.webp)
C++ String strcspn() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C++ programming, working with strings is a common task, and the Standard Template Library (STL) provides various functions for efficient string manipulation.
The strcspn()
function is one such function, and it is part of the <cstring> header.
This function is used to find the length of the initial segment of a string that does not contain any characters from a specified set.
In this tutorial, we'll explore the usage and functionality of the strcspn()
function in C++.
đĄ Syntax
The signature of the strcspn()
function is as follows:
size_t strcspn(const char *str1, const char *str2);
This function takes two parameters:
- str1: A pointer to the null-terminated string to be examined.
- str2: A pointer to the null-terminated string containing the characters to match.
đ Example
Let's delve into an example to illustrate how the strcspn()
function works.
#include <iostream>
#include <cstring>
int main() {
const char * text = "C++ Programming";
// Find the length of the initial segment without characters from "aeiou"
size_t length = strcspn(text, "aeiou");
// Output the result
std::cout << "Length of the initial segment without vowels: " << length << std::endl;
return 0;
}
đģ Output
Length of the initial segment without vowels: 6
đ§ How the Program Works
In this example, the strcspn()
function is used to find the length of the initial segment of the string "C++ Programming" that does not contain any vowels ('a', 'e', 'i', 'o', 'u').
âŠī¸ Return Value
The strcspn()
function returns the length of the initial segment of the string str1 that does not contain any characters from the string str2.
đ Common Use Cases
The strcspn()
function is useful when you need to identify the length of the prefix of a string that does not contain specific characters. It is commonly used in tasks such as parsing or filtering strings based on a defined set of characters.
đ Notes
- The length returned by
strcspn()
is the number of characters before the first occurrence of any character from str2 in str1.
đĸ Optimization
The strcspn()
function is already optimized for efficient string processing. However, for very large strings or performance-critical applications, consider profiling your code and exploring alternative approaches if needed.
đ Conclusion
The strcspn()
function in C++ is a powerful tool for finding the length of the initial segment of a string without specific characters. It enhances the efficiency and readability of code when dealing with string manipulation tasks.
Feel free to experiment with different strings and character sets to explore the behavior of the strcspn()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
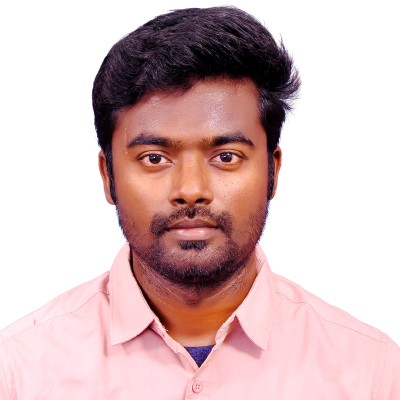
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String strcspn() Function), please comment here. I will help you immediately.