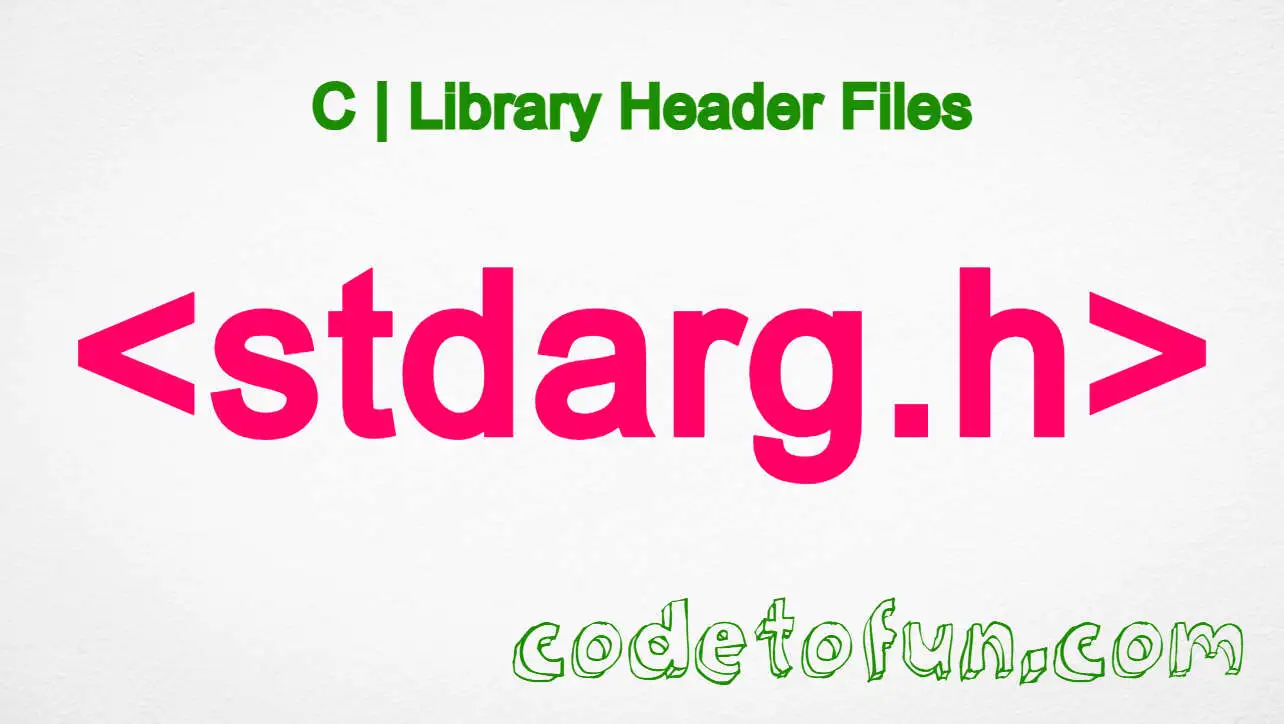
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Check Perfect Square
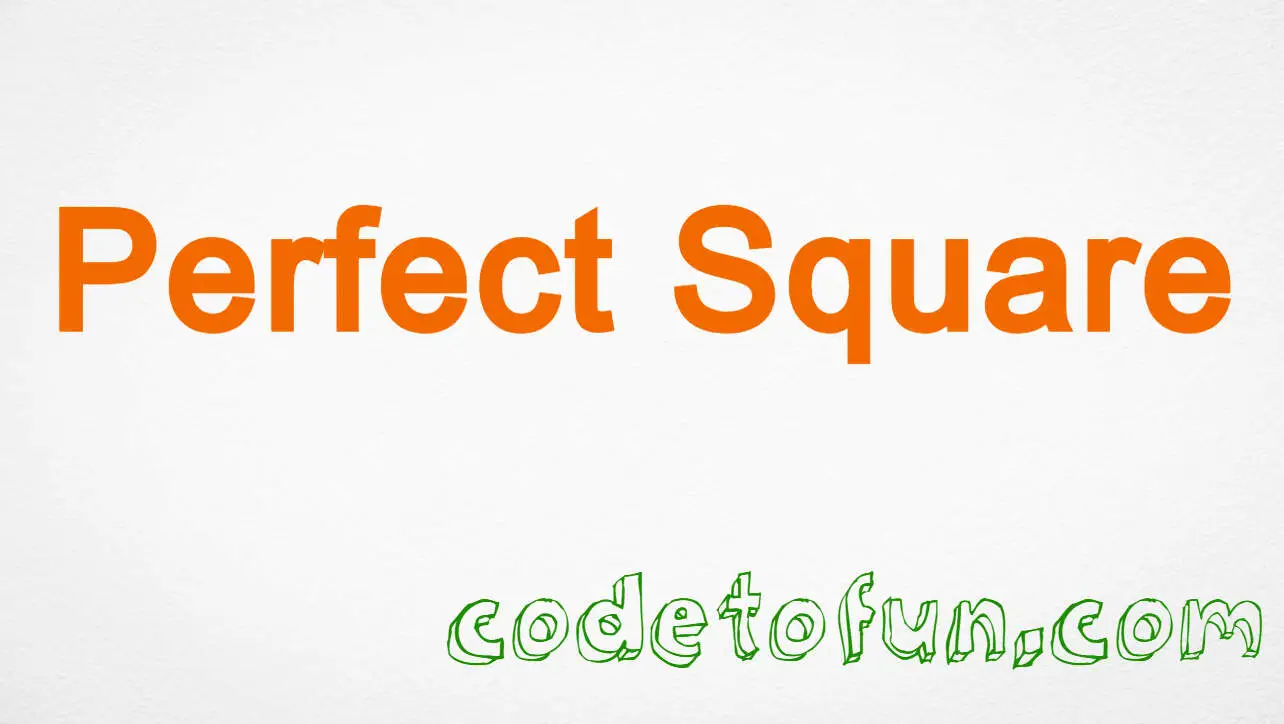
Photo Credit to CodeToFun
π Introduction
In the realm of programming, various mathematical problems challenge programmers to devise efficient solutions. One such problem is determining whether a given number is a perfect square.
A perfect square is a number that is the square of an integer, meaning it can be expressed as the product of an integer multiplied by itself.
In this tutorial, we'll delve into a C program designed to check whether a given number is a perfect square or not.
π Example
Let's explore the C code that accomplishes this task.
#include <stdio.h>
// Function to check if a number is a perfect square
int isPerfectSquare(int number) {
int i;
for (i = 1; i * i <= number; i++) {
// If i * i is equal to the number, it's a perfect square
if (i * i == number) {
return 1; // Return true
}
}
return 0; // Return false
}
// Driver program
int main() {
// Replace this value with your desired number
int testNumber = 16;
// Check if the number is a perfect square
if (isPerfectSquare(testNumber)) {
printf("%d is a perfect square.\n", testNumber);
} else {
printf("%d is not a perfect square.\n", testNumber);
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of testNumber in the main function.
16 is a perfect square.
Compile and run the program to see if the number is a perfect square.
π§ How the Program Works
- The program defines a function isPerfectSquare that takes an integer as input and returns 1 if the number is a perfect square, and 0 otherwise.
- Inside the function, it iterates through integers starting from 1 until the square of the current integer is greater than the given number.
- If the square of the current integer is equal to the given number, the function returns 1 indicating a perfect square.
- The main program then checks if a specific number (in this case, 16) is a perfect square and prints the result.
π Between the Given Range
Let's delve into the C code that accomplishes this task.
#include <stdio.h>
#include <math.h>
// Function to check if a number is a perfect square
int isPerfectSquare(int num) {
int root = sqrt(num);
return (root * root == num);
}
// Driver program
int main() {
printf("Perfect Squares in the Range 1 to 50:\n");
for (int i = 1; i <= 50; ++i) {
if (isPerfectSquare(i)) {
printf("%d ", i);
}
}
printf("\n");
return 0;
}
π» Testing the Program
The provided code checks for perfect squares in the range from 1 to 50. The output format is as follows:
Perfect Squares in the Range 1 to 50: 1 4 9 16 25 36 49
π§ How the Program Works
- The program defines a function isPerfectSquare that takes a number as input and returns whether it is a perfect square.
- Inside the main function, it iterates through numbers from 2 to 50 (excluding 1, as it is not considered a perfect square).
- For each number, it checks if it is a perfect square using the isPerfectSquare function.
- If a number is a perfect square, it is printed.
π§ Understanding the Concept of Perfect Square
Before diving into the code, let's take a moment to understand the concept of perfect squares.
A perfect square is a number that can be expressed as the product of an integer multiplied by itself.
For example, 4, 9, and 16 are perfect squares because they are the squares of 2, 3, and 4, respectively.
π’ Optimizing the Program
While the provided program is effective, there are alternative methods for checking perfect squares that involve mathematical properties. Consider exploring and implementing such optimizations to enhance the efficiency of your program.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
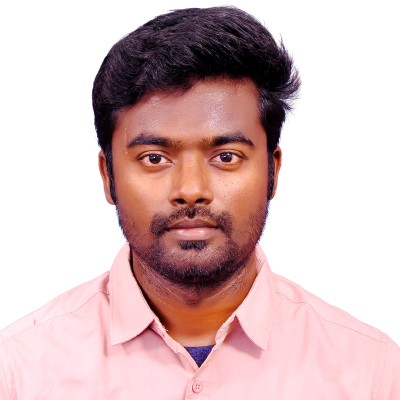
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Program to Check Perfect Square), please comment here. I will help you immediately.