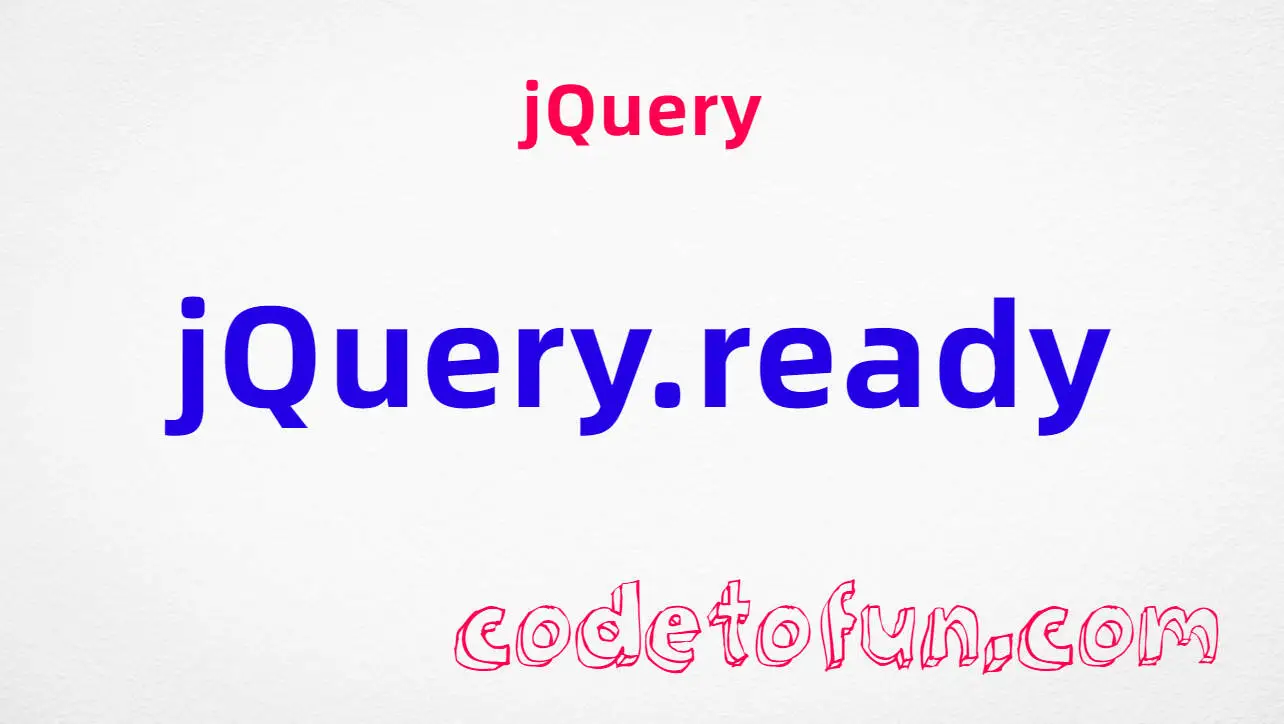
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery jQuery.fx.interval Property

Photo Credit to CodeToFun
🙋 Introduction
jQuery provides a suite of tools for creating smooth and responsive animations on your web pages. One such tool is the jQuery.fx.interval
property, which allows you to control the timing of animations. By adjusting this property, you can fine-tune the performance and smoothness of your animations to suit your needs.
In this guide, we'll explore the jQuery.fx.interval
property, its usage, and practical examples to help you understand how to leverage it effectively.
🧠 Understanding jQuery.fx.interval Property
The jQuery.fx.interval
property defines the frequency, in milliseconds, at which jQuery's internal animation timer ticks. By default, this value is set to 13, which means that the animations update approximately every 13 milliseconds. Adjusting this value can affect the smoothness and performance of animations on your web page.
💡 Syntax
The syntax for the jQuery.fx.interval
property is straightforward:
jQuery.fx.interval = value;
Where value is the number of milliseconds between each animation frame.
📝 Example
Increasing the Interval for Better Performance:
If you have complex animations that are causing performance issues, you can increase the interval to reduce the load on the browser. For example:
example.jsCopiedjQuery.fx.interval = 20;
This sets the animation update interval to 20 milliseconds, which can help improve performance by reducing the frequency of updates.
Decreasing the Interval for Smoother Animations:
For smoother and more fluid animations, you might want to decrease the interval. However, be cautious as this can increase the load on the browser. For instance:
example.jsCopiedjQuery.fx.interval = 10;
This sets the animation update interval to 10 milliseconds, potentially making animations appear smoother.
Fine-Tuning Animations for Different Devices:
Different devices have varying performance capabilities. On high-performance devices, you might want smoother animations:
example.jsCopiedif (highPerformanceDevice) { jQuery.fx.interval = 10; } else { jQuery.fx.interval = 20; }
This conditional adjustment ensures that animations are optimized based on the device's performance.
Debugging Animation Performance Issues:
If you're experiencing performance issues with animations, adjusting the
jQuery.fx.interval
can be a useful debugging tool. By increasing the interval, you can quickly determine if the issue is related to the frequency of animation updates:example.jsCopiedjQuery.fx.interval = 50; // Temporarily increase for debugging
This allows you to see if reducing the animation update frequency improves performance.
🎉 Conclusion
The jQuery.fx.interval
property is a powerful yet often overlooked tool for controlling the performance and smoothness of animations in jQuery. By understanding and adjusting this property, you can optimize your animations to run more efficiently across various devices and scenarios. Whether you're looking to enhance performance, achieve smoother animations, or debug issues, the jQuery.fx.interval
property provides a flexible solution.
By mastering this property, you can create more responsive and visually appealing web pages.
👨💻 Join our Community:
Author
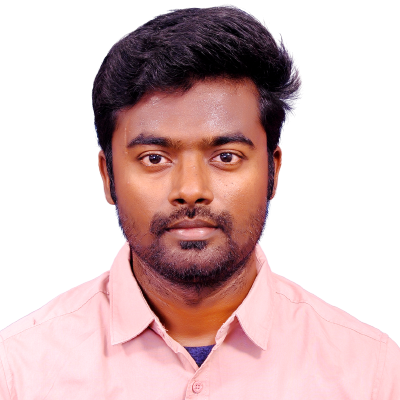
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.fx.interval Property), please comment here. I will help you immediately.