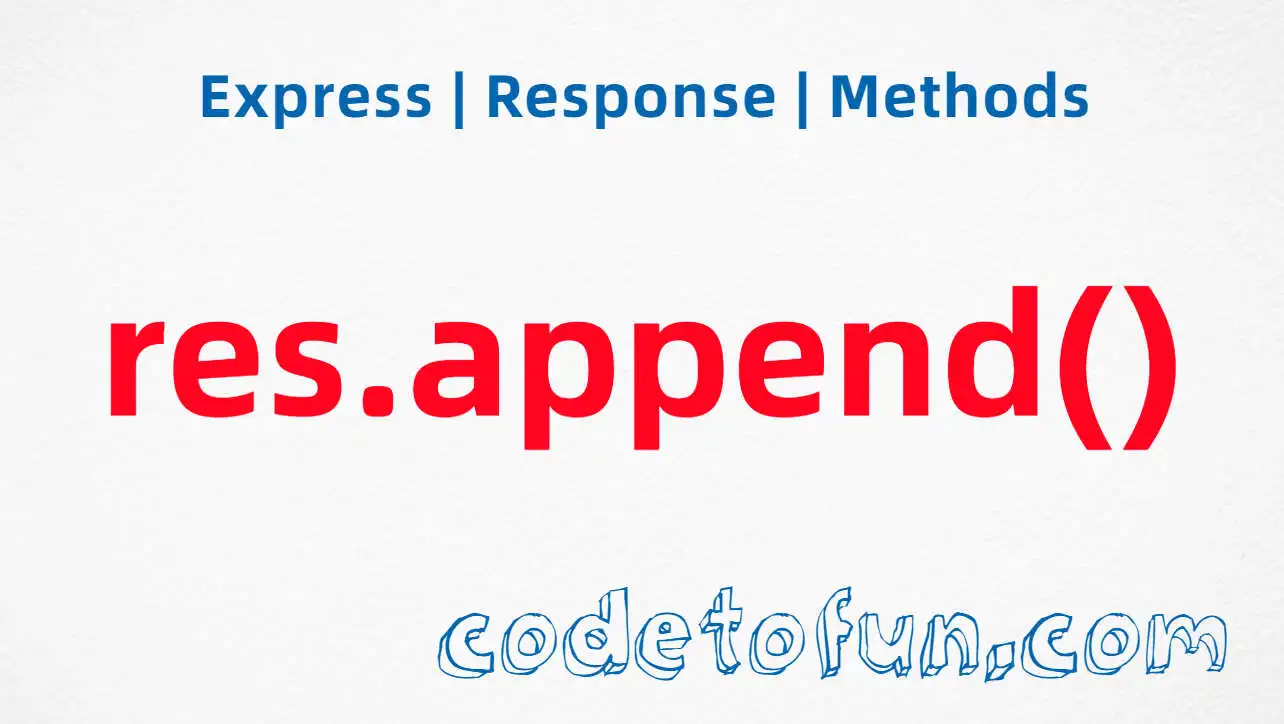
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.set() Method
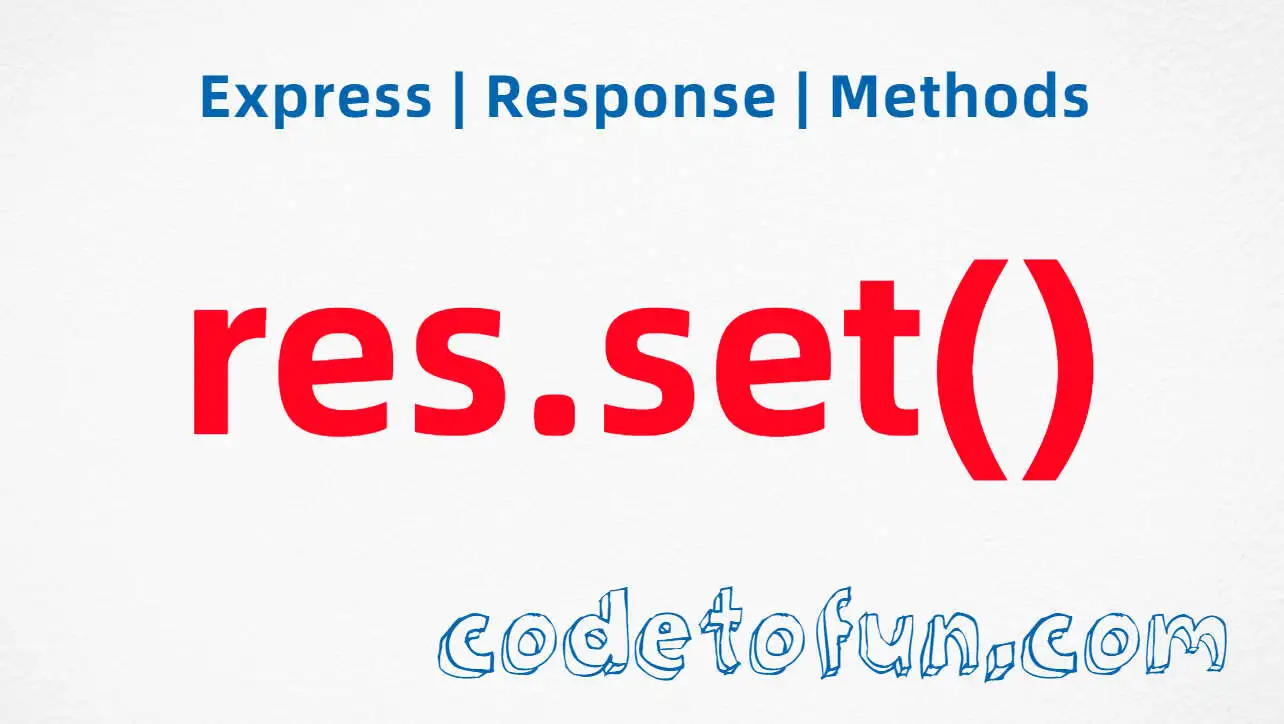
Photo Credit to CodeToFun
🙋 Introduction
When building web applications with Express.js, it's crucial to have control over the HTTP response headers sent to the client. The res.set()
method in Express allows developers to set various response headers easily.
In this guide, we'll explore the syntax, use cases, and best practices for the res.set()
method.
💡 Syntax
The syntax for the res.set()
method is straightforward:
res.set(field, [value])
- field: A string representing the name of the HTTP header.
- value: The value to set for the specified header field.
📡 Setting HTTP Response Headers
The res.set()
method is commonly used to customize HTTP response headers. This can include setting headers such as Content-Type, Cache-Control, or custom headers.
app.get('/api/data', (req, res) => {
// Set custom response headers
res.set('Content-Type', 'application/json');
res.set('Cache-Control', 'public, max-age=3600');
// Send JSON response
res.json({ message: 'Data retrieved successfully' });
});
In this example, we use res.set()
to set the Content-Type and Cache-Control headers before sending a JSON response.
📚 Use Cases
Content-Type Configuration:
Use
res.set()
to specify the Content-Type header when serving different types of files.example.jsCopiedapp.get('/download/pdf', (req, res) => { // Set Content-Type for PDF files res.set('Content-Type', 'application/pdf'); // Send PDF file res.sendFile('/path/to/file.pdf'); });
Caching Strategy:
Leverage
res.set()
to define caching strategies for static assets, improving performance by reducing server requests.example.jsCopiedapp.get('/images/:imageName', (req, res) => { const imageName = req.params.imageName; // Set Cache-Control for images with a one-day cache res.set('Cache-Control', 'public, max-age=86400'); // Send image res.sendFile(`/path/to/images/${imageName}`); });
🏆 Best Practices
Be Specific with Headers:
Be specific when setting headers to ensure the correct behavior. For instance, use Content-Type for specifying the type of content being sent.
example.jsCopied// Set Content-Type for JSON response res.set('Content-Type', 'application/json');
Chain Multiple Headers:
You can chain multiple
res.set()
calls to set multiple headers in a concise manner.example.jsCopiedres .set('Content-Type', 'text/html') .set('Cache-Control', 'no-cache');
Conditional Header Setting:
Conditionally set headers based on certain criteria or request parameters.
example.jsCopiedapp.get('/secure/data', (req, res) => { if (req.isAuthenticated()) { // Set custom header for authenticated users res.set('X-Authenticated-User', req.user.username); } // Process and send response // ... });
🎉 Conclusion
The res.set()
method in Express.js provides a flexible way to manage HTTP response headers. Whether configuring content types, implementing caching strategies, or adding custom headers, understanding how to use res.set()
enhances your control over the server's communication with clients.
Now, with a solid understanding of the res.set()
method, go ahead and optimize your Express.js applications by fine-tuning your HTTP response headers!
👨💻 Join our Community:
Author
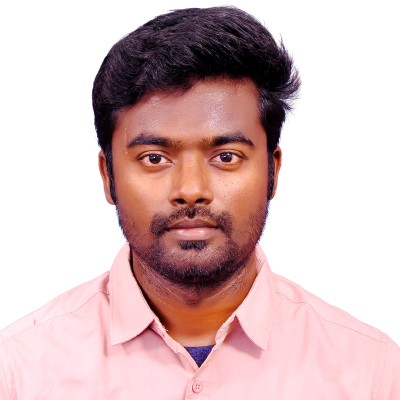
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.set() Method), please comment here. I will help you immediately.