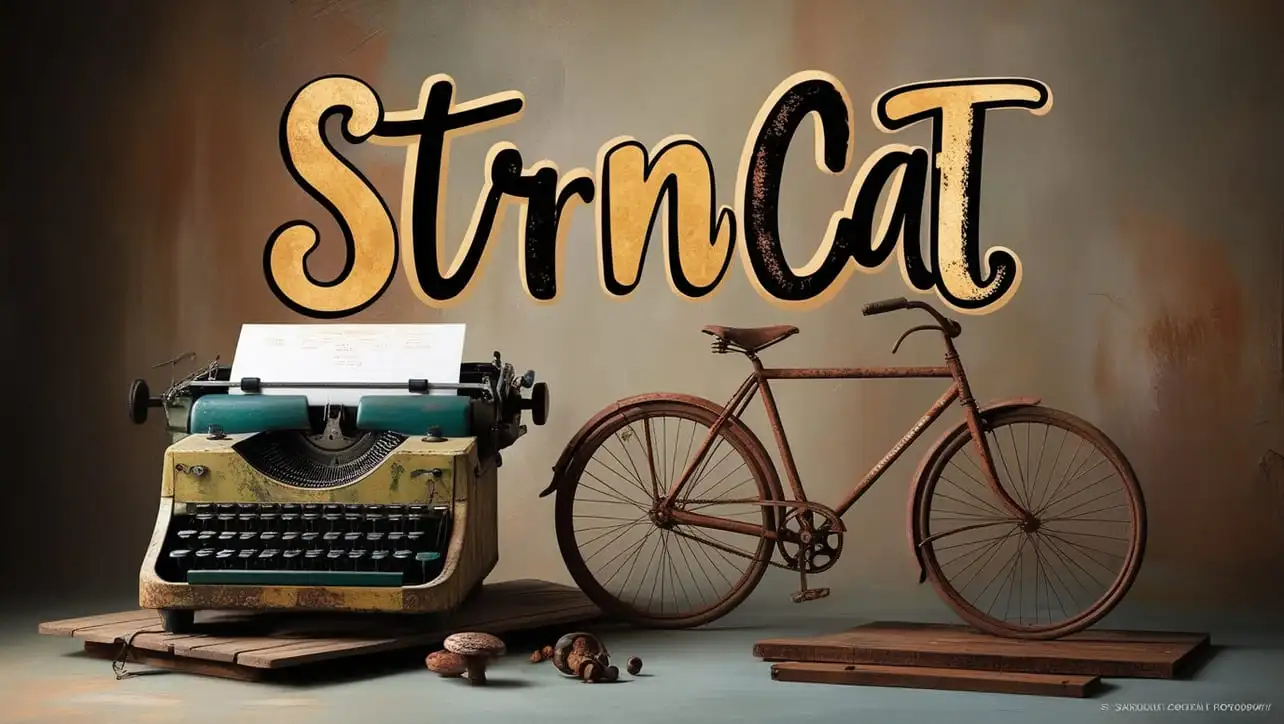
C++ String Functions
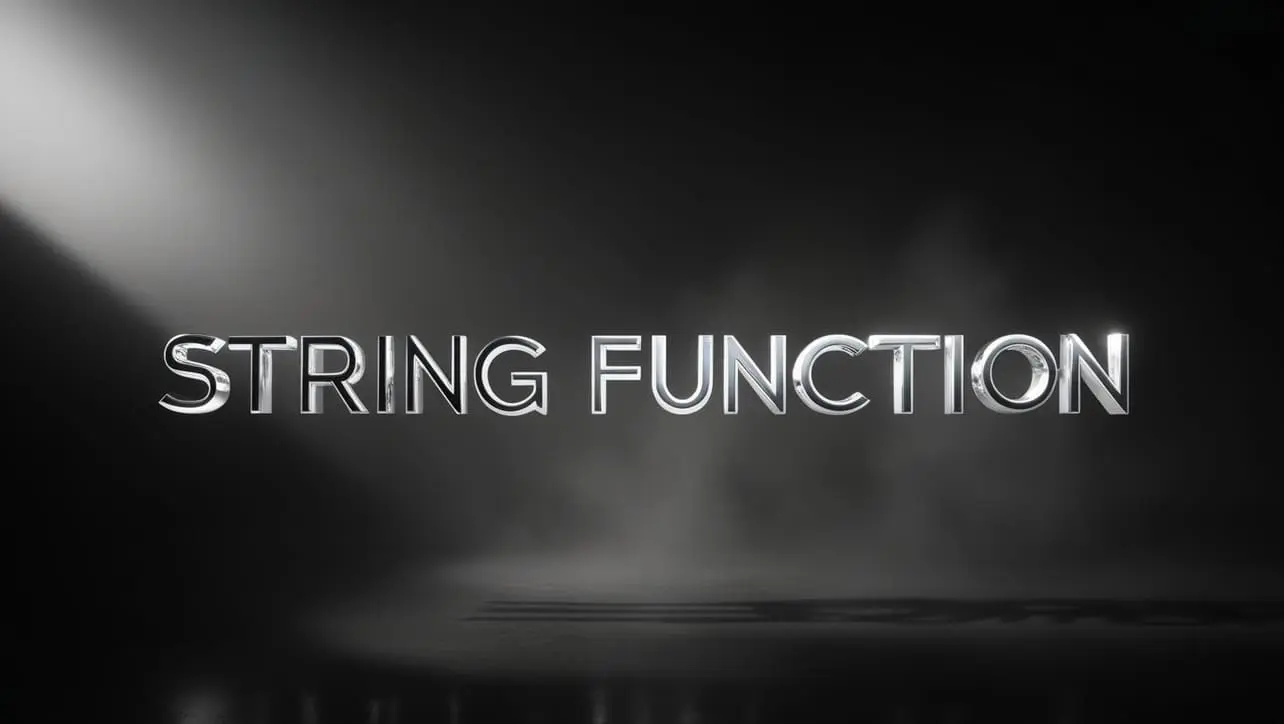
Photo Credit to CodeToFun
Introduction
C++ provides a rich set of built-in functions for working with strings.
These functions enable you to perform various operations on strings, including manipulation, comparison, and searching.
In this reference guide, we'll explore some commonly used C++ string functions to enhance your understanding and usage of strings in C++.
Table of Contents
Some of the commonly used string functions in C++ includes:
Functions | Explanation |
---|---|
find() | Used to locate the position of a substring within a string, returning the index of the first occurrence or npos if not found. |
strcat() | Used to concatenate (append) one C-string to the end of another. |
strchr() | Searches for the first occurrence of a character in a C-style string and returns a pointer to that character or null if not found. |
strcmp() | Used to compare two strings lexically, returning an integer that indicates whether the first string is less than, equal to, or greater than the second string. |
strcoll() | Used for string comparison based on the current locale, providing localized sorting and collation capabilities. |
strcpy() | Used to copy a C-style string (character array) from a source to a destination, providing a fundamental mechanism for string manipulation. |
strcspn() | Returns the length of the initial segment of a string that consists of characters not found in a specified set. |
strerror() | Retrieves the descriptive error message corresponding to the value of errno. |
strlen() | Returns the length of a C-style string, measuring the number of characters before the null terminator. |
strncat() | Concatenates a specified number of characters from one string to another, ensuring a defined limit to prevent buffer overflow. |
strncmp() | Compares specified numbers of characters between two strings and returns an integer indicating their relationship. |
strncpy() | Copies a specified number of characters from one string to another, providing control over the length of the copied substring. |
strpbrk() | Searches a string for any character in a specified set, returning a pointer to the first occurrence or a null pointer if none is found. |
strrchr() | Returns a pointer to the last occurrence of a specified character in a given string. |
strspn() | Returns the length of the initial segment of a string consisting of only characters that match those in another string. |
Usage Tips
Explore the following tips to make the most out of C++ string functions:
- Efficiency: Consider the efficiency of different functions when performing operations on large strings.
- Error Handling: Pay attention to return values and error conditions, especially in functions that search or compare strings.
- C++11 and Beyond: Explore modern C++ features for additional string manipulation capabilities introduced in C++11 and later.
- Read Documentation: Refer to the official C++ documentation for detailed information on each string function.
Example
// Example: Using C++ string functions
#include <iostream>
#include <string>
int main() {
std::string originalString = "Hello, C++ Strings!";
// Use various string functions
size_t length = originalString.length();
std::string substring = originalString.substr(7, 3);
int position = originalString.find("C++");
originalString.replace(position, 3, "C++17");
// Output the results
std::cout << "Original String: " << originalString << std::endl;
std::cout << "Length: " << length << std::endl;
std::cout << "Substring: " << substring << std::endl;
return 0;
}
Testing the Program
Original String: Hello, C++17 Strings! Length: 19 Substring: C++
How the Program Works
This example showcases the use of length(), substr(), find(), and replace() functions to manipulate a C++ string. Experiment with different functions and parameters to see the diverse capabilities of C++ string functions.
Conclusion
This reference guide provides an overview of essential C++ string functions. By incorporating these functions into your code, you can efficiently manipulate and analyze strings in various ways. Experiment with these functions to discover their versatility and enhance your C++ programming skills.
If you have any questions or want to delve deeper into a specific function, please comment on this page.
Join our Community:
Author
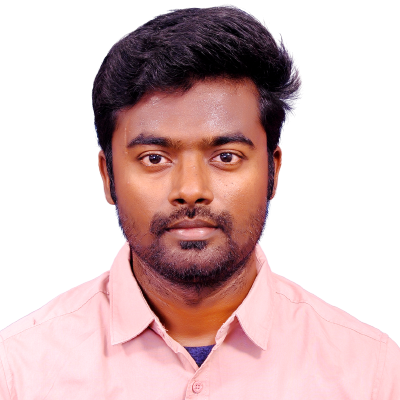
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String Functions), please comment here. I will help you immediately.