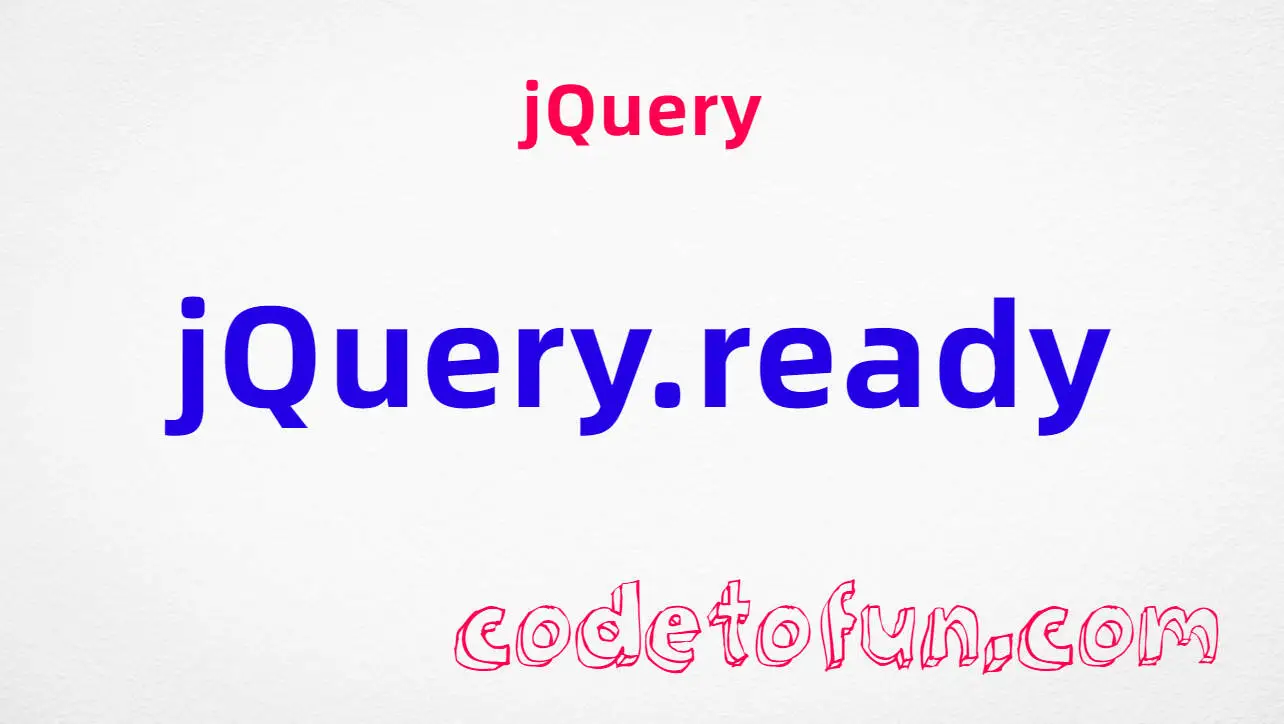
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery .ajaxStop() Method
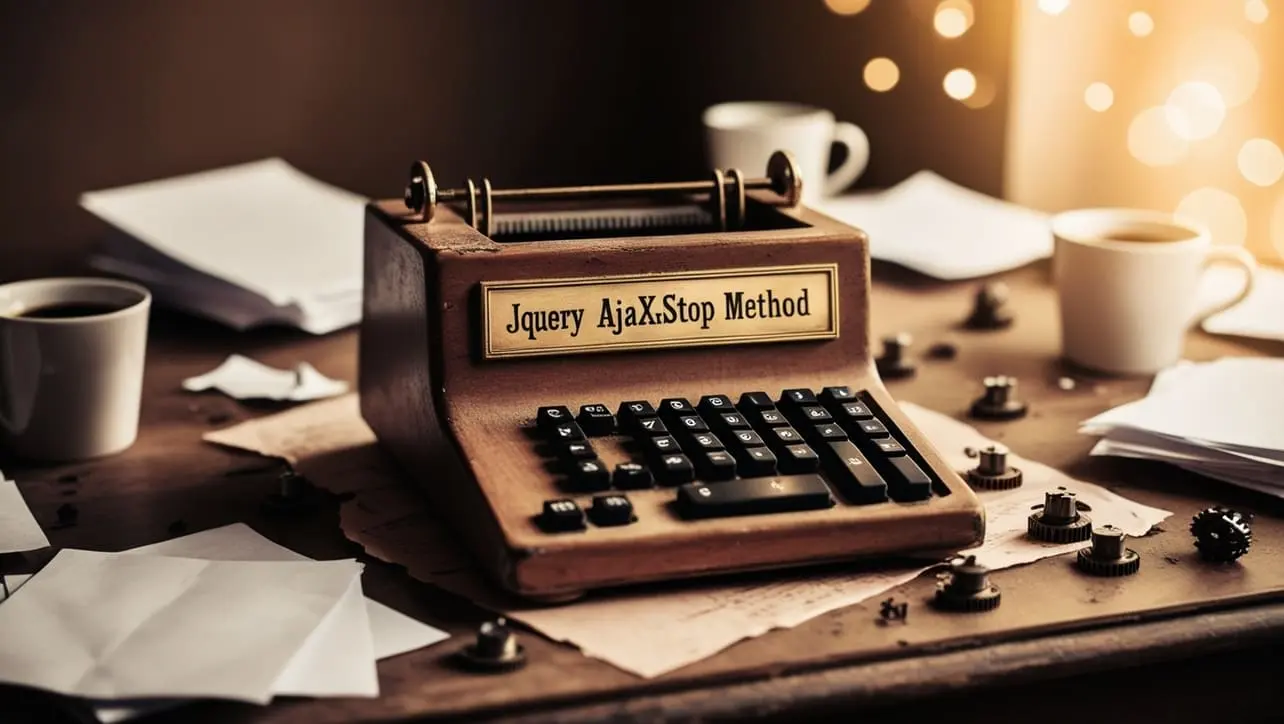
Photo Credit to CodeToFun
🙋 Introduction
In the world of web development, handling asynchronous operations effectively is crucial for creating smooth and responsive user experiences. jQuery provides a range of methods to manage AJAX calls, and one particularly useful method is jQuery.ajaxStop()
. This method allows you to execute a function when all AJAX requests have completed.
In this guide, we'll explore the jQuery.ajaxStop()
method in detail, with examples to help you integrate it into your projects seamlessly.
🧠 Understanding .ajaxStop() Method
The jQuery.ajaxStop()
method is an event handler that triggers a callback function when all AJAX requests have completed. This can be particularly useful for managing loading indicators, updating the UI, or performing any final tasks after multiple AJAX requests have finished.
💡 Syntax
The syntax for the .ajaxStop()
method is straightforward:
$(document).ajaxStop(function() {
// Your code here
});
📝 Example
Displaying a Loading Indicator:
One common use case for
jQuery.ajaxStop()
is to hide a loading indicator when all AJAX requests are done.index.htmlCopied<div id="loading">Loading...</div>
example.jsCopied$(document).ajaxStart(function() { $("#loading").show(); }).ajaxStop(function() { $("#loading").hide(); });
In this example, the loading indicator (#loading) is shown when an AJAX request starts and hidden when all AJAX requests have completed.
Updating the UI:
You might want to update certain parts of your UI once all AJAX requests are finished.
index.htmlCopied<div id="content">Content will be loaded here</div>
example.jsCopied$(document).ajaxStop(function() { $("#content").text("All AJAX requests completed."); });
This will change the text of the #content div to "All AJAX requests completed." once all AJAX requests are done.
Performing Cleanup Tasks:
You can use
jQuery.ajaxStop()
to perform cleanup tasks or reset variables after AJAX requests are finished.example.jsCopied$(document).ajaxStop(function() { // Reset variables or perform cleanup console.log("Cleanup tasks completed."); });
This will log a message to the console indicating that cleanup tasks have been completed.
Chaining with Other AJAX Events:
You can chain
jQuery.ajaxStop()
with other AJAX event methods like ajaxStart(), ajaxSend(), ajaxComplete(), etc., to have more granular control over your AJAX interactions.example.jsCopied$(document).ajaxStart(function() { console.log("AJAX request started."); }).ajaxStop(function() { console.log("All AJAX requests finished."); });
This example logs messages to the console when an AJAX request starts and when all AJAX requests are finished.
🎉 Conclusion
The jQuery.ajaxStop()
method is a powerful tool for managing the completion of multiple AJAX requests. Whether you need to hide a loading indicator, update the UI, perform cleanup tasks, or chain it with other AJAX events, this method provides a seamless way to enhance your web applications.
By mastering jQuery.ajaxStop()
, you can create more responsive and user-friendly interfaces.
👨💻 Join our Community:
Author
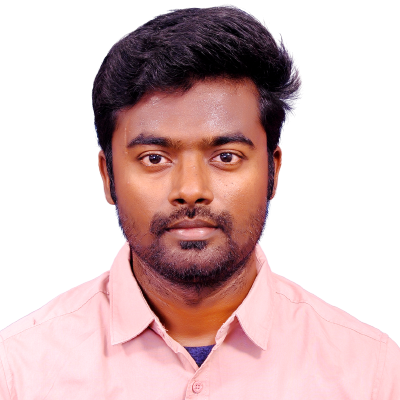
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .ajaxStop() Method), please comment here. I will help you immediately.