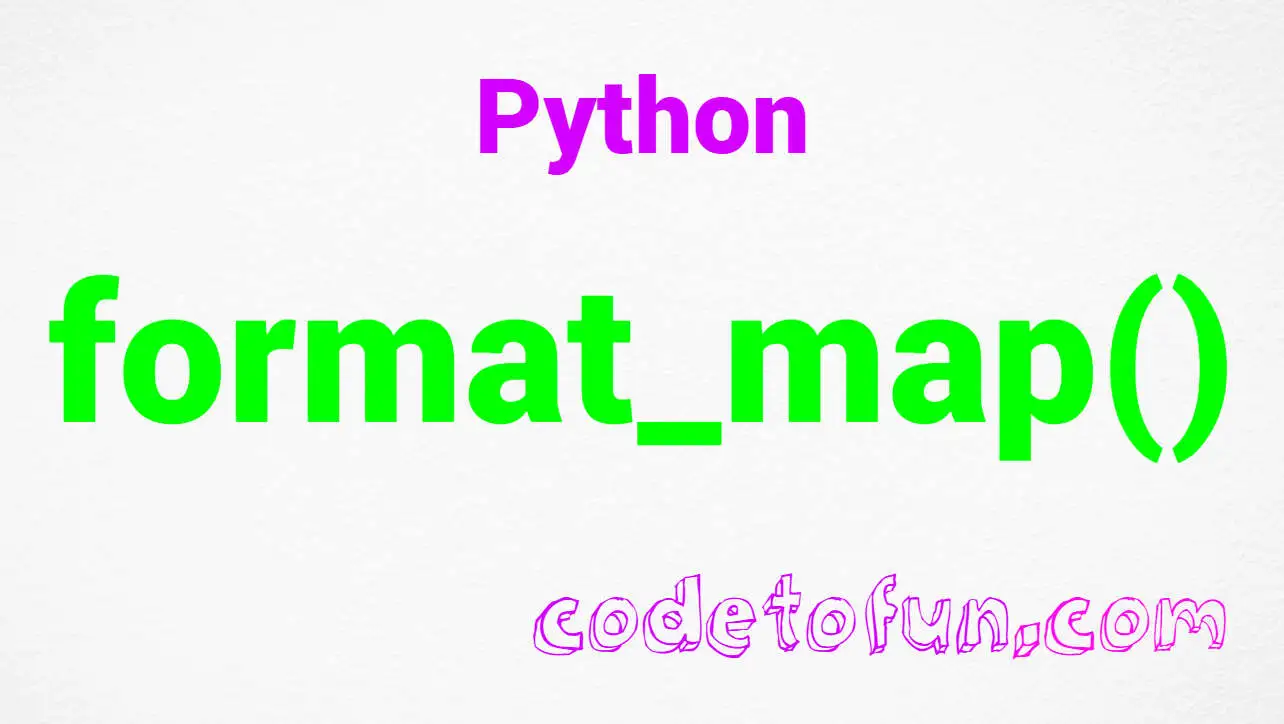
Python Basic
Python String Methods
Python string index() Method
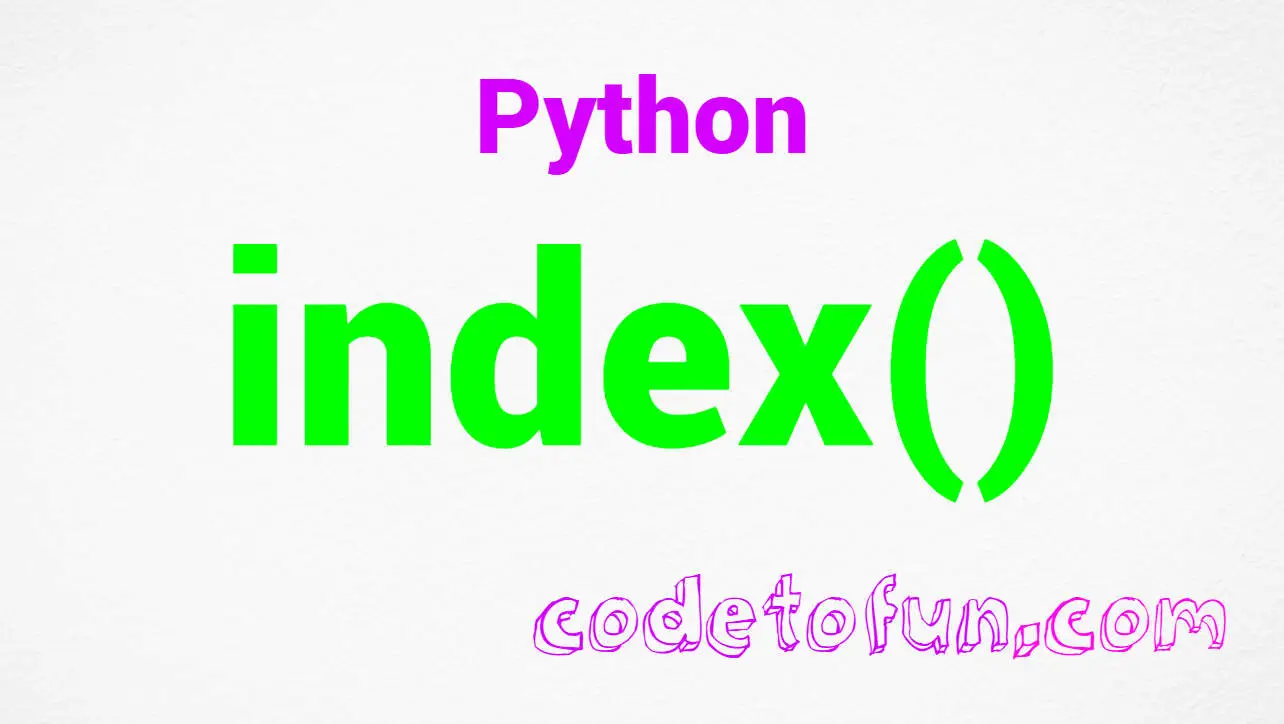
Photo Credit to CodeToFun
đ Introduction
In Python, strings are a fundamental data type, and manipulating them is a common programming task.
The index()
method is a built-in string method that allows you to find the index of the first occurrence of a substring within a string.
In this tutorial, we'll explore the syntax, usage, and considerations of the index()
method in Python.
đĄ Syntax
The syntax for the index()
method is as follows:
string.index(substring, start, end)
- string: The string in which the search will be performed.
- substring: The substring that you want to find within the string.
- start (optional): The starting index for the search. If not specified, the search starts from the beginning of the string.
- end (optional): The ending index for the search. If not specified, the search extends to the end of the string.
đ Example 1
Let's explore an example to understand how the index()
method works.
text = "Hello, World!"
result1 = text.index("World")
print("Example 1:", result1)
đģ Testing the Program
Example 1: 7
đ§ How the Program Works
In Example 1, the index()
method searches for the substring "World" within the string "Hello, World!" and returns the index of the first occurrence, which is 7.
đ Example 2
Let's explore another example to understand how the index()
method works.
text = "Hello, World!"
try:
result2 = text.index("Python")
print("Example 2:", result2)
except ValueError as e:
print("Example 2:", e)
đģ Testing the Program
Example 2: substring not found
đ§ How the Program Works
In Example 2, the substring Python is not found, and the method raises a ValueError. It's important to handle this exception when using index()
to avoid program termination.
đ Example 3
You can specify the start and end parameters to narrow down the search within a specific portion of the string, similar to the find() method.
text = "Hello, Hello, World!"
result = text.index("Hello", 7, 15)
print(result)
đģ Testing the Program
7
đ§ How the Program Works
In this example, the search is restricted to the substring between indices 7 and 15, resulting in the index 9 for the first occurrence of Hello.
âŠī¸ Return Value
The index()
method returns the index of the first occurrence of the substring within the string. If the substring is not found, it raises a ValueError.
This behavior is different from the find() method, which returns -1 when the substring is not found.
đ Common Use Cases
The index()
method is useful when you need to locate the position of a specific substring within a string, similar to find()
However, it provides a different error-handling mechanism by raising an exception instead of returning -1.
đ Notes
- Like find(), the
index()
method is case-sensitive. If a case-insensitive search is needed, consider converting the string and the substring to lowercase or uppercase using lower() or upper() before callingindex()
.
đĸ Optimization
The index()
method is already optimized for efficiency. However, for large strings and frequent searches, consider storing the result in a variable if you need to use it multiple times to avoid redundant computations.
đ Conclusion
The index()
method in Python is a powerful tool for locating substrings within strings. It provides flexibility with optional parameters and is a valuable asset in string manipulation tasks.
When using index()
, it's crucial to handle potential ValueError exceptions to ensure the robustness of your code.
Feel free to experiment with different strings and substrings to deepen your understanding of the index()
method. Happy coding!
đ¨âđģ Join our Community:
Author
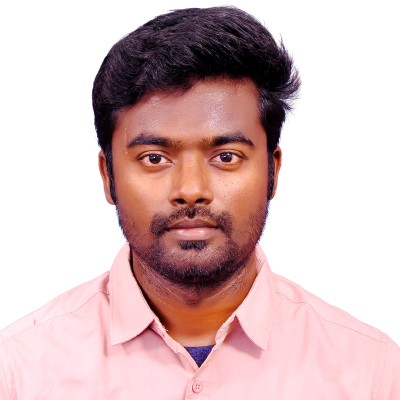
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python string index() Method), please comment here. I will help you immediately.