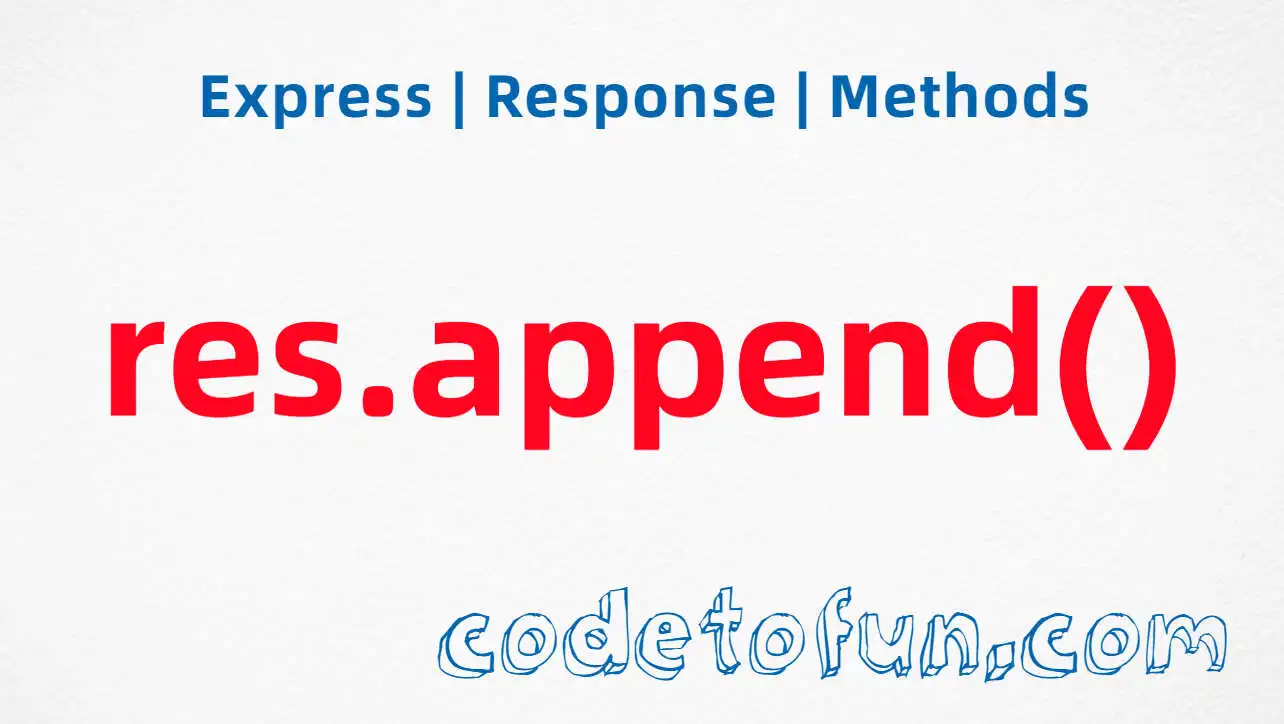
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.attachment() Method
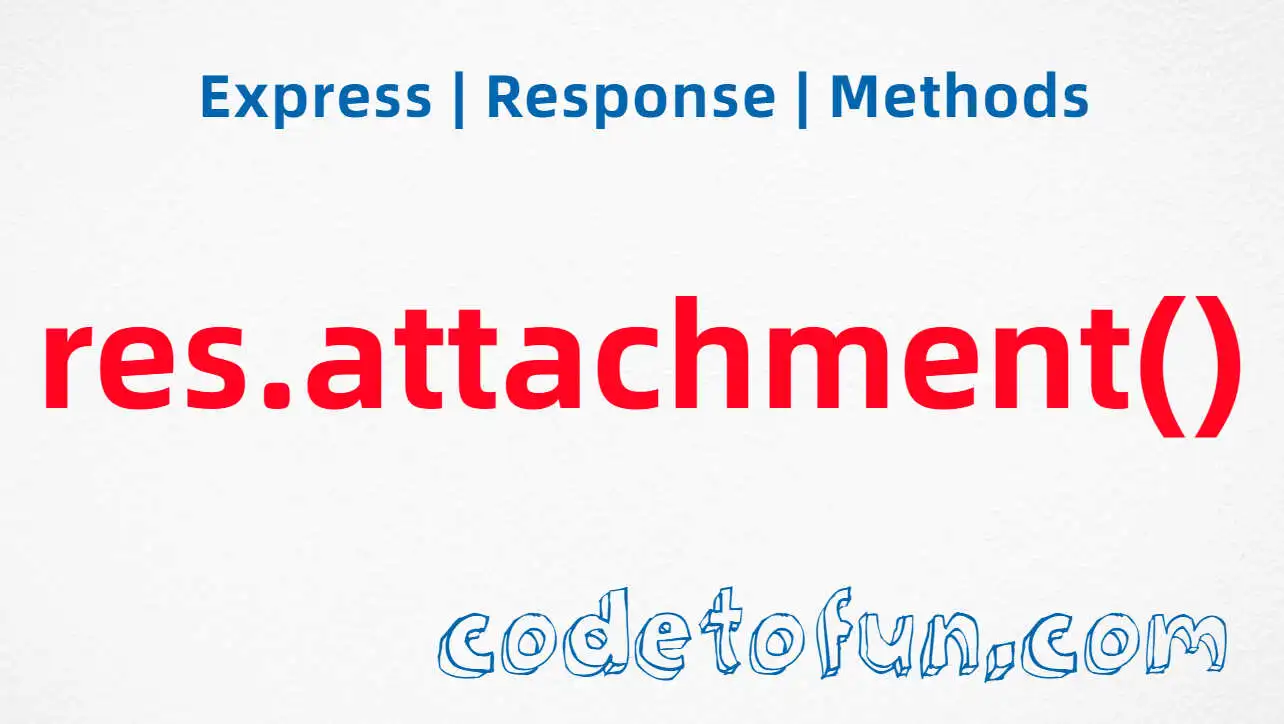
Photo Credit to CodeToFun
🙋 Introduction
Handling file downloads is a common requirement in web applications. In Express.js, the res.attachment()
method proves to be a valuable tool for streamlining this process.
In this guide, we'll explore the syntax, usage, and practical examples of the res.attachment()
method to enhance your understanding of serving and managing file downloads in Express.js.
💡 Syntax
The syntax for the res.attachment()
method is straightforward:
res.attachment([filename])
- filename (optional): A string specifying the suggested filename for the attachment. If provided, it sets the Content-Disposition header with the suggested filename.
❓ How res.attachment() Works
The res.attachment()
method in Express.js simplifies the process of serving file downloads by setting appropriate headers, including Content-Disposition. This header suggests to the browser that the response should be treated as an attachment and prompts the user to download the file.
app.get('/download/:filename', (req, res) => {
const filename = req.params.filename;
const filePath = path.join(__dirname, 'downloads', filename);
// Set the appropriate headers for file download
res.attachment(filename);
// Stream the file to the response
const fileStream = fs.createReadStream(filePath);
fileStream.pipe(res);
});
In this example, the route /download/:filename serves as a dynamic endpoint for downloading files. The res.attachment(filename) sets the Content-Disposition header, and the file is streamed to the response.
📚 Use Cases
Downloading User-Generated Content:
Use
res.attachment()
to facilitate secure and controlled downloads of user-generated content, ensuring proper headers are set.example.jsCopiedapp.get('/download/user-content/:filename', (req, res) => { const filename = req.params.filename; const filePath = path.join(__dirname, 'user-uploads', filename); // Set the appropriate headers for file download res.attachment(filename); // Stream the user-generated content to the response const fileStream = fs.createReadStream(filePath); fileStream.pipe(res); });
Serving Downloadable Reports:
Leverage
res.attachment()
to serve downloadable reports securely, enhancing the user experience for accessing important documents.example.jsCopiedapp.get('/download/report/:filename', (req, res) => { const filename = req.params.filename; const filePath = path.join(__dirname, 'reports', filename); // Set the appropriate headers for file download res.attachment(filename); // Stream the report file to the response const fileStream = fs.createReadStream(filePath); fileStream.pipe(res); });
🏆 Best Practices
Dynamic File Serving:
Serve files dynamically based on user requests by using parameters in the route handler. This allows for a flexible and controlled file download experience.
example.jsCopiedapp.get('/download/:category/:filename', (req, res) => { const { category, filename } = req.params; const filePath = path.join(__dirname, 'downloads', category, filename); // Set the appropriate headers for file download res.attachment(filename); // Stream the file to the response const fileStream = fs.createReadStream(filePath); fileStream.pipe(res); });
Error Handling:
Implement error handling to manage scenarios where the requested file is not found or cannot be served. Ensure proper HTTP status codes and informative error messages.
example.jsCopiedapp.get('/download/:filename', (req, res) => { const filename = req.params.filename; const filePath = path.join(__dirname, 'downloads', filename); // Check if the file exists if (!fs.existsSync(filePath)) { res.status(404).send('File not found'); return; } // Set the appropriate headers for file download res.attachment(filename); // Stream the file to the response const fileStream = fs.createReadStream(filePath); fileStream.pipe(res); });
🎉 Conclusion
The res.attachment()
method in Express.js simplifies the process of serving file downloads by setting crucial headers. Whether you're facilitating user-generated content downloads or serving important reports, understanding how to use res.attachment()
enhances your ability to provide a seamless and secure file download experience in your Express.js applications.
Now, equipped with knowledge about the res.attachment()
method, go ahead and optimize file downloads in your Express.js projects!
👨💻 Join our Community:
Author
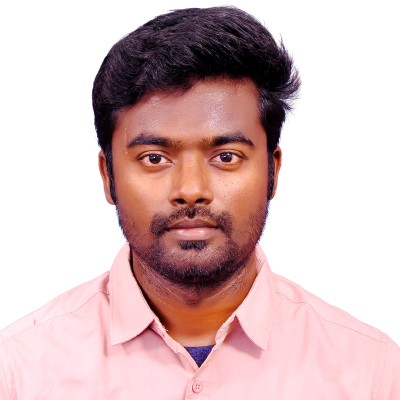
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.attachment() Method), please comment here. I will help you immediately.