.webp)
C++ String strncpy() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C++ programming, managing and manipulating strings is a common task.
The strncpy()
function is a part of the C Standard Library and is used for copying a specified number of characters from one string to another.
In this tutorial, we'll explore the usage and functionality of the strncpy()
function in C++.
đĄ Syntax
The signature of the strncpy()
function is as follows:
char* strncpy(char* destination, const char* source, size_t num);
This function copies up to num characters from the source string to the destination string.
đ Example
Let's delve into an example to illustrate how the strncpy()
function works.
#include <iostream>
#include <cstring>
int main() {
const char * source = "Hello, World!";
char destination[20]; // Allocate space for the destination string
// Copy up to 10 characters from source to destination
strncpy(destination, source, 10);
// Null-terminate the destination string to ensure proper printing
destination[10] = '\0';
// Output the result
std::cout << "Copied String: " << destination << std::endl;
return 0;
}
đģ Output
Copied String: Hello, Wor
đ§ How the Program Works
In this example, the strncpy()
function copies up to 10 characters from the source string "Hello, World!" to the destination string.
âŠī¸ Return Value
The strncpy()
function returns a pointer to the destination string.
đ Common Use Cases
The strncpy()
function is useful when you need to copy a specific number of characters from one string to another. It provides control over the number of characters copied, which can be beneficial in scenarios where you want to limit the length of the destination string.
đ Notes
- The
strncpy()
function does not guarantee null-termination of the destination string if the number of characters copied equals num. It's recommended to null-terminate the destination string manually if needed.
đĸ Optimization
When using strncpy()
, be mindful of null-terminating the destination string appropriately, especially if the number of characters copied equals num. Additionally, consider alternatives like std::string if dynamic memory allocation and null-termination are handled automatically.
đ Conclusion
The strncpy()
function in C++ is a valuable tool for copying a specified number of characters between strings. Understanding its usage and nuances is essential for effective string manipulation.
Feel free to experiment with different source and destination strings, and explore the behavior of the strncpy()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
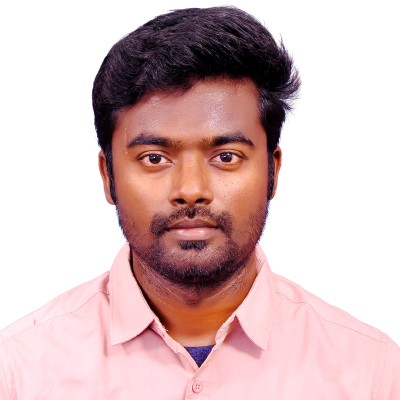
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String strncpy() Function), please comment here. I will help you immediately.