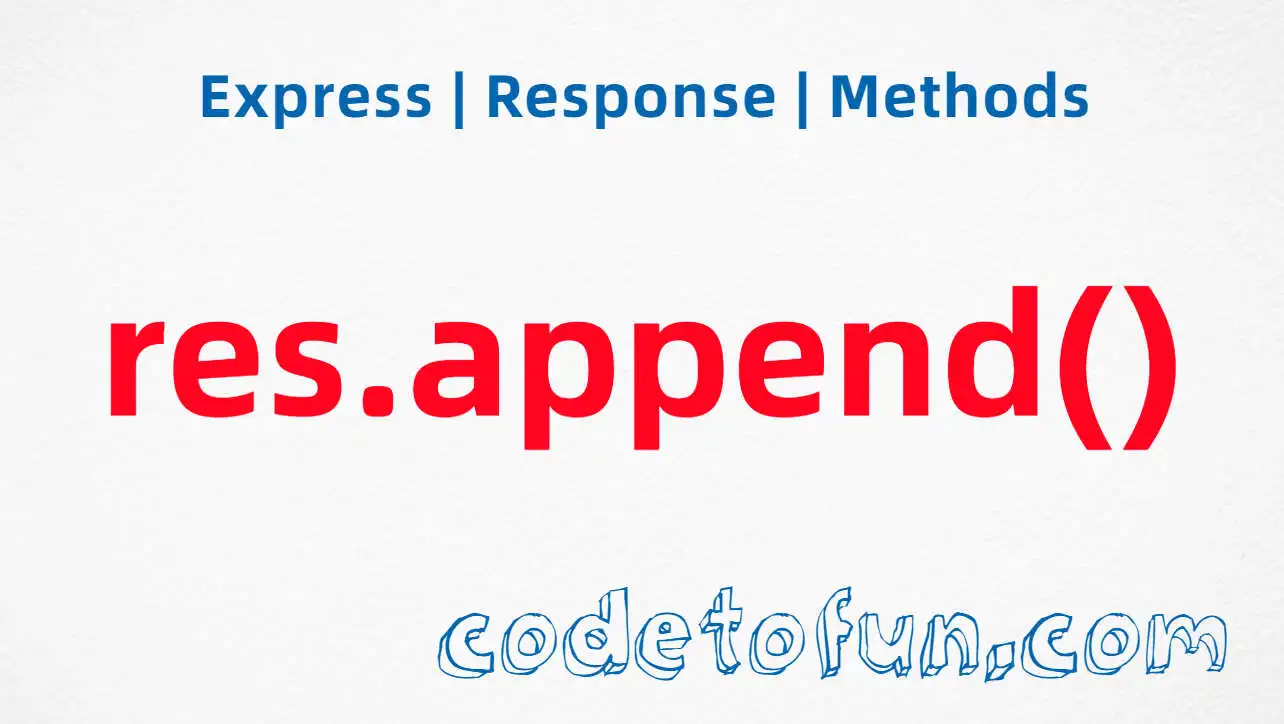
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.jsonp() Method
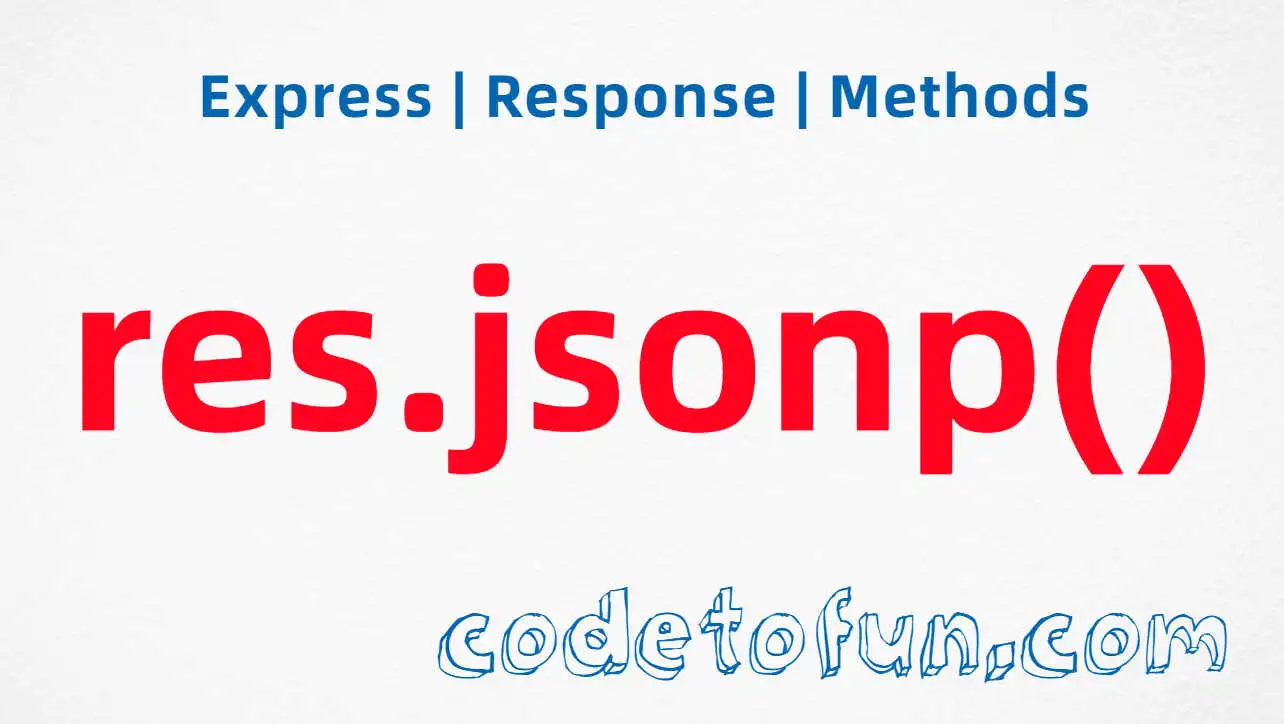
Photo Credit to CodeToFun
🙋 Introduction
In web development, cross-origin requests can pose challenges due to browser security policies.
JSONP (JSON with Padding) is a technique that allows for cross-origin requests in a browser. The res.jsonp()
method in Express.js simplifies the generation of JSONP responses.
In this guide, we'll explore the syntax, use cases, and best practices for using res.jsonp()
.
💡 Syntax
The syntax for the res.jsonp()
method is straightforward:
res.jsonp([body])
- body: The data to be sent as the JSONP response. This can be any JSON-serializable data.
❓ How res.jsonp() Works
The res.jsonp()
method in Express.js is an extension of the res.json() method, specifically designed for JSONP responses. It wraps the JSON data in a function call, allowing for dynamic script tag insertion in the browser, which is a common approach for JSONP requests.
app.get('/api/data', (req, res) => {
const jsonData = { message: 'Hello, JSONP!' };
res.jsonp(jsonData);
});
In this example, a route /api/data responds with JSONP-formatted data.
📚 Use Cases
Cross-Origin Data Requests:
JSONP is particularly useful for making cross-origin requests in scenarios where CORS (Cross-Origin Resource Sharing) is not supported.
example.jsCopied// Client-side JavaScript const script = document.createElement('script'); script.src = 'http://example.com/api/data?callback=handleData'; document.body.appendChild(script); // Callback function function handleData(data) { console.log(data); }
Here, the client-side script dynamically creates a script tag with the JSONP request, and the server responds with the data wrapped in the specified callback function.
Third-Party API Integration:
When integrating with third-party APIs that support JSONP, the
res.jsonp()
method simplifies handling the responses.example.jsCopiedapp.get('/external/api', (req, res) => { // Make a request to an external API const externalData = fetchDataFromExternalAPI(); // Send JSONP response res.jsonp(externalData); });
🏆 Best Practices
Secure Callback Handling:
Ensure that the callback parameter is properly validated to prevent potential security vulnerabilities like Cross-Site Scripting (XSS).
example.jsCopiedapp.get('/api/data', (req, res) => { const jsonData = { message: 'Hello, JSONP!' }; // Validate and sanitize the callback parameter const callbackFunction = req.query.callback.replace(/[^\w.]/g, ''); // Send JSONP response with validated callback res.jsonp(callbackFunction, jsonData); });
Error Handling:
Implement robust error handling to gracefully handle situations where JSONP responses cannot be generated.
example.jsCopiedapp.get('/api/data', (req, res) => { try { const jsonData = fetchData(); res.jsonp(jsonData); } catch (error) { console.error(error); res.status(500).jsonp({ error: 'Internal Server Error' }); } });
🎉 Conclusion
The res.jsonp()
method in Express.js simplifies the generation of JSONP responses, making it easier to handle cross-origin requests and integrate with third-party APIs. Understanding its syntax, use cases, and best practices will enhance your ability to implement secure and effective JSONP responses in your Express.js applications.
Now, equipped with knowledge about the res.jsonp()
method, go ahead and streamline your JSONP responses in Express.js!
👨💻 Join our Community:
Author
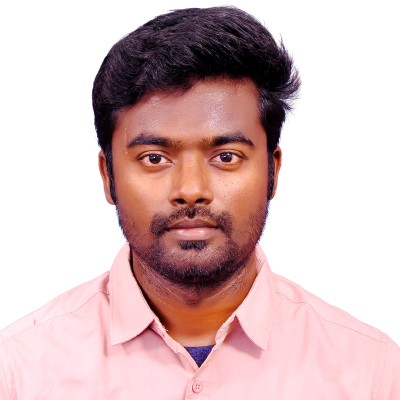
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.jsonp() Method), please comment here. I will help you immediately.