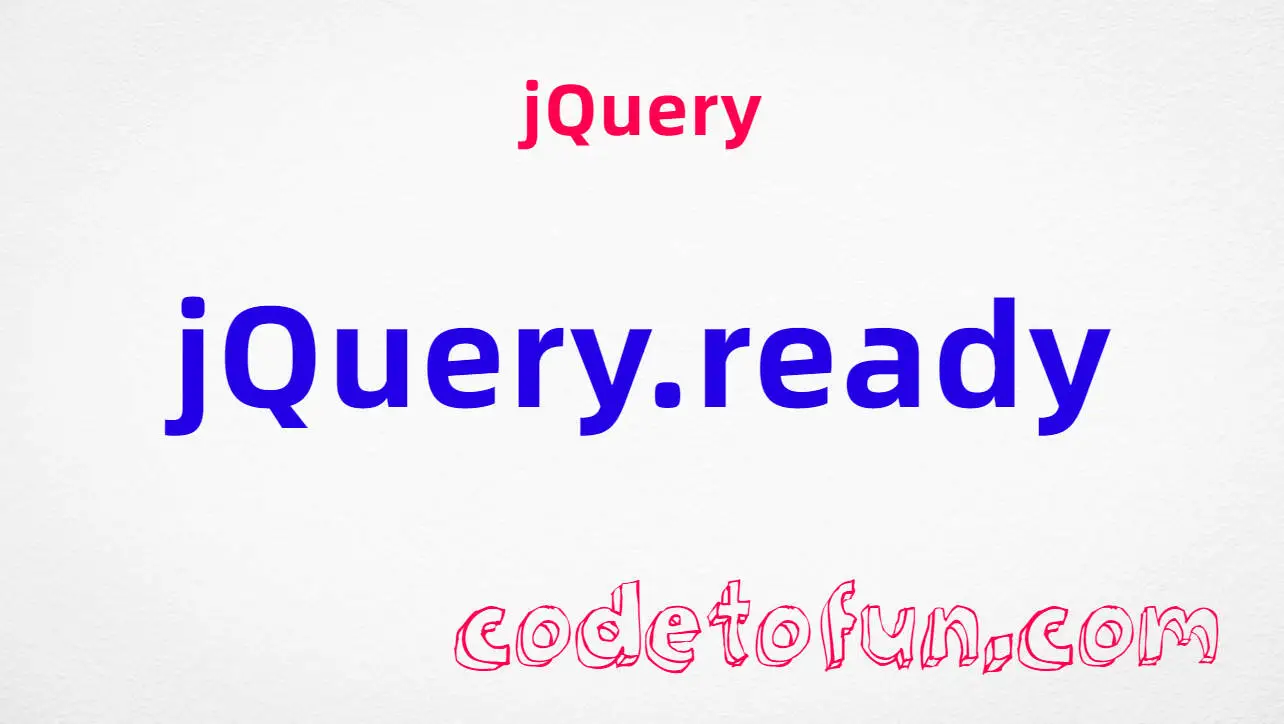
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery jQuery.holdReady() Method

Photo Credit to CodeToFun
🙋 Introduction
jQuery offers numerous methods to control the execution flow of scripts, ensuring that your web page behaves as expected. One such method is jQuery.holdReady()
, which allows you to delay the execution of the $(document).ready() event. This can be particularly useful when you need to wait for other scripts to load or perform some setup tasks before allowing the rest of the code to run.
In this guide, we'll explore the jQuery.holdReady()
method in detail, complete with examples to illustrate its practical use.
🧠 Understanding jQuery.holdReady() Method
The jQuery.holdReady()
method is used to hold or release the execution of the $(document).ready() event. By delaying this event, you can ensure that all necessary resources or scripts are loaded before your main jQuery code executes.
💡 Syntax
The syntax for the jQuery.holdReady()
method is straightforward:
jQuery.holdReady(hold)
Parameters:
- hold: A boolean value.
true
to hold the execution,false
to release it.
📝 Example
Holding the ready Event:
To demonstrate the basic use of
jQuery.holdReady()
, let's see how to hold the ready event until another script has been loaded.example.jsCopied<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> jQuery.holdReady(true); // Simulate loading another script setTimeout(function() { console.log("Additional script loaded."); jQuery.holdReady(false); // Release the hold }, 3000); $(document).ready(function() { console.log("Document is ready!"); }); </script>
In this example, the $(document).ready() event will be delayed by 3 seconds to simulate the loading of an additional script.
Ensuring All Dependencies Are Loaded:
If your project relies on multiple scripts that must be loaded in a specific order,
jQuery.holdReady()
can help manage this sequence.example.jsCopied<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> jQuery.holdReady(true); // Load external scripts $.getScript("https://example.com/extra-script.js", function() { console.log("Extra script loaded."); jQuery.holdReady(false); // Release the hold after the script is loaded }); $(document).ready(function() { console.log("Document is ready with all dependencies."); }); </script>
Here, the $(document).ready() event will only fire after the external script has been loaded, ensuring all dependencies are available.
Conditional Loading:
Sometimes, you might need to conditionally hold the ready event based on certain conditions.
example.jsCopied<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> var shouldHoldReady = true; if (shouldHoldReady) { jQuery.holdReady(true); // Simulate an asynchronous condition check setTimeout(function() { console.log("Condition met, releasing hold."); jQuery.holdReady(false); // Release the hold based on condition }, 2000); } $(document).ready(function() { console.log("Document is ready, conditionally."); }); </script>
In this example, the ready event is conditionally held and released based on a simulated asynchronous condition check.
🎉 Conclusion
The jQuery.holdReady()
method is a powerful tool for managing the execution timing of your jQuery code. By holding the $(document).ready() event, you can ensure that all necessary resources and scripts are loaded before your main jQuery code runs, leading to more reliable and predictable behavior in your web applications.
Mastering this method will give you greater control over the initialization process of your web pages, making it an essential technique for any advanced jQuery developer.
👨💻 Join our Community:
Author
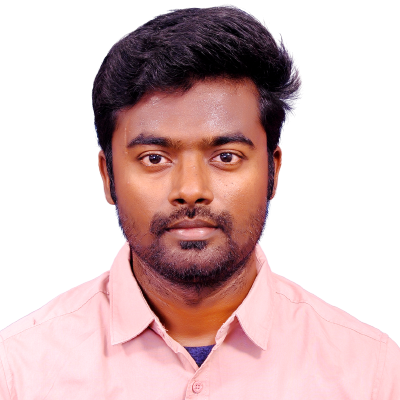
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.holdReady() Method), please comment here. I will help you immediately.