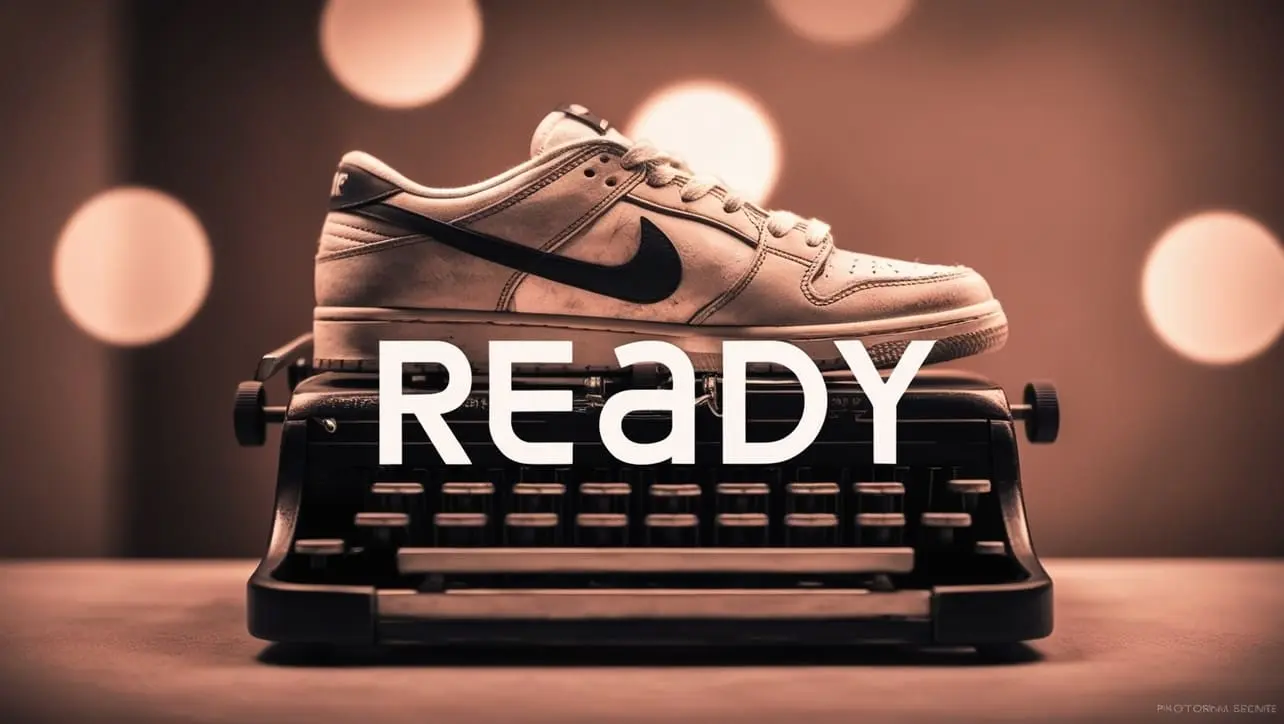
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery mouseleave Event
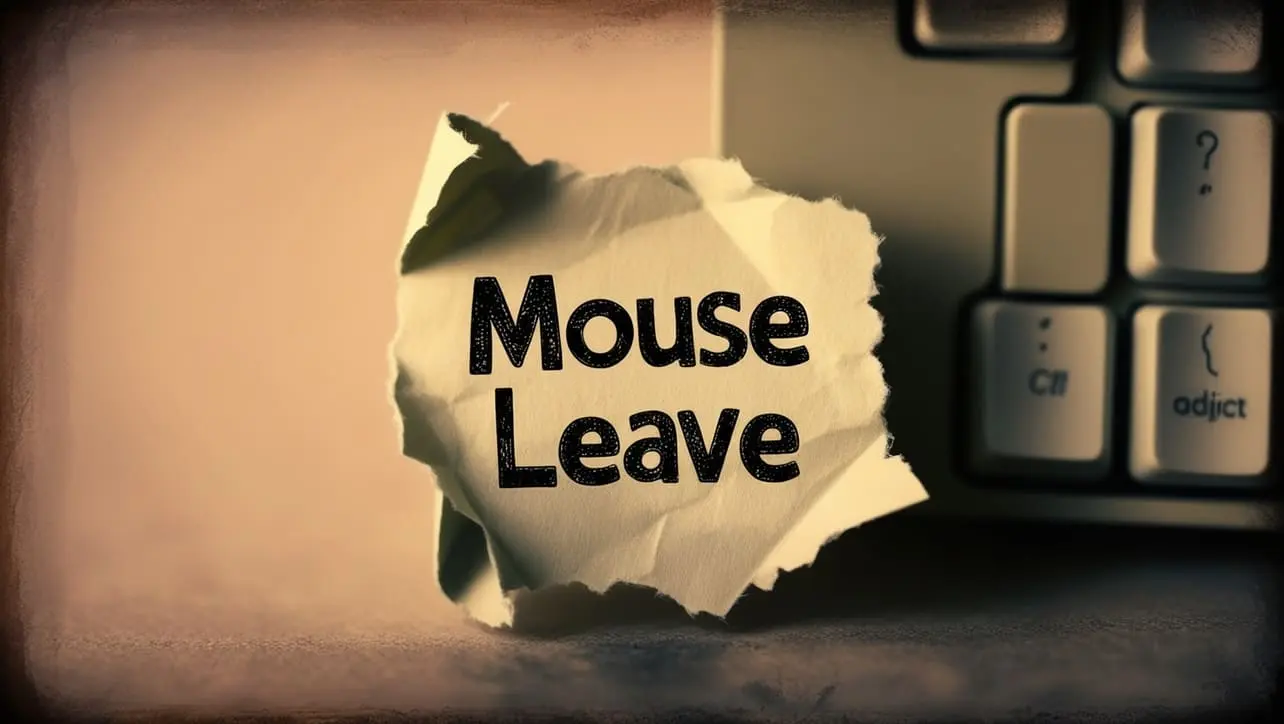
Photo Credit to CodeToFun
Introduction
In jQuery, event handling is crucial for creating dynamic and interactive web pages. One commonly used event is mouseleave, which occurs when the mouse pointer leaves an element. Although the .mouseleave() method has been deprecated, jQuery provides a powerful alternative - the .on() method.
This guide explores how to use the .on() method to handle the mouseleave
event effectively.
Understanding mouseleave Event
The .on() method allows you to attach event handlers to elements, including the mouseleave
event. Its syntax for handling mouseleave is as follows:
Syntax
The syntax for the mouseleave
event is straightforward:
.on("mouseleave", [, eventData ], handler)
Syntax Breakdown:
- mouseleave: Specifies the event to listen for, in this case, the mouse leaving the element.
- [eventData] (optional): Additional data to pass to the event handler.
- handler: The function to execute when the
mouseleave
event occurs.
Example
Basic Usage:
Suppose you want to change the background color of a div when the mouse leaves it. You can achieve this using the .on() method as follows:
index.htmlCopied<div id="target">Hover over me</div>
example.jsCopied$("#target").on("mouseleave", function() { $(this).css("background-color", "red"); });
This will change the background color of the div with the ID target to red when the mouse leaves it.
Event Delegation:
Event delegation is a powerful concept in jQuery that allows you to attach a single event handler to a parent element, which will then apply to all matching child elements, present now or in the future. Here's an example of using event delegation with .on() for the
mouseleave
event:index.htmlCopied<ul id="list"> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul>
example.jsCopied$("#list").on("mouseleave", "li", function() { $(this).css("background-color", "blue"); });
This will change the background color of the <li> element to blue when the mouse leaves it.
Passing Additional Data:
You can also pass additional data to the event handler if needed. For instance:
example.jsCopied$("#target").on("mouseleave", {message: "Goodbye!"}, function(event) { alert(event.data.message); });
This will alert "Goodbye!" when the mouse leaves the element with the ID target.
Conclusion
The .on() method provides a versatile way to handle the mouseleave
event and other events in jQuery. By understanding its syntax and usage, you can create responsive and interactive web pages efficiently.
Embracing this method allows you to adapt to changes in jQuery and ensures your code remains up-to-date and maintainable.
Join our Community:
Author
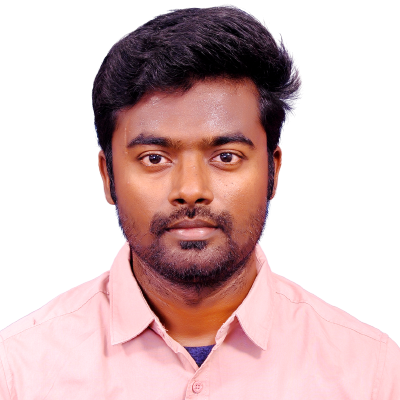
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery mouseleave Event), please comment here. I will help you immediately.