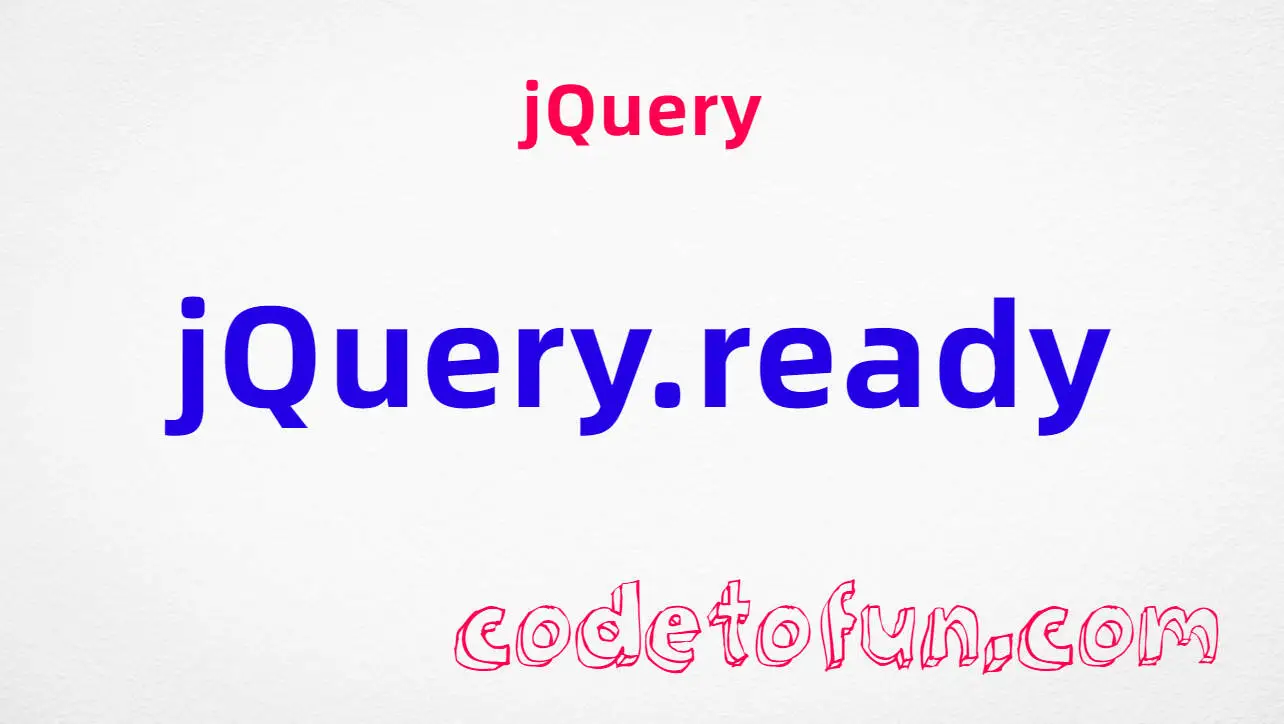
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery event.metaKey Property

Photo Credit to CodeToFun
π Introduction
jQuery is a versatile library that makes handling events in JavaScript simpler and more efficient. One useful feature for handling keyboard and mouse events is the event.metaKey
property. This property allows you to detect if the Meta key (Command key on Mac keyboards, Windows key on Windows keyboards) was pressed during an event.
This guide will help you understand how to use the event.metaKey
property effectively with practical examples.
π§ Understanding event.metaKey Property
The event.metaKey
property is a boolean property that indicates whether the Meta key was pressed when an event occurred. It is particularly useful for handling complex keyboard shortcuts and enhancing user interactions.
π‘ Syntax
The syntax for the event.metaKey
property is straightforward:
event.metaKey
The property returns true
if the Meta key was pressed, otherwise false
.
π Example
Detecting Meta Key in Keyboard Events:
You can use the
event.metaKey
property to detect if the Meta key was pressed during a keydown event. Hereβs an example:index.htmlCopied<input type="text" id="textInput" placeholder="Type here">
example.jsCopied$("#textInput").keydown(function(event) { if(event.metaKey) { console.log("Meta key is pressed."); } else { console.log("Meta key is not pressed."); } });
In this example, whenever a key is pressed inside the text input, it checks if the Meta key was also pressed and logs the result to the console.
Using Meta Key with Mouse Events:
The
event.metaKey
property is not limited to keyboard events; it can also be used with mouse events. For instance, you can detect if the Meta key was pressed during a click event:index.htmlCopied<button id="myButton">Click Me</button>
example.jsCopied$("#myButton").click(function(event) { if(event.metaKey) { alert("Meta key was pressed during the click."); } else { alert("Meta key was not pressed during the click."); } });
This code will display an alert indicating whether the Meta key was pressed when the button was clicked.
Combining Meta Key with Other Modifier Keys:
You can combine the
event.metaKey
property with other modifier keys such as event.ctrlKey, event.shiftKey, and event.altKey to create complex shortcuts. For example:index.htmlCopied<input type="text" id="shortcutInput" placeholder="Try a shortcut">
example.jsCopied$("#shortcutInput").keydown(function(event) { if(event.metaKey && event.shiftKey) { console.log("Meta + Shift keys are pressed together."); } });
This example checks if both the Meta key and the Shift key are pressed simultaneously during a keydown event.
Implementing Custom Shortcuts:
You can use the
event.metaKey
property to create custom keyboard shortcuts for your web application. For instance, you might want to implement a shortcut that saves data when the user presses Meta + S:index.htmlCopied<button id="saveButton">Save</button>
example.jsCopied$(document).keydown(function(event) { if(event.metaKey && event.key === "s") { event.preventDefault(); // Prevent the default browser action $("#saveButton").click(); // Trigger the save button click event } });
This code prevents the default action of the Meta + S combination (e.g., saving the page) and triggers a custom save action instead.
π Conclusion
The jQuery event.metaKey
property is a powerful tool for enhancing user interactions by detecting the state of the Meta key during events. Whether youβre creating custom shortcuts, handling complex user inputs, or simply improving the user experience, understanding how to use event.metaKey
can make your web applications more intuitive and responsive.
By mastering this property, you can unlock new possibilities in your web development projects.
π¨βπ» Join our Community:
Author
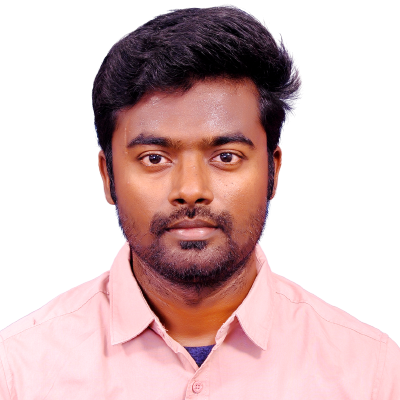
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery event.metaKey Property), please comment here. I will help you immediately.