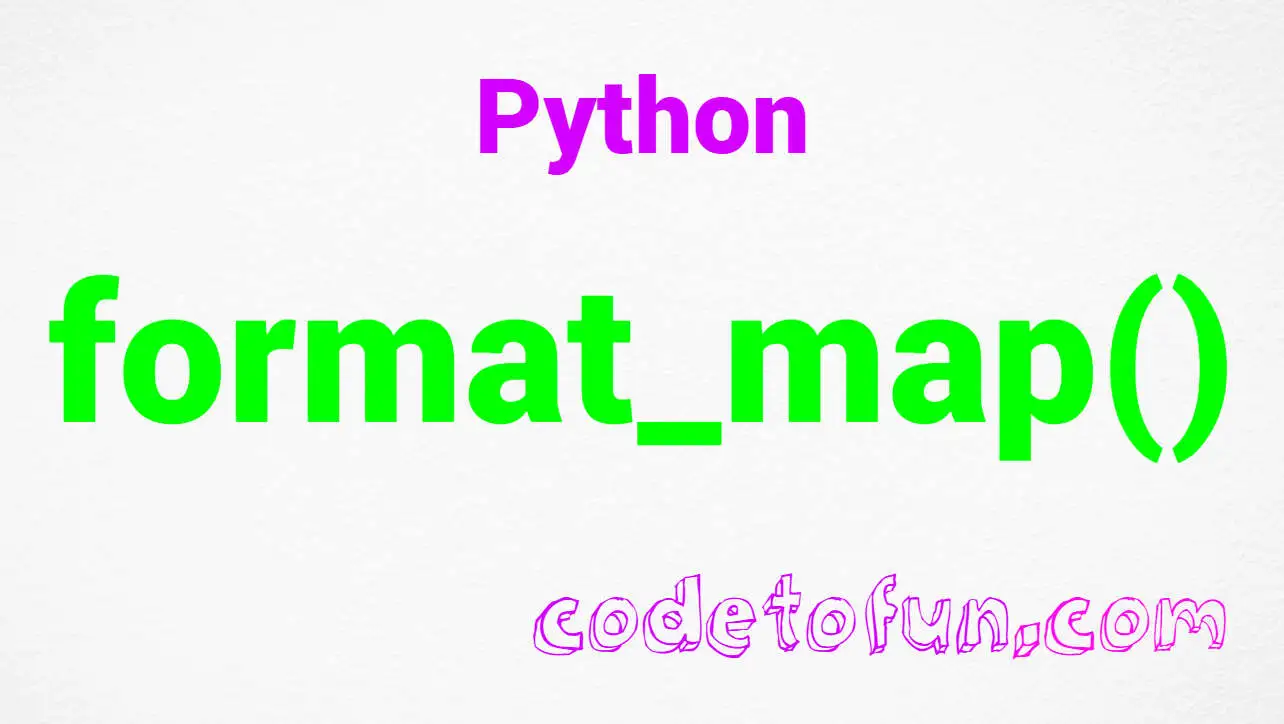
Python Basic
Python String Methods
Python string find() Method
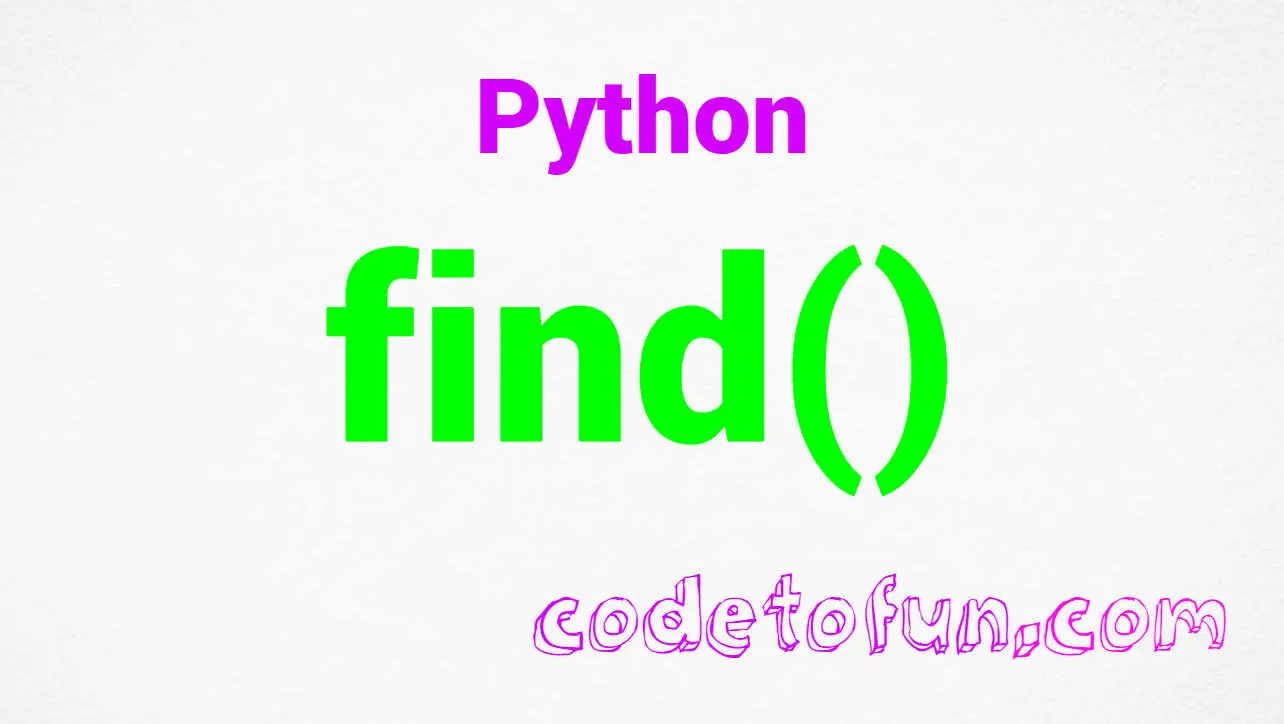
Photo Credit to CodeToFun
đ Introduction
In Python, working with strings is a common and fundamental task.
The find()
method is a built-in string method that allows you to search for a substring within a string and returns the index of the first occurrence of the substring.
In this tutorial, we'll explore the usage and functionality of the find()
method in Python.
đĄ Syntax
The syntax for the find()
method is as follows:
string.find(substring, start, end)
- string: The string in which the search will be performed.
- substring: The substring that you want to find within the string.
- start (Optional): The starting index for the search. If not specified, the search starts from the beginning of the string.
- end (Optional): The ending index for the search. If not specified, the search extends to the end of the string.
đ Example
Let's dive into some examples to illustrate how the find()
method works.
text = "Hello, World!"
result1 = text.find("World")
print("Example 1:", result1)
đģ Testing the Program
Example 1: 7
đ§ How the Program Works
In Example 1, the find()
method searches for the substring "World" within the string "Hello, World!" and returns the index of the first occurrence, which is 7.
đ Example
This Python program searches for the substring Python in the text Hello, World! and prints the result of the find operation, indicating that Python is not found in the text.
text = "Hello, World!"
result2 = text.find("Python")
print("Example 2:", result2) (not found)
đģ Testing the Program
Example 2: -1
đ§ How the Program Works
In Example 2, the substring Python is not found, so the method returns -1.
âŠī¸ Return Value
The find()
method returns the index of the first occurrence of the substring. If the substring is not found, it returns -1.
đ Common Use Cases
The find()
method is particularly useful when you need to determine the position of a specific substring within a larger string. It's commonly employed in scenarios where you want to extract or manipulate portions of text.
đ Notes
- The
find()
method is case-sensitive, meaning that it distinguishes between uppercase and lowercase letters. - If you need a case-insensitive search, you can convert the string and the substring to lowercase (or uppercase) using the lower() or upper() methods before calling
find()
.
Using Start and End Parameters
You can specify the start and end parameters to narrow down the search within a specific portion of the string.
text = "Hello, Hello, World!"
result = text.find("Hello", 7, 15)
print(result)
đģ Testing the Program
7
đ§ How the Program Works
In this example, the search is restricted to the substring between indices 7 and 15, resulting in the index 9 for the first occurrence of "Hello."
đĸ Optimization
The find()
method is already optimized for efficiency. However, for large strings and frequent searches, consider storing the result in a variable if you need to use it multiple times to avoid redundant computations.
đ Conclusion
The find()
method in Python is a powerful tool for searching for substrings within strings. It provides flexibility with optional parameters and is a valuable asset in string manipulation tasks.
Feel free to experiment with different strings and substrings to deepen your understanding of the find()
method. Happy coding!
đ¨âđģ Join our Community:
Author
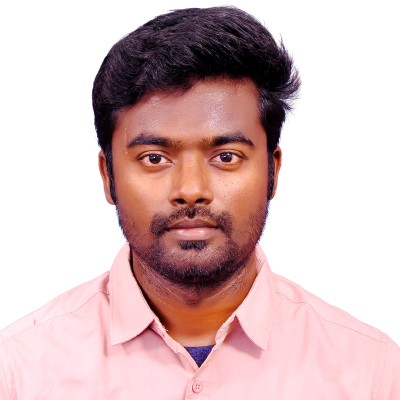
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python string find() Method), please comment here. I will help you immediately.