.webp)
C++ String strncat() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C++ programming, manipulating strings is a common task, and the Standard Template Library (STL) provides various functions to perform these operations efficiently.
The strncat()
function is part of the C++ Standard Library and is used to concatenate a specified number of characters from one string to another.
In this tutorial, we'll explore the usage and functionality of the strncat()
function.
đĄ Syntax
The signature of the strncat()
function is as follows:
char* strncat(char* destination, const char* source, size_t num);
This function appends the first num characters of the source string to the end of the destination string.
đ Example
Let's dive into an example to illustrate how the strncat()
function works.
#include <iostream>
#include <cstring>
int main() {
char destination[20] = "Hello, ";
const char * source = "World!";
// Concatenate the first 6 characters from source to destination
strncat(destination, source, 6);
// Output the concatenated string
std::cout << "Concatenated String: " << destination << std::endl;
return 0;
}
đģ Output
Concatenated String: Hello, World!
đ§ How the Program Works
In this example, the strncat()
function concatenates the first 6 characters from the source string to the end of the destination string.
âŠī¸ Return Value
The strncat()
function returns a pointer to the modified destination string.
đ Common Use Cases
The strncat()
function is useful when you need to concatenate a specified number of characters from one string to another, preventing buffer overflows and ensuring controlled string concatenation.
đ Notes
- Ensure that the size of the destination array is large enough to accommodate the concatenated result. Failure to do so may result in undefined behavior.
đĸ Optimization
The strncat()
function is optimized for efficiency. However, as a general practice, always ensure that you are working with properly sized buffers to avoid memory-related issues.
đ Conclusion
The strncat()
function in C++ is a powerful tool for controlled string concatenation. It provides a flexible and efficient way to concatenate a specified number of characters from one string to another.
Feel free to experiment with different strings and explore the behavior of the strncat()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
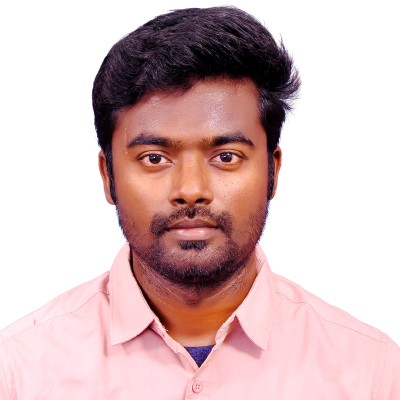
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String strncat() Function), please comment here. I will help you immediately.