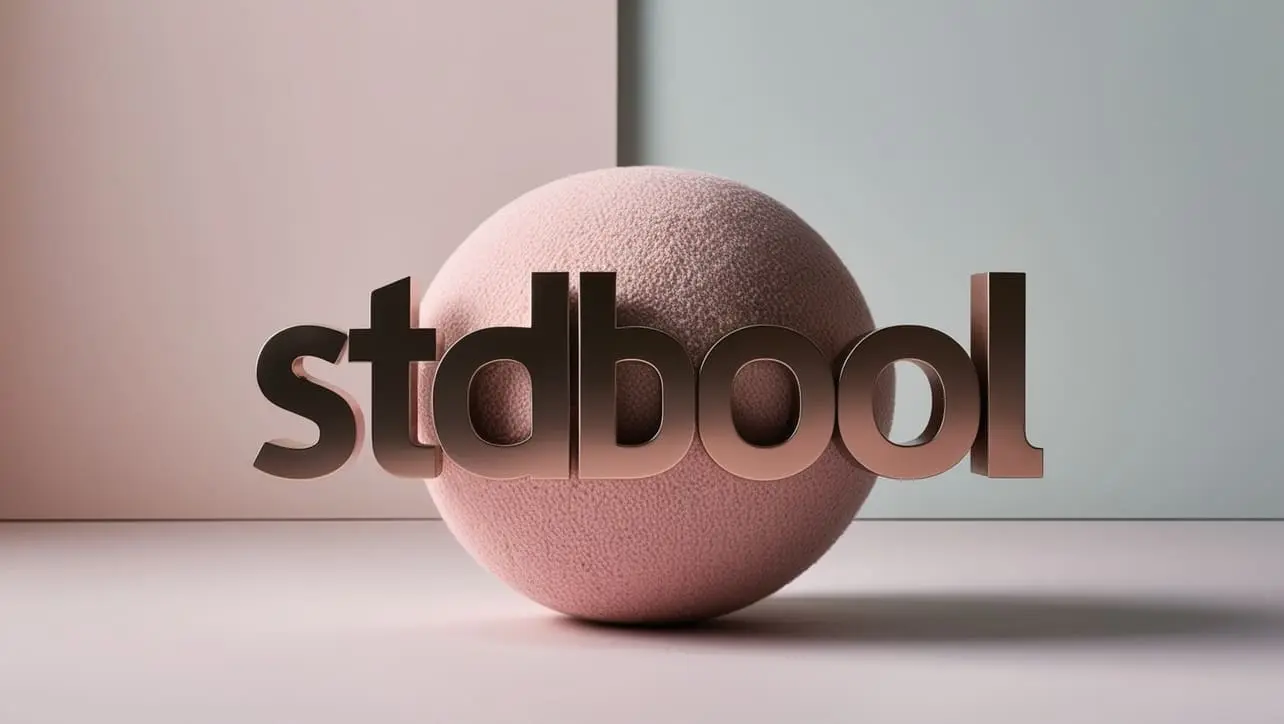
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strupr() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, strings are an essential data type, and various functions are available for string manipulation.
The strupr()
function is one such function that converts all the characters in a string to uppercase.
In this tutorial, we'll explore the usage and functionality of the strupr()
function in C.
đĄ Syntax
The syntax for the strupr()
function is as follows:
char *strupr(char *str);
- str: A pointer to the null-terminated string whose characters will be converted to uppercase.
đ Example 1
Let's dive into an example to illustrate how the strupr()
function works.
Warning: The strupr()
function is not part of the standard C library and is not supported by all compilers.
#include <stdio.h>
#include <string.h>
int main() {
char originalString[] = "Hello, C!";
// Convert the string to uppercase
char * uppercaseString = strupr(originalString);
// Output the result
printf("Original String: %s\n", originalString);
printf("Uppercase String: %s\n", uppercaseString);
return 0;
}
đģ Output
Original String: Hello, C! Uppercase String: HELLO, C!
đ§ How the Program Works
In this example, the strupr()
function is used to convert the string "Hello, C!" to uppercase, and the result is printed.
đ Example 2
In this example, we will consider using an alternative approach or implementing your own function to convert a string to uppercase. Here's an example using a simple function:
#include <stdio.h>
#include <ctype.h>
void stringToUpper(char * str) {
while ( * str) {
* str = toupper((unsigned char) * str);
str++;
}
}
int main() {
char originalString[] = "Hello, C!";
printf("Original String: %s\n", originalString);
// Convert the string to uppercase
stringToUpper(originalString);
// Output the result
printf("Uppercase String: %s\n", originalString);
return 0;
}
đģ Output
Original String: Hello, C! Uppercase String: HELLO, C!
đ§ How the Program Works
In this example, the stringToUpper() function is used to convert the string to uppercase. It iterates through each character in the string and uses the toupper() function from the ctype.h header to convert it to uppercase.
This approach is standard and should work across different compilers without causing warnings about implicit declarations.
âŠī¸ Return Value
The strupr()
function returns a pointer to the modified string, which is the same as the input string. The modification is done in place.
đ Common Use Cases
The strupr()
function is useful when you need to convert a string to uppercase for comparison or display purposes. It is commonly employed in scenarios where case-insensitive string operations are required.
đ Notes
- The
strupr()
function is not a standard C library function and may not be available on all platforms. It is often provided as an extension in some compilers. - If portability is a concern, consider using alternative methods, such as manually iterating through the characters of the string and converting them to uppercase.
đĸ Optimization
The strupr()
function itself is a straightforward operation, and optimization is typically not a primary concern. However, consider the availability of this function on your target platform.
đ Conclusion
The strupr()
function in C is a convenient tool for converting a string to uppercase. It simplifies the process of case manipulation and is valuable in situations where uppercase consistency is essential.
Feel free to experiment with different strings and explore the behavior of the strupr()
function. Keep in mind the portability considerations when using this function in your programs. Happy coding!
đ¨âđģ Join our Community:
Author
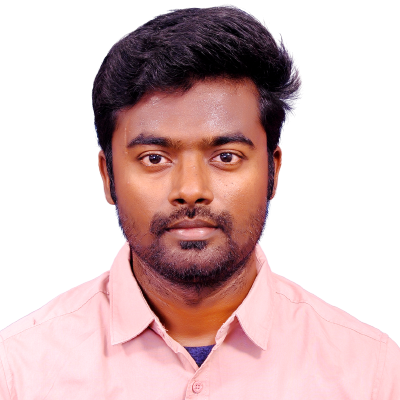
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C strupr() Function) please comment here. I will help you immediately.