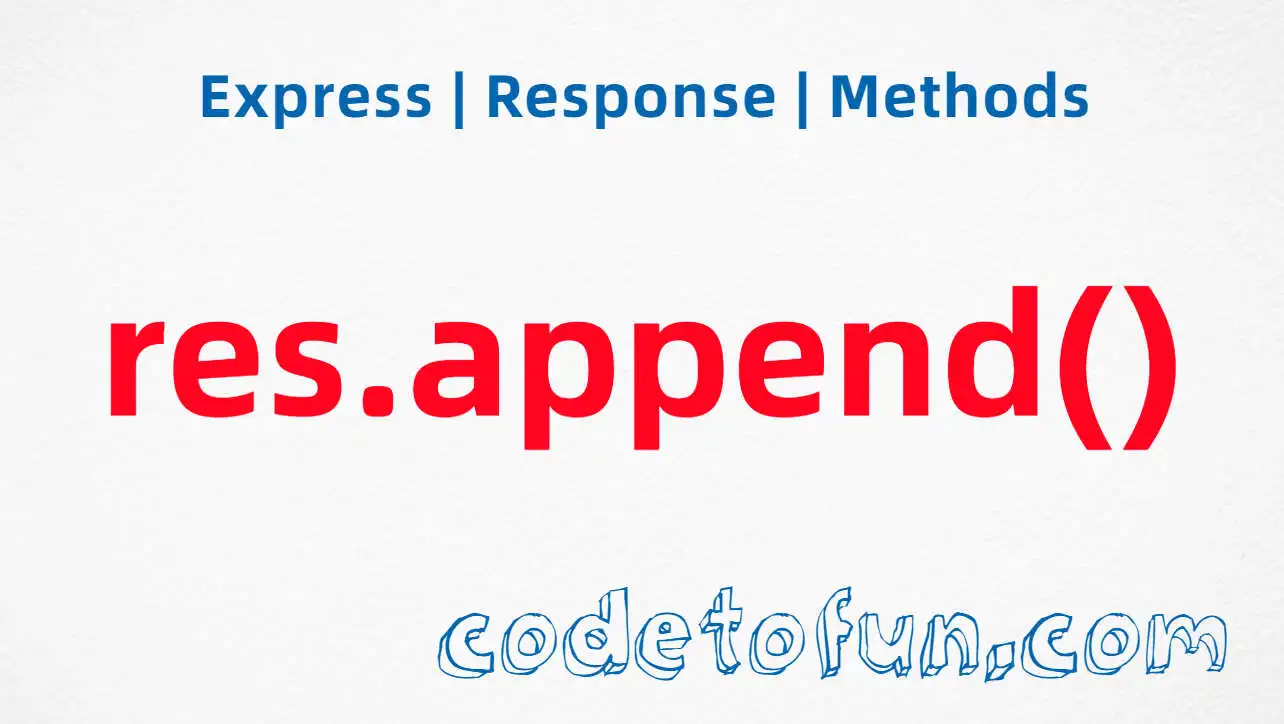
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.download() Method
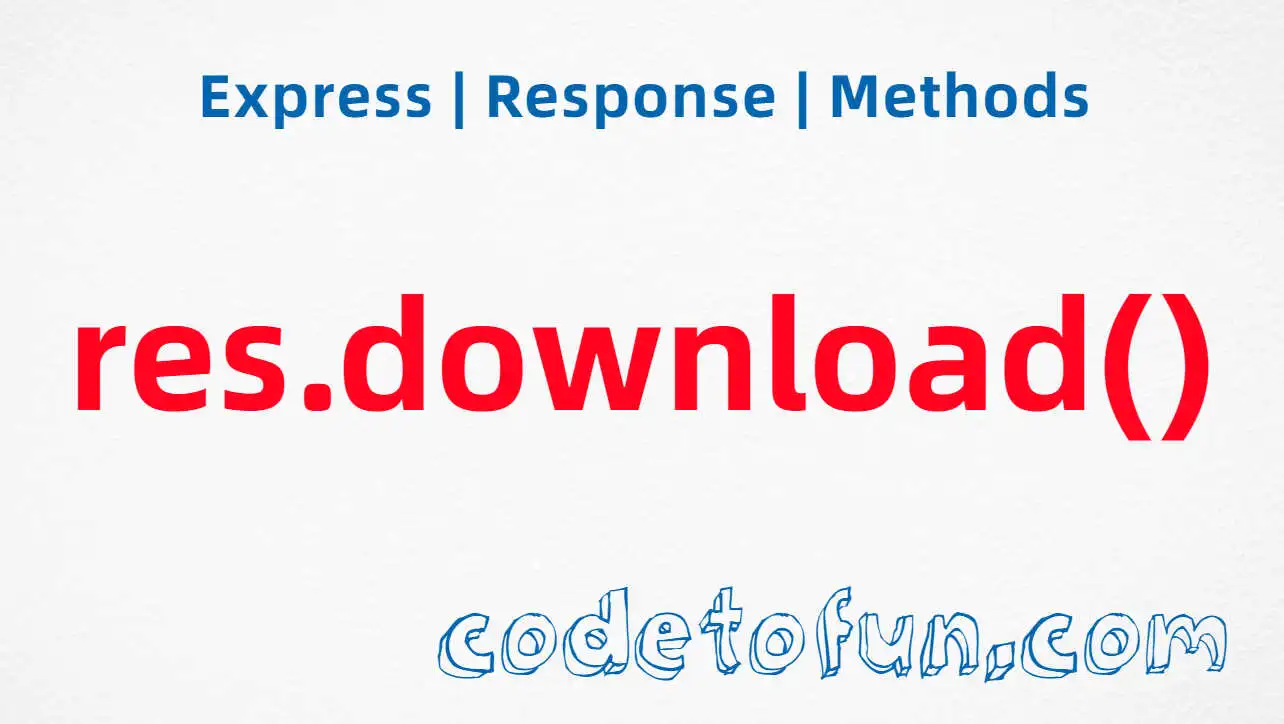
Photo Credit to CodeToFun
🙋 Introduction
File downloads are a common requirement in web applications, and Express.js simplifies this process with the res.download()
method. This powerful feature allows you to initiate file downloads seamlessly.
In this guide, we'll explore the syntax, usage, and practical examples of the res.download()
method, providing you with the tools to enhance file handling in your Express.js applications.
💡 Syntax
The syntax for the res.download()
method is straightforward:
res.download(path [, filename] [, options] [, callback])
- path: The file path on the server.
- filename (optional): The name to suggest to the user when saving the file.
- options (optional): Additional options, such as headers.
- callback (optional): A callback function to be executed after the file is sent.
❓ How res.download() Works
The res.download()
method prompts the user to download a file from the server. It sets the appropriate headers for file download and handles any errors that may occur during the process.
app.get('/download', (req, res) => {
const filePath = '/path/to/your/file.txt';
res.download(filePath, 'custom-filename.txt', (err) => {
if (err) {
// Handle errors, such as file not found
res.status(404).send('File not found');
}
});
});
In this example, accessing the /download route triggers a file download with a custom filename.
📚 Use Cases
Secure File Downloads:
Leverage
res.download()
to ensure that file downloads are secure by implementing authentication and authorization checks.example.jsCopiedapp.get('/secure-download', (req, res) => { const filePath = '/path/to/secure/file.pdf'; // Perform authentication or authorization checks before allowing the download if (/* authentication or authorization logic */) { res.download(filePath, 'document.pdf'); } else { res.status(401).send('Unauthorized'); } });
Serving Dynamic Files:
Use
res.download()
to serve dynamically generated content by creating a temporary file.example.jsCopiedapp.get('/dynamic-download', (req, res) => { const dynamicContent = generateDynamicContent(); // Your logic to generate dynamic content // Create a temporary file with dynamic content const tempFilePath = '/path/to/temp/file.txt'; fs.writeFileSync(tempFilePath, dynamicContent); // Initiate download with the temporary file res.download(tempFilePath, 'dynamic-file.txt', (err) => { // Clean up the temporary file after download fs.unlinkSync(tempFilePath); if (err) { res.status(500).send('Internal Server Error'); } }); });
🏆 Best Practices
Error Handling:
Implement robust error handling to address scenarios like file not found or internal server errors during the download process.
example.jsCopiedapp.get('/download', (req, res) => { const filePath = '/path/to/your/file.txt'; res.download(filePath, (err) => { if (err) { // Handle errors, such as file not found if (err.code === 'ENOENT') { res.status(404).send('File not found'); } else { res.status(500).send('Internal Server Error'); } } }); });
Setting Custom Headers:
Adjust headers as needed, such as controlling caching behavior or specifying content type.
example.jsCopiedapp.get('/download', (req, res) => { const filePath = '/path/to/your/file.txt'; // Set custom headers const options = { headers: { 'Content-Type': 'application/octet-stream', 'Content-Disposition': 'attachment; filename="custom-filename.txt"', }, }; res.download(filePath, options, (err) => { if (err) { // Handle errors res.status(500).send('Internal Server Error'); } }); });
🎉 Conclusion
The res.download()
method in Express.js provides a streamlined way to facilitate file downloads in your web applications. Whether you're serving static files or dynamically generating content, incorporating res.download()
enhances user experience and simplifies file handling.
Now, armed with knowledge about the res.download()
method, go ahead and implement efficient file downloads in your Express.js projects!
👨💻 Join our Community:
Author
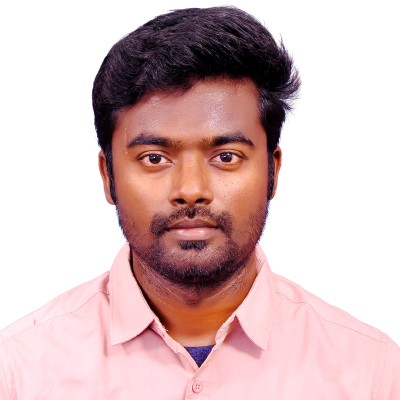
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.download() Method), please comment here. I will help you immediately.