.webp)
C++ String strcoll() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C++ programming, comparing and sorting strings based on locale-specific collation order is a common task.
The strcoll()
function is part of the C++ Standard Library and is designed for this purpose. It performs a locale-specific string comparison, taking into account cultural and linguistic differences.
In this tutorial, we'll explore the usage and functionality of the strcoll()
function.
đĄ Syntax
The signature of the strcoll()
function is as follows:
int strcoll(const char* str1, const char* str2);
This function takes two strings, str1 and str2, and performs a locale-specific collation.
đ Example
Let's delve into an example to illustrate how the strcoll()
function works.
#include <iostream>
#include <cstring>
#include <clocale>
int main() {
// Set the locale to the user's default
std::setlocale(LC_COLLATE, "");
const char * str1 = "apple";
const char * str2 = "Orange";
// Compare strings using strcoll()
int result = std::strcoll(str1, str2);
// Output the result
if (result < 0) {
std::cout << "String 1 is less than String 2 in collation order.\n";
} else if (result > 0) {
std::cout << "String 1 is greater than String 2 in collation order.\n";
} else {
std::cout << "String 1 is equal to String 2 in collation order.\n";
}
return 0;
}
đģ Output
String 1 is less than String 2 in collation order.
đ§ How the Program Works
In this example, the strcoll()
function compares the strings "apple" and "Orange" based on locale-specific collation and prints the result.
âŠī¸ Return Value
The strcoll()
function returns an integer less than, equal to, or greater than zero if str1 is found, respectively, to be less than, to match, or be greater than str2. The comparison is based on locale-specific collation.
đ Common Use Cases
The strcoll()
function is particularly useful when sorting strings or comparing them in a locale-sensitive manner. It takes into account language and cultural differences, making it suitable for internationalization and localization scenarios.
đ Notes
- Ensure that you set the desired locale using std::setlocale() before using
strcoll()
. The default locale is often suitable for most cases.
đĸ Optimization
The strcoll()
function is optimized for locale-specific string comparison. However, for performance-critical applications, you may want to explore alternative approaches or consider implementing your optimized version based on specific requirements.
đ Conclusion
The strcoll()
function in C++ is a valuable tool for locale-specific string comparison. It enhances the flexibility and internationalization capabilities of your C++ programs, making them more versatile in different language and cultural contexts.
Feel free to experiment with different strings and locales to explore the behavior of the strcoll()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
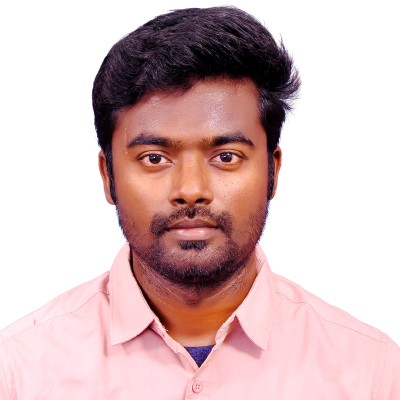
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String strcoll() Function), please comment here. I will help you immediately.