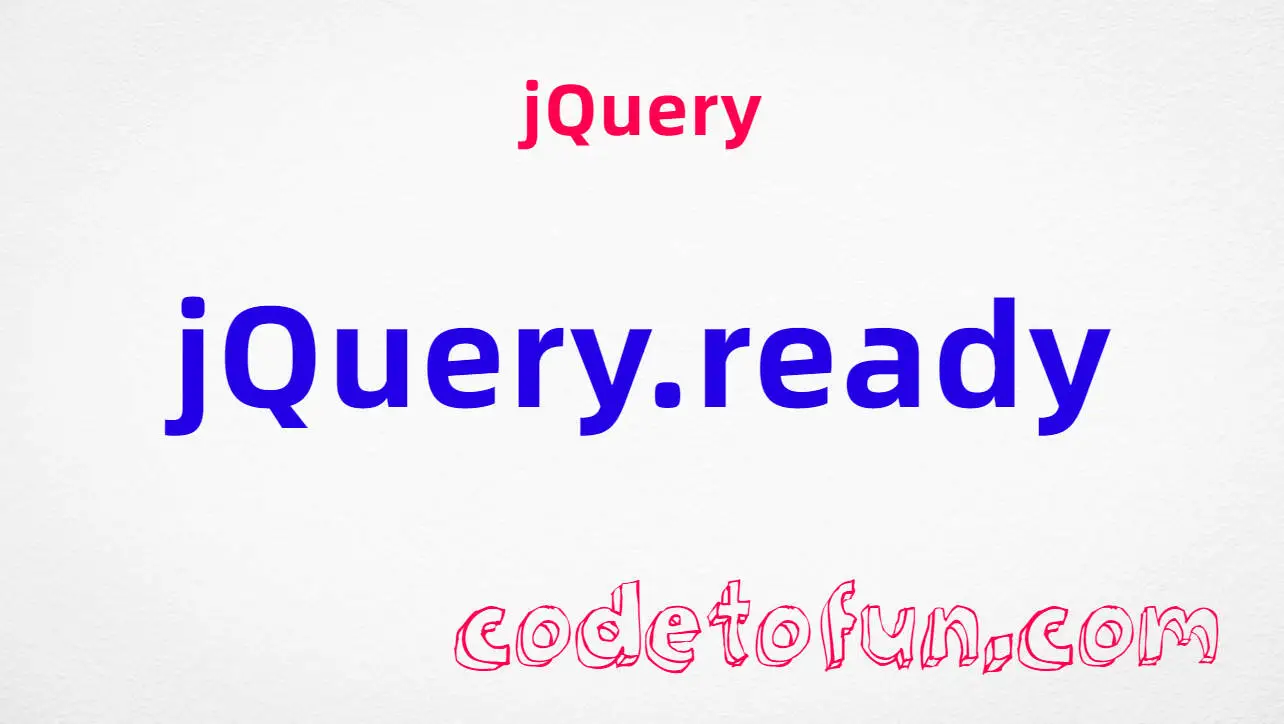
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery jQuery.speed Property

Photo Credit to CodeToFun
π Introduction
jQuery is known for its simplicity and power in handling a wide range of web development tasks, including animations. One of the key features that make jQuery animations versatile is the .speed
property. This property allows developers to control the duration and easing of animations, making it easier to create smooth and visually appealing effects.
In this guide, we'll explore the jQuery .speed
property, its syntax, and practical examples to help you harness its full potential.
π§ Understanding jQuery.speed Property
The .speed
property in jQuery is used internally to manage animation speeds. Although it is not a direct method you call, understanding how to control animation speed using the .animate(), .fadeIn(), .fadeOut(), and other similar methods is crucial for effective animations.
π‘ Syntax
When using jQuery methods that involve animations, such as .animate(), .fadeIn(), or .slideUp(), you can specify the speed in milliseconds or by using predefined strings like "slow"
, "fast"
, or a numeric value for precise control.
$(selector).animate(properties, speed, easing, callback);
π Example
Basic Animation Speed:
Using the .animate() method, you can control the speed of an animation. Hereβs an example where we animate a div to move 200px to the right over 1000 milliseconds (1 second):
index.htmlCopied<div id="box" style="width:100px;height:100px;background-color:blue;position:relative;"></div> <button id="animateButton">Animate</button>
example.jsCopied$("#animateButton").click(function() { $("#box").animate({ left: "200px" }, 1000); });
This will move the div to the right over 1 second when the button is clicked.
Using Predefined Speeds:
jQuery also allows using predefined speed strings for convenience. These are slow (600ms) and fast (200ms):
index.htmlCopied<button id="slowButton">Slow Animation</button> <button id="fastButton">Fast Animation</button>
example.jsCopied$("#slowButton").click(function() { $("#box").animate({ left: "200px" }, "slow"); }); $("#fastButton").click(function() { $("#box").animate({ left: "200px" }, "fast"); });
Clicking these buttons will move the div at the predefined speeds.
Combining Speed with Easing:
Easing functions define the acceleration pattern of an animation. jQuery supports swing (default) and linear easing. Hereβs an example using both:
index.htmlCopied<button id="swingButton">Swing Easing</button> <button id="linearButton">Linear Easing</button>
example.jsCopied$("#swingButton").click(function() { $("#box").animate({ left: "200px" }, 1000, "swing"); }); $("#linearButton").click(function() { $("#box").animate({ left: "200px" }, 1000, "linear"); });
The div will move with a swing effect (start slow, speed up, then slow down) or a linear effect (constant speed).
Callback Functions:
You can also execute a function after the animation completes by providing a callback function:
index.htmlCopied<button id="callbackButton">Animate with Callback</button>
example.jsCopied$("#callbackButton").click(function() { $("#box").animate({ left: "200px" }, 1000, function() { alert("Animation Complete!"); }); });
This will alert "Animation Complete!" after the div has finished moving.
π Conclusion
Understanding and utilizing the jQuery .speed
property within animation methods is essential for creating smooth and effective animations on your web pages. By mastering the control over animation speed, easing, and callbacks, you can enhance user experiences and add a professional touch to your website.
Use these techniques to bring your web elements to life with dynamic and responsive animations.
π¨βπ» Join our Community:
Author
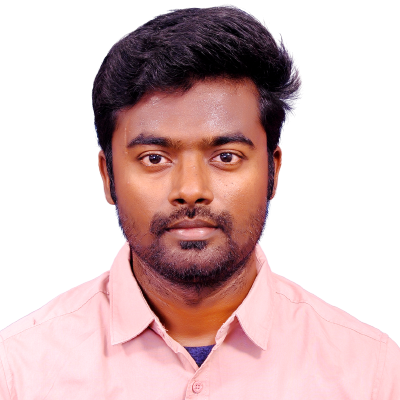
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.speed Property), please comment here. I will help you immediately.