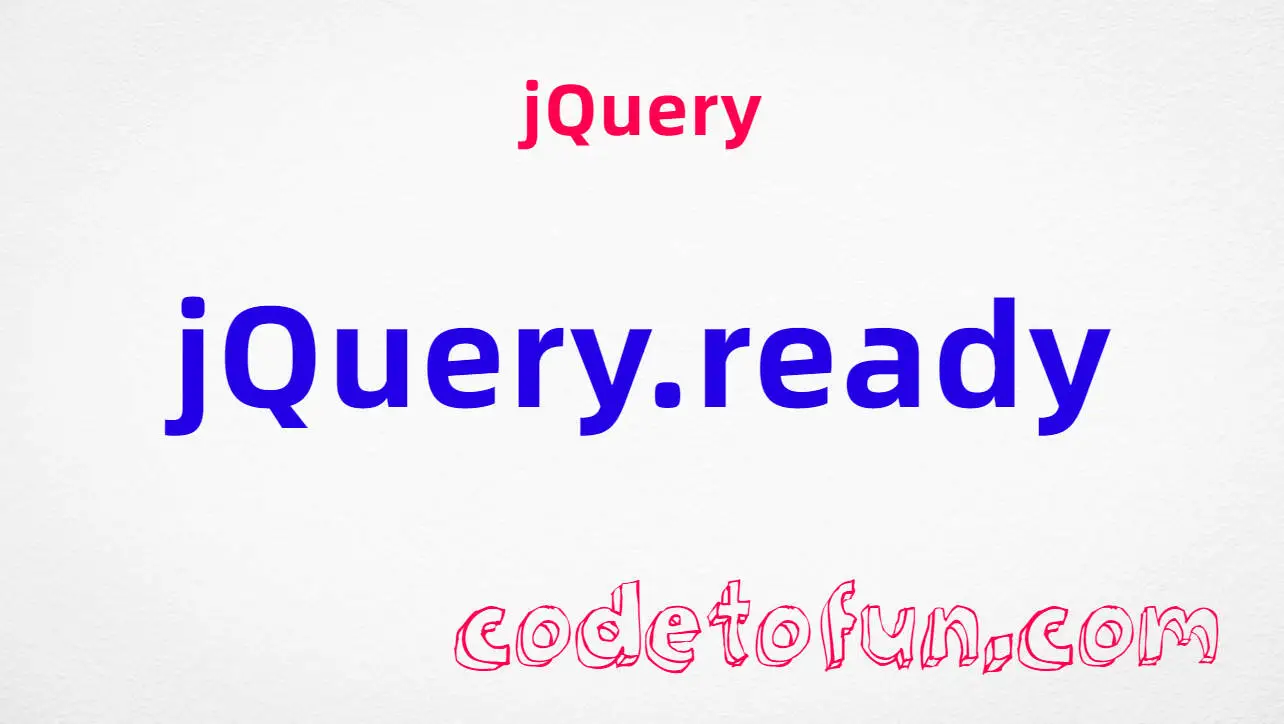
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery event.pageY Property

Photo Credit to CodeToFun
π Introduction
jQuery offers a wide array of properties and methods to enhance web development, particularly for handling events. One such property is event.pageY
, which provides the vertical coordinate of an event relative to the whole document. This property is particularly useful for tracking mouse movements and interactions on a webpage.
In this guide, we'll explore the event.pageY
property with clear examples to help you leverage its potential in your projects.
π§ Understanding event.pageY Property
The event.pageY
property returns the vertical position of the mouse pointer when an event is triggered, measured in pixels from the top edge of the document. This property is part of the event object in jQuery, which contains information about the event that was triggered.
π‘ Syntax
The syntax for the event.pageY
property is straightforward:
event.pageY
π Example
Getting the Vertical Position of a Click:
Suppose you want to display the vertical position where a user clicks on the document. You can use the
event.pageY
property to achieve this:index.htmlCopied<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>event.pageY Example</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <h1>Click anywhere on the document</h1> <script> $(document).click(function(event) { alert("Vertical position: " + event.pageY + "px"); }); </script> </body> </html>
This script will display an alert showing the vertical position in pixels whenever the user clicks anywhere on the document.
Tracking Mouse Movement:
You can use the
event.pageY
property to track the vertical position of the mouse as it moves across the document. Hereβs an example:index.htmlCopied<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Track Mouse Movement</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <h1>Move your mouse around the document</h1> <p id="position"></p> <script> $(document).mousemove(function(event) { $("#position").text("Vertical position: " + event.pageY + "px"); }); </script> </body> </html>
This code will continuously update a paragraph element with the vertical position of the mouse as it moves.
Using event.pageY in a Drag and Drop Interface:
When creating a drag-and-drop interface, you might want to know the vertical position of the mouse to position elements correctly. Hereβs a basic example:
index.htmlCopied<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Drag and Drop Example</title> <style> #draggable { width: 100px; height: 100px; background-color: lightblue; position: absolute; } </style> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <div id="draggable">Drag me</div> <script> $("#draggable").on("mousedown", function(event) { $(document).on("mousemove.dragging", function(event) { $("#draggable").css({ top: event.pageY - 50 + "px" // Adjusting to center the element on the cursor }); }); }); $(document).on("mouseup", function() { $(document).off("mousemove.dragging"); }); </script> </body> </html>
This script allows a user to drag a div vertically, updating its position based on the
event.pageY
property.Combining event.pageY with Other Event Properties:
You can combine
event.pageY
with other properties like event.pageX (for horizontal position) to get comprehensive control over event handling. For example, you can determine the exact position of the mouse pointer in both vertical and horizontal axes.
π Conclusion
The jQuery event.pageY
property is a valuable tool for obtaining the vertical position of mouse events, which can be utilized in various interactive web applications. Whether you need to display click positions, track mouse movements, or create dynamic drag-and-drop interfaces, event.pageY
provides a straightforward solution.
By mastering this property, you can enhance the interactivity and responsiveness of your web pages.
π¨βπ» Join our Community:
Author
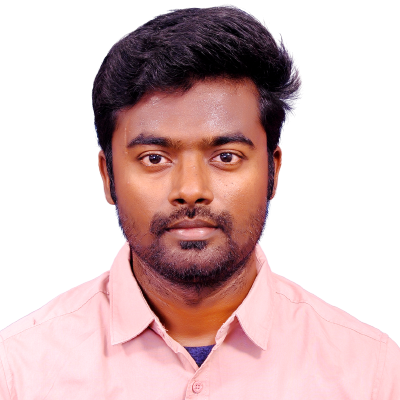
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery event.pageY Property), please comment here. I will help you immediately.