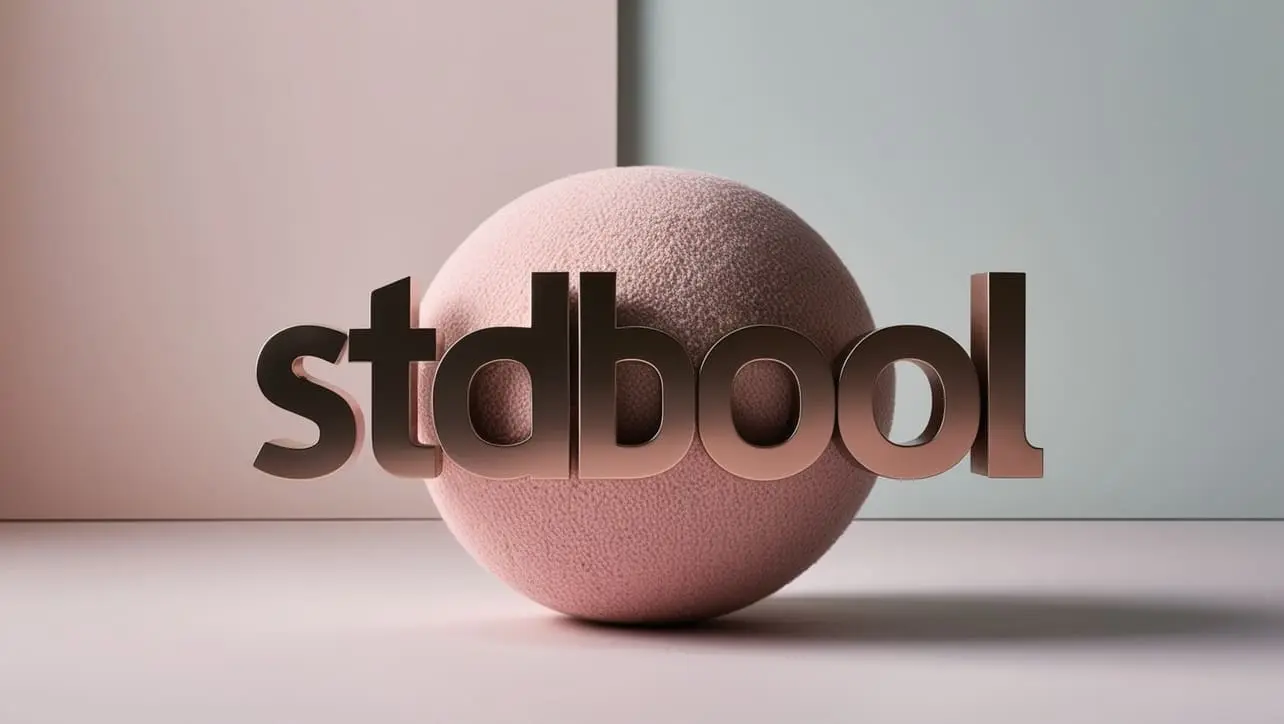
C strrev() Function
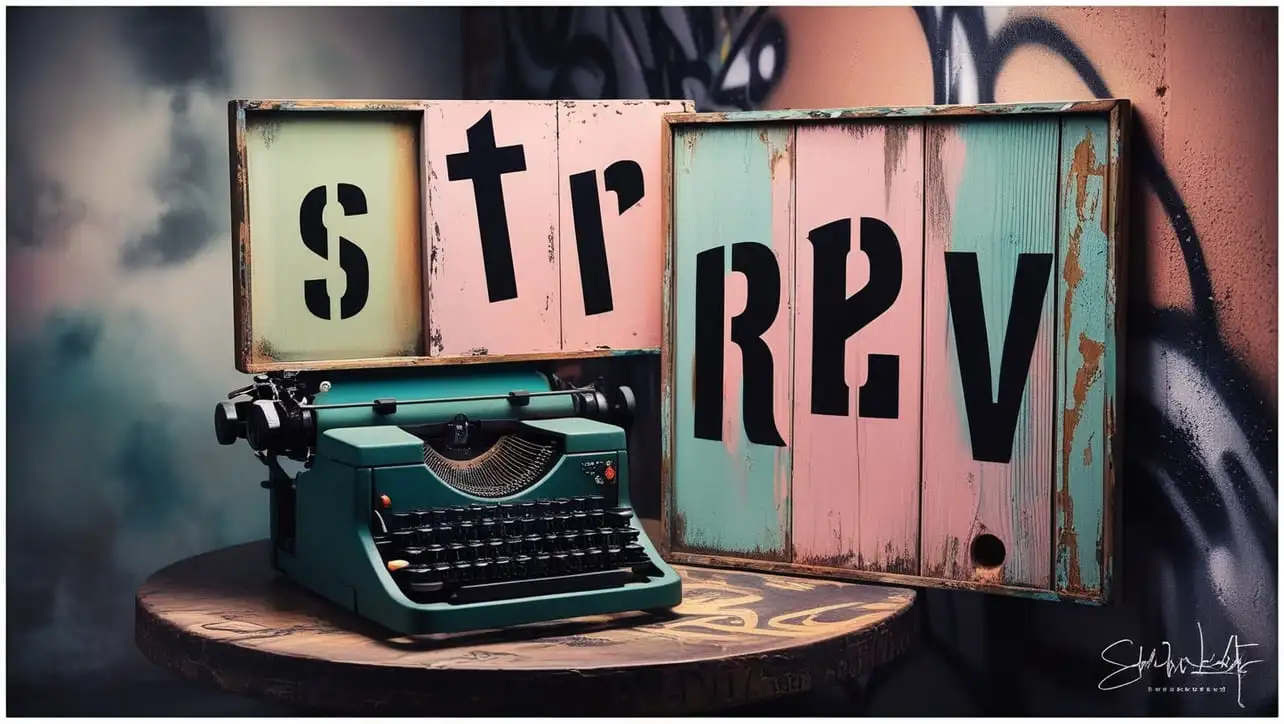
Photo Credit to CodeToFun
đ Introduction
In C programming, manipulating strings is a common task, and the strrev()
function is a useful tool for reversing a string.
The strrev()
function is not a standard C library function, but it is available in many C compilers as an extension.
In this tutorial, we'll explore the usage and functionality of the strrev()
function in C.
đĄ Syntax
The prototype for the strrev()
function is generally as follows:
char* strrev(char* str);
- str: A pointer to the null-terminated string to be reversed.
đ Example 1
Let's dive into an example to illustrate how the strrev()
function works.
Warning: The strrev()
function is not a standard C library function, and its availability might vary across different compilers.
#include <stdio.h>
#include <string.h>
int main() {
char original[] = "Hello, C!";
// Reverse the string
char * reversed = strrev(original);
// Output the reversed string
printf("Original: %s\nReversed: %s\n", original, reversed);
return 0;
}
đģ Output
Original: Hello, C! Reversed: !C ,olleH
đ§ How the Program Works
In this example, the strrev()
function is used to reverse the string "Hello, C!" and then prints both the original and reversed strings.
đ Example 2
Here's an alternative example using a loop to reverses it in place using a two-pointer approach.
#include <stdio.h>
#include <string.h>
// Function to reverse a string
void reverseString(char * str) {
int length = strlen(str);
int start = 0;
int end = length - 1;
while (start < end) {
// Swap characters at start and end indices
char temp = str[start];
str[start] = str[end];
str[end] = temp;
// Move indices towards the center
++start;
--end;
}
}
int main() {
char original[] = "Hello, C!";
// Reverse the string
reverseString(original);
// Output the reversed string
printf("Original: %s\nReversed: %s\n", "Hello, C!", original);
return 0;
}
đģ Output
Original: Hello, C! Reversed: !C ,olleH
đ§ How the Program Works
In this program, the reverseString() function takes a character array as input and reverses it in place using a two-pointer approach. The start pointer begins at the first character, and the end pointer begins at the last character. In each iteration, the characters at the start and end indices are swapped, and the pointers are moved towards the center until they meet.
âŠī¸ Return Value
The strrev()
function typically returns a pointer to the reversed string. Note that some compilers might not provide a return value for this function, and it directly modifies the input string.
đ Common Use Cases
The strrev()
function is particularly useful when you need to reverse a string for tasks such as palindrome checking or displaying data in reverse order.
đ Notes
- As mentioned earlier, the
strrev()
function is not part of the standard C library, so its availability depends on the compiler being used. - If the function is not available, you can implement your own string reversal function using a loop or recursion.
đĸ Optimization
The strrev()
function, if available, is generally optimized for efficiency. However, for custom implementations, consider the complexity of the reversal algorithm, especially for large strings.
đ Conclusion
The strrev()
function in C provides a straightforward way to reverse a string. While it's not part of the standard C library, it's commonly available as a compiler extension, making it a convenient tool for string manipulation tasks.
Feel free to experiment with different strings and explore the behavior of the strrev()
function. If the function is not available, consider implementing your own string reversal logic. Happy coding!
đ¨âđģ Join our Community:
Author
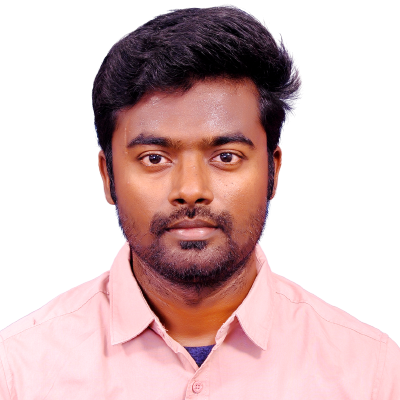
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C strrev() Function) please comment here. I will help you immediately.