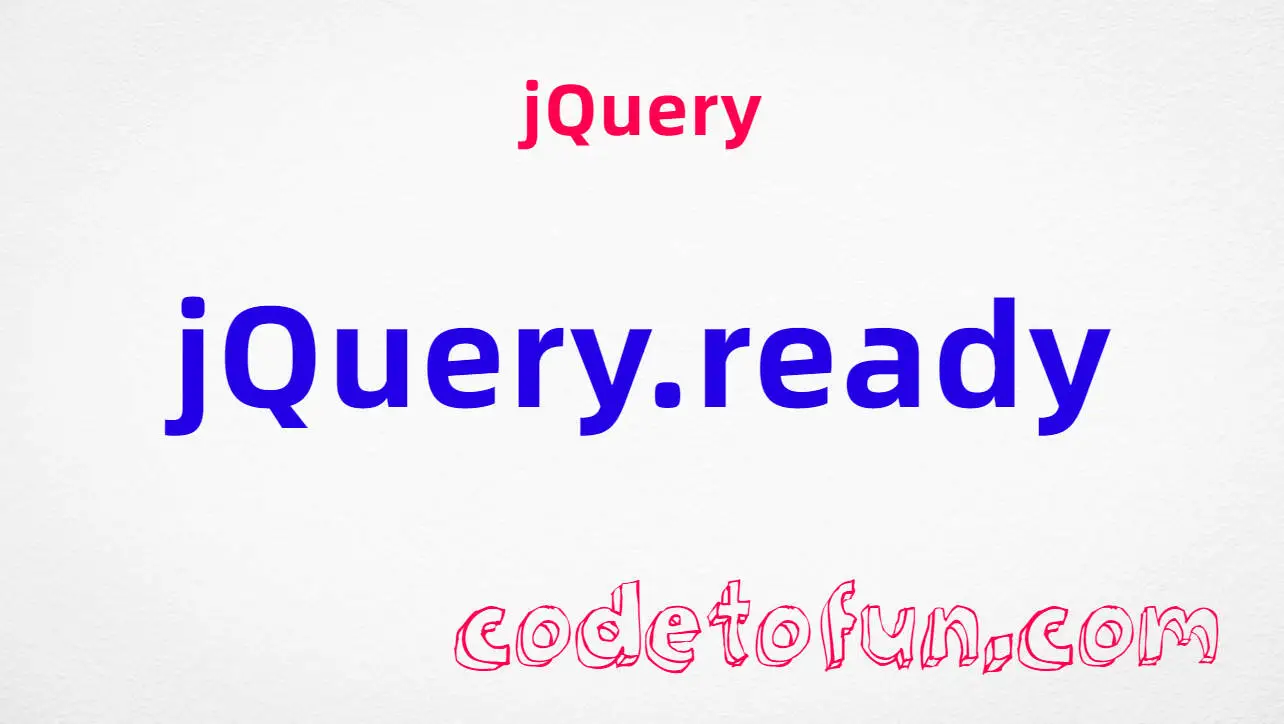
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery event.result Property

Photo Credit to CodeToFun
🙋 Introduction
jQuery provides developers with a rich set of tools for handling events in web applications. One such tool is the event.result
property, which plays a crucial role in event handling by allowing you to access the return value of event handlers. Understanding how to utilize this property effectively can significantly enhance your ability to manage event-driven interactions within your web pages.
In this guide, we'll explore the event.result
property in detail, providing clear examples to illustrate its usage and benefits.
🧠 Understanding event.result Property
The event.result
property provides access to the return value of the last handler executed for an event. It allows you to retrieve data or status information from event handlers, enabling you to make decisions or perform further actions based on the outcome of these handlers.
💡 Syntax
The syntax for the event.result
property is straightforward:
event.result
📝 Example
Accessing Return Values of Event Handlers:
Suppose you have a button click event handler that returns a value indicating the success of an operation. You can use the
event.result
property to access this return value and take appropriate action based on it:example.jsCopied$("#submitButton").click(function(event) { // Perform some operation var operationResult = performOperation(); // Return operation result return operationResult; }); $("#submitButton").click(function(event) { var result = event.result; if (result === true) { alert("Operation successful!"); } else { alert("Operation failed!"); } });
In this example, the second click event handler retrieves the return value of the first handler using
event.result
and displays an appropriate message based on the result.Enhancing Event-driven Interactions:
The
event.result
property can be particularly useful for enhancing event-driven interactions in your web applications. For instance, you can use it to determine whether to proceed with a form submission based on validation results:example.jsCopied$("form").submit(function(event) { // Perform form validation var isValid = validateForm(); // Return validation result return isValid; }); $("form").submit(function(event) { var isValid = event.result; if (!isValid) { event.preventDefault(); // Prevent form submission if validation fails alert("Please correct the errors in the form."); } });
Here, the second submit event handler checks the validation result retrieved via
event.result
and prevents the form submission if validation fails.
🎉 Conclusion
The jQuery event.result
property is a powerful tool for accessing the return values of event handlers, enabling you to make informed decisions and take appropriate actions based on the outcome of these handlers.
Whether you need to process the results of asynchronous operations, validate user input, or manage other event-driven interactions, understanding and leveraging the event.result
property can greatly enhance the functionality and usability of your web applications.
👨💻 Join our Community:
Author
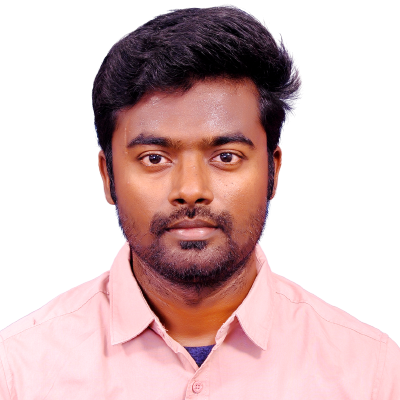
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery event.result Property), please comment here. I will help you immediately.