.webp)
C++ String find() Function
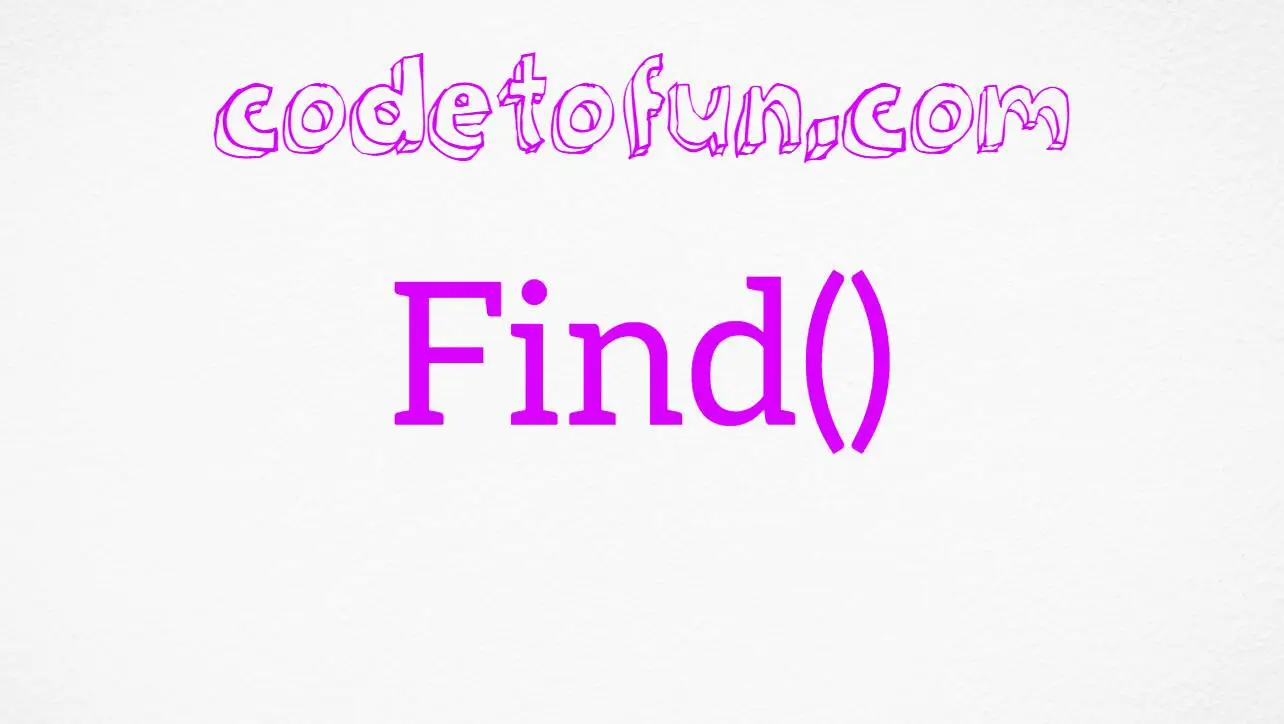
Photo Credit to CodeToFun
đ Introduction
In C++ programming, working with strings is a common and essential task.
The find()
function is a member of the std::string class and is used to search for a substring within a string.
In this tutorial, we'll explore the usage and functionality of the find()
function in C++.
đĄ Syntax
The syntax for the find()
function is as follows:
size_t find(const std::string& str, size_t pos = 0) const noexcept;
- str: The substring to search for within the string.
- pos (optional): The position in the string to start the search. If not specified, the search starts from the beginning of the string.
đ Example
Let's dive into some examples to illustrate how the find()
function works.
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, C++!";
// Find the position of the substring "C++"
size_t position = text.find("C++");
// Output the result
if (position != std::string::npos) {
std::cout << "Substring found at position: " << position << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
đģ Output
Substring found at position: 7
đ§ How the Program Works
In this example, the find()
function searches for the substring "C++" within the string "Hello, C++!" and prints the result.
âŠī¸ Return Value
The find()
function returns the position of the first character of the first occurrence of the substring in the string. If the substring is not found, it returns std::string::npos.
đ Common Use Cases
The find()
function is useful when you need to determine whether a substring exists in a string and, if so, where it is located. It's commonly used in tasks such as parsing and manipulating strings.
đ Notes
- The search is case-sensitive. If you need a case-insensitive search, consider converting the string and the substring to lowercase (or uppercase) before calling
find()
. - To find multiple occurrences of a substring, you can use a loop and update the starting position in each iteration.
đĸ Optimization
The find()
function is optimized for efficiency, but for large strings and frequent searches, consider optimizing by using more specialized algorithms if needed.
đ Conclusion
The find()
function in C++ is a powerful tool for searching for substrings within strings. It provides flexibility with optional parameters and is a valuable asset in string manipulation tasks.
Feel free to experiment with different strings and substrings to deepen your understanding of the find()
function. Happy coding!
đ¨âđģ Join our Community:
Author
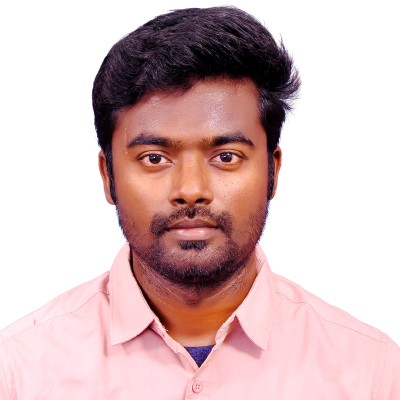
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String find() Function), please comment here. I will help you immediately.