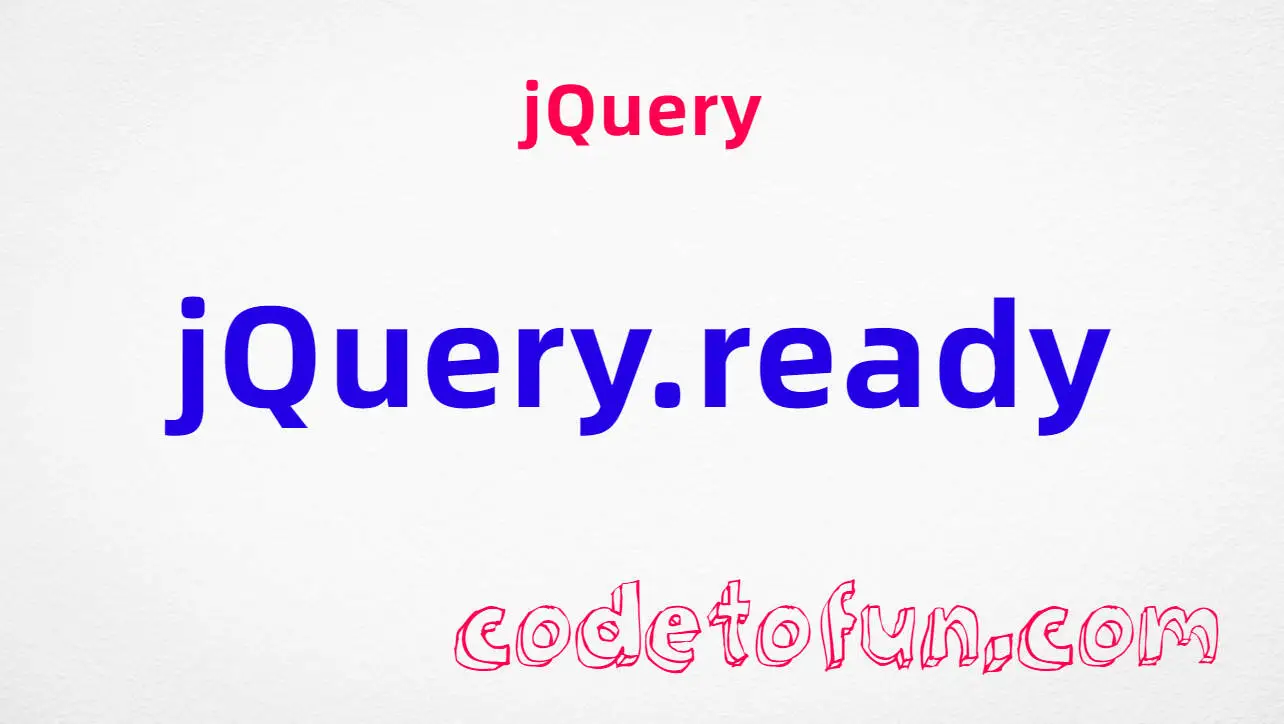
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery jQuery.ajax() Method

Photo Credit to CodeToFun
🙋 Introduction
jQuery's jQuery.ajax()
method is a powerful tool that allows developers to perform asynchronous HTTP (Ajax) requests easily. This method is highly versatile, enabling you to send data to a server, retrieve data from a server, and handle the server’s response without reloading the web page.
In this guide, we'll explore the jQuery.ajax()
method in detail, including its syntax, common use cases, and practical examples to help you effectively implement Ajax functionality in your web projects.
🧠 Understanding jQuery.ajax() Method
The jQuery.ajax()
method provides a way to perform asynchronous HTTP requests using JavaScript. This method offers numerous configuration options, making it adaptable to various needs, such as setting the request type, specifying headers, handling different data types, and managing success or error callbacks.
💡 Syntax
The syntax for the jQuery.ajax()
method is straightforward:
$.ajax({
url: "URL",
type: "GET/POST/PUT/DELETE",
data: {
// data to be sent to the server
},
success: function(response) {
// handle successful response
},
error: function(xhr, status, error) {
// handle errors
}
});
📝 Example
Performing a GET Request:
A GET request retrieves data from a specified URL. Here's an example of performing a GET request using
jQuery.ajax()
:example.jsCopied$.ajax({ url: "https://api.example.com/data", type: "GET", success: function(response) { console.log("Data retrieved: ", response); }, error: function(xhr, status, error) { console.error("Error fetching data: ", error); } });
This code sends a GET request to https://api.example.com/data and logs the retrieved data or any errors to the console.
Sending Data with a POST Request:
A POST request sends data to a server. Here’s how to send data using
jQuery.ajax()
:example.jsCopied$.ajax({ url: "https://api.example.com/submit", type: "POST", data: { name: "John Doe", age: 30 }, success: function(response) { console.log("Data submitted successfully: ", response); }, error: function(xhr, status, error) { console.error("Error submitting data: ", error); } });
This code sends a POST request to https://api.example.com/submit with name and age data, and logs the server’s response or any errors.
Handling JSON Data:
When dealing with JSON data, it's essential to specify the data type. Here’s an example:
example.jsCopied$.ajax({ url: "https://api.example.com/user", type: "GET", dataType: "json", success: function(response) { console.log("User data: ", response); }, error: function(xhr, status, error) { console.error("Error fetching user data: ", error); } });
This code sends a GET request to https://api.example.com/user, expects a JSON response, and handles it accordingly.
Setting Custom Headers:
You may need to send custom headers with your request. Here’s how to set them using
jQuery.ajax()
:example.jsCopied$.ajax({ url: "https://api.example.com/data", type: "GET", headers: { "Authorization": "Bearer YOUR_ACCESS_TOKEN" }, success: function(response) { console.log("Data retrieved: ", response); }, error: function(xhr, status, error) { console.error("Error fetching data: ", error); } });
This code sends a GET request with an authorization header for authentication purposes.
Using Promises:
The
jQuery.ajax()
method returns a promise, which can be used for more complex asynchronous operations. Here's an example:example.jsCopied$.ajax({ url: "https://api.example.com/data", type: "GET" }).done(function(response) { console.log("Data retrieved: ", response); }).fail(function(xhr, status, error) { console.error("Error fetching data: ", error); });
This approach uses done() and fail() methods to handle success and error cases, respectively.
🎉 Conclusion
The jQuery.ajax()
method is a powerful and flexible tool for making asynchronous HTTP requests. Whether you need to retrieve data, submit forms, handle JSON, or manage authentication headers, this method provides a robust solution.
By understanding its various options and configurations, you can enhance your web applications with seamless and dynamic server interactions.
👨💻 Join our Community:
Author
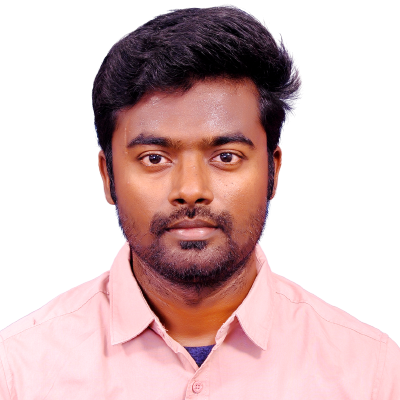
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.ajax() Method), please comment here. I will help you immediately.