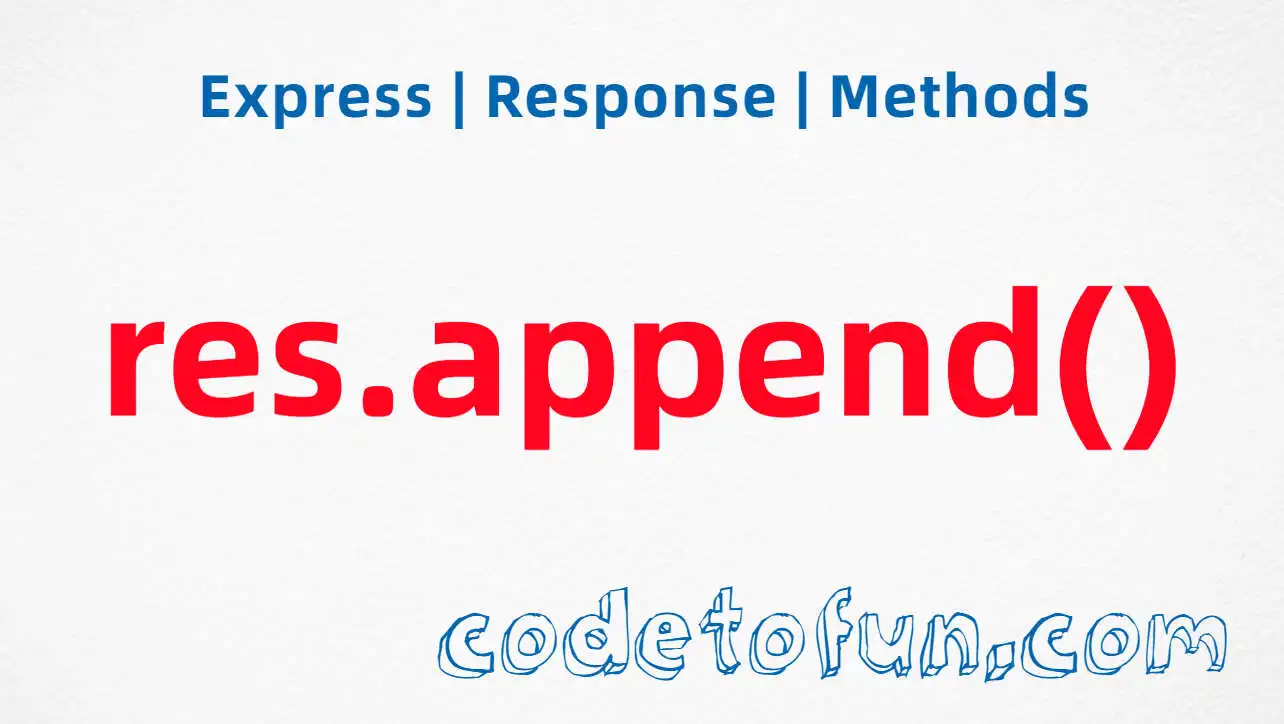
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.format() Method
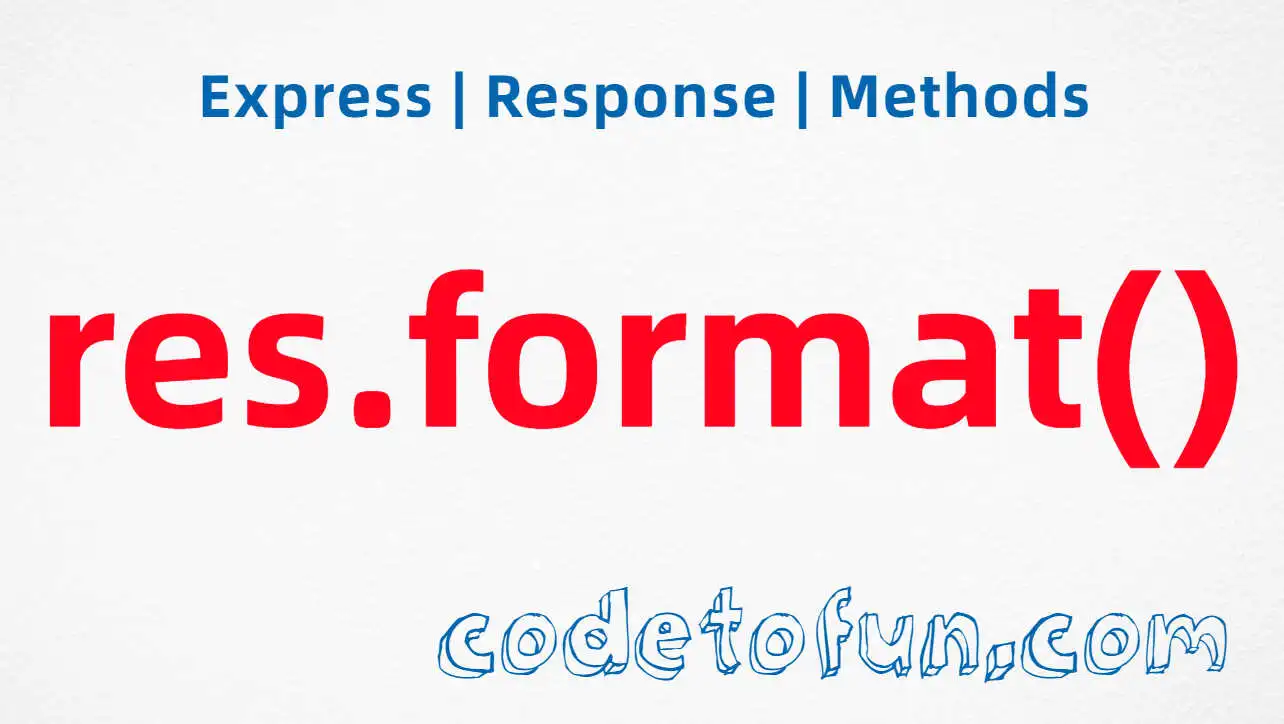
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a popular web application framework for Node.js, provides a variety of features for handling HTTP responses. One such powerful feature is the res.format()
method, which enables dynamic content negotiation.
In this guide, we'll explore the syntax, use cases, and best practices of the res.format()
method in Express.js.
💡 Syntax
The res.format()
method in Express.js has a flexible and expressive syntax:
res.format(object)
- object: An object where the keys are the possible content types and the values are the corresponding handler functions or data.
❓ How res.format() Works
res.format()
allows your Express.js application to respond with different content types based on the client's preferences. It inspects the Accept header in the request and selects the appropriate response format. This is particularly useful for supporting multiple data formats, such as JSON, XML, or HTML, based on the client's preferences.
app.get('/api/data', (req, res) => {
const responseData = {
message: 'Hello, Express!'
};
res.format({
'text/html': () => {
res.send('<h1>Hello, Express!</h1>');
},
'application/json': () => {
res.json(responseData);
},
'text/xml': () => {
const xmlResponse = `<message>Hello, Express!</message>`;
res.type('application/xml').send(xmlResponse);
}
});
});
In this example, the server responds with HTML, JSON, or XML based on the client's Accept header.
📚 Use Cases
API Responses with Multiple Formats:
Use
res.format()
to provide different response formats for API endpoints, allowing clients to request data in various formats.example.jsCopiedapp.get('/api/data', (req, res) => { const responseData = { message: 'Hello, Express!' }; res.format({ 'text/html': () => { res.render('index', { message: 'Hello, Express!' }); }, 'application/json': () => { res.json(responseData); } }); });
Content Negotiation for Webpages:
Apply content negotiation to web pages, responding with HTML or JSON based on the client's preferences.
example.jsCopiedapp.get('/webpage', (req, res) => { res.format({ 'text/html': () => { res.render('webpage'); }, 'application/json': () => { const jsonResponse = { content: 'This is a webpage in JSON format.' }; res.json(jsonResponse); } }); });
🏆 Best Practices
Provide Fallbacks:
Include a default or wildcard handler in your
res.format()
calls to handle cases where the client doesn't explicitly specify a supported format.example.jsCopiedres.format({ 'text/html': () => { res.render('index'); }, 'application/json': () => { res.json(responseData); }, default: () => { res.status(406).send('Not Acceptable'); } });
Support Common Content Types:
Be sure to support common content types that clients may request, such as HTML, JSON, XML, and others relevant to your application.
example.jsCopiedres.format({ 'text/html': () => { res.render('index'); }, 'application/json': () => { res.json(responseData); }, 'text/xml': () => { const xmlResponse = `<message>Hello, Express!</message>`; res.type('application/xml').send(xmlResponse); } });
🎉 Conclusion
The res.format()
method in Express.js provides a powerful mechanism for dynamic content negotiation, allowing your application to respond with different formats based on client preferences. Leveraging this feature enhances the flexibility and usability of your API endpoints or web pages.
Now, armed with knowledge about the res.format()
method, go ahead and implement dynamic content negotiation in your Express.js projects!
👨💻 Join our Community:
Author
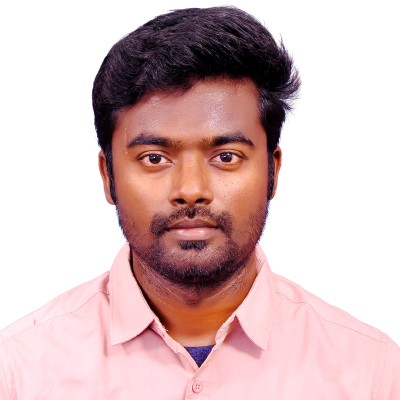
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.format() Method), please comment here. I will help you immediately.