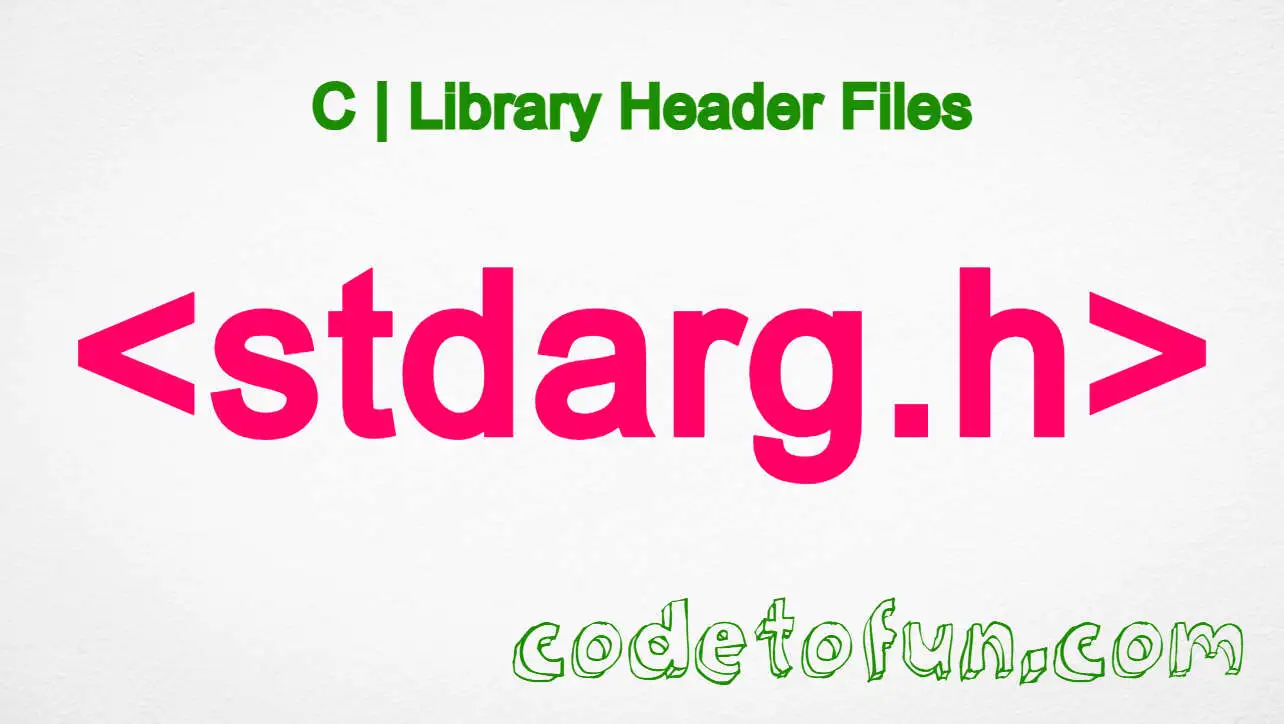
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Check Automorphic Number
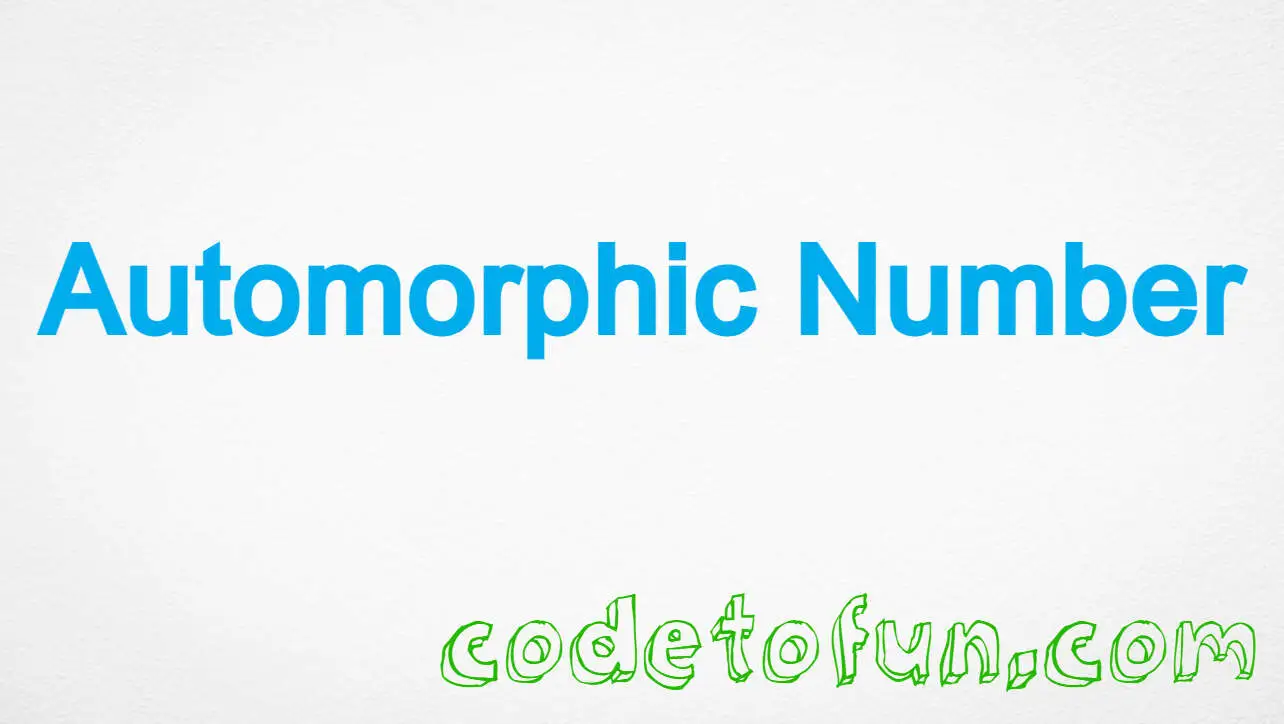
Photo Credit to CodeToFun
π Introduction
In the realm of C programming, exploring unique number properties is both fascinating and educational. An automorphic number is one such interesting concept.
An automorphic number (or circular number) is a number whose square ends with the number itself. In simpler terms, an n-digit number is called an automorphic number if the last n digits of its square are equal to the number itself.
In this tutorial, we'll delve into a C program that checks whether a given number is an automorphic number or not.
The program will take a number as input, square it, and then compare the last digits to determine if it meets the criteria of an automorphic number.
π Example
Let's explore the C code that accomplishes this task.
#include <stdio.h>
#include <math.h>
// Function to check if a number is automorphic
int isAutomorphic(int num) {
// Calculate the square of the number
long long square = num * (long long) num;
// Count the number of digits in the original number
int originalDigits = 0;
int temp = num;
while (temp != 0) {
temp /= 10;
originalDigits++;
}
// Extract the last digits from the square
long long lastDigits = square % (long long)(pow(10, originalDigits));
// Check if the last digits match the original number
return (int) lastDigits == num;
}
// Driver program
int main() {
// Replace this value with your desired number
int number = 76;
// Check if the number is automorphic
if (isAutomorphic(number)) {
printf("%d is an automorphic number.\n", number);
} else {
printf("%d is not an automorphic number.\n", number);
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the main function.
76 is an automorphic number.
Compile and run the program to check if the given number is an automorphic number.
π§ How the Program Works
- The program defines a function isAutomorphic that takes a number as input and returns whether it is an automorphic number or not.
- Inside the function, it calculates the square of the input number.
- It counts the number of digits in the original number to extract the corresponding number of last digits from the square.
- It compares the extracted last digits with the original number to determine if it's an automorphic number.
π Between the Given Range
Let's dive into the C code that checks for Automorphic Numbers in the range from 1 to 50.
#include <stdio.h>
// Function to check if a number is automorphic
int isAutomorphic(int number) {
long long square = number * (long long) number;
while (number > 0) {
if (number % 10 != square % 10) {
return 0; // Not automorphic
}
number /= 10;
square /= 10;
}
return 1; // Automorphic
}
// Driver program
int main() {
printf("Automorphic Numbers in the range 1 to 50:\n");
for (int i = 1; i <= 50; ++i) {
if (isAutomorphic(i)) {
printf("%d, ", i);
}
}
return 0;
}
π» Testing the Program
Automorphic Numbers in the range 1 to 50: 1, 5, 6, 25,
Run the program to check for automorphic numbers in the range from 1 to 50.
π§ How the Program Works
- The program defines a function isAutomorphic that checks if a given number is an automorphic number.
- Inside the function, it calculates the square of the number and compares the last digits of both the number and its square.
- The main function iterates through numbers from 1 to 50 and prints those that are automorphic.
π§ Understanding the Concept of Automorphic Numbers
Before delving into the code, let's take a moment to understand automorphic numbers.
An n-digit number is called an automorphic number if the last n digits of its square are equal to the number itself.
For example, 25 is an automorphic number because its square is 625, and the last two digits (25) match the original number.
π’ Optimizing the Program
While the provided program effectively checks for automorphic numbers, you can explore and implement further optimizations or variations based on your specific needs or preferences.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
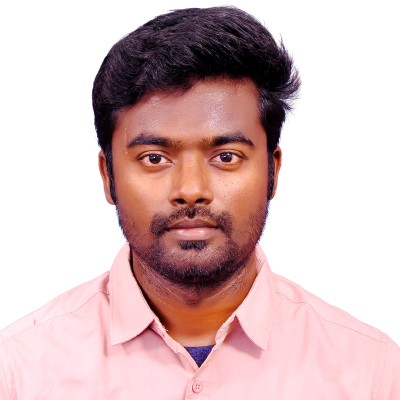
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Program to Check Automorphic Number), please comment here. I will help you immediately.