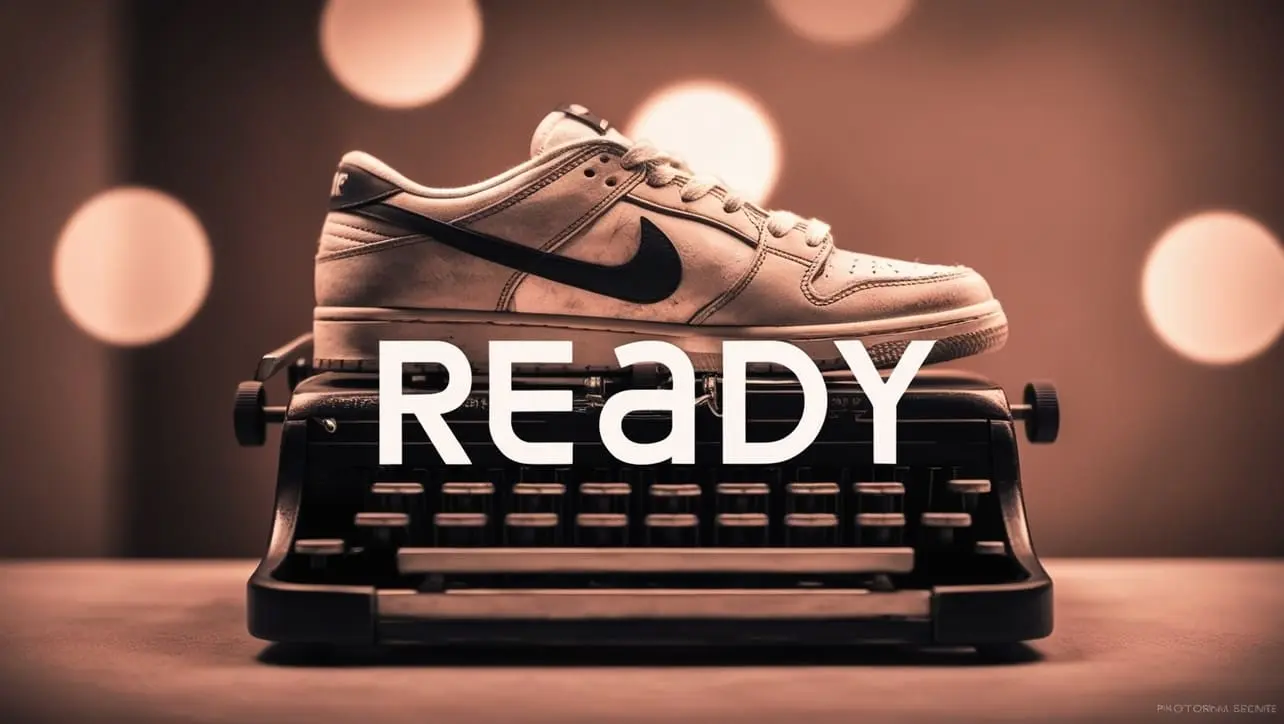
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery mouseout Event
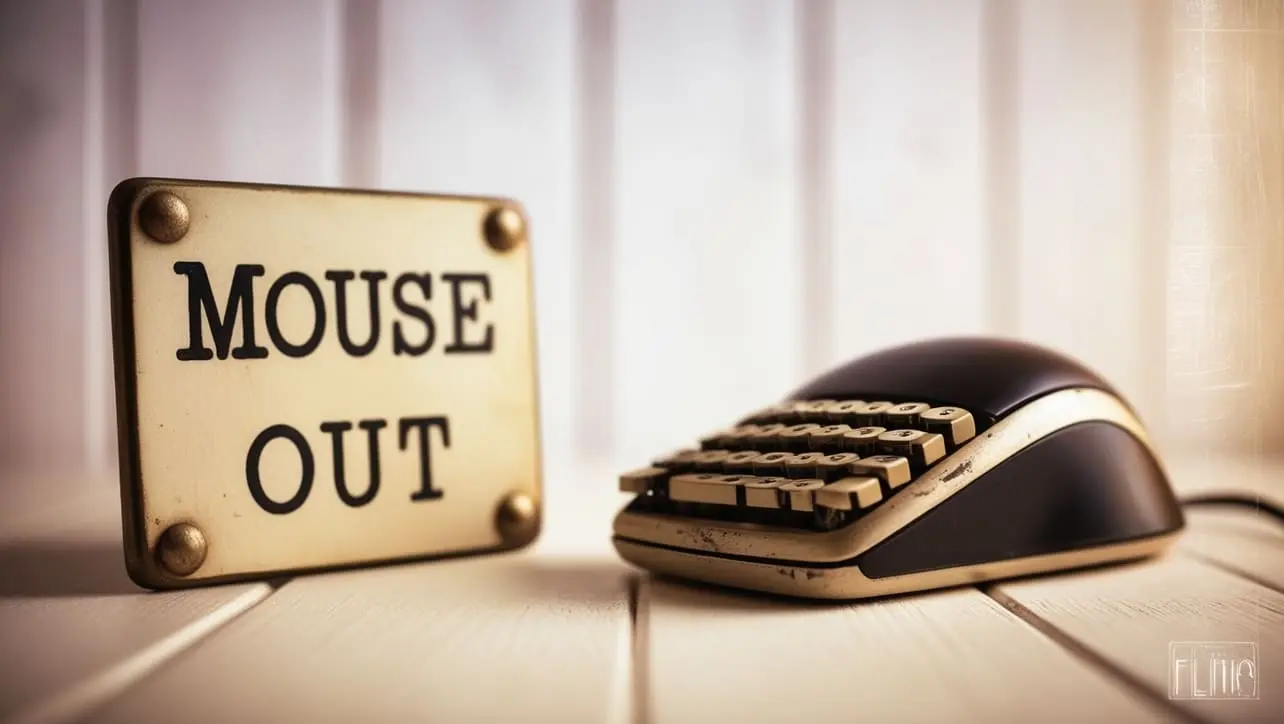
Photo Credit to CodeToFun
Introduction
jQuery offers a rich set of event handling methods to make web development more interactive and responsive. Among these, the mouseout
event stands out for its ability to trigger actions when the mouse pointer leaves an element. However, it's essential to note that the traditional .mouseout() method is now deprecated in favor of .on().
In this guide, we'll explore how to utilize the .on() method to harness the mouseout
event effectively.
Understanding mouseout Event
The .on() method in jQuery is a versatile tool for attaching event handlers to elements. It allows you to specify the event type, optional event data, and the handler function. When used with the mouseout
event, it enables you to execute code when the mouse pointer moves out of an element.
Syntax
The syntax for the mouseout
event is straightforward:
.on("mouseout", [, eventData ], handler)
Example
Basic Usage of .on() with mouseout:
Let's start with a simple example where we display an alert message when the mouse pointer leaves a <div> element.
index.htmlCopied<div id="target">Hover over me</div>
example.jsCopied$("#target").on("mouseout", function() { alert("Mouse pointer left the element!"); });
In this case, whenever the mouse pointer moves out of the <div> with the ID target, an alert message will pop up.
Using Event Data with .on():
You can pass additional data to the event handler function using the .on() method. Here's an example where we log the mouse coordinates when the pointer leaves a <div> element.
index.htmlCopied<div id="target">Hover over me</div>
example.jsCopied$("#target").on("mouseout", {x: 0, y: 0}, function(event) { console.log("Mouse pointer left at: " + event.pageX + ", " + event.pageY); });
This will log the coordinates of the mouse pointer when it leaves the <div> element.
Event Delegation with .on():
Event delegation is a powerful technique for handling events on dynamically added elements. With .on(), you can delegate the
mouseout
event to a parent element. Here's how:index.htmlCopied<ul id="parent"> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul>
example.jsCopied$("#parent").on("mouseout", "li", function() { console.log("Mouse pointer left an <li> element."); });
In this example, the
mouseout
event is delegated to the <ul> element with the ID parent, and the handler function is triggered when the mouse pointer leaves an <li> element within it.
Conclusion
By leveraging the .on() method in jQuery, you can effectively utilize the mouseout
event to create interactive and responsive web experiences. Whether you're triggering actions, passing event data, or employing event delegation, the .on() method provides a flexible and intuitive way to handle mouseout
events.
Keep experimenting with these techniques to enhance the interactivity of your web pages.
Join our Community:
Author
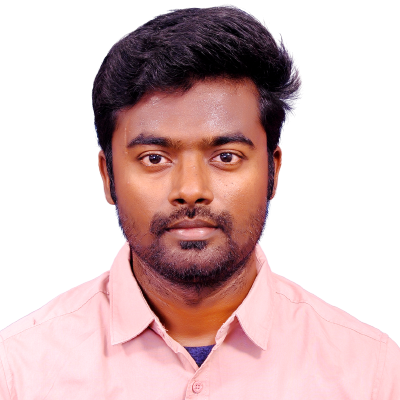
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery mouseout Event), please comment here. I will help you immediately.