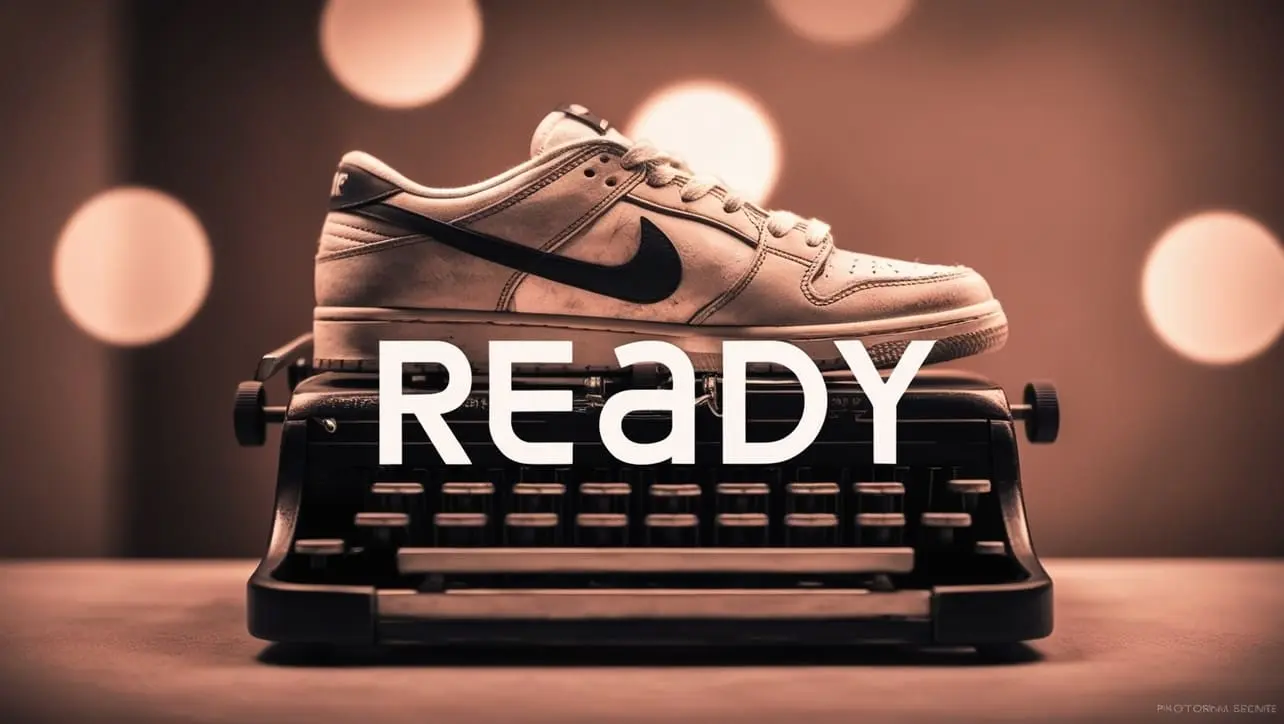
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery unload Event
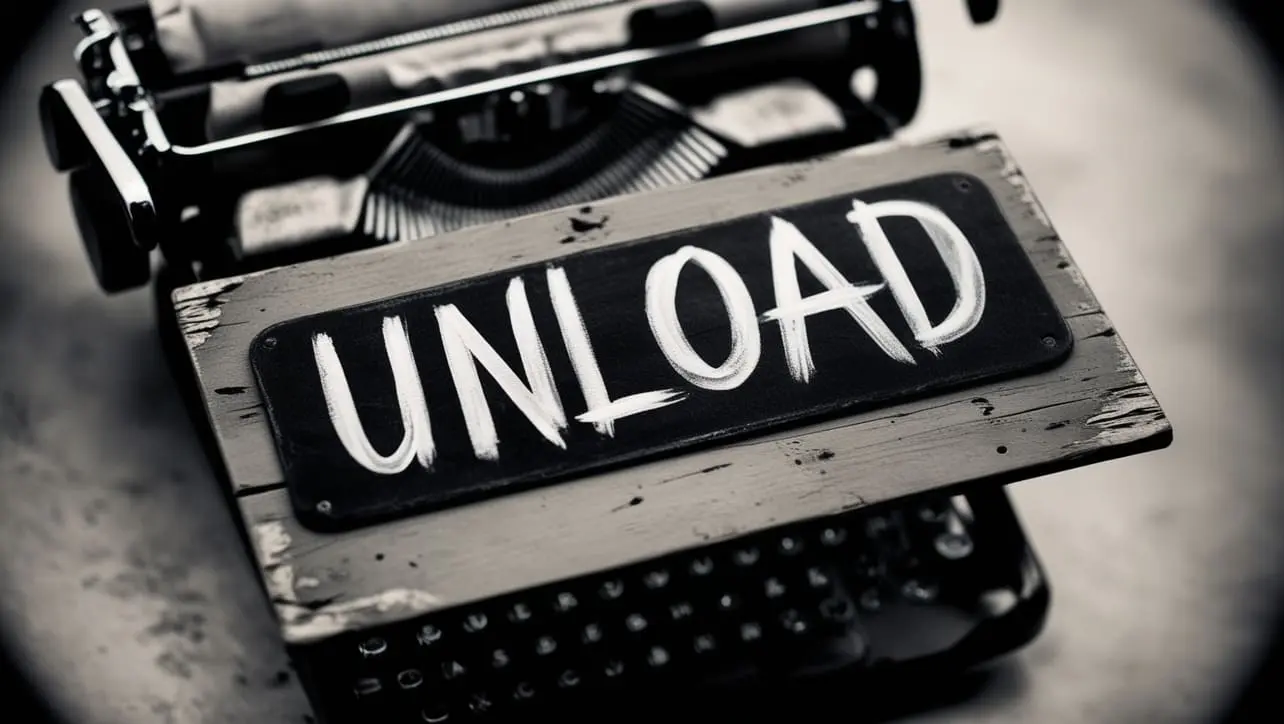
Photo Credit to CodeToFun
Introduction
In web development, managing events related to page unloading is crucial for ensuring a smooth user experience and handling cleanup tasks. jQuery provides an event handler specifically designed for this purpose called the unload
event. Although .unload() has been deprecated, jQuery recommends using .on() instead for event binding.
In this guide, we'll explore the unload
event in jQuery, its syntax using .on(), and how it can be effectively utilized in your web projects.
Understanding unload Event
The unload
event in jQuery is triggered when the user navigates away from the current page or refreshes it. It provides an opportunity to execute cleanup tasks, save user data, or perform any necessary actions before the page unloads.
Syntax
The syntax for the unload
event is straightforward:
$(window).on("unload", function() {
// Handler function
});
The .on() method is used to bind an event handler to the unload
event.
Example
Saving User Data on Page Unload:
Suppose you want to save some user data when they leave your page. You can achieve this using the
unload
event as follows:example.jsCopied$(window).on("unload", function() { // Save user data to server or local storage console.log("Saving user data before page unload..."); });
This will log a message to the console when the user navigates away from the page.
Confirming Page Unload:
You can prompt the user with a confirmation dialog before they leave the page:
example.jsCopied$(window).on("unload", function() { return "Are you sure you want to leave this page?"; });
This will display a confirmation dialog with the specified message when the user tries to leave the page.
Performing Cleanup Tasks:
Cleanup tasks such as closing connections or clearing resources can also be executed using the
unload
event:example.jsCopied$(window).on("unload", function() { // Close database connections, clean up resources, etc. console.log("Performing cleanup tasks before page unload..."); });
Conclusion
The jQuery unload
event, although deprecated in favor of .on(), remains a valuable tool for managing page unloading events in web development. Whether you need to save user data, prompt for confirmation, or perform cleanup tasks, the unload
event provides a reliable mechanism to handle such scenarios.
By leveraging this event effectively, you can enhance the user experience and ensure proper management of resources in your web applications.
Join our Community:
Author
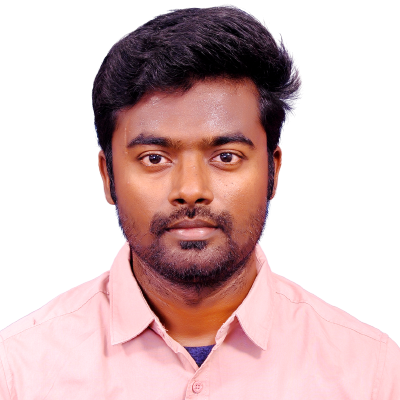
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery unload Event), please comment here. I will help you immediately.