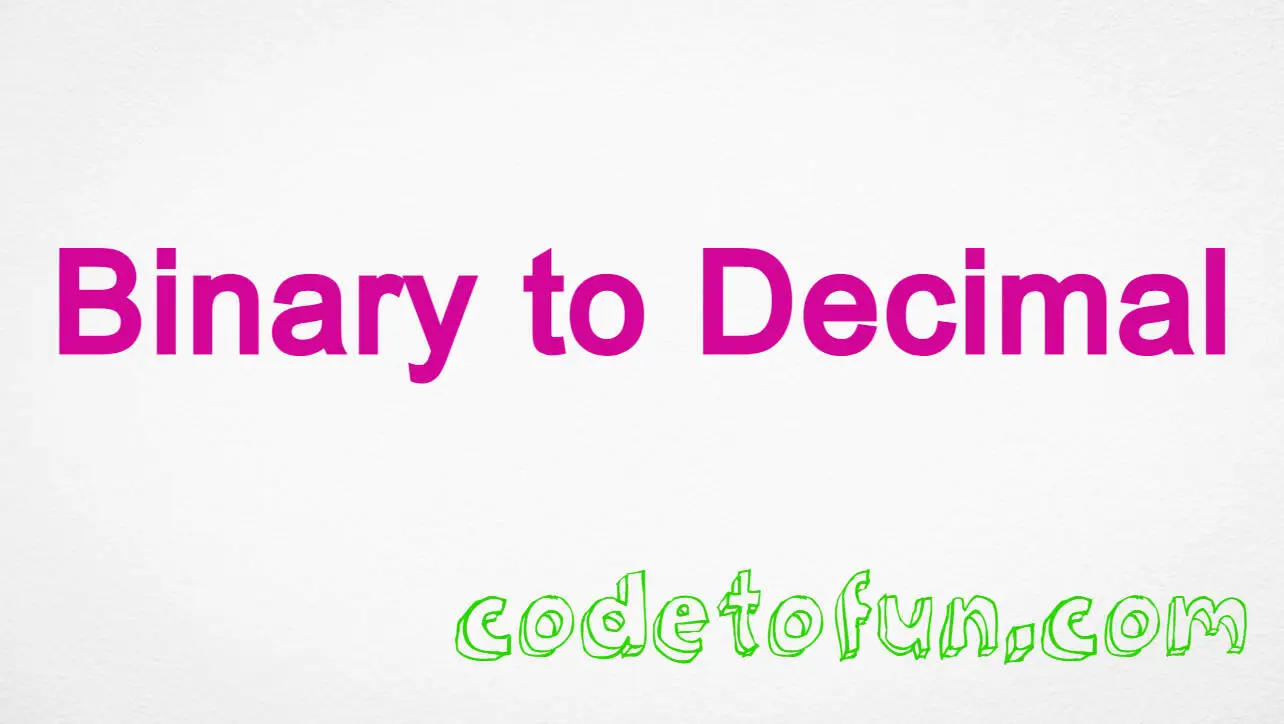
C Program to Converter a Binary to Decimal
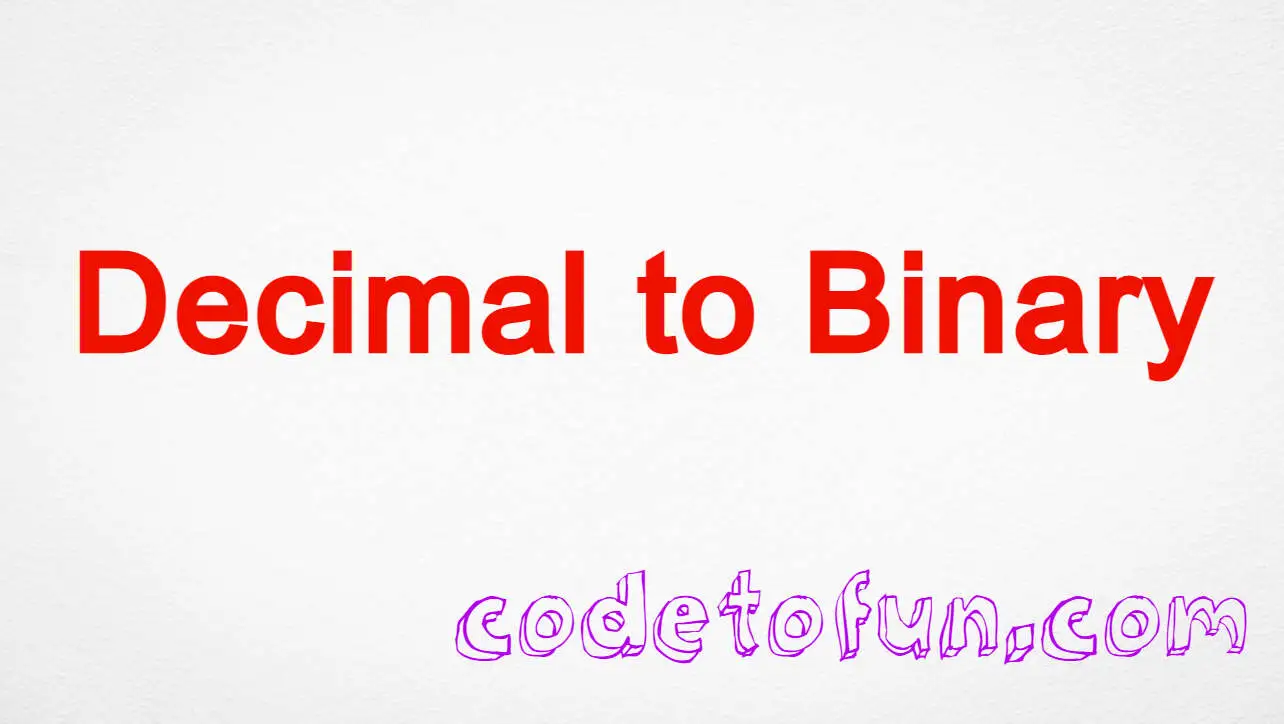
C Program to Converter a Decimal to Binary
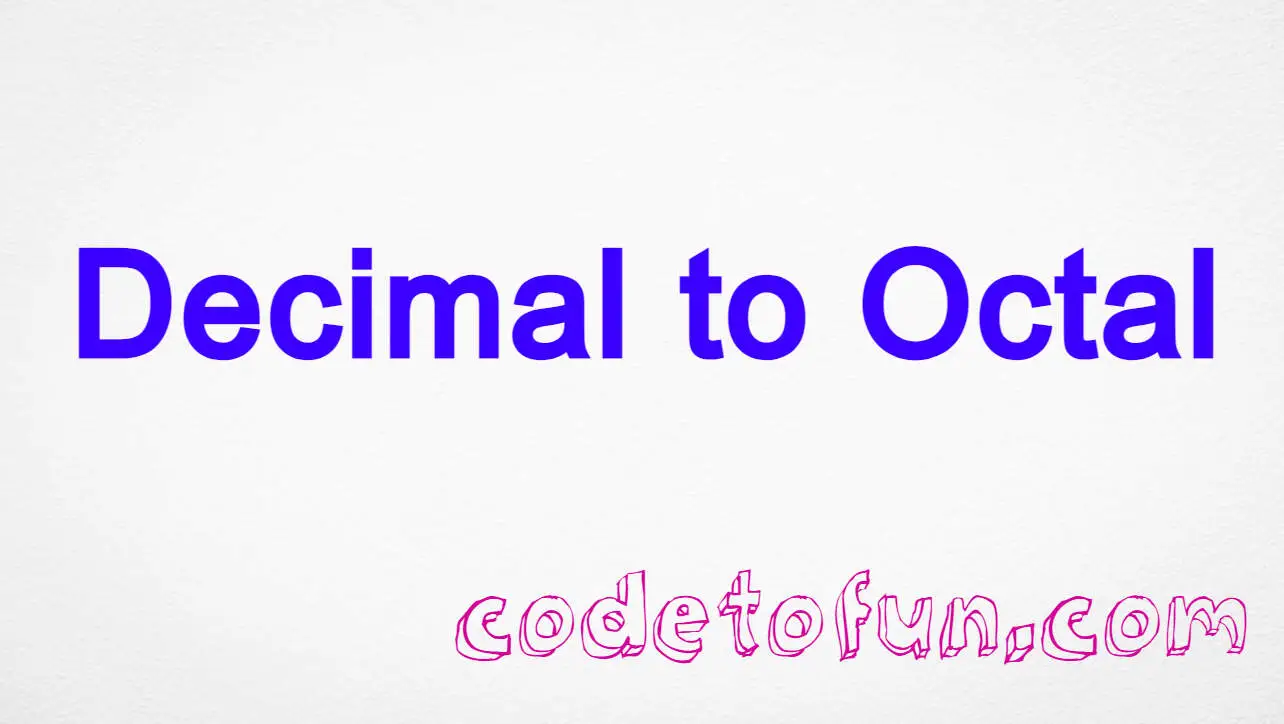
C Program to Converter a Decimal to Octal
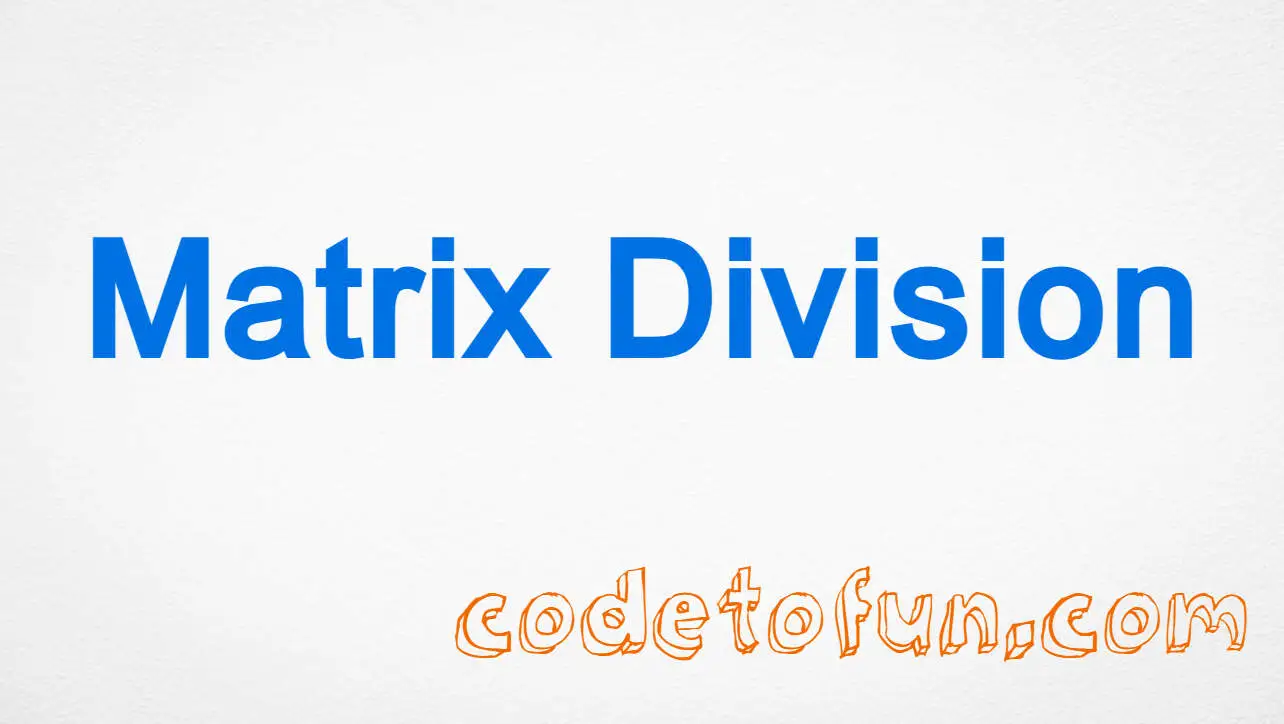
C Program to Perform Matrix Division
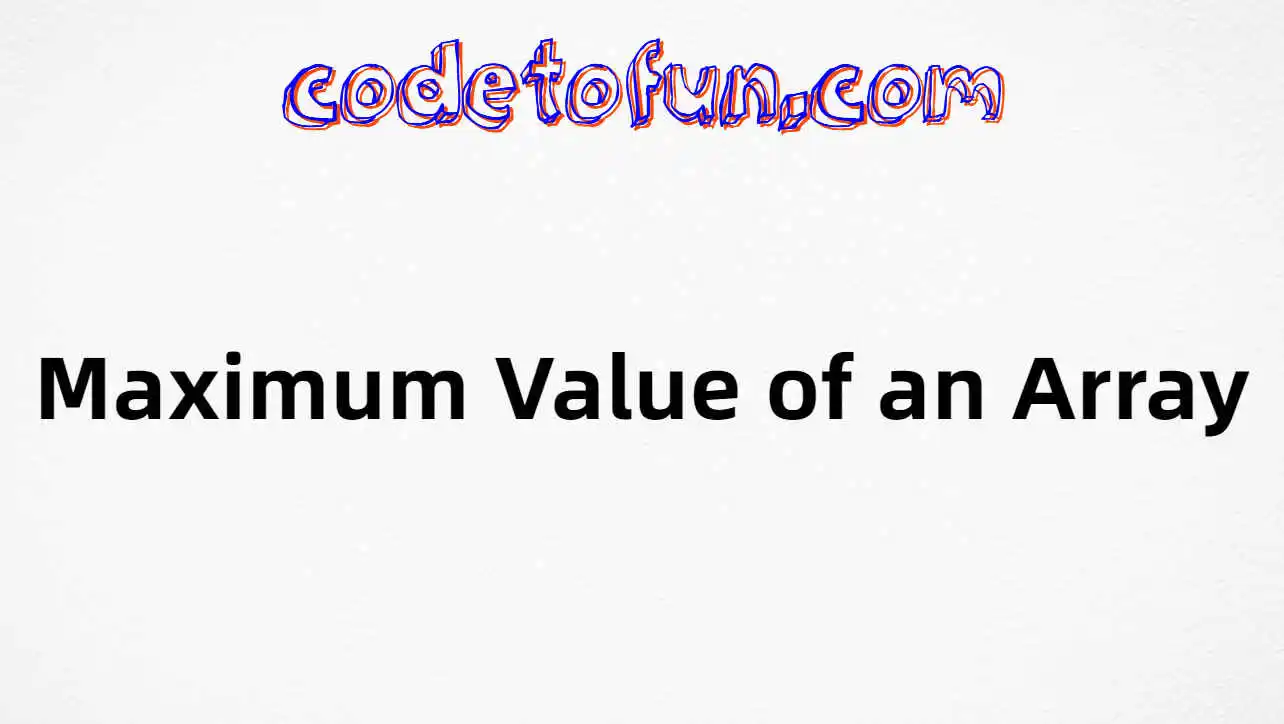
C Program to find Maximum Value of an Array
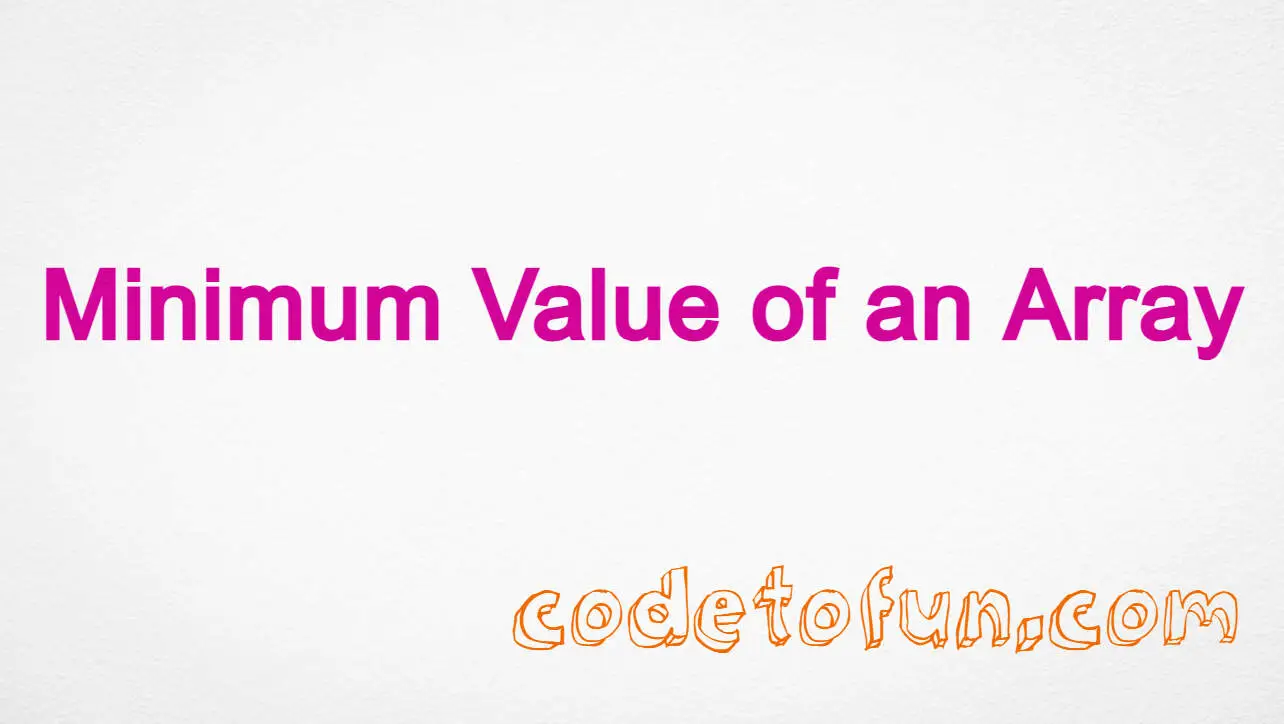
If you have any doubts regarding this article (C strncpy() Function) please comment here. I will help you immediately.