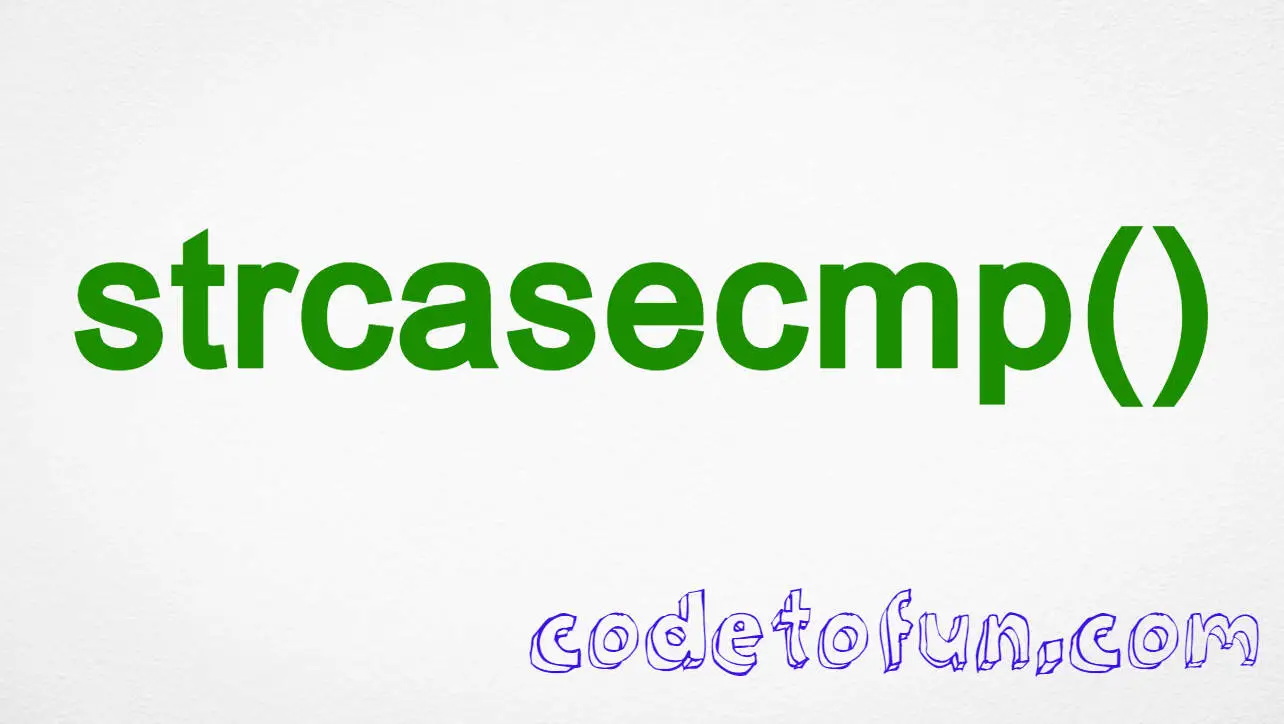
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strcmp() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, the strcmp()
function is a standard library function used for comparing strings.
It allows you to determine whether two strings are equal or determine their relative order lexicographically.
In this tutorial, we'll explore the usage and functionality of the strcmp()
function in C.
đĄ Syntax
The signature of the strcmp()
function is as follows:
int strcmp(const char *s1, const char *s2);
This function takes two strings, s1 and s2, and performs a lexicographical comparison.
đ Example
Let's delve into an example to illustrate how the strcmp()
function works.
#include <stdio.h>
#include <string.h>
int main() {
const char * str1 = "Hello, World!";
const char * str2 = "Hello, Universe!";
// Compare strings
int result = strcmp(str1, str2);
// Output the result
if (result == 0) {
printf("The strings are equal.\n");
} else if (result < 0) {
printf("str1 is less than str2.\n");
} else {
printf("str1 is greater than str2.\n");
}
return 0;
}
đģ Output
str1 is greater than str2.
đ§ How the Program Works
In this example, the strcmp()
function compares the strings "Hello, World!" and "Hello, Universe!" and prints the result.
âŠī¸ Return Value
The strcmp()
function returns an integer less than, equal to, or greater than zero if s1 is found, respectively, to be less than, to match, or be greater than s2. The comparison is based on the ASCII values of the characters in the strings.
đ Common Use Cases
The strcmp()
function is useful when you need to compare strings for equality or determine their order. It is commonly used in sorting algorithms, searching for specific strings, or checking user input.
đ Notes
- The comparison is case-sensitive. For case-insensitive comparison, consider using strcasecmp() in POSIX systems or _stricmp() in Windows.
đĸ Optimization
The strcmp()
function is optimized for lexicographical string comparison. For performance-critical applications, you may want to explore alternative approaches or consider implementing your optimized version based on specific requirements.
đ Conclusion
The strcmp()
function in C is a fundamental tool for string comparison. It provides a standardized and efficient way to compare strings, enabling you to make decisions based on their order or equality.
Feel free to experiment with different strings and explore the behavior of the strcmp()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
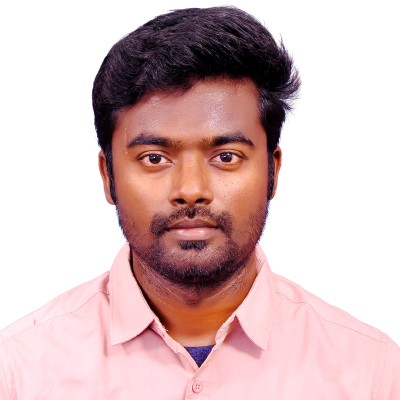
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in C
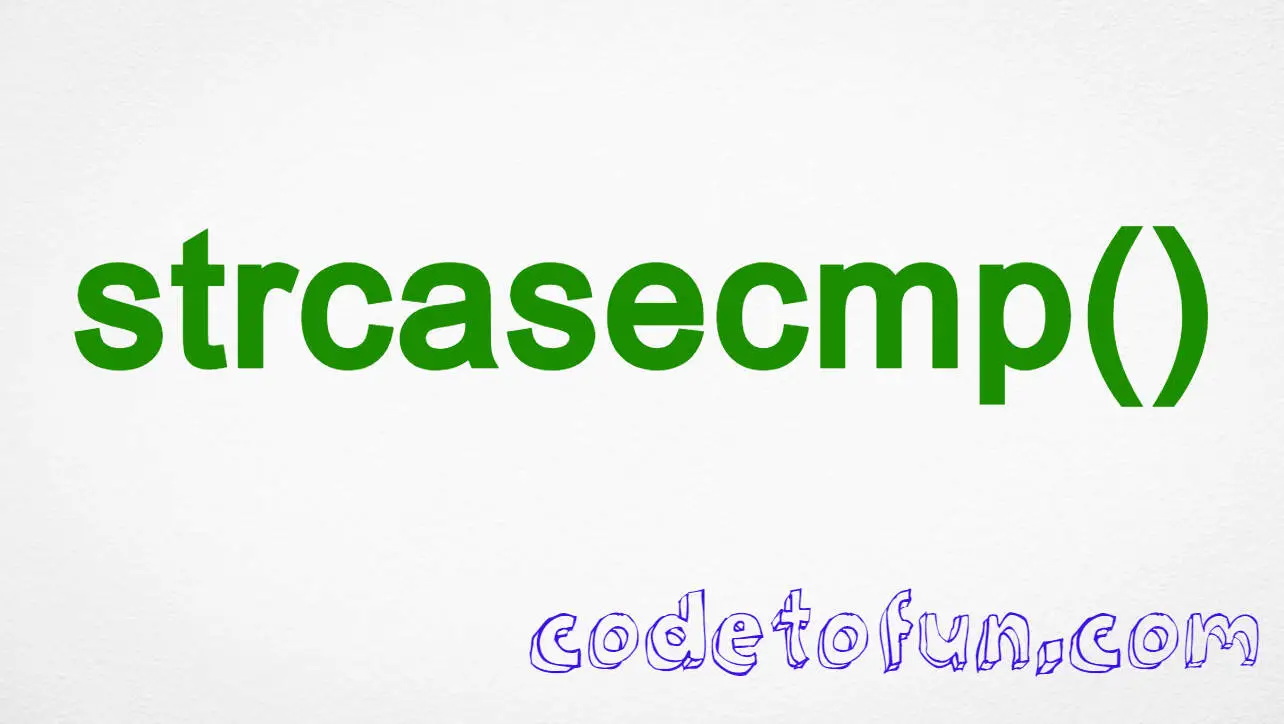
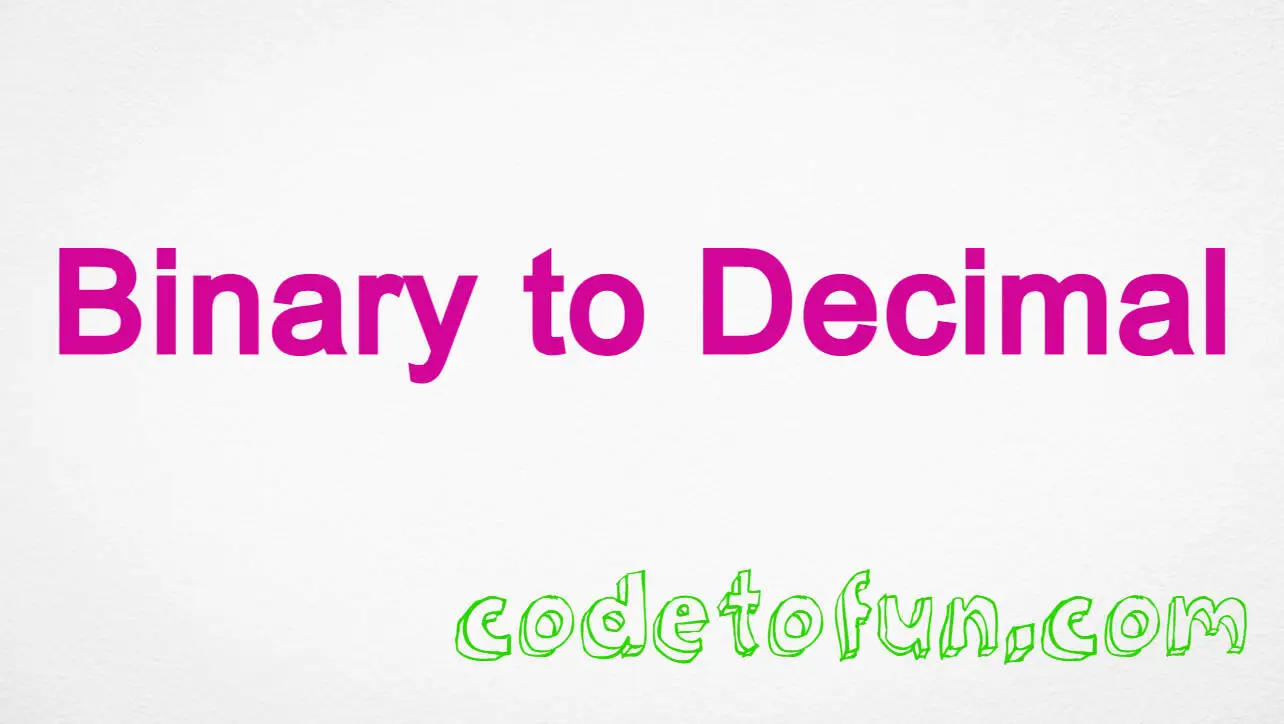
C Program to Converter a Binary to Decimal
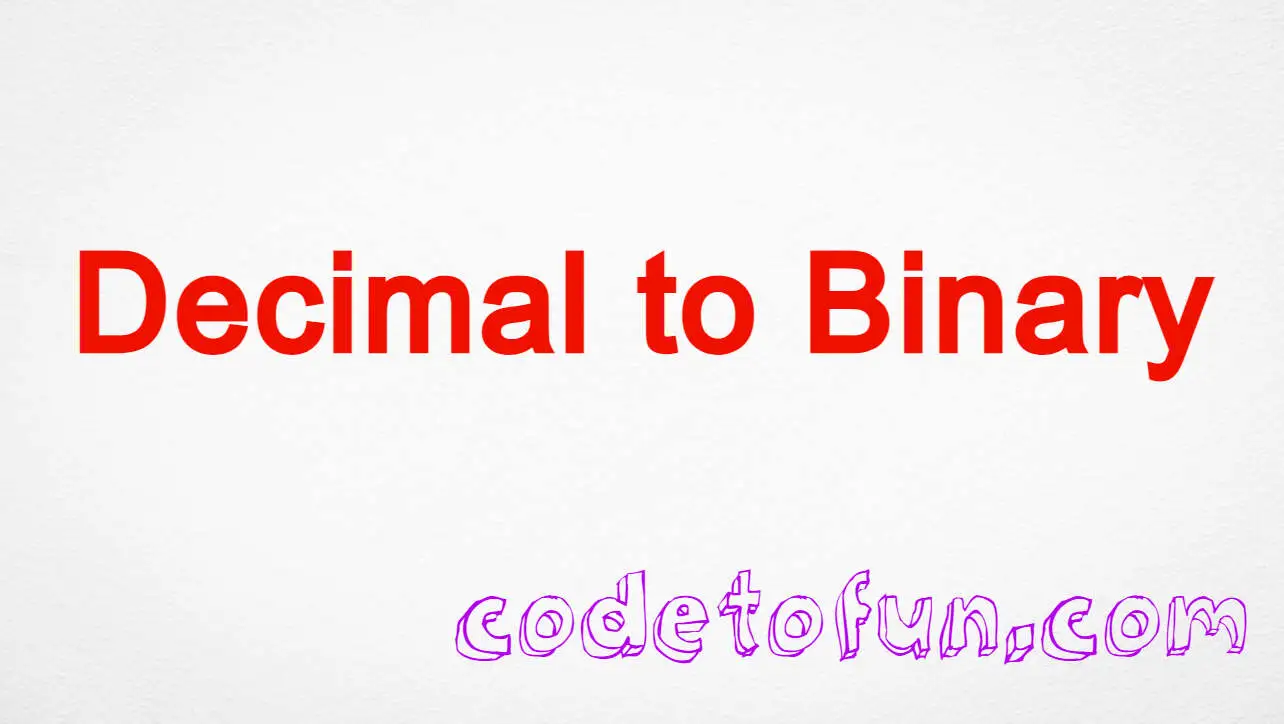
C Program to Converter a Decimal to Binary
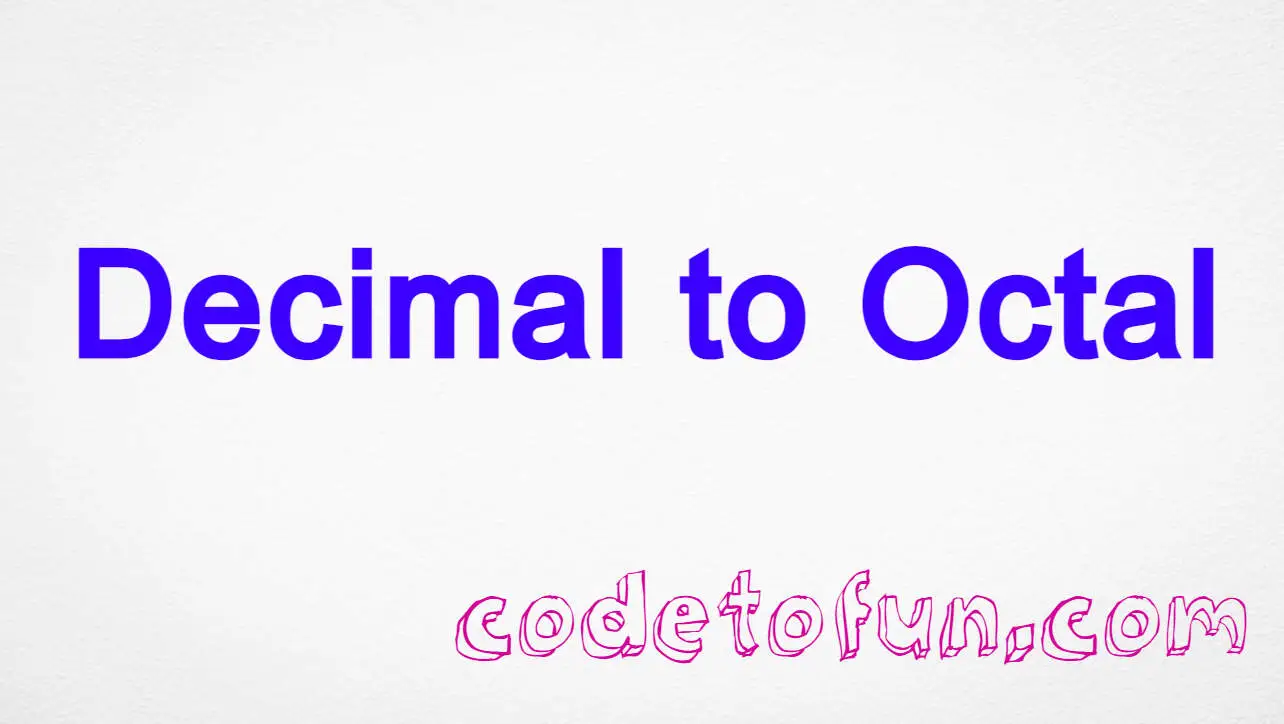
C Program to Converter a Decimal to Octal
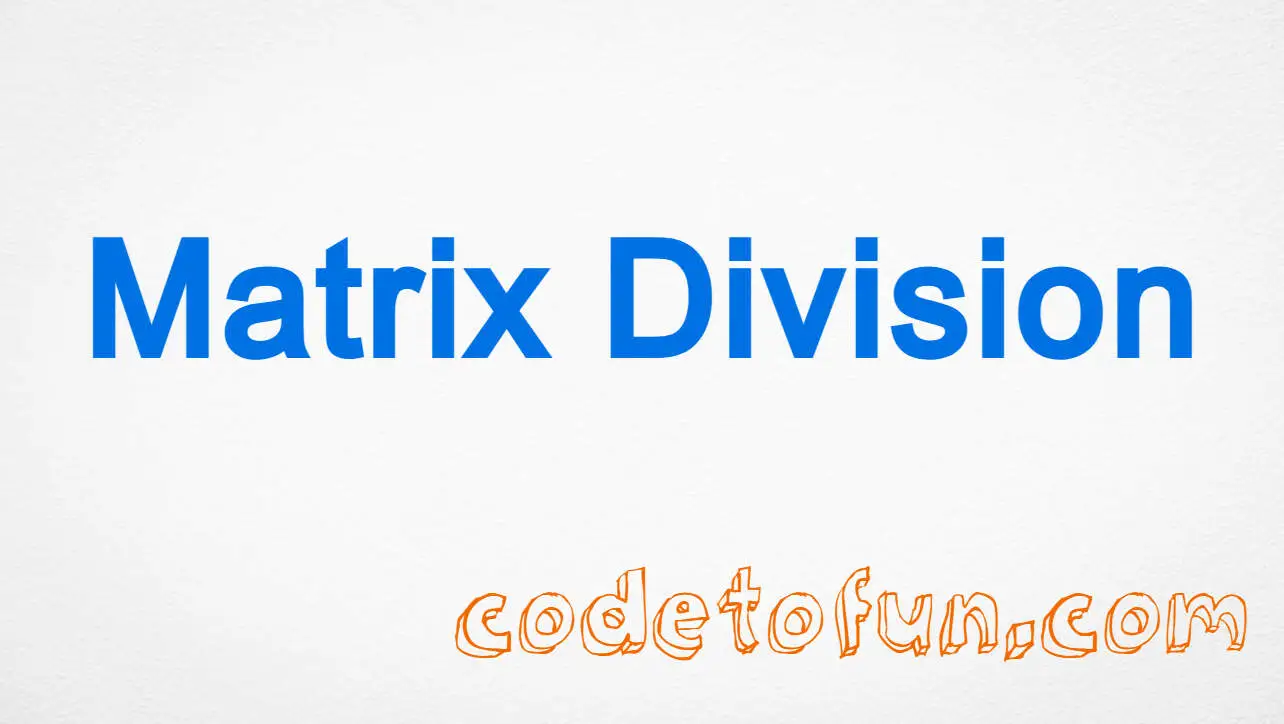
C Program to Perform Matrix Division
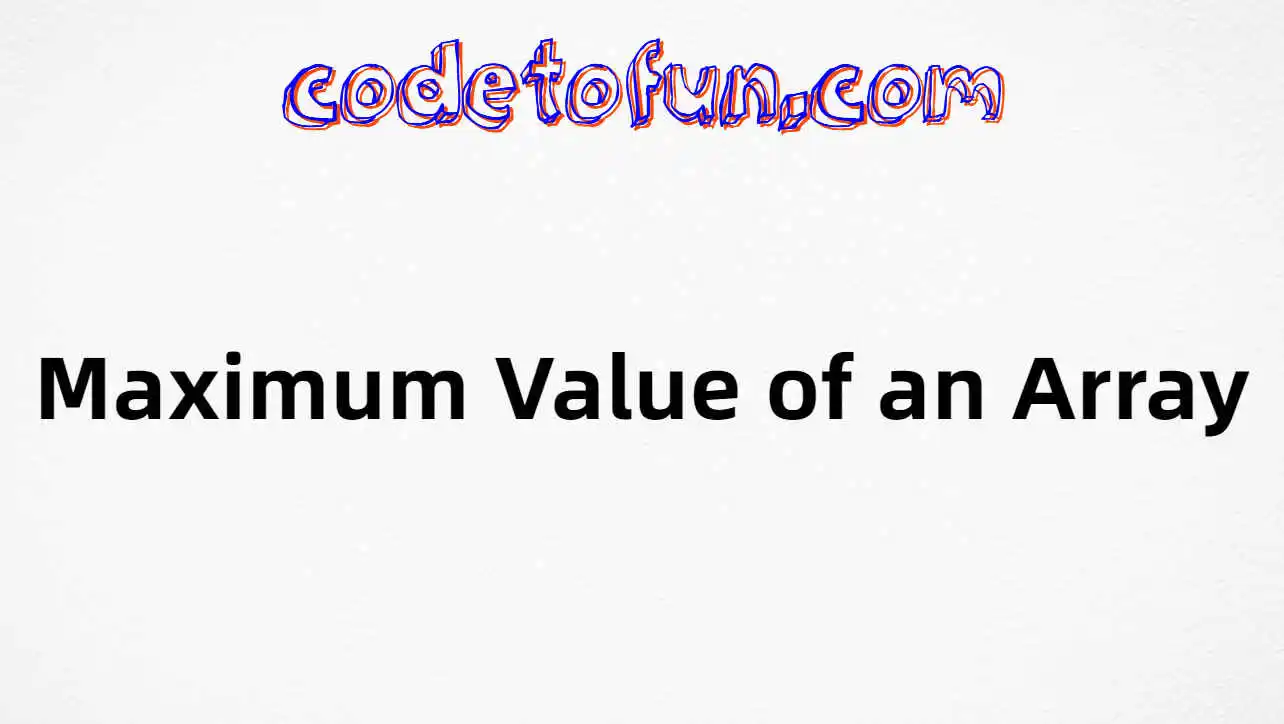
C Program to find Maximum Value of an Array
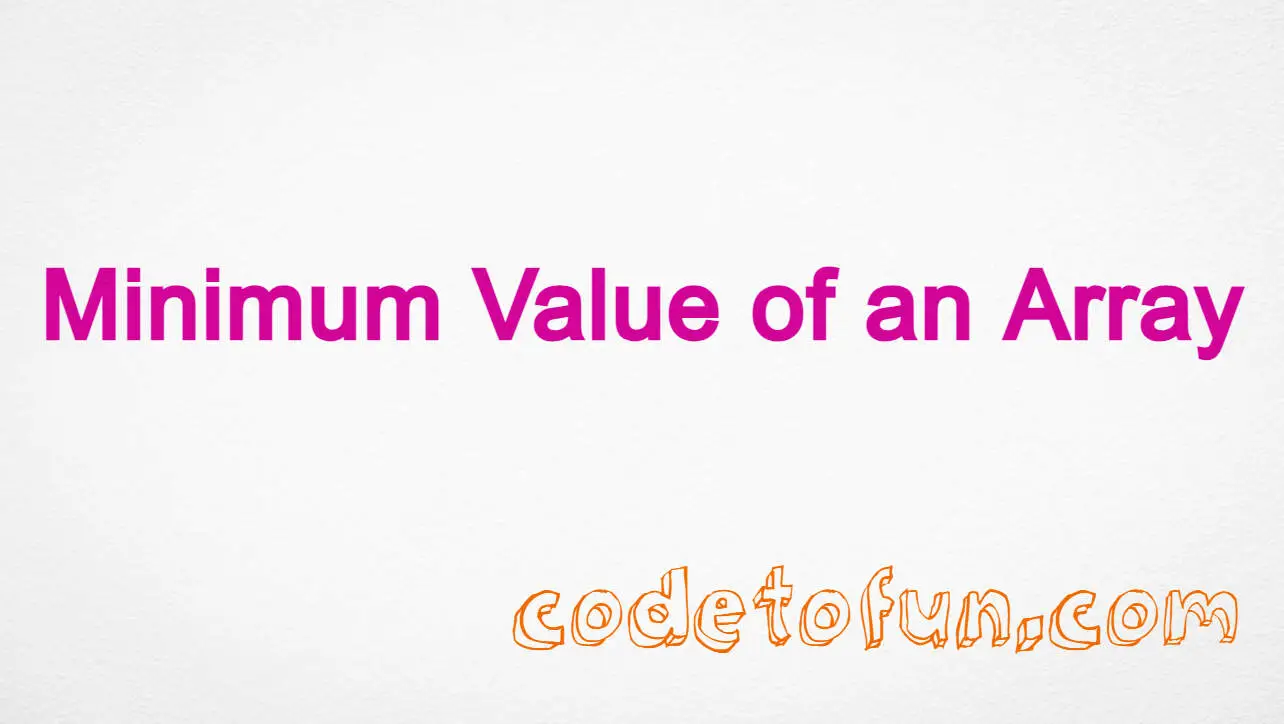
If you have any doubts regarding this article (C strcmp() Function) please comment here. I will help you immediately.