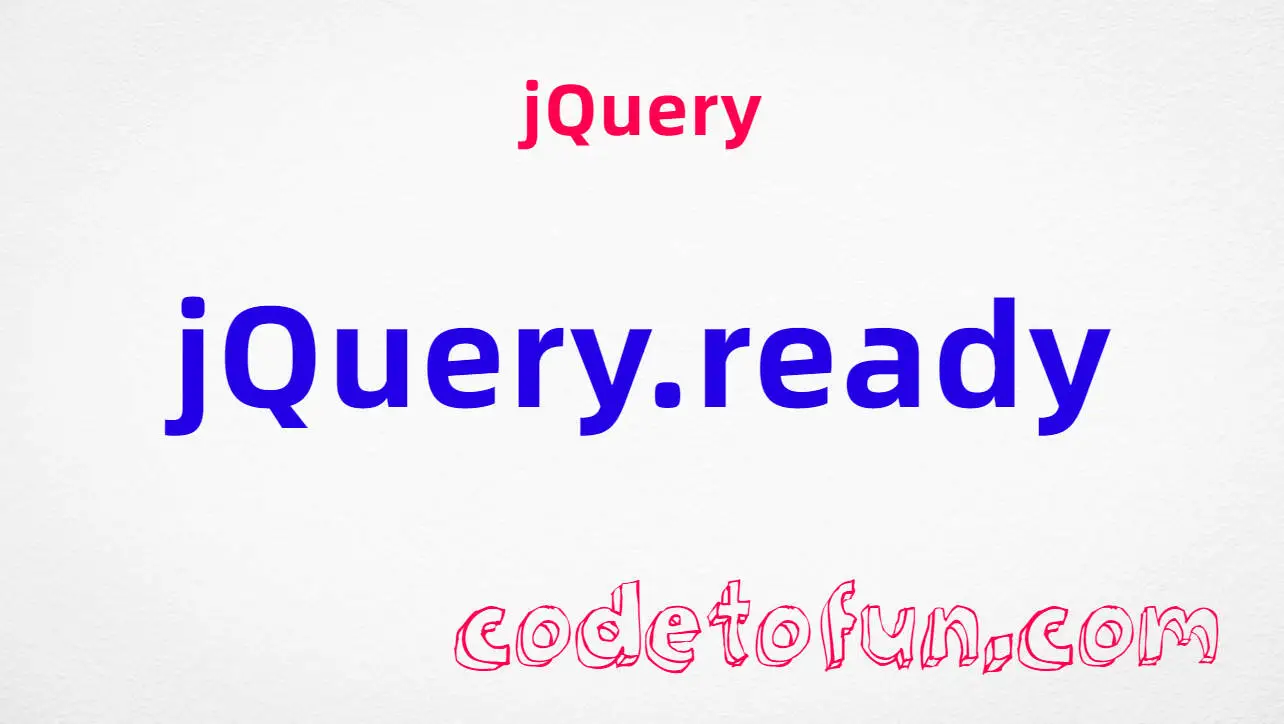
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery jQuery.htmlPrefilter() Method

Photo Credit to CodeToFun
🙋 Introduction
The jQuery library provides a variety of methods to manipulate HTML content dynamically and efficiently. One such method is jQuery.htmlPrefilter()
, which allows developers to filter HTML strings before they are set in the DOM. This method is particularly useful for pre-processing HTML content to ensure it meets specific criteria or to sanitize input.
In this guide, we'll explore the jQuery.htmlPrefilter()
method, its syntax, and practical examples to help you understand how to use it effectively.
🧠 Understanding jQuery.htmlPrefilter() Method
The jQuery.htmlPrefilter()
method allows you to define a function that modifies the HTML string before it is set to the DOM. This method can be overridden to customize how jQuery handles HTML content, providing an extra layer of control over the insertion of HTML.
💡 Syntax
The syntax for the jQuery.htmlPrefilter()
method is straightforward:
jQuery.htmlPrefilter(function(html) {
// Modify the HTML string here
return modifiedHtml;
});
Parameters:
- html: The original HTML string that will be processed.
Return Value:
Returns the modified HTML string.
📝 Example
Basic Usage:
You can use the
jQuery.htmlPrefilter()
method to modify HTML content before it is inserted into the DOM. For example, you can remove script tags from the HTML string to prevent script execution:example.jsCopiedjQuery.htmlPrefilter(function(html) { return html.replace(/<script[^>]*>([\S\s]*?)<\/script>/gmi, ''); }); // Usage example $("#content").html('<div>Hello World</div><script>alert("Hello!");</script>'); // The script tag will be removed, and only the div will be inserted.
Sanitizing HTML Input:
You can sanitize user input to prevent the injection of unwanted HTML elements. This is especially useful for mitigating security risks like Cross-Site Scripting (XSS):
example.jsCopiedjQuery.htmlPrefilter(function(html) { // Remove all potentially harmful elements return html.replace(/<\/?[^>]+(>|$)/g, ""); }); // Usage example $("#userInput").html('<b>User</b> input with <i>tags</i>'); // All HTML tags will be removed, and only plain text will be inserted.
Customizing HTML Content:
You can customize HTML content by adding or altering elements before they are inserted into the DOM. For example, adding a specific class to all paragraph elements:
example.jsCopiedjQuery.htmlPrefilter(function(html) { return html.replace(/<p>/g, '<p class="custom-class">'); }); // Usage example $("#content").html('<p>This is a paragraph.</p>'); // The paragraph will have the class 'custom-class'.
Combining with Other jQuery Methods:
The
jQuery.htmlPrefilter()
method can be combined with other jQuery methods to achieve more complex manipulations. For example, you can use it alongside .append() or .prepend() for consistent HTML filtering across different insertion methods:example.jsCopiedjQuery.htmlPrefilter(function(html) { return html.replace(/<img[^>]*>/g, ''); }); // Usage example $("#content").append('<p>Image: <img src="image.jpg"></p>'); // The image tag will be removed before the content is appended.
🎉 Conclusion
The jQuery.htmlPrefilter()
method is a powerful tool for pre-processing HTML strings before they are inserted into the DOM.
By mastering this method, you can sanitize input, customize content, and enhance the security of your web applications. Understanding and utilizing jQuery.htmlPrefilter()
allows for greater control over HTML content manipulation, ensuring a more robust and secure web development process.
👨💻 Join our Community:
Author
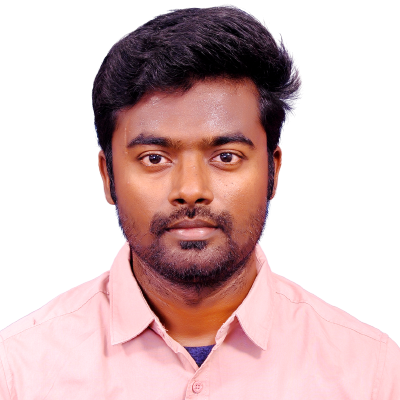
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.htmlPrefilter() Method), please comment here. I will help you immediately.