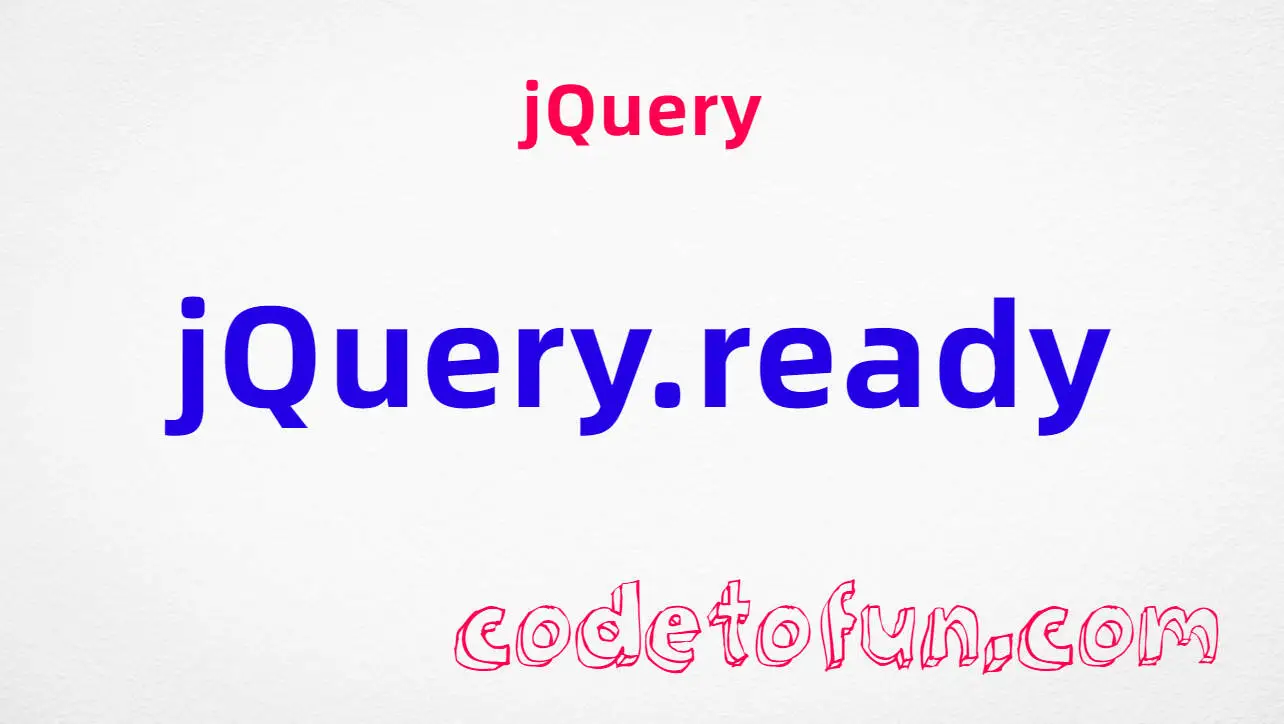
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery jQuery.ajaxSetup() Method

Photo Credit to CodeToFun
🙋 Introduction
jQuery provides a variety of methods to facilitate AJAX (Asynchronous JavaScript and XML) interactions, enabling web applications to retrieve data from a server asynchronously without interfering with the display and behavior of the existing page. One powerful tool in jQuery's arsenal is the jQuery.ajaxSetup()
method. This method allows you to configure global AJAX settings, simplifying your code and ensuring consistency across multiple AJAX requests.
In this guide, we'll explore how to use jQuery.ajaxSetup()
effectively with clear examples to enhance your web development skills.
🧠 Understanding jQuery.ajaxSetup() Method
The jQuery.ajaxSetup()
method is used to set default values for future AJAX requests. By configuring global settings, you can avoid repetition and ensure that all AJAX requests adhere to a consistent configuration, making your code cleaner and more maintainable.
💡 Syntax
The syntax for the jQuery.ajaxSetup()
method is straightforward:
jQuery.ajaxSetup(options)
Parameters:
- options: An object containing key-value pairs to configure the default settings for AJAX requests.
📝 Example
Setting Default AJAX Options:
Suppose you frequently make AJAX requests to the same server and need to include certain headers or settings for each request. You can use
jQuery.ajaxSetup()
to set these defaults once:example.jsCopied$.ajaxSetup({ url: 'https://api.example.com/data', method: 'GET', dataType: 'json', headers: { 'Authorization': 'Bearer YOUR_API_KEY' } });
This configuration ensures that every AJAX request you make will use the specified URL, method, data type, and headers unless overridden.
Making an AJAX Request with Default Settings:
With the default settings configured, making an AJAX request becomes simpler:
example.jsCopied$.ajax({ success: function(response) { console.log(response); } });
This request will automatically use the defaults set by $.ajaxSetup().
Overriding Default Settings:
If you need to override the default settings for a specific request, you can do so by passing the new settings directly into the $.ajax() method:
example.jsCopied$.ajax({ url: 'https://api.example.com/other-data', method: 'POST', data: { key: 'value' }, success: function(response) { console.log(response); } });
This request will use the new URL and method specified, while other settings will still fall back to the defaults.
Handling Global AJAX Events:
Using $.ajaxSetup(), you can also set global event handlers for AJAX requests, such as beforeSend, complete, and error. This is useful for adding uniform behavior across all AJAX requests:
example.jsCopied$.ajaxSetup({ beforeSend: function() { console.log('AJAX request is about to be sent'); }, complete: function() { console.log('AJAX request completed'); }, error: function(jqXHR, textStatus, errorThrown) { console.error('AJAX request failed:', textStatus, errorThrown); } });
Resetting AJAX Settings:
If you need to reset the AJAX settings to their defaults at any point, you can call $.ajaxSetup({}) with an empty object:
example.jsCopied$.ajaxSetup({});
This will clear any settings previously defined.
🎉 Conclusion
The jQuery jQuery.ajaxSetup()
method is an invaluable tool for setting default configurations for AJAX requests. By using this method, you can ensure consistency across your AJAX interactions, reduce repetitive code, and handle global events efficiently.
Mastering jQuery.ajaxSetup()
will streamline your AJAX implementation, making your web applications more robust and easier to maintain.
👨💻 Join our Community:
Author
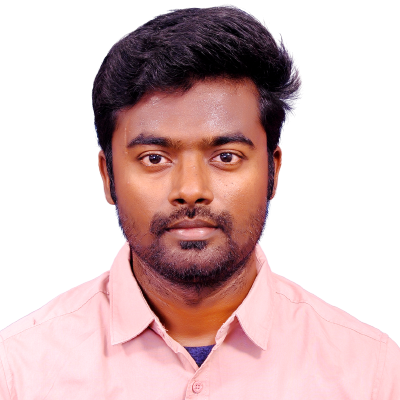
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.ajaxSetup() Method), please comment here. I will help you immediately.