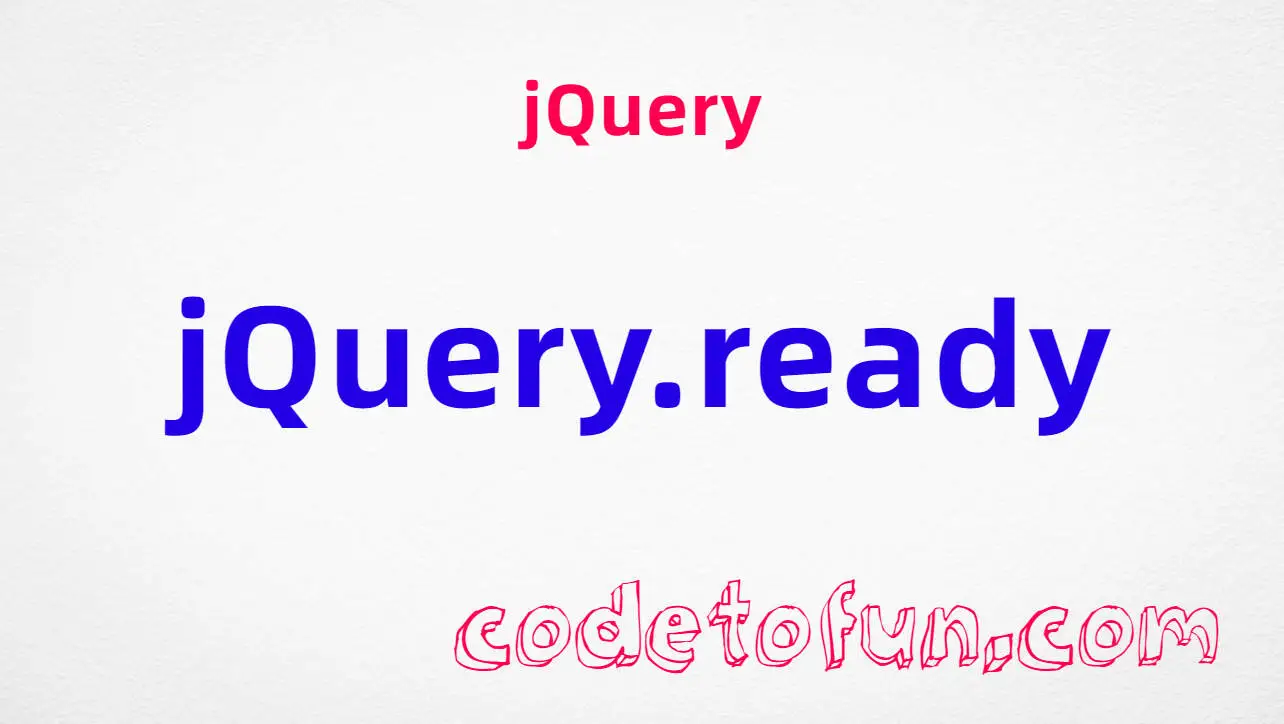
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery .ajaxStart() Method
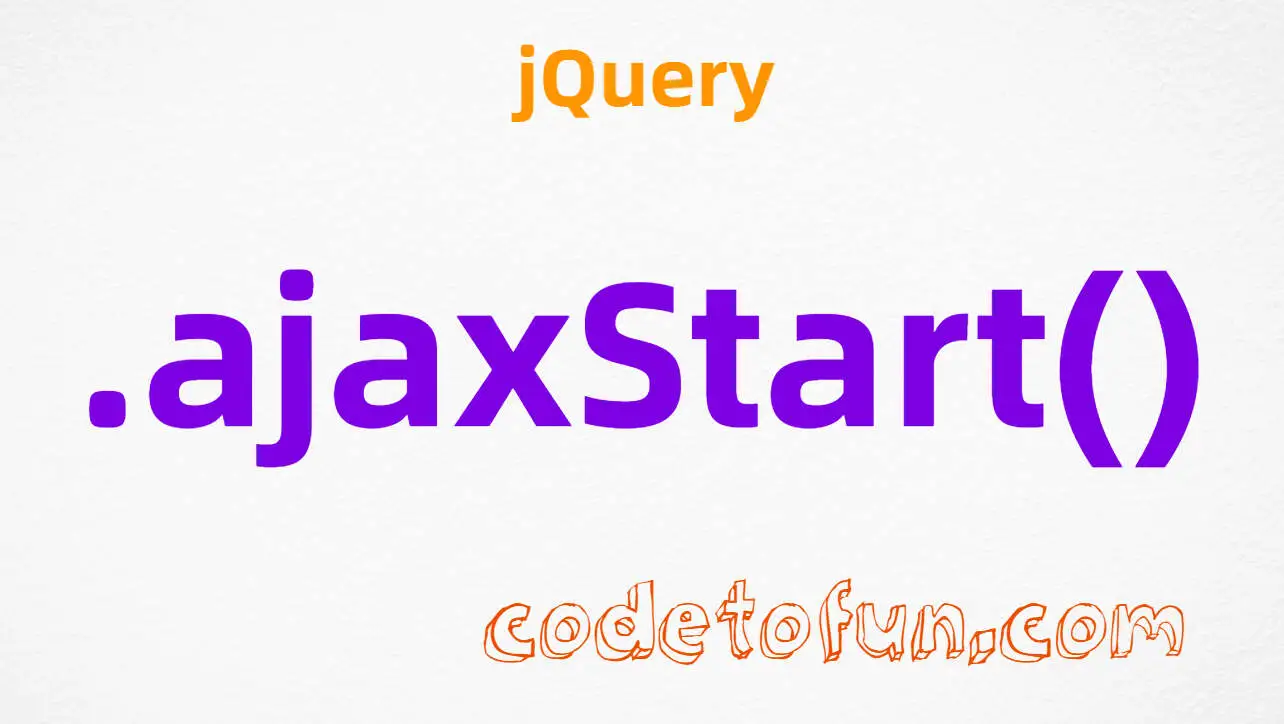
Photo Credit to CodeToFun
🙋 Introduction
jQuery offers a wide range of methods to manage and manipulate AJAX (Asynchronous JavaScript and XML) operations seamlessly. One such method is ajaxStart
, which is a global event handler that triggers whenever an AJAX request starts. This can be particularly useful for providing feedback to users, such as showing a loading spinner.
In this guide, we'll explore the ajaxStart
method, its usage, and practical examples to help you leverage this functionality effectively.
🧠 Understanding .ajaxStart() Method
The ajaxStart
method is part of jQuery's AJAX event handling system. It is triggered whenever an AJAX request is initiated, providing a convenient hook to execute code before the request is sent. This method is often used to show indicators that a background operation is in progress.
💡 Syntax
The syntax for the .ajaxStart()
method is straightforward:
$(document).ajaxStart(function() {
// Code to run when an AJAX request starts
});
📝 Example
Showing a Loading Spinner:
A common use case for
ajaxStart
is to display a loading spinner when an AJAX request is in progress. Here's an example:index.htmlCopied<div id="loading" style="display:none;">Loading...</div>
example.jsCopied$(document).ajaxStart(function() { $("#loading").show(); }).ajaxStop(function() { $("#loading").hide(); });
In this example, the #loading element is shown when an AJAX request starts and hidden when the request completes.
Disabling Form Submission:
You can use
ajaxStart
to disable a form's submit button while an AJAX request is in progress, preventing multiple submissions:index.htmlCopied<form id="myForm"> <input type="text" name="data"> <button type="submit" id="submitBtn">Submit</button> </form>
example.jsCopied$(document).ajaxStart(function() { $("#submitBtn").prop("disabled", true); }).ajaxStop(function() { $("#submitBtn").prop("disabled", false); });
This ensures that the submit button is disabled during the AJAX request and re-enabled once the request completes.
Combining with Other AJAX Events:
You can combine
ajaxStart
with other AJAX event handlers like ajaxStop, ajaxError, and ajaxSuccess for more comprehensive AJAX request handling:example.jsCopied$(document).ajaxStart(function() { $("#loading").show(); }).ajaxStop(function() { $("#loading").hide(); }).ajaxError(function(event, jqxhr, settings, thrownError) { alert("An error occurred: " + thrownError); });
This example shows a loading indicator during the request and handles errors by displaying an alert.
Handling Multiple AJAX Requests:
If your application makes multiple AJAX requests,
ajaxStart
will trigger at the start of each request. To avoid repetitive actions, ensure that your handling logic accounts for multiple requests:example.jsCopied$(document).ajaxStart(function() { if ($(".loading-indicator").length === 0) { $("body").append('<div class="loading-indicator">Loading...</div>'); } }).ajaxStop(function() { $(".loading-indicator").remove(); });
This ensures that only one loading indicator is shown regardless of the number of AJAX requests.
🎉 Conclusion
The jQuery ajaxStart
method is a powerful tool for managing AJAX request states, allowing you to provide real-time feedback to users. By mastering its usage, you can enhance user experience by showing loading indicators, preventing multiple submissions, and handling errors gracefully.
Incorporate the ajaxStart
method into your projects to create more interactive and responsive web applications.
👨💻 Join our Community:
Author
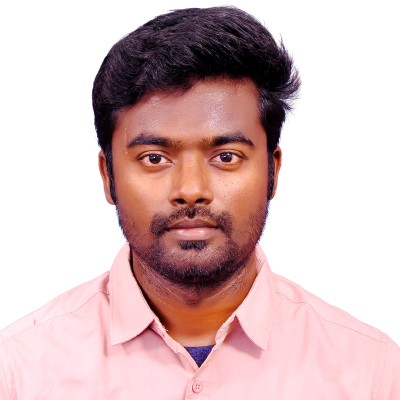
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee