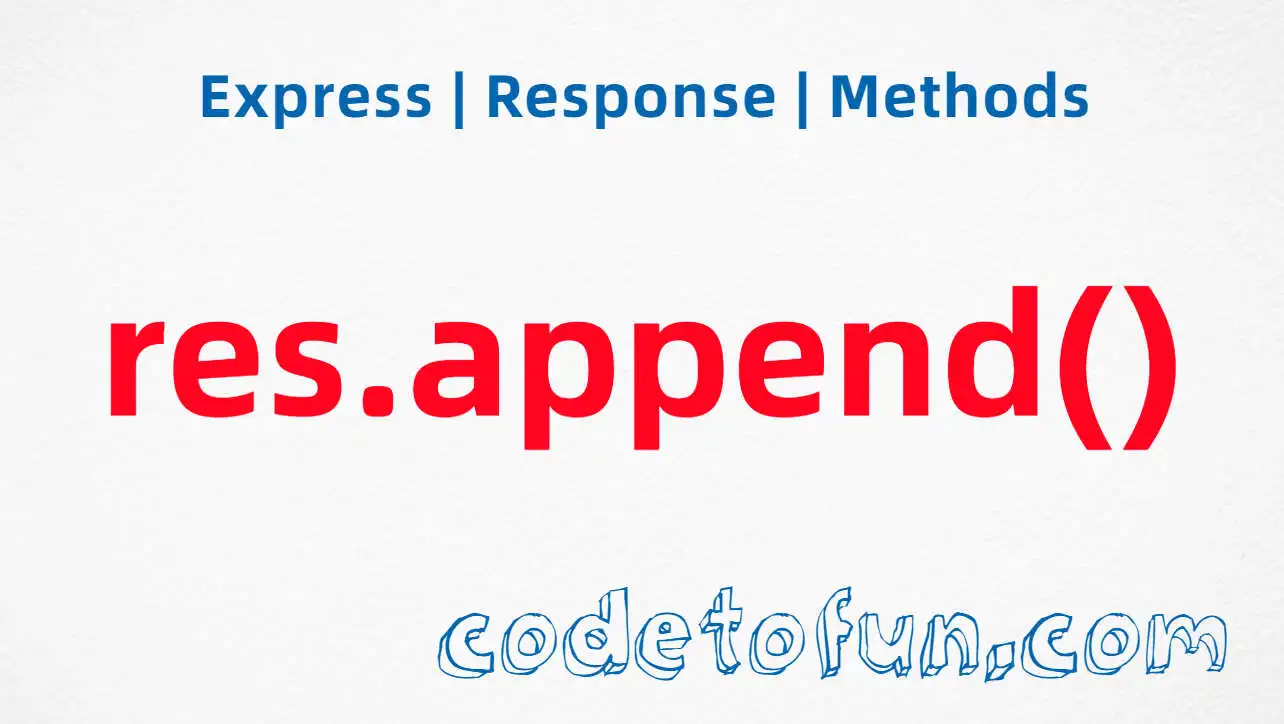
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.headersSent Property
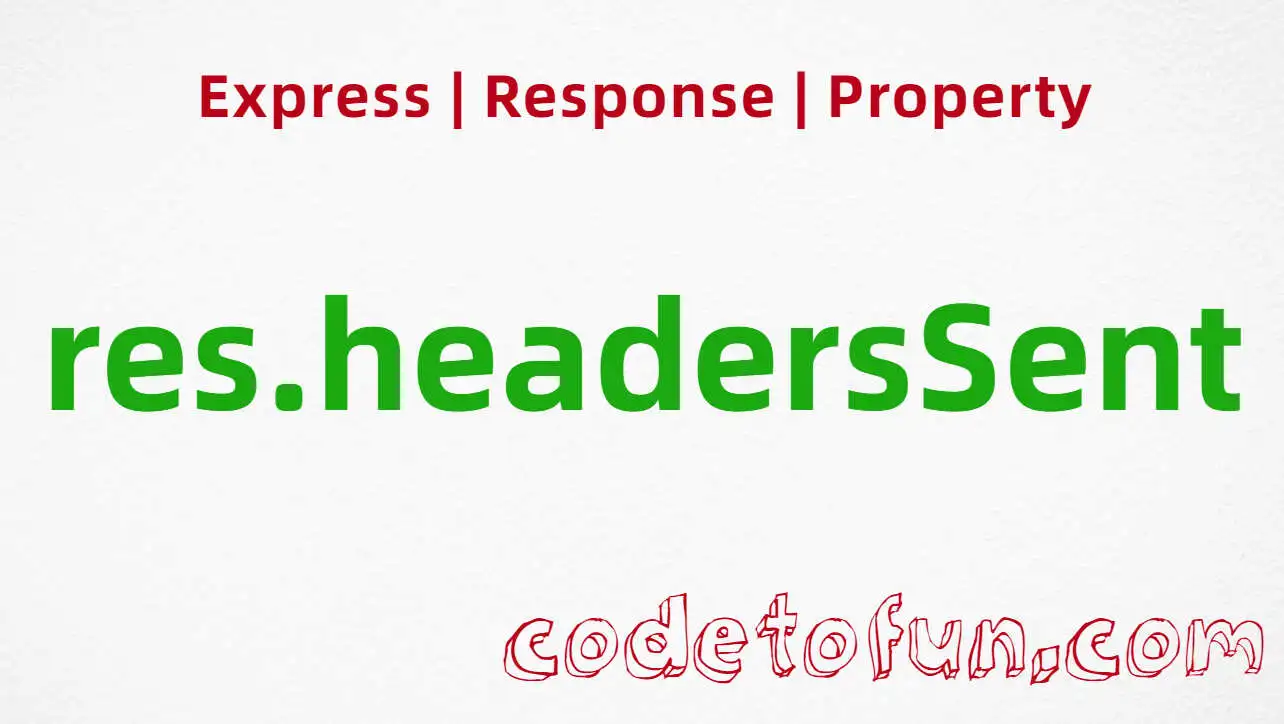
Photo Credit to CodeToFun
🙋 Introduction
In Express.js, managing HTTP headers is crucial for controlling the behavior of your web application. The res.headersSent
property is a valuable tool that allows you to check whether headers have been sent in the HTTP response.
In this guide, we'll explore the syntax, use cases, and best practices for utilizing the res.headersSent
property in your Express.js applications.
💡 Syntax
The res.headersSent
property can be accessed directly from the response object:
if (res.headersSent) {
// Headers have been sent
} else {
// Headers have not been sent
}
❓ How res.headersSent Works
The res.headersSent
property is a boolean value that indicates whether headers have been sent in the HTTP response. This is particularly useful when you need to conditionally set headers based on certain conditions or ensure that headers are not modified after being sent.
app.get('/example', (req, res) => {
if (!res.headersSent) {
// Set headers if not already sent
res.setHeader('Content-Type', 'text/plain');
}
res.send('Hello, Express!');
});
In this example, the res.headersSent
property is used to conditionally set the 'Content-Type' header before sending a response.
📚 Use Cases
Conditional Header Setting:
Use
res.headersSent
to conditionally set headers based on certain criteria before sending a response.conditional-header-settingCopiedapp.get('/conditional', (req, res) => { if (!res.headersSent) { // Set headers based on a condition if (req.query.format === 'json') { res.setHeader('Content-Type', 'application/json'); } else { res.setHeader('Content-Type', 'text/plain'); } } res.send('Conditional Header Setting'); });
Preventing Header Modification:
Leverage
res.headersSent
to prevent unintentional modification of headers after they have been sent.preventing-header-modification.jsCopiedapp.get('/immutable', (req, res) => { // Check if headers are already sent before attempting to modify if (!res.headersSent) { // Set headers res.setHeader('Cache-Control', 'no-store'); } // Attempting to modify headers after they are sent will throw an error res.send('Immutable Headers'); });
🏆 Best Practices
Early Check for Headers:
Perform checks for
res.headersSent
early in your route handler to ensure headers are set or modified before being sent.early-check-for-headersCopiedapp.get('/bestpractice', (req, res) => { // Early check for headers if (!res.headersSent) { // Set or modify headers as needed res.setHeader('Content-Type', 'text/html'); } res.send('Best Practice Example'); });
Use Conditional Statements:
Employ conditional statements with
res.headersSent
to dynamically adjust header settings based on application logic.use-conditional-statements.jsCopiedapp.get('/conditionalbest', (req, res) => { if (!res.headersSent) { // Set headers conditionally res.setHeader('X-Powered-By', 'Express'); if (req.query.debug === 'true') { res.setHeader('Debug-Mode', 'enabled'); } } res.send('Conditional Best Practice'); });
🎉 Conclusion
The res.headersSent
property in Express.js is a powerful tool for managing HTTP headers effectively. Whether you need to conditionally set headers or prevent unintended modifications, understanding how to leverage res.headersSent
is crucial for fine-tuning your Express.js applications.
Now, equipped with knowledge about res.headersSent
, go ahead and enhance the control over your HTTP headers in your Express.js projects!
👨💻 Join our Community:
Author
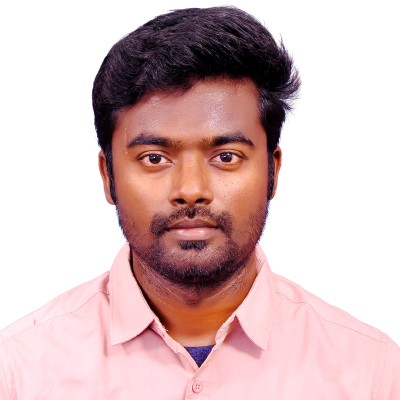
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.headersSent Property), please comment here. I will help you immediately.